Building Cryptocurrency Apps with Flutter: A Developer’s Guide

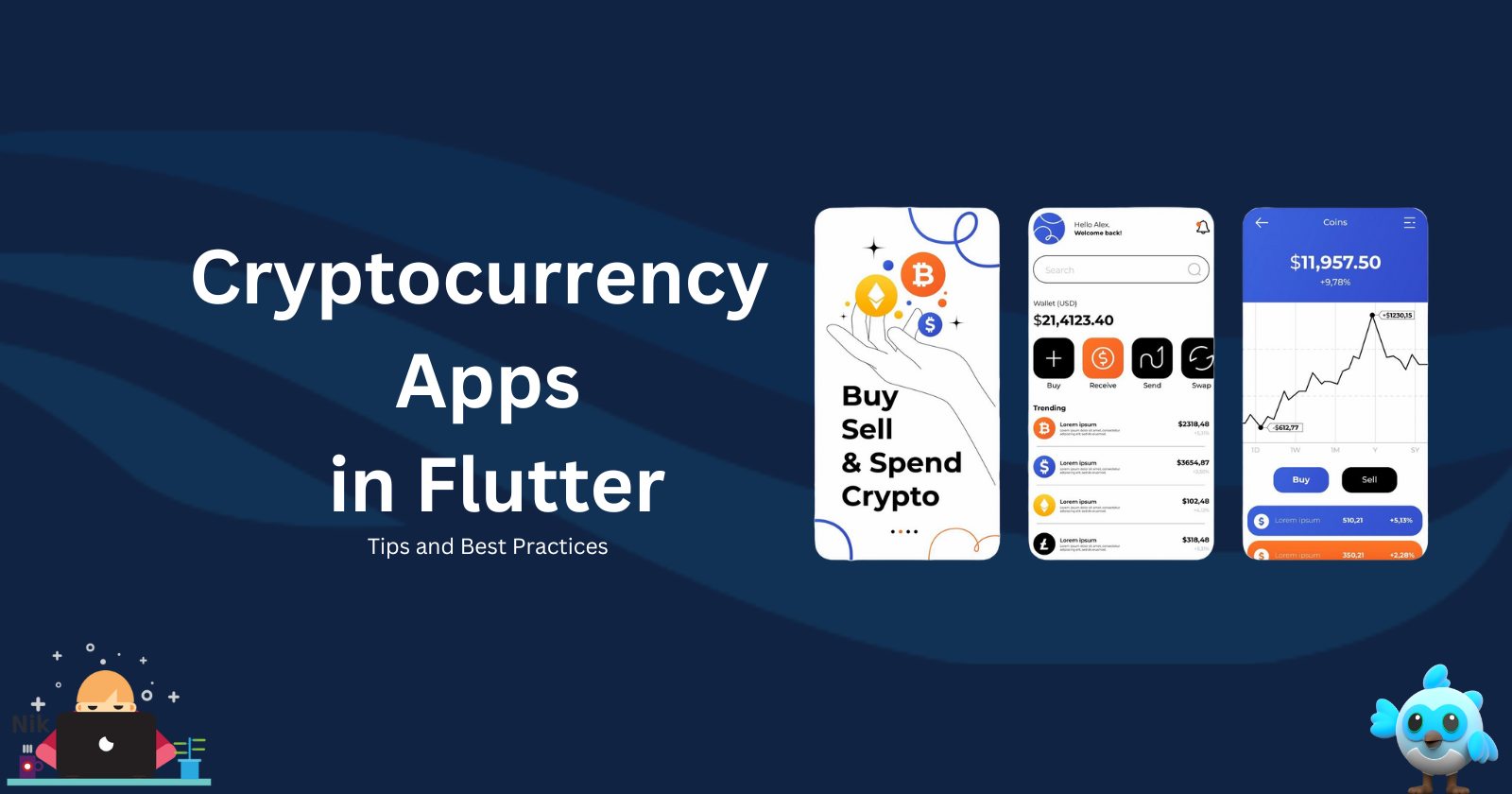
Cryptocurrency has been gaining global traction, transforming from a niche concept into a multi-billion-dollar industry. Developers, now more than ever, are tasked with building apps that track prices, manage wallets, and perform crypto transactions. Flutter, with its cross-platform capabilities and robust development environment, offers an excellent framework for building secure, fast, and user-friendly cryptocurrency apps. In this blog, we’ll explore how to build cryptocurrency-related applications using Flutter, best practices for ensuring performance, and tips for integrating crypto APIs.
Why Choose Flutter for Cryptocurrency Apps?
In the world of cryptocurrency, time-sensitive data like price fluctuations, quick transactions, and market trends are crucial. Flutter provides a real-time, reactive framework that allows developers to craft responsive UIs and deliver performance-driven apps that work seamlessly on both Android and iOS platforms. Here’s why Flutter is perfect for this:
Cross-Platform Development: Flutter allows you to write once and deploy on multiple platforms, saving time and resources.
Fast Performance: With the Dart language and Flutter’s direct compilation to native ARM code, apps built on Flutter are highly performant and perfect for real-time data handling.
Expressive UIs: Flutter’s widget-based architecture makes it simple to create rich, customizable UIs, which is essential for displaying detailed cryptocurrency market data in an intuitive format.
Wide Ecosystem: With a variety of ready-made packages and libraries available, developers can easily integrate APIs, authentication systems, and blockchain features.
Getting Started: Core Components of a Crypto App
In a typical cryptocurrency app, you will need to integrate the following functionalities:
1.Live Price Tracking
A vital part of any crypto app is real-time price tracking. You can integrate APIs from popular cryptocurrency data providers like CoinGecko, CoinMarketCap, or CryptoCompare. Flutter’s http
package makes this integration seamless, allowing you to fetch live data and update the UI dynamically.
Example:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<Map<String, dynamic>> getCryptoPrices() async {
final response = await http.get(Uri.parse('https://api.coingecko.com/api/v3/coins/markets?vs_currency=usd'));
if (response.statusCode == 200) {
return json.decode(response.body);
} else {
throw Exception('Failed to load prices');
}
}
2. Wallet Management
Allowing users to store, send, and receive cryptocurrencies is essential. While Flutter doesn’t natively support blockchain interactions, integrating third-party libraries such as Web3dart for Ethereum-based assets or Bitcoin libraries like BitCoinJS for wallet operations makes this achievable.
Example:
import 'package:web3dart/web3dart.dart';
var apiUrl = "https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID"; // Ethereum Mainnet
var httpClient = new Client();
var ethClient = new Web3Client(apiUrl, httpClient);
// Function to get Ethereum balance
Future<void> getBalance(String address) async {
EthereumAddress ethAddress = EthereumAddress.fromHex(address);
var balance = await ethClient.getBalance(ethAddress);
print('Balance: ${balance.getValueInUnit(EtherUnit.ether)}');
}
3. Portfolio Management
Users will want to track their cryptocurrency investments. You can provide features such as portfolio management, where users can add cryptocurrencies to their portfolio, track historical data, and analyze trends.
Best Practice:
Use Flutter’s provider
or riverpod
packages for managing the state of the user’s portfolio. This will ensure that the app remains performant even as the number of tracked assets grows.
Real-Time Price Notifications and Alerts
Push notifications are essential in crypto apps for price alerts. Users can set thresholds for specific coins and get notified when prices cross certain levels. You can implement this using Flutter’s firebase_messaging
package to send and manage real-time notifications.
Example of integrating Firebase Messaging:
import 'package:firebase_messaging/firebase_messaging.dart';
void setupFirebaseMessaging() {
FirebaseMessaging messaging = FirebaseMessaging.instance;
messaging.subscribeToTopic('crypto_updates');
FirebaseMessaging.onMessage.listen((RemoteMessage message) {
print('Got a message: ${message.notification?.title}');
});
}
Security Considerations
In any app dealing with financial assets, security is paramount. Here are key aspects to focus on when building a crypto app:
Secure API Calls: Always use HTTPS and ensure that sensitive data (such as API keys or wallet information) is encrypted.
User Authentication: Implement strong authentication mechanisms such as biometric logins, two-factor authentication (2FA), or OAuth using Flutter packages like
flutter_secure_storage
andfirebase_auth
.Data Encryption: Store sensitive information, such as private keys, securely using packages like
flutter_secure_storage
to encrypt data at rest.
Integrating Cryptocurrency Charts
Displaying historical price data in an interactive chart format can help users analyze market trends. Use Flutter’s fl_chart
or syncfusion_flutter_charts
packages to implement interactive charts.
Example of displaying a crypto price chart:
import 'package:fl_chart/fl_chart.dart';
LineChartData mainChart() {
return LineChartData(
lineBarsData: [
LineChartBarData(
spots: [
FlSpot(0, 1000),
FlSpot(1, 1100),
FlSpot(2, 1200),
FlSpot(3, 1300),
FlSpot(4, 1400),
],
),
],
);
}
Conclusion
Building cryptocurrency apps with Flutter is not only feasible but also highly rewarding. With its ability to create high-performance, cross-platform apps, Flutter allows developers to build beautiful, secure, and responsive cryptocurrency apps that can handle real-time data and transactions. Whether you’re working on a price-tracking app, a wallet, or a full-scale crypto portfolio manager, Flutter provides all the tools you need to create something impactful in the ever-evolving world of cryptocurrency.
#FlutterNik #DevsNik #Flutter #CryptoApps #Cryptocurrency #CrossPlatformDevelopment #CryptoWallet #CryptoPortfolio #RealTimeData #FlutterDev #FlutterCommunity
Subscribe to my newsletter
Read articles from Ravi Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ravi Patel
Ravi Patel
"📱 Passionate Flutter Developer with 6+ years of experience, dedicated to crafting exceptional mobile applications. 🚀 Adept at turning ideas into polished, user-friendly experiences using Flutter's magic. 🎨 Design enthusiast who believes in the power of aesthetics and functionality. 🛠️ Expertise in translating complex requirements into clean, efficient code. 🌟 Committed to staying updated with the latest trends and continuously pushing boundaries. Let's create something extraordinary together!"