"Mastering Python: A Comprehensive Guide to Data Types"

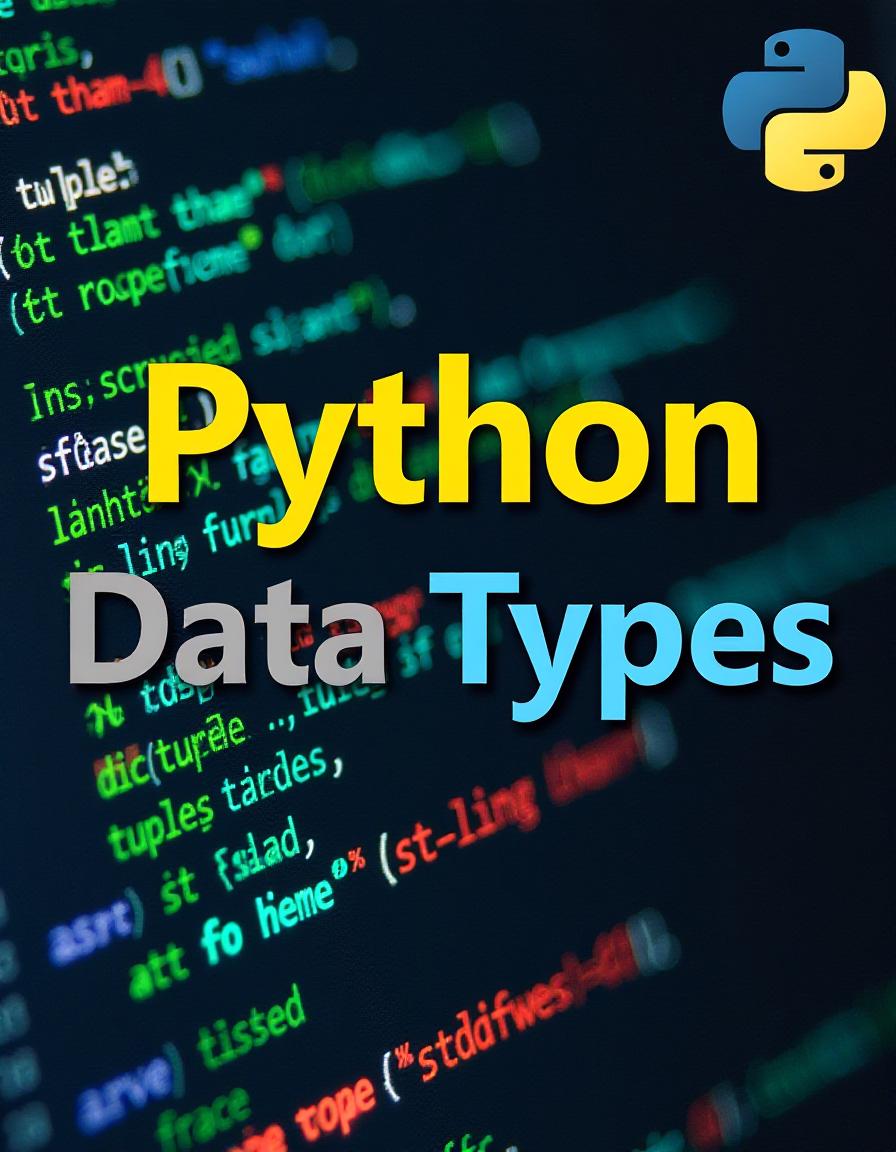
Python, a versatile and powerful programming language, is renowned for its simplicity and readability. One of the fundamental aspects of Python that every programmer must understand is its data types. Data types in Python define a variable's value, and mastering them is crucial for efficient coding. In this guide, we'll explore the various data types in Python and how to use them effectively.
Integer
Float
Boolean
String
Complex
List
Tuple
Sets
Dictionary
Integer
In Python, integers (int
) are a built-in data type used to represent whole numbers without a fractional component. Python's int
type can handle arbitrarily large values, as it automatically expands based on memory availability.
print(50)
will output the value50
, which is an integer.type(50)
returns the type of the value50
, which will output<class 'int'>
, indicating that the value is of integer type.
Float
In Python, float
is a data type used to represent real numbers with a decimal point
print(6.4)
will output the value6.4
, which is a floating-point number.type(6.4)
returns the type of the value6.4
, which will output<class 'float'>
, indicating that the value is offloat
type.
Boolean
In Python, Boolean data types (bool
) represent two values: True
or False
print(True)
will outputTrue
, andtype(True)
returns<class 'bool'>
, indicating thatTrue
is of the Boolean type.Similarly,
print(False)
will outputFalse
, andtype(False)
returns<class 'bool'>
, showing thatFalse
is also a Boolean type.
String
In Python, a string (str
) is a sequence of characters enclosed in quotes (single, double, or triple) used to represent text.
The
print()
function is used to display the string "Nabaranjan" on the output console.- The
type()
function checks the type of the input, which in this case is the string "Nabaranjan". It will return, confirming that the data type of "Nabaranjan" is a string.
- The
Output:
The string "Nabaranjan" is printed.
The type of the string is
<class 'str'>
, which is abbreviated asstr
in the output.
Complex
Complex data types in Python include lists, tuples, dictionaries, and sets, used to store collections of data with varying mutability, order, and key-value pairing capabilities.
The
print()
function outputs the complex number5 + 6j
.The
type()
function checks the type of the input, which is a complex number (5 + 6j
). It returns<class 'complex'>
, indicating that the data type is a complex number.
Output:
The complex number
(5 + 6j)
is printed.The type of the complex number is
complex
.
List
In Python, a list is a mutable, ordered collection of elements, allowing duplicate items and supporting various data types.
The
print()
function outputs the list[1, 2, 3, 4, 5]
.The
type()
function checks the type of the input, which is a list in this case. It will return<class 'list'>
, confirming that the data type is a list.
Output:
The list
[1, 2, 3, 4, 5]
is printed.The type of the list is
list
.
Tuple
In Python, a tuple is an immutable, ordered collection of elements, allowing duplicates and supporting multiple data types.
The code is creating a tuple with the values
(1, 2, 3)
.print((1, 2, 3))
: This prints the tuple(1, 2, 3)
to the console.type((1, 2, 3))
: This checks the type of the object(1, 2, 3)
, and the output istuple
, confirming that it is a tuple.
Sets
A set in Python is an unordered, mutable collection of unique, hashable elements that supports mathematical set operations.
print({1, 2, 3})
: This prints the set{1, 2, 3}
to the console. Sets are displayed with curly braces{}
and contain unique elements.type({1, 2, 3})
: This checks the type of the object{1, 2, 3}
, and the output isset
, confirming that the object is a set.
Dictionary
A dictionary in Python is an unordered, mutable collection of key-value pairs, where each key is unique and used to access its corresponding value.
print({"Name": "Nabaranjan", "age": 27})
: This prints the dictionary to the console. The dictionary consists of key-value pairs, where"Name"
is the key with the value"Nabaranjan"
, and"age"
is the key with the value27
.type({"Name": "Nabaranjan", "age": 27})
: This checks the type of the object, which is a dictionary (dict
).
Conclusion
Understanding Python's data types is essential for writing efficient and effective code. By mastering these data types, you can fully utilise Python's capabilities and write powerful, easy-to-understand programs. Whether dealing with numbers, sequences, mappings, or sets, Python provides rich data types to meet your programming needs.
Subscribe to my newsletter
Read articles from Nabaranjan palatasingh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Nabaranjan palatasingh
Nabaranjan palatasingh
Aspiring Data Scientist | Machine Learning | AI | DevOps