Easy Fix for the "Unexpected NUL in Input" Error in Go Programming

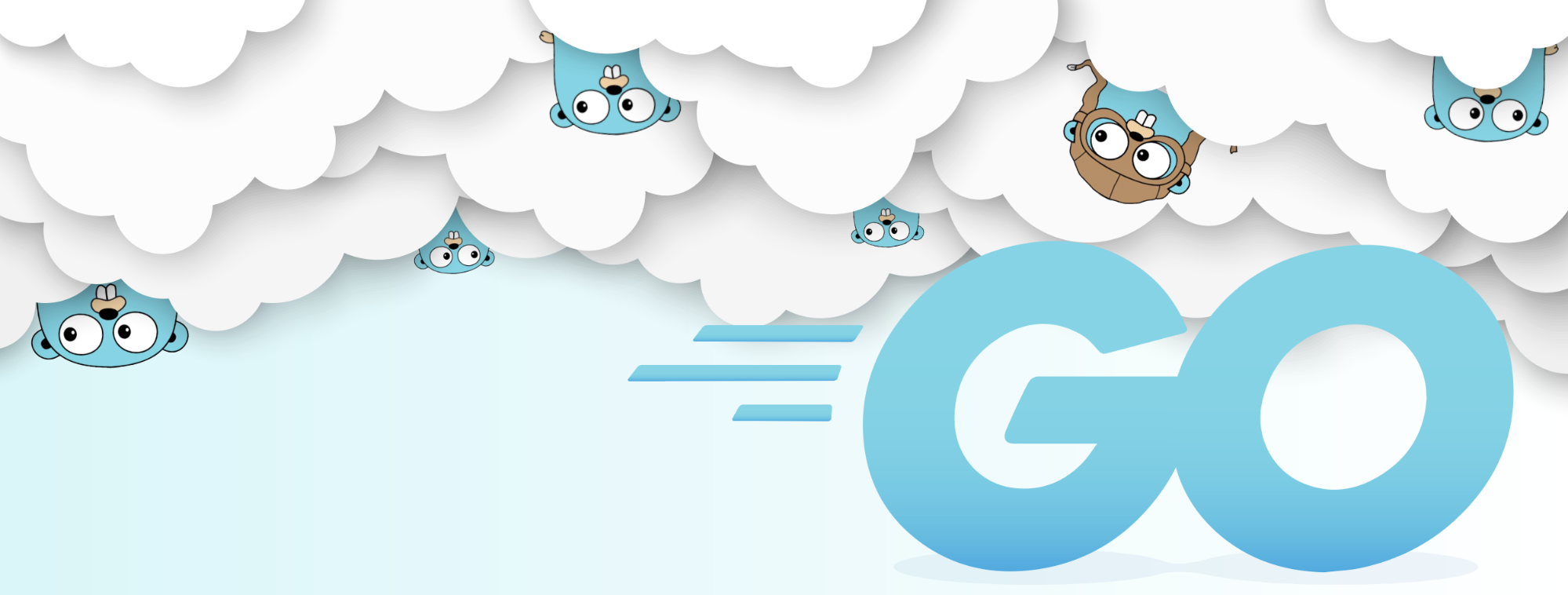
When learning Go (Golang), you may encounter an unexpected error called "Unexpected NUL in input"
. This error can be confusing for beginners, but its root cause and solution are relatively simple. In this article, we'll explore what causes the error, why it occurs, and how changing the encoding to "UTF-8"
solves the problem. We'll also dive into an example to show you how to resolve it in practice.
Understanding the “Unexpected NUL in Input” Error
The "Unexpected NUL in input"
error typically occurs when Go reads a file or input that contains NULL
bytes (also represented as \0
). In text files, a NULL
byte is often considered an invalid character, especially when dealing with text encodings that don't expect such characters. Go, being strict about input and file processing, raises this error to signal that something unexpected has happened.
A common cause of this issue is when you're working with files that use a non-UTF-8 encoding (e.g., UTF-16, ISO-8859-1). In these encodings, extra bytes like NULL
may be present, which Go’s standard library doesn’t handle well for text processing.
Example Scenario
Imagine you're reading a text file in Go, and that file happens to be encoded in UTF-16 or another encoding. Here’s an example of how such a scenario could lead to the "Unexpected NUL in input"
error:
Problematic Code
package main
import (
"fmt"
"io/ioutil"
"log"
)
func main() {
// Attempt to read a file (assumed to be non-UTF-8 encoded)
content, err := ioutil.ReadFile("example.txt")
if err != nil {
log.Fatal(err)
}
fmt.Println(string(content))
}
In this code, ReadFile
reads a file (example.txt
) and attempts to print its content. However, if example.txt
contains NULL
bytes, you might encounter the error:
panic: unexpected NUL in input
This error occurs because the file is likely encoded in a format like UTF-16, which is not handled natively as text by Go's UTF-8-based string functions.
Why Changing the Encoding to UTF-8 Solves the Issue
Go expects input to be in UTF-8
, which is the most widely used encoding for text on the web and in programming. When you change the file’s encoding to UTF-8
, it removes or replaces the NULL
bytes with valid UTF-8 characters, allowing Go to read and process the file without encountering unexpected characters.
Here’s how you can convert a file to UTF-8 using popular text editors or command-line tools:
Using Visual Studio Code (VSCode): Open the file, click on the bottom-right corner where the encoding is displayed (it may say
UTF-16
or something else), and selectUTF-8
. Then save the file.Using Notepad++: Open the file, go to
Encoding
>Convert to UTF-8
, and save the file.
Once the file is saved in UTF-8, Go can process it without any issues.
Fixing the Error in Go
If you want to handle this error programmatically in Go, one option is to convert the file content to UTF-8
within your Go program itself. You can use Go’s "
golang.org/x/text/encoding/unicode
"
package to decode files that are not UTF-8 encoded.
Here’s an example of how to handle a UTF-16 encoded file in Go:
Corrected Code Using UTF-16 Decoding
package main
import (
"fmt"
"io/ioutil"
"log"
"golang.org/x/text/encoding/unicode"
"golang.org/x/text/transform"
"bytes"
)
func main() {
// Read the non-UTF-8 encoded file
content, err := ioutil.ReadFile("example.txt")
if err != nil {
log.Fatal(err)
}
// Convert from UTF-16 to UTF-8
utf16Decoder := unicode.UTF16(unicode.LittleEndian, unicode.UseBOM).NewDecoder()
decodedContent, _, err := transform.String(utf16Decoder, string(content))
if err != nil {
log.Fatal(err)
}
fmt.Println(decodedContent)
}
Explanation:
unicode.UTF16(): This function is used to create a decoder for UTF-16 encoded files. It automatically detects the file’s byte order mark (BOM) and adjusts accordingly.
transform.String(): This function applies the UTF-16 decoder and converts the content to UTF-8, making it readable by Go.
By using this approach, you can ensure that your Go application can process files with different encodings without running into the "Unexpected NUL in input"
error.
Conclusion
The "Unexpected NUL in input"
error in Go is a result of encountering unexpected NULL
bytes in input files, which usually occur due to non-UTF-8 encodings like UTF-16. Converting your files to UTF-8
solves the issue, but you can also programmatically handle it by decoding the file’s content within Go. By understanding the root cause and using the right encoding, you can avoid this error and build more robust Go applications.
Subscribe to my newsletter
Read articles from Logeshwaran N directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Logeshwaran N
Logeshwaran N
I’m a dedicated Cloud and Backend Developer with a strong passion for building scalable solutions in the cloud. With expertise in AWS, Docker, Terraform, and backend technologies, I focus on designing, deploying, and maintaining robust cloud infrastructure. I also enjoy developing backend systems, optimizing APIs, and ensuring high performance in distributed environments. I’m continuously expanding my knowledge in backend development and cloud security. Follow me for in-depth articles, hands-on tutorials, and tips on cloud architecture, backend development, and DevOps best practices.