Understanding State Updates in React: Using `setCount` Inside and Outside `useEffect`

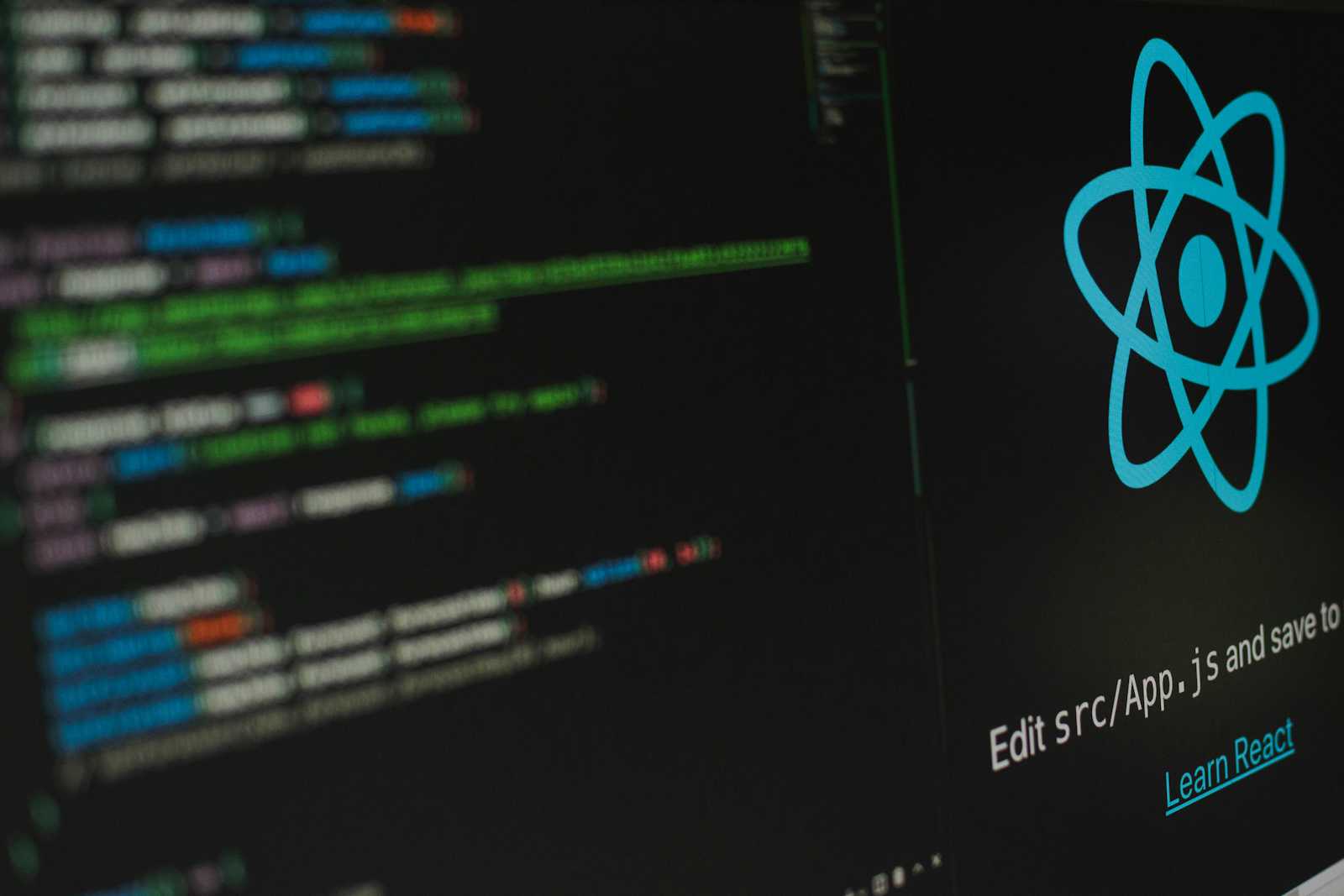
The difference in how you use setCount
inside and outside of useEffect
is due to how React handles state updates and closures.
Outside of useEffect
When you use setCount(count + 1)
outside of useEffect
, you are directly using the current value of count
to calculate the new state. This works fine in event handlers or other synchronous code because the state value is up-to-date at the time the function is called.
Example:
function handleClick() {
setCount(count + 1);
}
Inside useEffect
When you use setCount
inside useEffect
, you often need to ensure that you are working with the most recent state value. This is because useEffect
can run asynchronously and the state value captured by the closure might be stale.
Using the functional form of setCount
ensures that you always get the latest state value. The functional form takes a function as an argument, which receives the previous state and returns the new state.
Example:
useEffect(() => {
const interval = setInterval(() => {
setCount(prevCount => prevCount + 1);
}, 1000);
return () => clearInterval(interval);
}, []);
Detailed Explanation
State Updates and Closures:
When you define a function inside a component, it captures the state values at the time of its creation. This is known as a closure.
If you use
setCount(count + 1)
insideuseEffect
, it captures thecount
value at the timeuseEffect
is run. Ifcount
changes later, the captured value does not update, leading to potential bugs.
Functional Updates:
The functional form
setCount(prevCount => prevCount + 1)
ensures that the state update is based on the most recent state value.React guarantees that the function passed to
setCount
will receive the latest state value, even if the state has changed since the effect was created.Example Scenario
Consider a counter that increments every second:
import React, { useState, useEffect } from 'react'; function Counter() { const [count, setCount] = useState(0); useEffect(() => { const interval = setInterval(() => { setCount(prevCount => prevCount + 1); // Using functional form }, 1000); return () => clearInterval(interval); }, []); return <h1>Count: {count}</h1>; } export default Counter;
In this example:
setCount(prevCount => prevCount + 1)
ensures that the counter increments correctly every second.If you used
setCount(count + 1)
, thecount
value would be captured whenuseEffect
runs, and it would not update correctly on subsequent intervals.
Conclusion
Using the functional form of setCount
inside useEffect
ensures that you always work with the most recent state value, avoiding issues related to stale closures. This pattern is essential for ensuring consistent and correct state updates in asynchronous scenarios.
Subscribe to my newsletter
Read articles from Nitesh Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
