Learn About JavaScript Variables

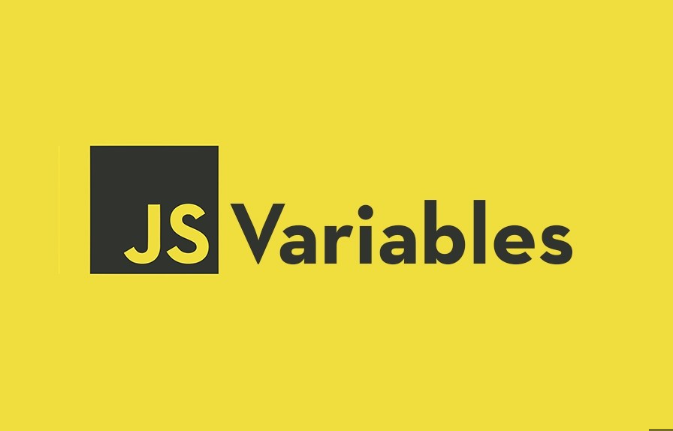
Introduction:-
What is a Variable?
A variable in programming is like a container that holds any type of value, which you can use throughout your code. It stores data that can be accessed and modified as needed.
For example:-
Imagine you go to the market and buy some sugar. To keep it safe and organized, you put the sugar into a container, such as a bottle or a box. This container allows you to store the sugar until you need it later. Similarly, in programming, a variable acts like this container. It holds data, allowing you to store and organize information that you can access and modify whenever you need it in your code. Just as you can use the sugar whenever you want by taking it out of the container, you can use the data stored in a variable at any point in your program.
Types of Keywords to Declare a Variable in JavaScript
In earlier versions of JavaScript, variables were declared using var. With ECMAScript 6 (ES6) in 2015, let and const were introduced as new ways to declare variables.
var
:-This is the traditional way to declare a variable in JavaScript. It allows you to create a container for any data type, such as numbers, strings, or objects. A key feature var is its flexibility in reassigning and redeclaring variables.
Reassign: You can change a variable's value at any time. For example, a variable initially holding the number 10 can later be changed to 20 or a string like "Hello, World!".
Redeclare: You can declare the same variable name multiple times in the same scope without errors. However, this can lead to bugs if not managed carefully, as it may overwrite previous values.
Example:-
var name = "Alice"; // Declare and assign a value name = "Bob"; // Reassign a new value var name = "Charlie"; // Redeclare the variable
let
:-Redeclare: If we try to redeclare a variable let within the same scope(block), it will result in a syntax error. This restriction helps prevent accidental overwriting of variable values, making your code more reliable and easier to debug.
Reassign: The let keyword allows you to reassign a variable's value after it has been initially declared. This means you can update the value stored in the variable as needed throughout your code. This feature provides flexibility, enabling you to adapt the variable's content as your program's logic evolves.
Example:-
let a=2; console.log(a) // output is 2 a=5; console.log(a) // output is 5 let a=5; console.log(a) // SyntaxError: Identifier 'a' has already been declared
const
:-When you use const, you create a variable that should keep the same value throughout your program. Once you assign a value, you can't change or redeclare it in the same scope. This is helpful when you want to make sure certain values stay the same, preventing accidental changes. const is great for setting up configuration settings, fixed values, or any data that shouldn't change. Using const helps you write more reliable and error-free code because it clearly shows that the value is meant to stay constant.
Example:-
const a=2; console.log(a) //output is 2 a=5; console.log(a) // showing syntaxerror const a=5; console.log(a) // showing syntaxerror
Scope in js
In the middle of the document, we used one word "Scope." So, what exactly is the scope?
Scope is a fundamental concept in programming that defines the accessibility and visibility of variables within different parts of your code. It determines where a variable can be used and where it cannot.
Before introducing scope, we had two types of scope: global scope and function scope. After introducing let or const, we now have block-level scope.
So, let's learn about the types of scope and how they different from each other.
1 . Global scope:-
Understanding global scope is quite easy. Imagine you are in a classroom. If something (like chalk) is on the teacher's desk, anyone in the classroom can use it because it's available to everyone. Similarly, a variable in the global scope is accessible anywhere in the entire program.
If you declare a variable outside of a function or block, it is called a global variable, and you can use it in any function or any part of the code.
Example:-
var x = 10; // Global variable
function displayX() {
if (true) {
let x = 20; // Block-scoped variable (inside if block)
console.log("Inside block (let x):", x); // Output: 20
}
console.log("Outside block (var x):", x); // Output: 10 (Accessing global var x)
}
displayX();
console.log("Global scope (var x):", x); // Output: 10
In this example, the variable x is declared using var, making it a global variable. This means that it can be accessed from anywhere in the code, both inside and outside of functions or blocks. When a variable is declared globally, it remains available throughout the entire program.
2 . Function scope:-
Function scope means that variables declared inside a function are only accessible within that specific function. These variables cannot be accessed outside the function. In JavaScript, variables declared using var inside a function have function scope.
Example:-
function showMessage() {
var message = "Hello, World!"; // Variable with function scope
console.log(message); // Accessible inside the function, Output: Hello, World!
}
showMessage(); // Output: Hello, World!
console.log(message); // Error: message is not defined
Explanation:
Inside the function: The variable message is declared using var inside the showMessage function, so it is only accessible within that function.
Outside the function: If you try to access the message variable outside the function, it will give an error because it is limited to the function scope.
3.Block scope:-
Block scope refers to variables that are accessible only within the block they are declared in. A block is any code enclosed in curly braces {}, like inside loops, conditionals, or functions. In JavaScript, variables are declared using let and const have block scope, meaning they cannot be accessed outside the block in which they are defined.
Example:-
if (true) {
let blockVariable = "I am inside a block!"; // Block-scoped variable
console.log(blockVariable); // Accessible inside the block, Output: I am inside a block!
}
console.log(blockVariable); // Error: blockVariable is not defined
Understand the difference between scope in one code
var x = 10; // Global scope
function testScopes() {
var x = 20; // Function scope
if (true) {
let x = 30; // Block scope
console.log("Block scope x:", x); // Output: 30
}
console.log("Function scope x:", x); // Output: 20
}
testScopes();
console.log("Global scope x:", x); // Output: 10
Explanation:
Global scope: First, the value of x is set to 10, which is accessible globally.
Function scope: Inside the function, the value of x becomes 20, which is only valid inside the function.
Block scope: Within the block (if statement), using let, the value of x is 30, which is only accessible inside the block.
Conclusion:-
Understanding how variables work and their scopes in JavaScript helps you write more efficient and error-free code. Whether you're using var, let, or const, it's essential to know how and where you can access these variables in your program.
Subscribe to my newsletter
Read articles from Vinita Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinita Gupta
Vinita Gupta
Full-stack development student at Navgurukul, blending creativity with technical skills. Experienced in HTML, CSS, and JavaScript. Selected for advanced training by HVA, I have strong leadership abilities and a passion for continuous learning. Aspiring to excel in DSA and become a proficient full-stack developer.