GraphQL with Python Strawberry

Table of contents
- Step 1: Setting the Scene - Installing the Essentials
- Step 2: Understanding GraphQL Basics
- Step 3: Creating Your First Query - Ask for Exactly What You Need
- Step 4: Expanding Queries with Nested Fields
- Step 5: Mutations - Making Changes to Your Grocery List
- Step 6: Real-Time Updates with Subscriptions
- Step 7: Integrating Strawberry with FastAPI - A Seamless Checkout
- Conclusion: The Perfect Grocery Store Experience with Strawberry and GraphQL
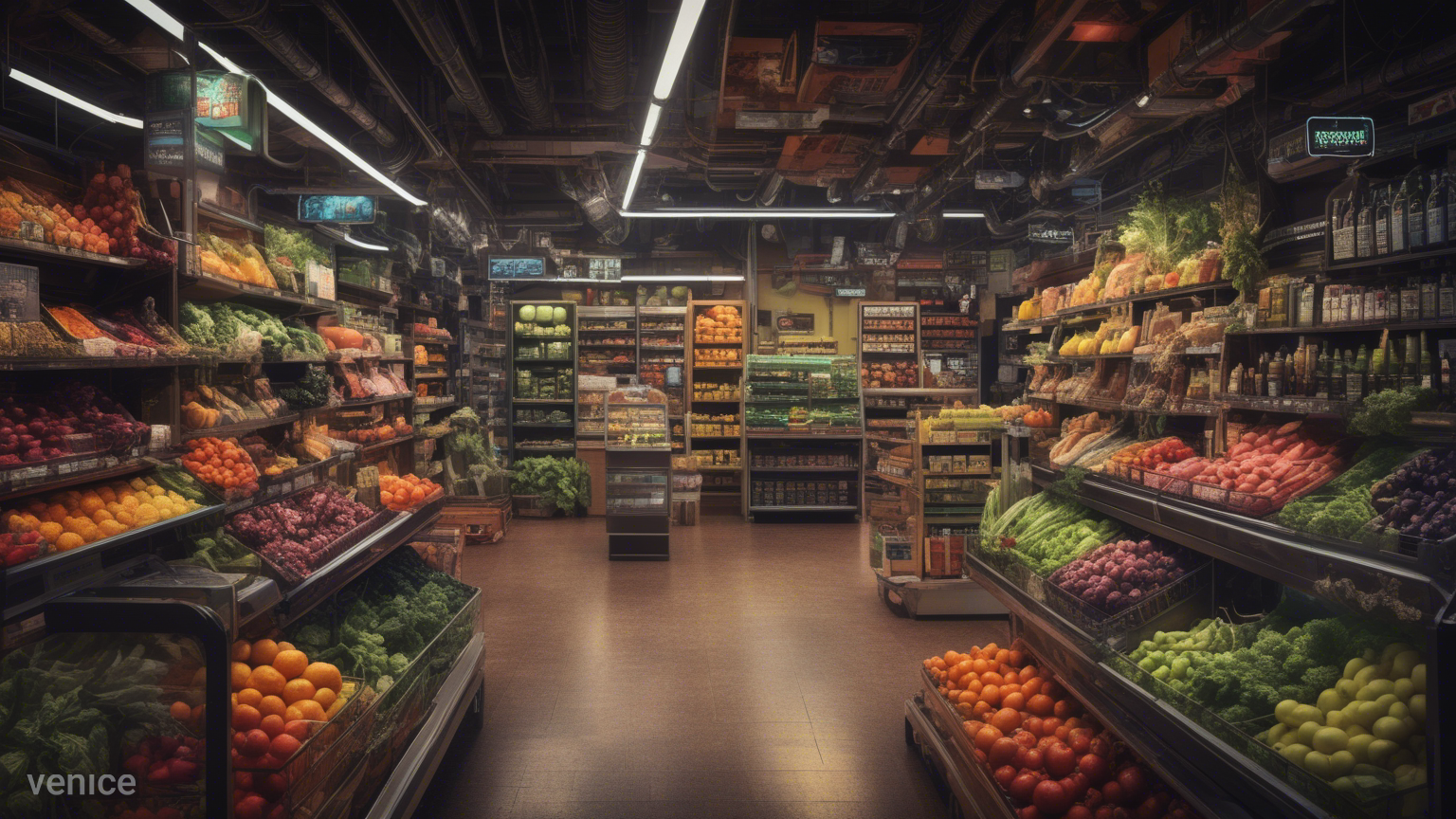
Imagine this: You walk into your favorite grocery store, ready to fill your cart with the essentials. You’re in a hurry, so you only want specific items—some organic strawberries, a loaf of sourdough, and almond milk. Now, instead of wandering through every aisle and picking things manually (like in traditional APIs), you simply hand your list to the store manager, who collects exactly what you need and delivers it directly to you. Efficient, right?
This is the beauty of GraphQL. Rather than pulling all possible data from an endpoint like in REST APIs, GraphQL allows you to ask for only what you need. No extra data, no unnecessary overload. And what makes this experience even better is Python Strawberry, an easy-to-use library that allows you to set up GraphQL servers with Python.
In this extended journey, we’ll explore the core concepts of Strawberry, delve into some practical use cases. Let’s not only learn how to install and use Strawberry, but also dive deeper into the technical aspects of creating, mutating, and handling real-time data.
Step 1: Setting the Scene - Installing the Essentials
Before you embark on this seamless grocery shopping experience, you need the right tools. Just like how you wouldn't start grocery shopping without a cart, we need to install a few key packages to get Strawberry and GraphQL up and running.
To install the required packages, you’ll need to have Python 3.10+ installed. After that, Strawberry can be installed using pip:
pip install strawberry-graphql
If you want to integrate Strawberry with FastAPI (which we will later in the article), install the FastAPI package too:
pip install fastapi
pip install strawberry-graphql[fastapi]
You’ll also need Uvicorn, an ASGI server to run your FastAPI app:
pip install uvicorn
Now you’re all set to begin building with Strawberry!
Gathering the Right Ingredients
Think of installing these packages like gathering the ingredients you need for a recipe. You wouldn’t start cooking without the basics like flour, eggs, and milk. Similarly, you need these libraries to kick off your GraphQL adventure. Once you have them in place, you’re ready to start cooking up some awesome APIs.
Step 2: Understanding GraphQL Basics
Before diving into coding, let’s take a moment to appreciate why GraphQL is so powerful. Think of your data as a grocery store. In the traditional REST API world, you walk down every aisle (or hit every endpoint) to collect your items, but you often end up grabbing things you don’t need, like that family-size bag of chips (unwanted data). The REST API doesn’t let you be selective—either you get everything in the aisle, or nothing.
GraphQL flips this concept on its head. Instead of walking through every aisle, you simply hand over a precise shopping list (your query) to the store manager (the GraphQL server). The manager retrieves only the specific items you requested, down to the last strawberry. It’s efficient, precise, and saves you the headache of sifting through a pile of unnecessary data.
So, how do you get started with this neat querying system? Let’s jump into our first example.
Step 3: Creating Your First Query - Ask for Exactly What You Need
Let’s say you want to retrieve information about a particular fruit from a database (or your hypothetical grocery store). You’re only interested in a strawberry and its level of sweetness, not all the fruits available in the store. This is where GraphQL shines—you define what data you need and get exactly that.
Here's how you create a Strawberry Schema in Python to query specific fruit details:
import strawberry
@strawberry.type
class Fruit:
name: str
sweetness: int
@strawberry.type
class Query:
@strawberry.field
def get_fruit(self) -> Fruit:
return Fruit(name="Strawberry", sweetness=8)
schema = strawberry.Schema(query=Query)
In this example, we define a Fruit type with two fields: name
and sweetness
. Then, we create a Query
type that fetches a specific fruit. Here, the query always returns a strawberry with a sweetness level of 8.
Now that we’ve set up the schema, we can query it by executing a GraphQL request like this:
{
getFruit {
name
sweetness
}
}
The response:
{
"data": {
"getFruit": {
"name": "Strawberry",
"sweetness": 8
}
}
}
Customizing Your Grocery List
This is like telling the store manager, "I need only strawberries and I want them to have a sweetness level of 8." You’re being very specific with what you need, and the manager delivers exactly that. In contrast, if you had used a REST API, you might have received a whole fruit basket, with apples and bananas, even though you only wanted one type of fruit.
Step 4: Expanding Queries with Nested Fields
What if you want to know not only the fruit details but also information about the farm it came from? With GraphQL, you can use nested fields to get more detailed information in a single query. You’ll ask for strawberries, and also request details about the farm where they were grown.
@strawberry.type
class Farm:
name: str
location: str
@strawberry.type
class Fruit:
name: str
sweetness: int
farm: Farm
@strawberry.type
class Query:
@strawberry.field
def get_fruit(self) -> Fruit:
return Fruit(
name="Strawberry",
sweetness=8,
farm=Farm(name="Sunny Farms", location="California")
)
schema = strawberry.Schema(query=Query)
Now, when you query for a fruit, you can also request information about the farm:
{
getFruit {
name
sweetness
farm {
name
location
}
}
}
The Response:
{
"data": {
"getFruit": {
"name": "Strawberry",
"sweetness": 8,
"farm": {
"name": "Sunny Farms",
"location": "California"
}
}
}
}
Asking for More Details
Imagine you walk up to the store manager and say, “I’d like to know more about these strawberries—where are they from?” The manager provides you with not only the strawberries but also the name of the farm and its location. This nested query feature in GraphQL helps you get exactly what you want, without the extra clutter.
Step 5: Mutations - Making Changes to Your Grocery List
What if you want to add a new item to your cart while shopping? In GraphQL, this is called a mutation. Mutations allow you to modify data on the server, such as adding, updating, or deleting records.
Here’s an example of adding a new fruit using a mutation:
@strawberry.type
class Mutation:
@strawberry.mutation
def add_fruit(self, name: str, sweetness: int) -> Fruit:
return Fruit(name=name, sweetness=sweetness)
schema = strawberry.Schema(query=Query, mutation=Mutation)
To execute the mutation:
mutation {
addFruit(name: "Blueberry", sweetness: 6) {
name
sweetness
}
}
The Response:
{
"data": {
"addFruit": {
"name": "Blueberry",
"sweetness": 6
}
}
}
Changing Your Grocery List on the Fly
This is like telling the store manager, “Hey, I want to add some blueberries to my cart.” The manager doesn’t hesitate—they promptly update your cart with the new item. Similarly, mutations allow you to adjust data in real-time, adding or changing items as needed.
Step 6: Real-Time Updates with Subscriptions
Let’s say you’re waiting for fresh strawberries to be restocked at the store. Wouldn’t it be great if you could get notified the moment new strawberries are available? This is where GraphQL subscriptions come in handy. Subscriptions allow you to listen for real-time updates from the server.
In Strawberry, setting up a subscription is easy:
import asyncio
@strawberry.type
class Subscription:
@strawberry.subscription
async def new_fruit(self) -> Fruit:
await asyncio.sleep(1)
return Fruit(name="Strawberry", sweetness=9)
schema = strawberry.Schema(query=Query, subscription=Subscription)
You can subscribe to this real-time data by executing a GraphQL subscription query:
subscription {
newFruit {
name
sweetness
}
}
This setup will notify you when a new fruit is added or updated.
Waiting for Fresh Stock
Imagine you’re waiting for the latest batch of fresh strawberries to arrive. Instead of constantly checking the produce section, the store manager promises to notify you the moment they’re available. Similarly, with GraphQL subscriptions, you can wait for real-time updates and be notified immediately when changes occur.
Step 7: Integrating Strawberry with FastAPI - A Seamless Checkout
What good is a grocery trip without a seamless checkout? FastAPI is like the lightning-fast cashier, ensuring that your requests are processed with minimal wait time. Integrating Strawberry with FastAPI is incredibly simple, and it combines the power of GraphQL with the speed and efficiency of FastAPI.
Here’s how you can set it up:
import strawberry
from fastapi import FastAPI
from strawberry.fastapi import GraphQLRouter
@strawberry.type
class Query:
@strawberry.field
def get_fruit(self) -> str:
return "Strawberry"
schema = strawberry.Schema(query=Query)
graphql_app = GraphQLRouter(schema)
app = FastAPI()
app.include_router(graphql_app, prefix="/graphql")
Smooth Checkout
At the end of your grocery run, you want to ensure the checkout is fast and painless. FastAPI, in this analogy, is the cashier who scans your items with lightning speed, ensuring that you get in and out efficiently. Strawberry, integrated with FastAPI, ensures your data requests are processed just as quickly and smoothly.
Conclusion: The Perfect Grocery Store Experience with Strawberry and GraphQL
By the time you walk out of the store, you're carrying the exact items you wanted, nothing more, nothing less. And this is the magic of Python Strawberry—it gives you precision, flexibility, and control over your API requests, ensuring efficient data handling every step of the way.
From querying to mutations, real-time subscriptions to error handling, Strawberry simplifies the complex world of GraphQL with elegance. And when paired with FastAPI, it’s like having the ultimate grocery store experience—efficient, flexible, and always responsive to your needs.
So, the next time you’re thinking about API design, imagine yourself in that grocery store, requesting only what you need, when you need it.
Subscribe to my newsletter
Read articles from Ashish Sam T George directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ashish Sam T George
Ashish Sam T George
👋 Hey there! I'm getwithashish, a seasoned software engineer with a passion for AI. Whether it's diving deep into neural networks or architecting scalable systems, I thrive on challenges that push the boundaries of what's possible. 💻 My journey? It's a blend of code, creativity, and coffee-fueled brainstorming sessions. I believe in not just writing SOLID code, but crafting solutions that make a difference. 🚀 Apart from my ability to turn caffeine into code, I'm your go-to guy for transforming ideas into reality. 📫 Let's connect! Whether you're looking to collaborate on AI-driven innovations or simply want to geek out over the latest tech, drop me a message.