Understanding SQL Joins: Practical Examples You Need

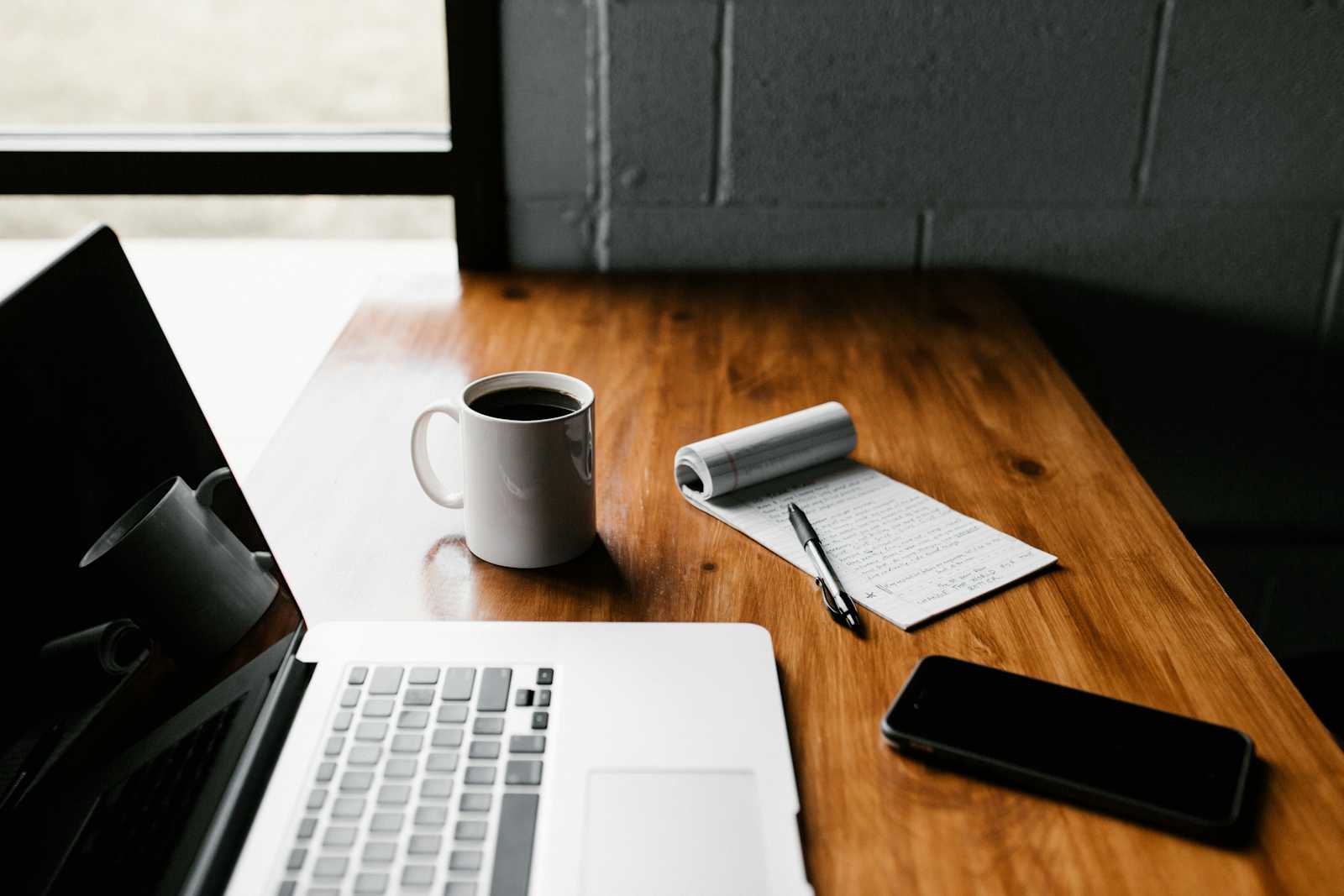
Understanding SQL Joins
SQL joins are used to combine rows from two or more tables based on a related column between them. Joins are essential for querying data across multiple tables in a relational database. There are several types of joins, each serving a different purpose. Let’s explore the most common types: INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL OUTER JOIN.
1. INNER JOIN
An INNER JOIN returns only the rows that have matching values in both tables. If there is no match, the row is not included in the result set.
Example:
Let’s say we have two tables, employees and departments.
employees:
employee_id | name | department_id |
1 | Alice | 1 |
2 | Bob | 2 |
3 | Charlie | 3 |
departments:
department_id | department_name |
1 | HR |
2 | IT |
4 | Marketing |
To get a list of employees along with their department names, we can use an INNER JOIN:
SELECT employees.name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
Result:
name | department_name |
Alice | HR |
Bob | IT |
2. LEFT JOIN (or LEFT OUTER JOIN)
A LEFT JOIN returns all rows from the left table and the matched rows from the right table. If there is no match, the result is NULL on the side of the right table.
Example:
SELECT employees.name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.department_id;
Result:
name | department_name |
Alice | HR |
Bob | IT |
Charlie | NULL |
3. RIGHT JOIN (or RIGHT OUTER JOIN)
A RIGHT JOIN returns all rows from the right table and the matched rows from the left table. If there is no match, the result is NULL on the side of the left table.
Example:
SELECT employees.name, departments.department_name
FROM employees
RIGHT JOIN departments ON employees.department_id = departments.department_id;
Result:
name | department_name |
Alice | HR |
Bob | IT |
NULL | Marketing |
4. FULL OUTER JOIN
A FULL OUTER JOIN returns all rows when there is a match in either left or right table. If there is no match, the result is NULL from the side where there is no match.
Example:
SELECT employees.name, departments.department_name
FROM employees
FULL OUTER JOIN departments ON employees.department_id = departments.department_id;
Result:
name | department_name |
Alice | HR |
Bob | IT |
Charlie | NULL |
NULL | Marketing |
5. CROSS JOIN
A CROSS JOIN returns the Cartesian product of the two tables, meaning it returns all possible combinations of rows from the tables.
Example:
SELECT employees.name, departments.department_name
FROM employees
CROSS JOIN departments;
Result:
name | department_name |
Alice | HR |
Alice | IT |
Alice | Marketing |
Bob | HR |
Bob | IT |
Bob | Marketing |
Charlie | HR |
Charlie | IT |
Charlie | Marketing |
6. SELF JOIN
A SELF JOIN is a regular join but the table is joined with itself.
Example:
Let’s say we have a table employees with a column manager_id that references the employee_id of the manager.
employees:
employee_id | name | manager_id |
1 | Alice | NULL |
2 | Bob | 1 |
3 | Charlie | 1 |
4 | David | 2 |
To get a list of employees along with their managers, we can use a SELF JOIN:
SELECT e1.name AS employee, e2.name AS manager
FROM employees e1
LEFT JOIN employees e2 ON e1.manager_id = e2.employee_id;
Result:
employee | manager |
Alice | NULL |
Bob | Alice |
Charlie | Alice |
David | Bob |
Conclusion
SQL joins are powerful tools for querying and combining data from multiple tables. Understanding how and when to use each type of join is crucial for effective database management and data analysis. By mastering joins, you can unlock the full potential of relational databases and perform complex queries with ease.
Subscribe to my newsletter
Read articles from Code Canvas directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Code Canvas
Code Canvas
Code Canvas: Unveiling the Wonders of AI, ML, Data, and Dev. With over 10+ years of experience in cloud computing and data and data integration, I specialize in helping businesses optimize their Data with AI and ML for maximum efficiency and scalability. My expertise spans across various cloud platforms including AWS, Azure, and Google Cloud, as well as database technologies like Python, Docker, Kube, SQL, NoSQL, and data warehousing solutions.