JavaScript Event Bus Explained: Simple Guide for Beginners
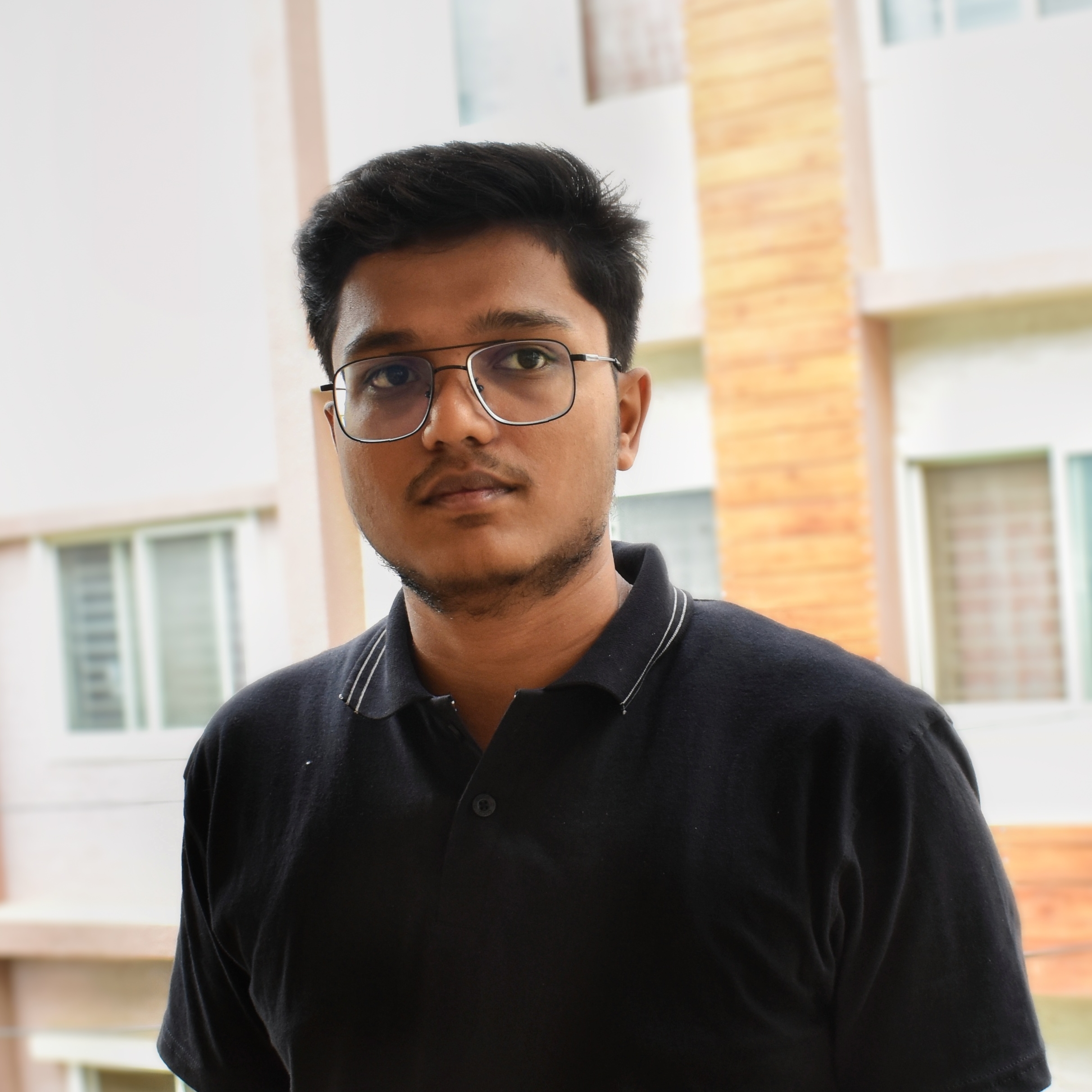
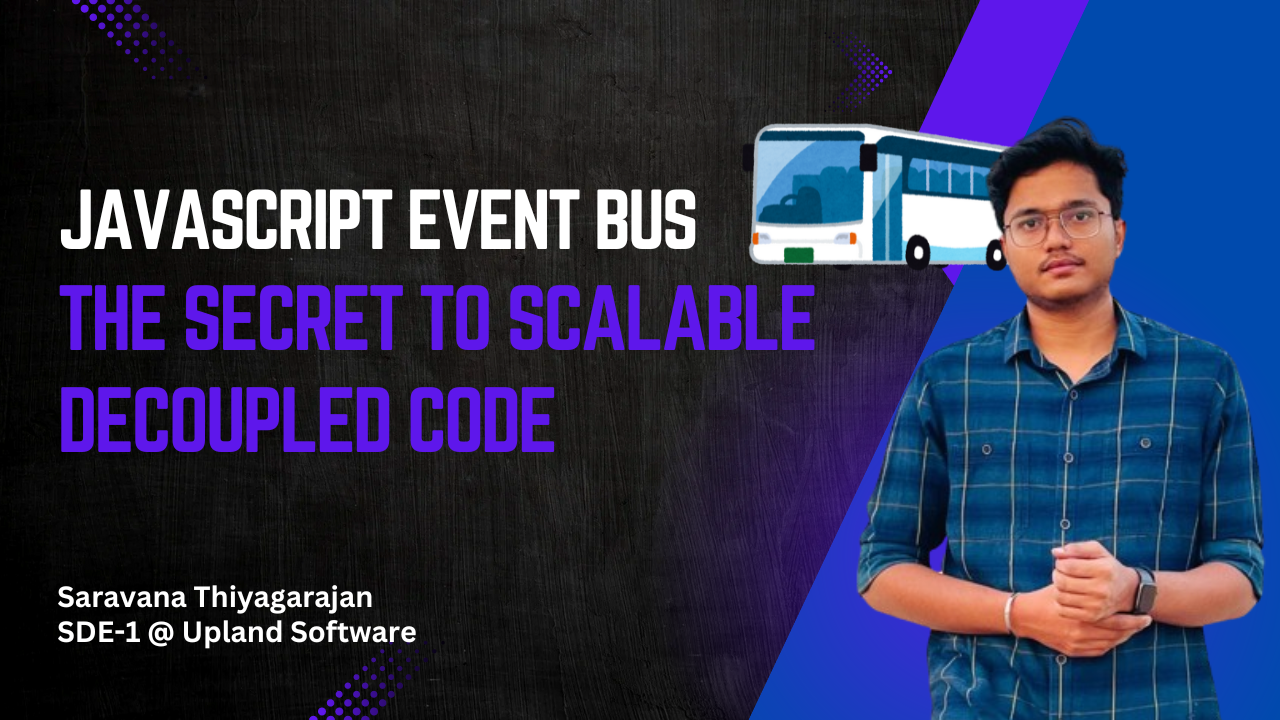
In this article, we explore the Event Bus pattern, a tool for decoupling components and facilitating communication in complex applications. The JavaScript Event Bus allows components to publish and subscribe to events, promoting loose coupling, scalability, and improved code organization. We provide an overview of event-driven architecture, the role of key components, and benefits of using event-driven systems. Additionally, we demonstrate implementing a simple Event Bus in JavaScript with example code to illustrate its usage.
Explanation of the JavaScript Event Bus
The JavaScript Event Bus is a design pattern that allows different components in an application to communicate with each other by publishing and subscribing to events. It acts as a central hub that enables components to send and receive messages without having a direct reference to each other.
Understanding Event-Driven Architecture
Event Publication: A publisher component publishes an event to the Event Bus by calling the
publish
method and passing the event name and data.Event Bus Routing: The Event Bus receives the event and checks if there are any subscribers for that event.
Subscriber Notification: If there are subscribers, the Event Bus notifies them by calling their callback functions with the event data.
Subscriber Processing: The subscriber components process the event data and perform the necessary actions.
Key Components of the Event Bus
Event: An event is a message that is published by a component to notify other components of a specific occurrence or action.
Event Bus: The Event Bus is the central hub that manages the publication and subscription of events. It is responsible for routing events to the appropriate components.
Publisher: A publisher is a component that publishes an event to the Event Bus.
Subscriber: A subscriber is a component that subscribes to an event on the Event Bus.
Benefits of using event-driven systems
Here are some of the key benefits of using event-driven systems
Loose Coupling: Event-driven systems promote loose coupling between components, making modifying or replacing individual components easier without affecting the rest of the application.
Scalability: Event-driven systems enable scalability by allowing components to be added or removed dynamically, making it easier to handle increasing traffic or user growth.
Decoupling: Event-driven systems decouple components from each other, making it easier to add, remove, or modify components without affecting the rest of the application.
Improved Code Organization: Event-driven systems promote a more organized code structure, with each component focused on a specific task or functionality.
Implementing a Simple JavaScript Event Bus
We define an
EventBus
the class that allows registering listeners for specific events using theregister
method.We define an
EventListener
interface that specifies thehandle
method that will be called when an event is fired.
EventBus Class Implementation
class EventBus {
constructor() {
this.listeners = {};
}
on(eventName, listener) {
if (!this.listeners[eventName]) {
this.listeners[eventName] = [];
}
this.listeners[eventName].push(listener);
}
off(eventName, listener) {
if (this.listeners[eventName]) {
const index = this.listeners[eventName].indexOf(listener);
if (index!== -1) {
this.listeners[eventName].splice(index, 1);
}
}
}
emit(eventName,...args) {
if (this.listeners[eventName]) {
this.listeners[eventName].forEach(listener => listener(...args));
}
}
}
ExampleEvent.js
class ExampleEvent {
constructor(data) {
this.data = data;
}
}
ExampleListener.js
We define an
ExampleListener
a class that has ahandle
method that will be called when an event is fired.We create an instance of the
EventBus
and register theExampleListener
for theexample:event
event.We create an instance of the
ExampleEvent
and emit it using theemit
method of
class ExampleListener {
handle(event) {
console.log(`Received event with data: ${event.data}`);
}
}
Usage
const eventBus = new EventBus();
const listener = new ExampleListener();
eventBus.on('example:event', listener.handle.bind(listener));
const event = new ExampleEvent('Hello, World!');
eventBus.emit('example:event', event);
When we run this code, the ExampleListener
will receive the ExampleEvent
and log "Received event with data: Hello, World!" to the console.
Note that in JavaScript, we use the bind
method to ensure that the handle
the method is called with the correct this
context. This is because when we pass a method as a callback, the this
context is lost.
Conclusion
we've explored the benefits of event-driven systems by understanding the benefits and best practices of event-driven systems, you can unlock the full potential of your frontend applications and deliver exceptional user experiences.
If you found this article helpful, please share it with your friends and colleagues who are interested in learning more about event-driven systems.
Subscribe to my newsletter
Read articles from Saravana Sai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
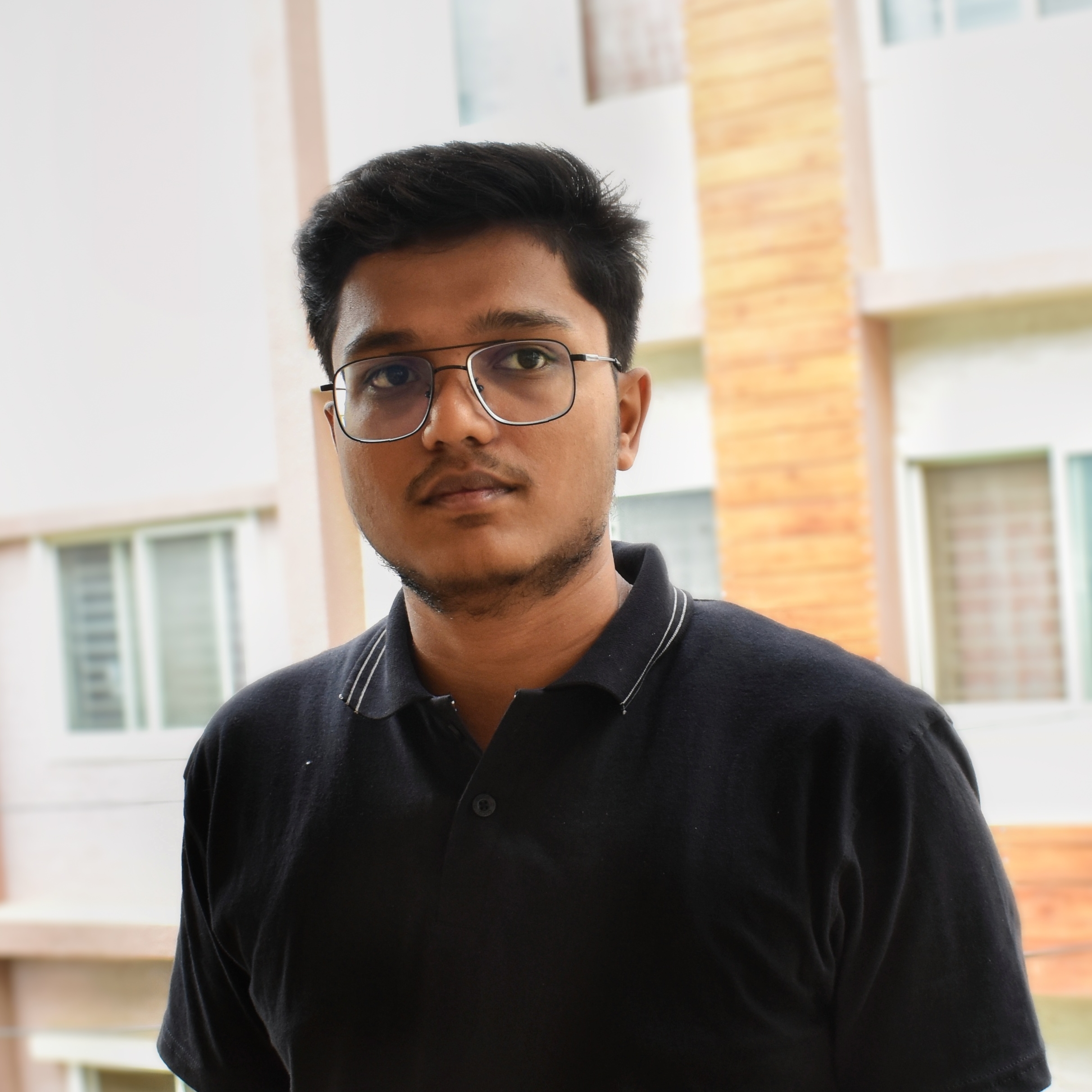
Saravana Sai
Saravana Sai
I am a self-taught web developer interested in building something that makes people's life awesome. Writing code for humans not for dump machine