Exploring Queues: What They Are and How They Work

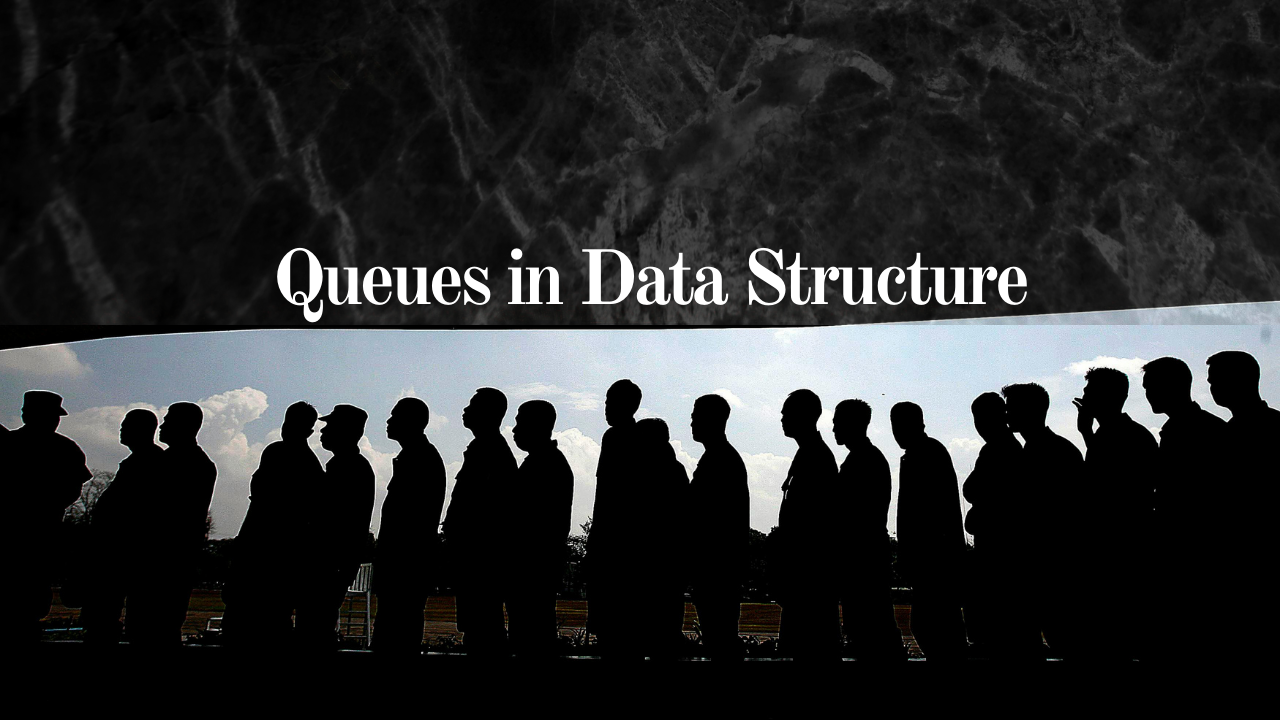
In our DSA series, we've covered various basic data structures, including linked lists, strings, arrays, and stacks. Now, it's time to look at queues, a basic data structure that follows the First-In-First-Out (FIFO) principle. Like a line at a ticket counter, queues ensure that the first element added is the first one removed, making them essential for managing tasks efficiently.
In this article, we will explore the main operations associated with queues, discuss their pros and cons, and highlight practical uses. Understanding queues will enhance your skills as you tackle programming challenges and improve data management in your projects.
What is a Queue?
A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. This means that the first element added to the queue will be the first one to be removed, just like in real-life queues, such as waiting in line for a ticket or service.
In technical terms, a queue can be defined as an abstract data type with two primary ends: one for insertion (rear) and one for deletion (front). Insertion takes place at the rear, while deletion occurs from the front.
Characteristics of a Queue:
Linear structure: Data is organized sequentially.
FIFO principle: The first item in is the first one out.
Two primary operations: Enqueue (insert) and Dequeue (remove).
Types of Queues
Simple Queue: This is the most basic type of queue that adheres to the FIFO principle, where elements are added at the rear and removed from the front. Simple queues are straightforward and are commonly used in scenarios like task scheduling and managing service requests, where maintaining the order of processing is essential. However, they do not support prioritization or reordering of elements once enqueued.
Circular Queue: In a circular queue, the last position is connected to the first, creating a circular structure. This design optimizes memory usage by allowing the reuse of empty spaces created by dequeued elements. As both the front and rear pointers wrap around when they reach the end, circular queues are particularly effective for fixed-size buffers, such as buffering data streams or managing resources in round-robin scheduling. This prevents the "overflow" condition that can occur in simple queues, even when unused slots are available.
Priority Queue: Unlike simple queues, a priority queue processes elements based on their assigned priority rather than their order of insertion. Each element is given a priority level, with higher-priority elements being processed first. This type of queue is vital in scenarios where certain tasks must take precedence, such as operating system task scheduling, network routing, and managing print jobs. Priority queues can be implemented using data structures like heaps or binary search trees to ensure efficient retrieval based on priority.
Double-ended Queue (Deque): A deque allows insertion and deletion at both ends, providing greater flexibility than a standard queue. This feature makes deques ideal for applications that require frequent additions or removals from either end. Deques can be implemented with arrays or linked lists and are commonly used in scenarios like undo operations in software, storing browsing history, or managing tasks in scheduling systems.
Key Operations on Queues
Here are the primary operations performed on a queue:
Enqueue (Insertion): The enqueue operation adds an element to the rear of the queue, allowing new tasks or data to be queued for future processing. For efficient queue operations in Python, the
collections.deque
is recommended over a list, as it offers faster append and pop operations. Here's a simple example usingcollections.deque
:from collections import deque # Create a queue queue = deque() # Enqueue elements queue.append('task1') queue.append('task2') queue.append('task3') print("Queue after enqueuing:", list(queue))
In this example, we create a queue and add three tasks using the
append()
method, which efficiently adds elements to the end of the deque.Dequeue (Deletion): The dequeue operation removes an element from the front of the queue, processing tasks in the order they were added and adhering to the FIFO principle. In
collections.deque
, elements can be removed efficiently using thepopleft()
method. Here's an example:from collections import deque # Create a queue queue = deque(['task1', 'task2', 'task3']) # Dequeue elements first_task = queue.popleft() print("Dequeued task:", first_task) print("Queue after dequeuing:", list(queue))
This snippet starts with three tasks in the queue and removes the first task using
popleft()
, demonstrating the dequeue operation.Front (Peek): The front operation retrieves the element at the front of the queue without removing it. This is useful for checking the next task to be processed. In Python, you can access the front element by indexing. Here's an example:
from collections import deque # Create a queue queue = deque(['task1', 'task2', 'task3']) # Peek at the front element front_task = queue[0] print("Front task:", front_task) print("Queue after peeking:", list(queue))
Here, accessing
queue[0]
allows us to see the first task without modifying the queue.Rear: The rear operation retrieves the last element in the queue without removing it. This helps to know the last task added. Here's an example:
from collections import deque # Create a queue queue = deque(['task1', 'task2', 'task3']) # Peek at the rear element rear_task = queue[-1] print("Rear task:", rear_task) print("Queue after peeking at the rear:", list(queue))
In this example,
queue[-1]
gives us the last task without altering the queue.isEmpty: The isEmpty operation checks if the queue is empty to prevent errors when accessing elements. In Python, this can be done using the
len()
function.from collections import deque # Check if the queue is empty if len(queue) == 0: print("The queue is empty.") else: print("The queue is not empty.")
isFull (for bounded queues): The isFull operation checks if a bounded queue has reached its maximum capacity. This is critical for queues where the size is predefined. In Python, you can implement this by comparing the current size to the maximum limit.
from collections import deque # Create a bounded queue with a maximum length of 3 max_length = 3 queue = deque(['task1', 'task2'], maxlen=max_length) # Function to check if the queue is full def is_full(queue): return len(queue) == queue.maxlen # Check if the queue is full if is_full(queue): print("The queue is full.") else: print("The queue is not full.")
This example creates a bounded queue with a maximum length of 3 and checks its fullness using the
is_full
function.
Advantages and Disadvantages of Queues
Advantages | Disadvantages |
Efficient in handling tasks sequentially | Limited access (only front and rear elements can be accessed) |
Simple to implement and understand | Fixed size if implemented using arrays, which can lead to memory wastage |
Useful for real-world scenarios like task scheduling and buffering | Operations like searching for an element are inefficient (O(n)) |
Follows a clear and predictable FIFO order | Can lead to performance issues in scenarios requiring random access |
Applications of Queues
Queues are used in various practical scenarios, including:
Task Scheduling: Operating systems use queues to schedule tasks for execution.
Breadth-First Search (BFS): Queues are used in graph algorithms like BFS to explore nodes layer by layer.
Print Spooling: In printers, tasks are added to a queue, and the printer processes them in order.
Handling Asynchronous Data: Queues are widely used in asynchronous communication like message queuing systems (e.g., RabbitMQ, Kafka).
Additional Resources
Queue Implementations in Python
Conclusion
Queues are a fundamental data structure in DSA, offering a straightforward way to manage tasks in a First-In-First-Out (FIFO) manner. Their efficiency in processing data makes them ideal for applications like task scheduling and buffering. However, it’s important to consider the limitations, such as fixed size in bounded queues and the inability to access elements randomly.
As we move forward, we'll delve into more advanced data structures that expand on these principles. For now, mastering queues is a key step in understanding how to handle data flow effectively in various programming scenarios.
Subscribe to my newsletter
Read articles from Keerthi Ravilla Subramanyam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Keerthi Ravilla Subramanyam
Keerthi Ravilla Subramanyam
Hi, I'm Keerthi Ravilla Subramanyam, a passionate tech enthusiast with a Master's in Computer Science. I love diving deep into topics like Data Structures, Algorithms, and Machine Learning. With a background in cloud engineering and experience working with AWS and Python, I enjoy solving complex problems and sharing what I learn along the way. On this blog, you’ll find articles focused on breaking down DSA concepts, exploring AI, and practical coding tips for aspiring developers. I’m also on a journey to apply my skills in real-world projects like predictive maintenance and data analysis. Follow along for insightful discussions, tutorials, and code snippets to sharpen your technical skills.