The Ultimate MERN Stack Roadmap for Developers
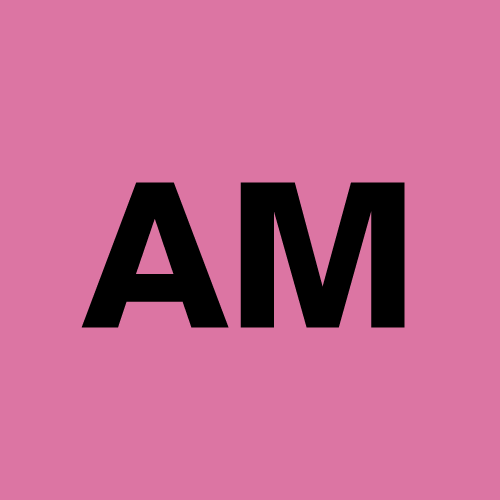
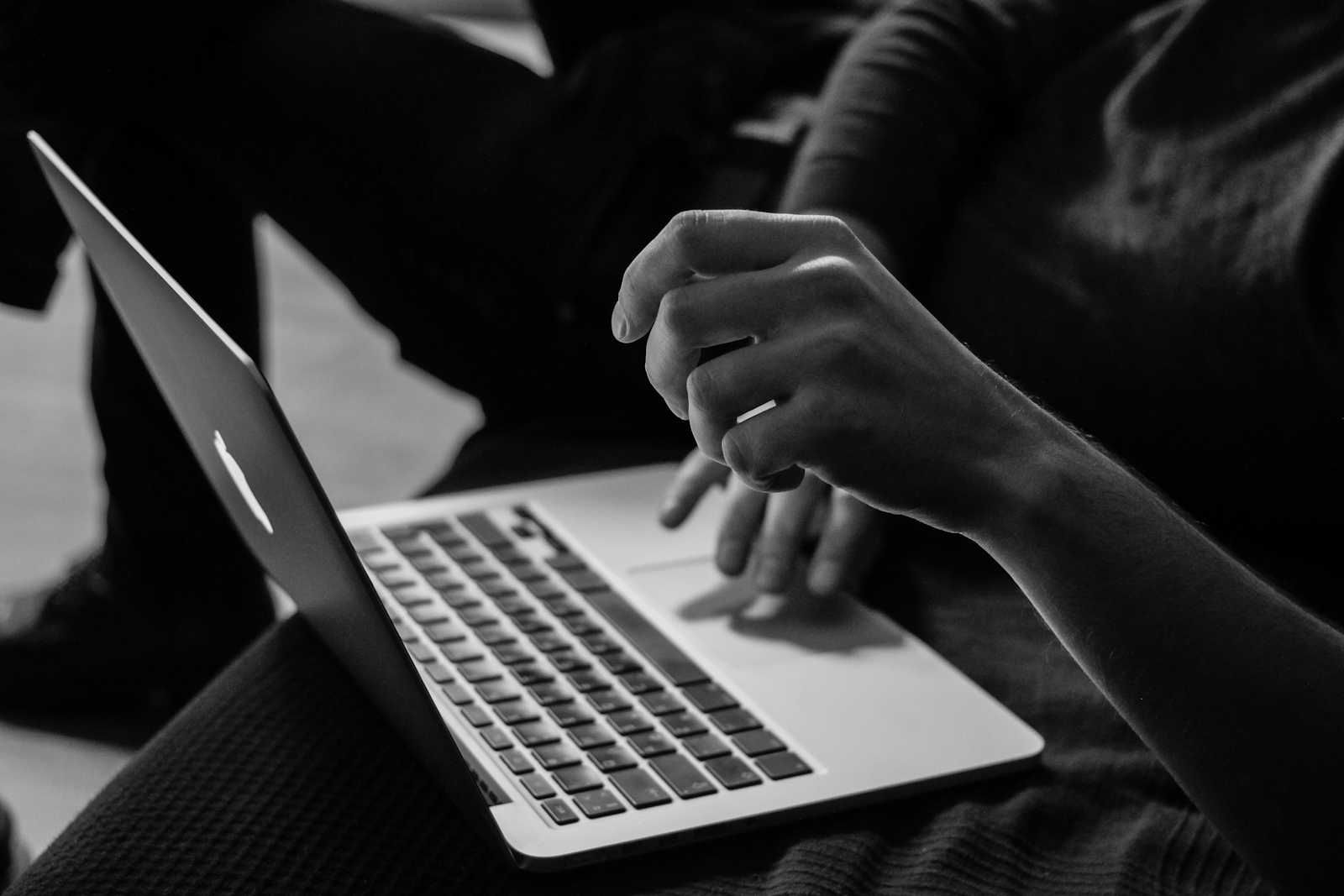
The MERN stack is one of the most popular tech stacks for full-stack development, consisting of MongoDB, Express.js, React, and Node.js. This blog post will provide you with a detailed roadmap to mastering each technology in the MERN stack, covering essential concepts and skills at each stage.
What is the MERN Stack?
The MERN stack is a powerful combination of JavaScript technologies used to build modern web applications. Here's what it comprises:
MongoDB: A NoSQL database for storing application data.
Express.js: A backend framework for handling routes and server-side logic.
React: A frontend JavaScript library for building user interfaces.
Node.js: A runtime environment to execute JavaScript code on the server.
Why Choose the MERN Stack?
Full-Stack JavaScript: With the MERN stack, you can work with JavaScript throughout the entire project—front-end, back-end, and database operations.
Scalability: It is highly scalable for both small-scale projects and enterprise-level applications.
Large Community: All four technologies have strong communities, extensive documentation, and lots of resources.
MERN Stack Roadmap: Step-by-Step Guide
1. Mastering JavaScript (ES6+)
Before diving into the MERN stack, it's crucial to be proficient in JavaScript, particularly ES6+ features. Make sure you're comfortable with:
Variables (let, const)
Arrow functions
Template literals
Promises and async/await
Destructuring and Spread/Rest operators
Higher-order functions (map, filter, reduce)
Resources:
2. Learning Node.js and Express
Node.js and Express are the backbones of the MERN stack, allowing you to create scalable server-side applications.
Node.js: Learn how to set up a Node server, understand its event-driven, non-blocking architecture, and work with file systems and modules.
Key Concepts:
Event loop and async programming
File handling (fs module)
HTTP module
Express.js: This lightweight framework simplifies route management and server logic.
Key Concepts:
Setting up an Express server
Middleware (how to use and create)
Routing
Error handling
Example Tasks:
Set up a basic Node/Express API
Create CRUD operations using Express routes
Implement middleware for logging, authentication, and error handling
Resources:
3. Working with MongoDB and Mongoose
MongoDB is a NoSQL database, and it's crucial for you to learn how to structure data and interact with it. Mongoose is a popular ODM (Object Data Modeling) library for MongoDB.
Key Concepts:
Collections and documents
Schema and Models in Mongoose
Queries (CRUD operations)
Indexing and relationships
Aggregation
Example Tasks:
Set up a MongoDB instance and connect it to your Express app
Create a model for storing user data
Implement CRUD operations for user records
Use aggregation to perform data analysis
Resources:
4. Frontend Development with React
React is a powerful library for building interactive user interfaces. It helps in building reusable UI components and makes the app efficient with its virtual DOM.
Key Concepts:
JSX (JavaScript XML)
React Hooks (useState, useEffect)
Component lifecycle
Props and State management
Conditional Rendering
React Router for navigating pages
Context API for managing global state
Example Tasks:
Set up a React app with
create-react-app
Build a reusable form component
Integrate your Express backend API with React
Implement routing between different pages (e.g., Home, About, Contact)
Build a state management system using Context API or Redux
Resources:
5. Integrating Frontend and Backend
This is where the magic of MERN happens. After building your frontend and backend separately, you'll need to integrate them. Here's how:
REST API: Use Express to create RESTful APIs that serve data to your React app.
Axios/Fetch: Use Axios or the native Fetch API in React to make HTTP requests to your Express backend.
CORS: Ensure that CORS (Cross-Origin Resource Sharing) is properly set up to allow your React app to communicate with your Node.js backend.
JWT Authentication: Use JSON Web Tokens (JWT) to handle user authentication securely.
Example Tasks:
Implement login and signup functionality
Fetch data from your MongoDB database and display it in React components
Protect certain routes using JWT authentication
Resources:
6. State Management with Redux (Optional)
For larger applications, managing state with React's Context API can get complicated. That's where Redux comes in. Redux allows you to manage global state across components effectively.
Key Concepts:
Redux store, actions, and reducers
Thunk for handling async actions
Dispatching actions from React components
Example Tasks:
Set up a Redux store
Connect your React components to the Redux state
Implement actions and reducers to manage user data
Resources:
7. Deployment
Once your MERN app is ready, it's time to deploy it. You can deploy the backend (Node.js and Express) separately or deploy everything as a single app.
Backend Deployment:
Use services like Heroku, AWS, or DigitalOcean to deploy the Node.js server.
MongoDB can be hosted using MongoDB Atlas.
Frontend Deployment:
- Use platforms like Netlify or Vercel to deploy your React app.
Example Tasks:
Deploy your MERN app on Heroku with a MongoDB Atlas database
Set up environment variables for sensitive data (API keys, DB credentials)
Resources:
Final Thoughts
Mastering the MERN stack opens up a world of possibilities in full-stack development. It allows you to build robust, scalable, and dynamic web applications using a single programming language—JavaScript. Follow this roadmap, practice consistently, and soon you'll be building your own end-to-end web applications.
Good luck, and happy coding!
Subscribe to my newsletter
Read articles from Adarsh Mishra directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
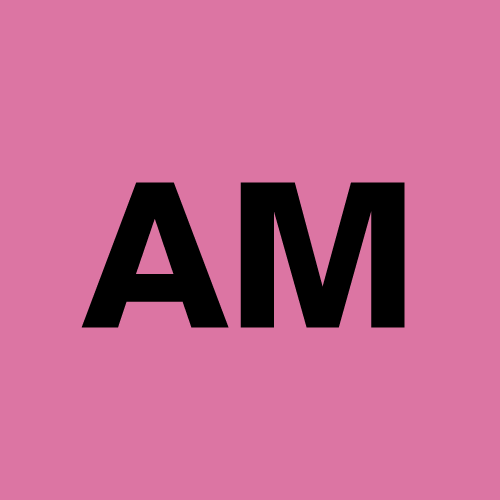