Mastering SwiftUI Stacks for Better App Layouts

1 min read
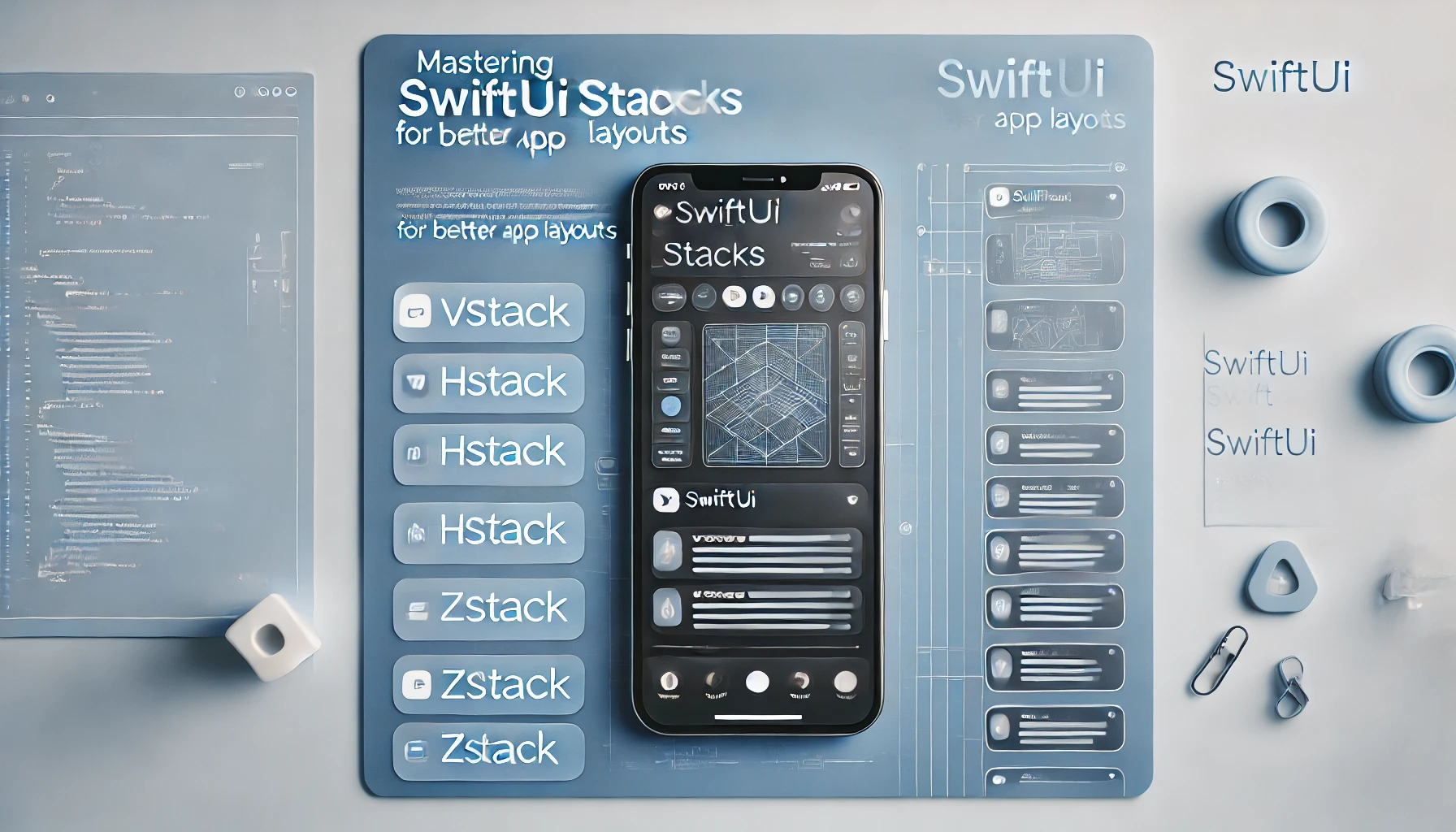
VStack: Use the VStack to arrange views in a vertical layout.
struct ContentView: View {
var body: some View {
VStack(spacing: 20) {
Text("Hello, Developer!")
.font(.title)
.foregroundColor(.blue)
.padding(.bottom, 10)
Text("You are Great!")
.font(.headline)
.foregroundColor(.green)
Text("You are necessary to the world!")
.font(.subheadline)
.foregroundColor(.orange)
.multilineTextAlignment(.center)
}
.padding()
.background(Color(.systemGray6)) // Soft background
.cornerRadius(10) // Rounded corners
.shadow(radius: 5) // Soft shadow
}
}
HStack: Use the HStack to arrange views in a horizontal layout.
var body: some View {
HStack(spacing: 15) {
Text("iPhone")
.font(.headline)
.foregroundColor(.blue)
Text("Operating")
.font(.headline)
.foregroundColor(.green)
Text("System")
.font(.headline)
.foregroundColor(.orange)
}
.padding()
.background(Color(.systemGray6)) // Soft background color
.cornerRadius(10) // Slight rounded corners
.shadow(radius: 5) // Soft shadow for depth
}
ZStack / Depth Stack: It organizes your views so they are stacked on top of each other.
struct ContentView: View {
var body: some View {
ZStack {
// Background color
Color.blue
.ignoresSafeArea()
// Circle in the middle
Circle()
.frame(width: 200, height: 200)
.foregroundColor(.yellow)
// Text overlaying the circle
Text("Hello, ZStack!")
.font(.largeTitle)
.foregroundColor(.white)
}
}
}
0
Subscribe to my newsletter
Read articles from thevenkat directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

thevenkat
thevenkat
I am a Senior iOS Developer, crafting Native iOS applications using Swift. My passion lies in creating innovative solutions that enhance user experience and drive customer satisfaction. I excel in problem-solving, code optimization, and staying updated with the latest trends and technologies in the iOS ecosystem. I thrive in collaborative environments and am always eager to share knowledge and learn from others.