Know Your Git Commands: A Comprehensive Git Checklist for Developers

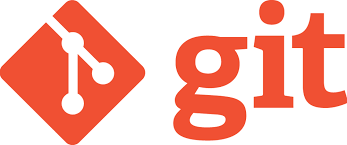
Git is an essential tool for developers, enabling efficient collaboration, version control, and project management. Whether you're a beginner or looking to refine your Git skills, this checklist covers all the important concepts and commands you need to master to use Git professionally.
Basic Concepts
1. Repository (Repo)
A repository is a directory that contains your project's files along with a special .git
folder, which includes all the version history and metadata. It's the core of any Git project.
Why It's Important: The repository is where all your code and its history are stored, enabling you to track changes and collaborate with others.
Example: Creating a new repository with
git init
initializes a.git
folder in your project directory.
2. Version Control
Version control is a system that records changes to files over time so that you can recall specific versions later. Git is a distributed version control system, meaning each developer has a complete copy of the repository.
Why It's Important: It allows multiple people to work on the same codebase without overwriting each other's work.
Example: Using Git commands to commit changes and revert to previous states.
3. Commit
A commit is a snapshot of your repository at a specific point in time. It records changes to one or more files and includes a message describing the changes.
Why It's Important: Commits form the building blocks of your project's history.
Example:
git commit -m "Add new feature"
4. Branch
A branch is a parallel version of your repository. It allows you to work on different features or fixes independently.
Why It's Important: Branches enable isolated development, preventing unfinished features from affecting the main codebase.
Example: Creating a new branch with
git branch feature-xyz
5. Main/Master Branch
The main or master branch is the default primary branch in Git repositories. It typically contains the stable, production-ready code.
Why It's Important: It's the central point where all other branches eventually merge back.
Example: Checking out the main branch with
git checkout main
6. HEAD
HEAD is a pointer to the current branch reference, essentially pointing to the last commit in the checked-out branch.
Why It's Important: It determines where you are in the repository's history.
Example: Viewing the current HEAD with
git log -1
7. Index (Staging Area)
The index or staging area is where you place changes you want to include in the next commit.
Why It's Important: It allows you to control which changes are committed.
Example: Adding files to the staging area with
git add filename
8. Working Directory
The working directory is your local checkout of the repository files where you make changes.
Why It's Important: It's where you work on your code before staging and committing.
Example: Editing files in your code editor.
9. Remote Repository
A remote repository is a version of your project hosted on a server or cloud service like GitHub, GitLab, or Bitbucket.
Why It's Important: Enables collaboration by allowing multiple people to push and pull changes.
Example: Cloning a remote repository with
git clone https://github.com/user/repo.git
10. Clone
To clone is to create a local copy of a remote repository.
Why It's Important: It sets up a local environment to work on the project.
Example:
git clone <repository-url>
11. Push
Pushing uploads your committed changes from your local repository to a remote repository.
Why It's Important: Shares your work with others and updates the remote repository.
Example:
git push origin main
12. Pull
Pulling fetches changes from a remote repository and merges them into your local repository.
Why It's Important: Keeps your local repository up-to-date with the remote.
Example:
git pull origin main
13. Fetch
Fetching downloads changes from the remote repository but doesn't merge them.
Why It's Important: Allows you to review changes before integrating them.
Example:
git fetch origin
14. Merge
Merging combines changes from one branch into another.
Why It's Important: Integrates features or fixes into the main codebase.
Example:
git merge feature-branch
15. Rebase
Rebasing moves or combines a sequence of commits to a new base commit.
Why It's Important: Maintains a linear project history.
Example:
git rebase main
16. Conflict
A conflict occurs when Git cannot automatically merge changes.
Why It's Important: Requires manual resolution to integrate changes.
Example: Merge conflicts during
git merge
17. Resolve Conflicts
Resolving conflicts involves manually fixing the conflicting parts of code and completing the merge.
Why It's Important: Ensures that the code integrates correctly.
Example: Editing files marked with conflict markers
<<<<<<<
,=======
,>>>>>>>
18. Checkout
Checking out switches between branches or restores files.
Why It's Important: Allows you to work on different features or revert files.
Example:
git checkout feature-branch
19. Reset
Resetting moves the current branch to a specified state.
Why It's Important: Can undo changes in your working directory and staging area.
Example:
git reset --hard HEAD~1
20. Revert
Reverting creates a new commit that undoes changes from a previous commit.
Why It's Important: Safely undoes changes without altering history.
Example:
git revert <commit-hash>
21. Cherry-pick
Cherry-picking applies changes from specific commits to your current branch.
Why It's Important: Useful for applying specific fixes without merging entire branches.
Example:
git cherry-pick <commit-hash>
22. Tag
A tag marks a specific point in history, often used for releases.
Why It's Important: Identifies important commits.
Example:
git tag -a v1.0 -m "Version 1.0 release"
23. Stash
Stashing temporarily saves changes not ready to be committed.
Why It's Important: Allows you to switch branches without losing work.
Example:
git stash
andgit stash apply
24. Fork
To fork is to create a personal copy of someone else's repository.
Why It's Important: Enables you to propose changes to the original project.
Example: Clicking "Fork" on GitHub to create a forked repository.
25. Pull Request (PR) / Merge Request (MR)
A pull request or merge request is a request to merge your changes into another branch or repository.
Why It's Important: Facilitates code review and collaborative development.
Example: Opening a PR on GitHub after pushing changes.
26. Blame
Blame annotates each line in a file with information about the last commit that modified it.
Why It's Important: Helps track changes and identify responsible parties.
Example:
git blame filename
27. Log
The log displays a history of commits.
Why It's Important: Allows you to review project history.
Example:
git log
28. Diff
Diff shows differences between commits, branches, or files.
Why It's Important: Helps understand changes made.
Example:
git diff HEAD~1 HEAD
29. Squash
Squashing combines multiple commits into one.
Why It's Important: Cleans up commit history before merging.
Example:
git rebase -i HEAD~3
and selectingsquash
30. Submodules
Submodules allow you to include other Git repositories within a repository.
Why It's Important: Manages dependencies in separate repositories.
Example:
git submodule add <repository-url>
31. Git Hooks
Git hooks are scripts that run automatically at certain points in Git's execution.
Why It's Important: Automates tasks like code linting or tests before commits.
Example: Placing scripts in the
.git/hooks
directory.
32. .gitignore
The .gitignore file specifies intentionally untracked files that Git should ignore.
Why It's Important: Prevents unnecessary files from cluttering the repository.
Example: Adding
node_modules/
to.gitignore
33. Fast-forward Merge
A fast-forward merge moves the branch pointer forward because there's a linear path from the current commit to the target commit.
Why It's Important: Simplifies history when no diverging commits exist.
Example: Merging a feature branch that is ahead of the main branch.
34. Detached HEAD
A detached HEAD occurs when HEAD points directly to a commit instead of a branch.
Why It's Important: Useful for inspecting or modifying past states without affecting branches.
Example:
git checkout <commit-hash>
35. Amend
Amending modifies the most recent commit.
Why It's Important: Corrects mistakes without adding new commits.
Example:
git commit --amend -m "Corrected commit message"
36. Shallow Clone
A shallow clone clones only the latest commits of a repository.
Why It's Important: Saves time and space when you don't need the full history.
Example:
git clone --depth 1 <repository-url>
37. Bare Repository
A bare repository contains the version history but no working directory.
Why It's Important: Used as a central repository for collaboration.
Example: Creating a bare repository with
git init --bare
38. Git Flow
Git Flow is a branching model that defines a strict workflow for managing releases and hotfixes.
Why It's Important: Provides a consistent method for managing complex projects.
Example: Using feature, develop, release, and hotfix branches.
39. Git Bisect
Git bisect uses binary search to find the commit that introduced a bug.
Why It's Important: Efficiently isolates problematic code.
Example:
git bisect start
,git bisect bad
,git bisect good
40. SHA-1 Hash
A SHA-1 hash uniquely identifies commits.
Why It's Important: Ensures integrity and allows precise references.
Example:
commit 1a2b3c4d5e6f7g8h9i0j...
Advanced Concepts
41. Reflog
Reflog records updates to the tips of branches and other references.
Why It's Important: Helps recover lost commits.
Example:
git reflog
42. Git Workflow
Git workflow refers to the methods for using Git in projects, such as feature branching or forking workflows.
Why It's Important: Defines how teams collaborate.
Example: Adopting the "GitHub Flow" for continuous deployment.
43. Git Subtree
Git subtree allows you to include one repository inside another as a sub-directories.
Why It's Important: Simplifies managing projects with dependencies.
Example:
git subtree add --prefix=dir <repository-url> main
44. Sparse Checkout
Sparse checkout allows you to check out only a portion of the repository.
Why It's Important: Useful for working with large repositories.
Example: Configuring sparse checkout with
git sparse-checkout init
45. Signed Commits
Signed commits use cryptographic signatures to verify authorship.
Why It's Important: Enhances security and trust in contributions.
Example:
git commit -S -m "Signed commit message"
46. Git Garbage Collection (gc)
Git garbage collection cleans up unnecessary files and optimizes the repository.
Why It's Important: Maintains repository performance.
Example:
git gc
47. Worktree
Worktrees allow multiple working directories associated with a single repository.
Why It's Important: Enables working on multiple branches simultaneously.
Example:
git worktree add ../new-worktree feature-branch
48. Git LFS (Large File Storage)
Git LFS manages large files by storing pointers in the repository while keeping the actual files in a separate location.
Why It's Important: Keeps repository sizes manageable.
Example: Tracking large files with
git lfs track "*.psd"
49. Interactive Rebase
Interactive rebase allows you to rewrite commit history by editing, reordering, or combining commits.
Why It's Important: Cleans up commits before sharing with others.
Example:
git rebase -i HEAD~5
Conclusion
Mastering Git's concepts is a journey that will significantly enhance your efficiency and collaboration in software development. By understanding and utilizing these concepts, you can manage your projects more effectively, contribute to team efforts seamlessly, and even guide others in best practices.
Remember, Git is more than just a tool—it's a critical component of modern software development workflows. Keep exploring, practicing, and applying these concepts to become a Git expert.
Happy coding!
Subscribe to my newsletter
Read articles from Gift Ayodele directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
