Understanding Objects and Classes in OOP (C++)
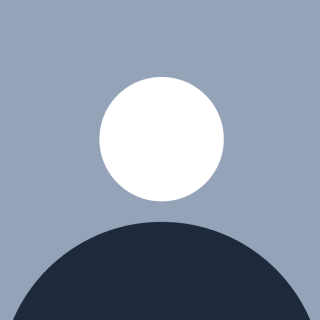
Object-Oriented Programming (OOP) is a fundamental concept in modern programming that allows developers to model real-world entities in code, making it more intuitive and easier to manage complex applications. Let's dive into the basics of objects and classes and see how they fit into the bigger picture of OOP.
Objects: An object in programming represents a real-world entity or concept. It can be anything from a person, a car, a book, or even an abstract idea like a bank account. Objects are instances of classes, meaning they carry the properties and behaviors defined by their class.
Classes: A class acts as a blueprint for objects. It defines the properties (data) and behaviors (functions) that an object will have. Think of a class as a template that groups related attributes and functions into one entity, allowing us to create multiple objects without repetitive code.
In simpler terms, a class is like a cookie-cutter, and objects are the cookies. The cutter (class) defines the shape and features, but each cookie (object) can have its own unique details (like flavor or toppings).
Why Use OOP?
Imagine you need to store details of all the teachers in a college. A beginner might create separate variables for each teacher—teacher1, teacher2, and so on. For 50 or 100 teachers, this would result in a massive, repetitive, and hard-to-manage codebase. OOP solves this problem by introducing the concepts of classes and objects to reduce redundancy and improve code structure.
Example: Let's consider a scenario where we define a class Teacher to store information about teachers like their name, department, subject, and salary.
#include<iostream>
#include<string>
using namespace std;
class Teacher {
// Declaring properties (attributes)
string name;
string department;
string subject;
double salary;
// Method to change the department of a teacher (function inside the class)
void changeDepartment(string new_Department) {
department = new_Department;
}
};
int main() {
Teacher t1; // Creating an object of class Teacher
return 0;
}
Why Does This Code Not Run?
In the above code, all the properties are declared as private by default. In C++, class members (attributes and methods) are private unless specified otherwise. This means that the properties like name, department, and salary are not accessible from outside the class, which leads to errors if we try to manipulate them directly in the main function.
To solve this, we need to introduce access specifiers.
Access Specifiers in C++
Private: Members declared as private can only be accessed from within the class itself. By default, all class members are private if no access specifier is declared.
Public: Members declared as public can be accessed from outside the class. This is useful when you want certain attributes or methods to be available to other parts of the program.
Protected: Members declared as protected can be accessed from within the class and by derived classes (in inheritance scenarios).
#include<iostream>
#include<string>
using namespace std;
class Teacher {
// Private attributes
private:
string name;
string department;
string subject;
double salary;
// Public methods
public:
// Setter methods to set private values
void setDetails(string t_name, string t_department, string t_subject, double t_salary) {
name = t_name;
department = t_department;
subject = t_subject;
salary = t_salary;
}
// Getter methods to retrieve private values
string getName() { return name; }
string getDepartment() { return department; }
string getSubject() { return subject; }
double getSalary() { return salary; }
// Method to display teacher details
void displayTeacherInfo() {
cout << "Name: " << name << "\nDepartment: " << department
<< "\nSubject: " << subject << "\nSalary: " << salary << endl;
}
};
int main() {
Teacher t1; // Creating an object of class Teacher
// Using setter to assign values to the object
t1.setDetails("John Doe", "Computer Science", "Data Structures", 50000);
// Display teacher's information
t1.displayTeacherInfo();
return 0;
}
Setter and Getter Methods
In this example, the setDetails method allows us to set the values for private attributes, while the getName, getDepartment, getSubject, and getSalary methods allow us to get the values of these private attributes. These are called setter and getter methods, respectively.
Setters modify private values.
Getters retrieve those values.
This pattern ensures that private data is only modified or accessed in a controlled way, adhering to the principles of encapsulation, which is one of the pillars of OOP.
Advanced Object-Oriented Programming (OOP) Concepts in C++
In the previous section, we explored the fundamentals of objects and classes. Now, let's take a deeper dive into more advanced concepts that truly define Object-Oriented Programming: Encapsulation, Constructors, Destructors, Inheritance, Polymorphism, and Abstraction.
Encapsulation
Encapsulation refers to the bundling of data and methods that operate on the data within a single unit, the class. It helps in protecting the sensitive information of an object by restricting direct access to its data members. This is achieved through the use of private access specifiers, where certain variables or methods are hidden from the outside world.
In simple terms: Encapsulation = Data Properties + Member Functions in a class.
Encapsulation also enables data hiding — a mechanism to prevent sensitive information from being accessed outside the class.
Consider a banking system where we store the username, account number, password, and balance of a customer. Here, the password and balance are sensitive data that we don't want to expose to the outside world, so we encapsulate them using the private
access specifier.
class BankAccount {
private:
string username;
int accountNumber;
string password; // sensitive data
double balance; // sensitive data
public:
BankAccount(string user, int accNo, string pass, double bal) {
username = user;
accountNumber = accNo;
password = pass;
balance = bal;
}
// Public methods to interact with sensitive data
void deposit(double amount) { balance += amount; }
void withdraw(double amount) { if (balance >= amount) balance -= amount; }
// Getter for balance, no direct access to password
double getBalance() { return balance; }
};
Constructors
A constructor is a special method that is called automatically when an object is created. It is primarily used for initialization. Constructors share the same name as the class and don’t have a return type.
Key Features of Constructors:
Automatically called when an object is created.
Used for memory allocation and initialization.
Cannot have a return type.
Types of Constructors:
Non-parameterized Constructor: A constructor with no arguments.
Parameterized Constructor: A constructor with parameters to initialize an object with specific values.
Copy Constructor: A constructor used to create a new object as a copy of an existing object.
class Teacher {
public:
string name;
string department;
double salary;
// Non-parameterized constructor
Teacher() {
cout << "Teacher object created." << endl;
}
// Parameterized constructor
Teacher(string n, string d, double s) {
name = n;
department = d;
salary = s;
}
void displayInfo() {
cout << "Name: " << name << "\nDepartment: " << department << "\nSalary: " << salary << endl;
}
};
int main() {
// Using parameterized constructor
Teacher t1("John", "Computer Science", 50000);
t1.displayInfo();
}
Copy Constructor
A copy constructor is used to copy the properties of one object to another. It uses pass by reference to avoid deep copying overhead.
class Teacher {
public:
string name;
string department;
double salary;
// Copy Constructor
Teacher(const Teacher &orgObj) {
name = orgObj.name;
department = orgObj.department;
salary = orgObj.salary;
}
};
Shallow vs Deep Copy
Shallow Copy: Copies all member values from one object to another, but references the same memory locations for dynamically allocated resources.
Deep Copy: Copies both the member values and allocates separate memory for dynamically allocated resources to avoid shared references.
Destructor
A destructor is the opposite of a constructor. It is automatically invoked when an object is destroyed to free up memory. It doesn't take arguments or have a return type, and only one destructor is allowed per class.
class Teacher {
public:
// Destructor
~Teacher() {
cout << "Teacher object destroyed." << endl;
}
};
Inheritance
Inheritance allows one class (child or derived class) to acquire the properties and behaviors of another class (parent or base class). It promotes reusability of code and enables the extension of existing functionality.
class Person {
public:
string name;
int age;
Person(string n, int a) : name(n), age(a) {}
};
class Student : public Person {
public:
int rollNumber;
Student(string n, int a, int r) : Person(n, a), rollNumber(r) {}
void displayInfo() {
cout << "Name: " << name << "\nAge: " << age << "\nRoll Number: " << rollNumber << endl;
}
};
int main() {
Student s1("Alice", 20, 101);
s1.displayInfo();
}
Types of Inheritance:
Single Inheritance: One class inherits from one base class.
Multilevel Inheritance: A derived class inherits from another derived class.
Multiple Inheritance: A derived class inherits from multiple base classes.
Hierarchical Inheritance: Multiple derived classes inherit from a single base class.
Polymorphism
Polymorphism means "many forms." It allows objects to behave differently based on their context. There are two types of polymorphism:
Compile-time Polymorphism: Achieved through function overloading and operator overloading.
class MathOperations {
public:
// Function overloading
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
};
Run-time Polymorphism: Achieved through function overriding and the use of virtual functions. This allows derived classes to provide specific implementations of methods defined in the base class.
class Animal {
public:
virtual void sound() {
cout << "Animal makes a sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Dog barks" << endl;
}
};
Abstraction
Abstraction focuses on hiding the implementation details and only exposing essential information. This can be achieved using abstract classes or interfaces. An abstract class provides a base for other classes to build on but cannot be instantiated directly.
class AbstractShape {
public:
virtual void draw() = 0; // Pure virtual function
};
class Circle : public AbstractShape {
public:
void draw() override {
cout << "Drawing a Circle" << endl;
}
};
The "this" Pointer
The this pointer is used to refer to the current object in a class. It’s helpful when dealing with member variables that might share names with function parameters.
class Employee {
string name;
public:
void setName(string name) {
this->name = name; // this pointer refers to the member variable
}
};
By combining these OOP concepts, you can build efficient, modular, and scalable software systems. Whether you're securing sensitive information with encapsulation, reusing code through inheritance, or enabling flexibility with polymorphism, mastering these concepts will make you a stronger C++ developer.
Below is a complete C++ program that demonstrates all the major Object-Oriented Programming (OOP) concepts: Encapsulation, Constructors, Destructors, Inheritance, Polymorphism, Abstraction, and more. The code is well-commented.
#include <iostream>
#include <string>
using namespace std;
// 1. Encapsulation: Wrapping up of data and methods into a single unit (class)
class BankAccount {
private:
string username; // Private: Data hiding
int accountNumber;
string password; // Sensitive information is hidden
double balance;
public:
// 2. Constructor: Initializes the object when it is created
BankAccount(string user, int accNo, string pass, double bal) {
username = user;
accountNumber = accNo;
password = pass;
balance = bal;
}
// Public method to deposit money
void deposit(double amount) {
balance += amount;
}
// Public method to withdraw money
void withdraw(double amount) {
if (balance >= amount)
balance -= amount;
else
cout << "Insufficient balance!" << endl;
}
// Getter for balance (encapsulation)
double getBalance() {
return balance;
}
// Destructor: Automatically called when the object is destroyed
~BankAccount() {
cout << "BankAccount object destroyed for user: " << username << endl;
}
};
// Base class: Person (for Inheritance demonstration)
class Person {
protected:
string name;
int age;
public:
// Parameterized constructor
Person(string n, int a) : name(n), age(a) {}
// Virtual function (polymorphism) for overriding in derived classes
virtual void displayInfo() {
cout << "Name: " << name << "\nAge: " << age << endl;
}
// Virtual Destructor: To ensure proper cleanup in inheritance
virtual ~Person() {
cout << "Person object destroyed" << endl;
}
};
// Derived class: Student (Inheritance from Person)
class Student : public Person {
private:
int rollNumber;
public:
// Parameterized constructor for Student class (Inheritance)
Student(string n, int a, int r) : Person(n, a), rollNumber(r) {}
// Overriding base class method (Polymorphism)
void displayInfo() override {
cout << "Name: " << name << "\nAge: " << age << "\nRoll Number: " << rollNumber << endl;
}
// Destructor
~Student() {
cout << "Student object destroyed" << endl;
}
};
// Another derived class: Teacher (Inheritance from Person)
class Teacher : public Person {
private:
string department;
double salary;
public:
// Parameterized constructor for Teacher class
Teacher(string n, int a, string dept, double sal) : Person(n, a), department(dept), salary(sal) {}
// Overriding base class method (Polymorphism)
void displayInfo() override {
cout << "Name: " << name << "\nAge: " << age << "\nDepartment: " << department << "\nSalary: " << salary << endl;
}
// Destructor
~Teacher() {
cout << "Teacher object destroyed" << endl;
}
};
// Abstract base class (for Abstraction demonstration)
class AbstractShape {
public:
// Pure virtual function (Abstract method)
virtual void draw() = 0; // This method must be overridden by derived classes
};
// Derived class from AbstractShape
class Circle : public AbstractShape {
public:
// Overriding abstract method
void draw() override {
cout << "Drawing a Circle" << endl;
}
};
// Derived class from AbstractShape
class Rectangle : public AbstractShape {
public:
// Overriding abstract method
void draw() override {
cout << "Drawing a Rectangle" << endl;
}
};
// Main function to demonstrate all concepts
int main() {
// Demonstrating Encapsulation and Constructors
BankAccount account1("Alice", 12345, "password123", 1000.50);
account1.deposit(500);
account1.withdraw(300);
cout << "Balance after transactions: $" << account1.getBalance() << endl;
// Demonstrating Inheritance and Polymorphism
Person* p1 = new Student("Bob", 21, 101); // Polymorphism: Base class pointer to derived class object
Person* p2 = new Teacher("John", 45, "Computer Science", 55000);
cout << "\nStudent Info:" << endl;
p1->displayInfo(); // Calls Student's displayInfo()
cout << "\nTeacher Info:" << endl;
p2->displayInfo(); // Calls Teacher's displayInfo()
// Cleanup using Destructors
delete p1;
delete p2;
// Demonstrating Abstraction
AbstractShape* shape1 = new Circle(); // Abstract base class pointer to derived class
AbstractShape* shape2 = new Rectangle();
cout << "\nDrawing Shapes:" << endl;
shape1->draw(); // Calls Circle's draw()
shape2->draw(); // Calls Rectangle's draw()
// Cleanup abstract shape objects
delete shape1;
delete shape2;
return 0; // End of program
}
Thank you for taking the time to read through this detailed exploration of Object-Oriented Programming concepts. I hope this guide has provided you with a clear understanding of how principles like encapsulation, inheritance, polymorphism, and abstraction come together to create efficient and scalable code. Your journey into mastering OOP will only grow stronger with practice, and I'm excited for the innovations you will bring using these powerful techniques.
Thank you
Happy Coding
-HK
Subscribe to my newsletter
Read articles from Harshil HK directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Harshil HK
Harshil HK
I am a Software Developer(SDE) & AI Innovator with expertise in AI, scalable architectures, and cloud-native systems. My experience spans building AI-powered applications, real-time data processing, and fintech solutions while integrating cutting-edge microservices and cloud technologies. AI & ML – Currently building an LLM model and exploring MLOps for scalable AI deployment. Fintech & Blockchain – Developing AI-powered payment systems with blockchain-based cross-border payments and offline transactions. Backend Engineering & Microservices – Built 4+ REST APIs with Spring Boot, JPA, Hibernate, Resilience4j (rate limiting, circuit breaker, bulkhead, retry mechanisms). Cloud & Scalable Systems – Experienced in Kubernetes, Apache Pulsar, and high-availability architectures. With a strong foundation in AI, fintech, and scalable backend development, I am passionate about solving real-world problems through intelligent, efficient, and secure technology. This keeps it crisp, impactful, and highlights your latest skills. Let me know if you’d like any refinements!