Understanding Nested Routes in React Router: A Beginner's Guide
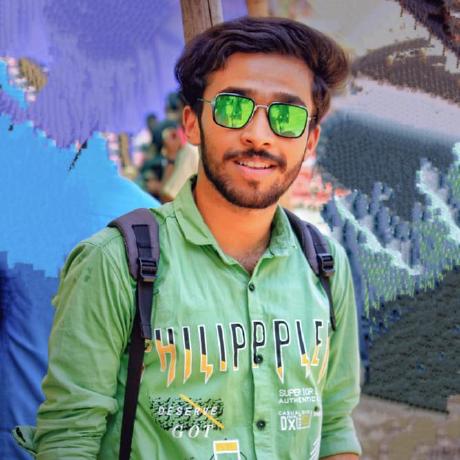
Hey everyone!
If you’re diving into React, one of the essential libraries you'll need to get familiar with is React Router Dom. Recently, I encountered a scenario involving nested routes in React, and I wanted to share my approach. Nested routing is common in many applications, and I had to implement it up to three levels deep. Initially, I found it a bit confusing, but with some guidance, I figured it out. So, let’s get started!
Setting Up the Project
For this tutorial, I'll be using create-react-app
to kickstart a simple project and will install react-router-dom
using npm.
Project Structure
We’re going to build a simple dashboard featuring a common sidebar with several links. One of the pages will have a dedicated navigation bar to access additional sub-pages. Additionally, we’ll have a login page that redirects us to the dashboard. Note that for simplicity, there will be no authentication or authorization in this tutorial.
Step 1: Implementing the Login Component
Let’s start by adding a simple login component. This will include a button that, when clicked, redirects the user to the
/home
page.import { Link } from 'react-router-dom'; const Login = () => { return ( <div className='login'> <p>Login to the app</p> <Link to='/home' className='button'>Login</Link> </div> ); }; export default Login;
Step 2: Defining Routes
Next, we need to define the routes for our application. We will create a separate file called Routes.js
that will store our route configuration. Each route will be an object containing the path and its corresponding component.
javascriptCopy codeimport Home from './Home';
import Login from './Login';
const routes = [
{ path: '/login', component: Login },
{ path: '/home', component: Home },
];
export default routes;
Step 3: Adding Nested Routes
Inside the Home component, we will set up additional nested routes. These routes will be organized in a similar fashion as above, but they will be nested within the /home
route.
javascriptCopy codeimport Home from './Home';
import Login from './Login';
import Page1 from './pages/Page1';
import Page2 from './pages/Page2';
const routes = [
{ path: '/login', component: Login },
{
path: '/home',
component: Home,
routes: [
{ path: '/home/page1', component: Page1 },
{ path: '/home/page2', component: Page2 },
],
},
];
export default routes;
Step 4: Setting Up the App Component
Now, let’s add the routes to our App.js
file. We’ll wrap our application in BrowserRouter
and use Switch
to handle route transitions.
javascriptCopy codeimport { BrowserRouter, Redirect, Switch } from 'react-router-dom';
import routes from './Routes';
import RouteWithSubRoutes from './utils/RouteWithSubRoutes';
function App() {
return (
<BrowserRouter>
<Switch>
<Redirect exact from='/' to='/login' />
{routes.map((route, i) => (
<RouteWithSubRoutes key={i} {...route} />
))}
</Switch>
</BrowserRouter>
);
}
export default App;
Step 5: Creating the RouteWithSubRoutes Component
The RouteWithSubRoutes
component will handle rendering our routes and passing down any nested routes.
javascriptCopy codeimport { Route } from 'react-router-dom';
const RouteWithSubRoutes = (route) => {
return (
<Route
path={route.path}
render={(props) => (
<route.component {...props} routes={route.routes} />
)}
/>
);
};
export default RouteWithSubRoutes;
- Finally, we’ll create the
Home
component to manage the sidebar and render the selected page based on the user's navigation.
javascriptCopy codeimport { Switch, Link } from 'react-router-dom';
import RouteWithSubRoutes from './utils/RouteWithSubRoutes';
const Home = ({ routes }) => {
const menu = [
{ path: '/home/page1', name: 'Page1' },
{ path: '/home/page2', name: 'Page2' },
];
return (
<div className='home'>
<div className='sidebar'>
<h2>React Nested Routes</h2>
<ul>
{menu.map((menuItem) => (
<li key={menuItem.name}>
<Link to={menuItem.path}>{menuItem.name}</Link>
</li>
))}
</ul>
</div>
<Switch>
{routes.map((route, i) => (
<RouteWithSubRoutes key={i} {...route} />
))}
</Switch>
</div>
);
};
export default Home;
With this setup, you can easily manage nested routes within your React application. The structure allows you to keep your routing organized and maintainable. You can continue to nest routes as needed.
Subscribe to my newsletter
Read articles from Supratim Dey directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
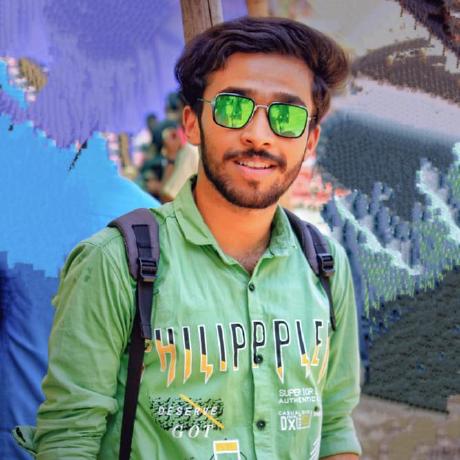