Why JSX? How JSX works behind the hood?

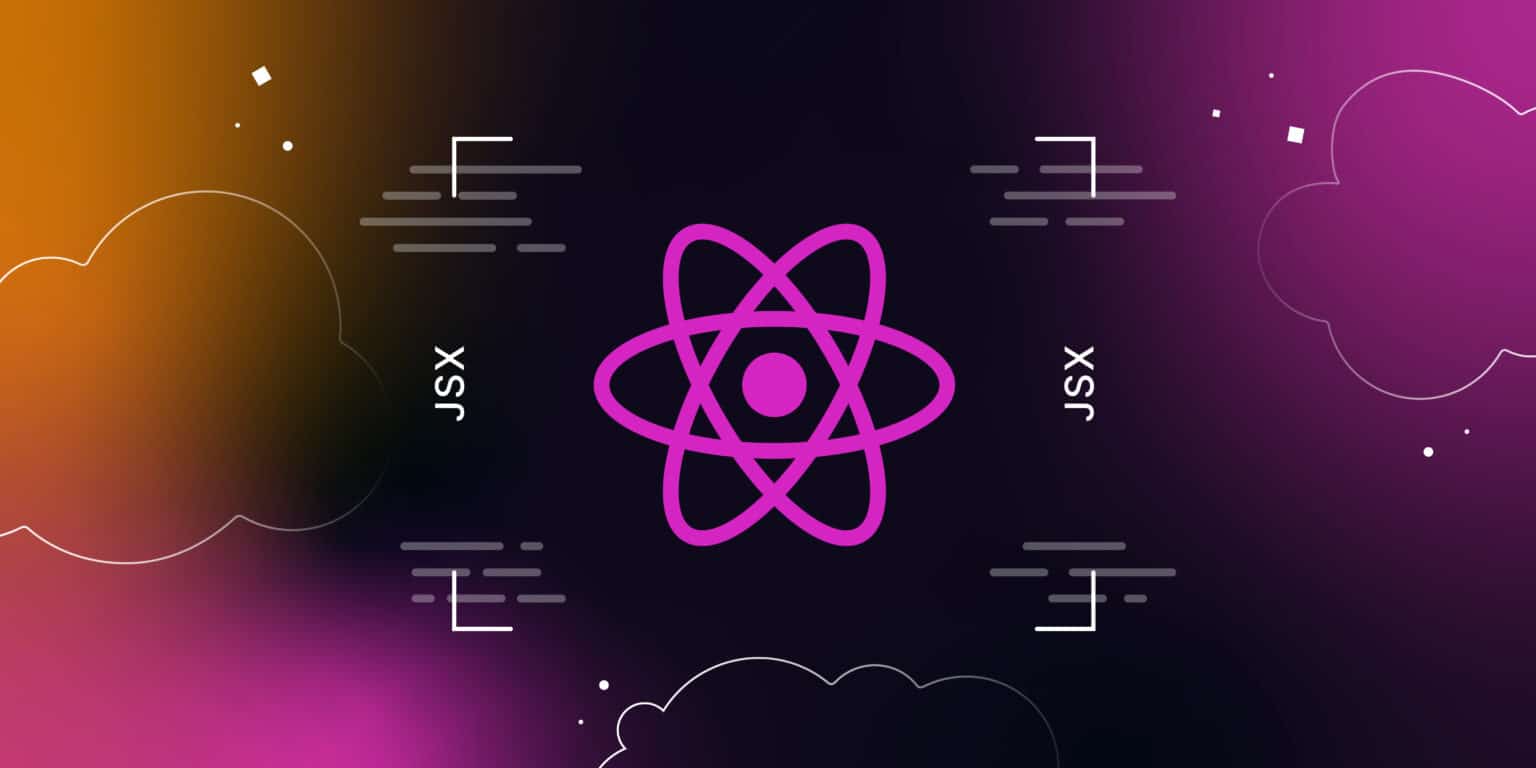
Did you know it is not mandatory to write react code using JSX only? That you can use React.JS using CDN? But that is only the react library. While using the CDN you have to import two packages: react and react-dom. React is the core library that provided the React functionalities from creating components and elements to context and hooks. React-dom is the library that renders those React elements to the DOM after creating a virtual dom. These two packages combined, let’s you use react in your project. But now you will have to React functions to create and render your react components. Like this,
<script>
ReactDOM.render(
<h1>Greeting everyone. Let's dive into JSX!</h1>,
document.getElementById("root")
);
</script>
If we try to use react directly we will have to write the whole application like this using react functions. For a simple code like this:
<div>
<h1>Today's checklist!</h1>
<ul>
<li>List item 1</li>
<li>List item 2</li>
</ul>
</div>
You’ll have to write in react:
React.createElement('div', null,
React.createElement('h1', null, 'Today's checklist!'),
React.createElement('ul',null,
React.createElement("li", null, "List item 1"),
React.createElement("li", null, "List item 2")
);
);
Where you create each element in react using React.createElement which takes the element name in the first parameter, props in second paremeter and children (or inner elements) in the third parameter. Just imagine how complex your codebase would look in this approach. That’s when JSX comes in.
JSX with React
JSX(JavaScript syntax extension) is a JavaScript extension that allows to declare React’s object tree using HTML like syntax. It is allows us to write JavaScript that looks like markup. When writing JSX, we are actually writing JavaScript. These JavaScript funtions are by the same name as HTML elements. When we write a <div></div> in JSX behind the screen, this div is actually a js function that calls the React.createElement method. So, actually react always creates elements and renders in the already given approach. What jsx does is, creating an environment that feels like writing HTML but. So, if we convert the already discussed code in JSX now:
<script>
function Intro() {
return(
<div>
<h1>Today's checklist!</h1>
<ul>
<li>List item 1</li>
<li>List item 2</li>
</ul>
</div>
)}
ReactDOM.render(
<Intro />,
document.getElementById("root")
);
</script>
Sweet. Now we can code similarly we code in HTML.
Transpiler
Now, one last thing. JSX is not a default or mandatory feature of React. To use it you will have to use a transpiler. A transpiler is a source-to-source translator. It takes the source code in a programming language (in this case JSX) as its input and produces an equivalent source code in the same or a different programming language (in this case React funtions). Notice one thing it can also convert from a language to the same language. As a result, enabling to support modern js Functions in order browsers. As a result, Even if we work with latest js features that are not supported by old browsers, babel will convert the modern JavaScript to the supported JavaScript version on those old browsers. Making our application versatile across all browsers. With react we'll be using Babel Transpiler offering us these two features. For that we'll just have to add the CDN of babel transpiler and our application ready to be written in JSX with react.
Thank you for reading the article. If you received any insight, feel free to give a heart to encourage me.
Subscribe to my newsletter
Read articles from shohanur rahman directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
