React Day 7/40
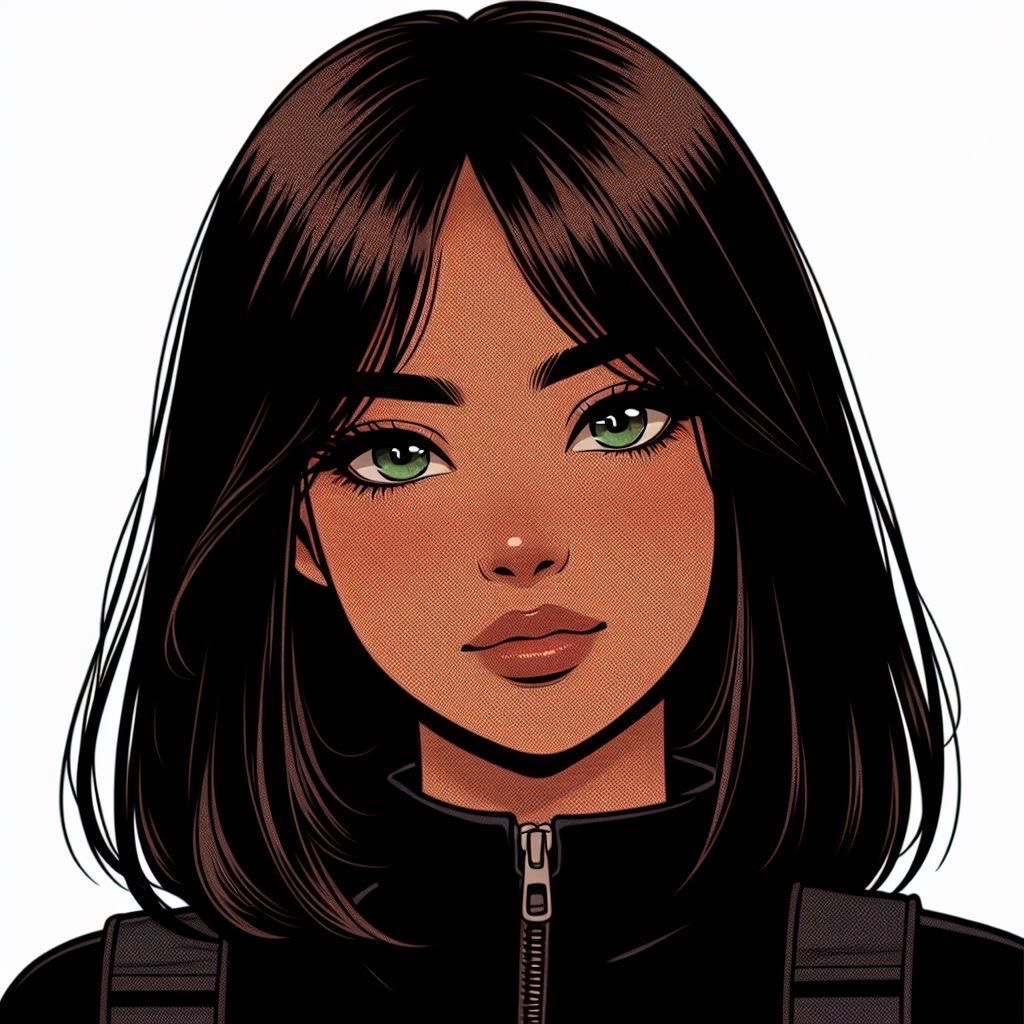
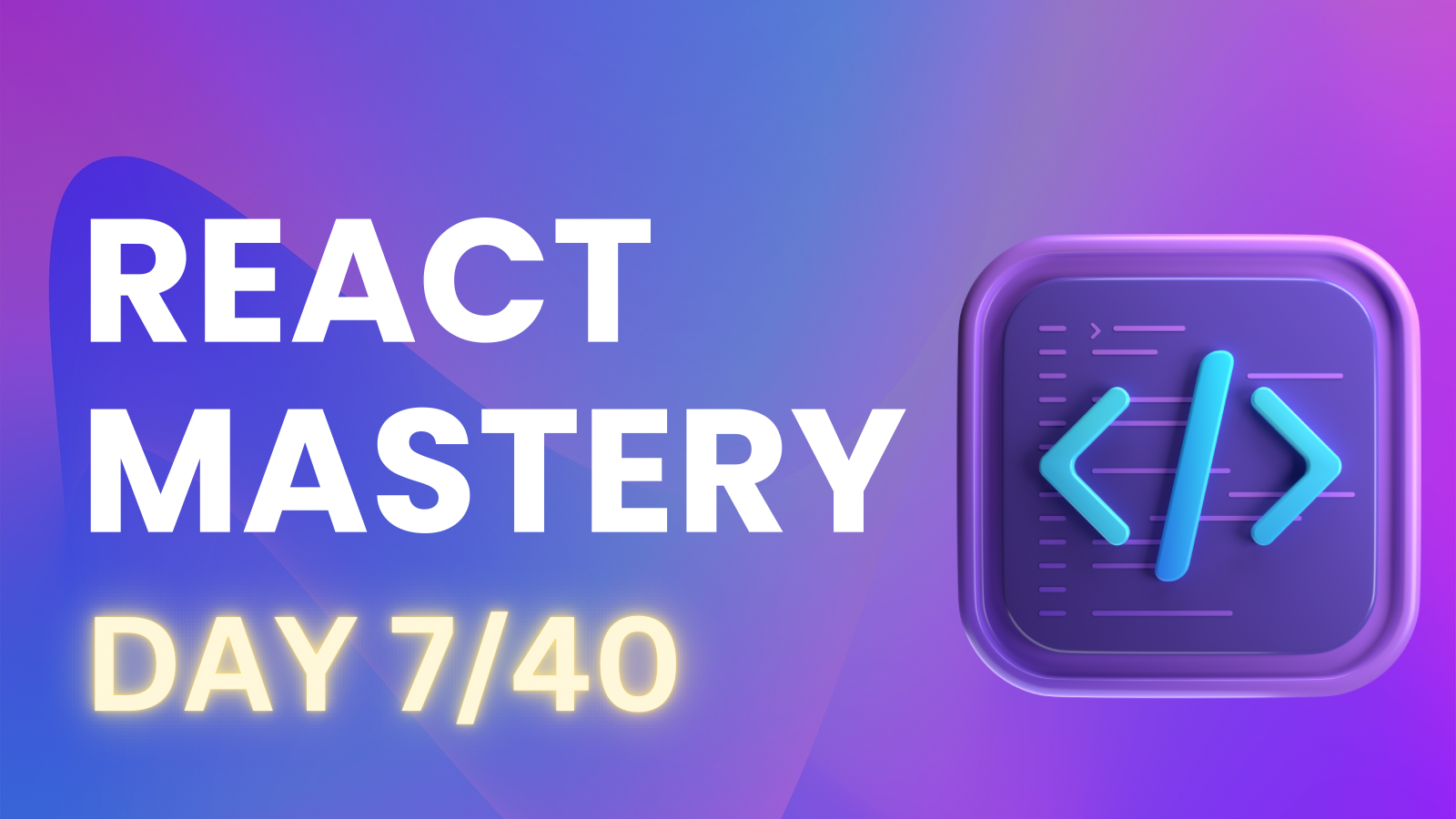
1st React Project
I built a simple project using Vite and Tailwind CSS that allows users to dynamically change the background color with the click of a button. While I used Tailwind for basic styling to make things visually appealing, the focus here is on functionality rather than design. I started by cleaning up the project to make it more organized.
The idea:
We want to change the background color. To do this, we need to store the color in a variable and make sure it updates in the UI. That’s why we’ll use useState.
const [color, setColor] = useState("black");
This creates a state variable called color
and sets it to “black” by default.
Applying the state in the UI:
Now, to use the color
variable in our App.jsx
file, we’ll pass it to the style
attribute inside the return
statement. This will apply the background color dynamically based on the current state.
Incorrect way:
<div style={{ backgroundColor: { color } }}>
This is incorrect because we are wrapping color
in curly braces { }
twice. In JSX, the first set of curly braces is used to write JavaScript inside the style
attribute, but the second set around color
is unnecessary and creates an object inside another object. This makes the code invalid.
Correct way:
<div style={{ backgroundColor: color }}>
This works because we're correctly telling the div
to use the value stored in color
for the backgroundColor
property.
Understanding the onClick Handler in React
The onClick
expects a function. So, when we write:
onClick={() => setColor("red")}
We use this arrow function because we need to pass a parameter (in this case, "red"
).
Why not just write onClick={setColor}
?
You could, but this only passes a reference to the function without calling it. That means it won’t allow us to pass parameters like "red"
.
What aboutonClick={setColor("red")}
?
The function would execute immediately when the component renders, not when the button is clicked. This happens because because we're calling the function right there. setColor("red")
returns the result of the function, but onClick
needs the function itself, not the result.
To fix this, we wrap setColor("red")
in an arrow function () => setColor("red")
, which creates a callback that only runs when the button is clicked.
Code Snippet
import { useState } from "react"
function App() {
const [color, setColor] = useState("grey")
return (
<>
<div className="w-full h-screen" style={{backgroundColor: color}}>
<div className="bg-slate-300 flex-wrap fixed mx-3 my-44 rounded-3xl">
<button onClick={() => setColor("red")}>red</button> <br />
<button onClick={() => setColor("blue")}>blue</button> <br />
<button onClick={() => setColor("green")}>green</button> <br />
</div>
</div>
</>
)
}
export default App
This was a very basic project aimed at understanding functionality rather than focusing on design. I didn't prioritize beautiful colors or buttons, but I learned how the click handler works and became more comfortable with building projects. I'm excited to create more projects with improved functionality and explore new concepts. Stay tuned for my next blog post, where I'll dive into more exciting features and share my journey as I continue to build and learn!
Subscribe to my newsletter
Read articles from Aaks directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
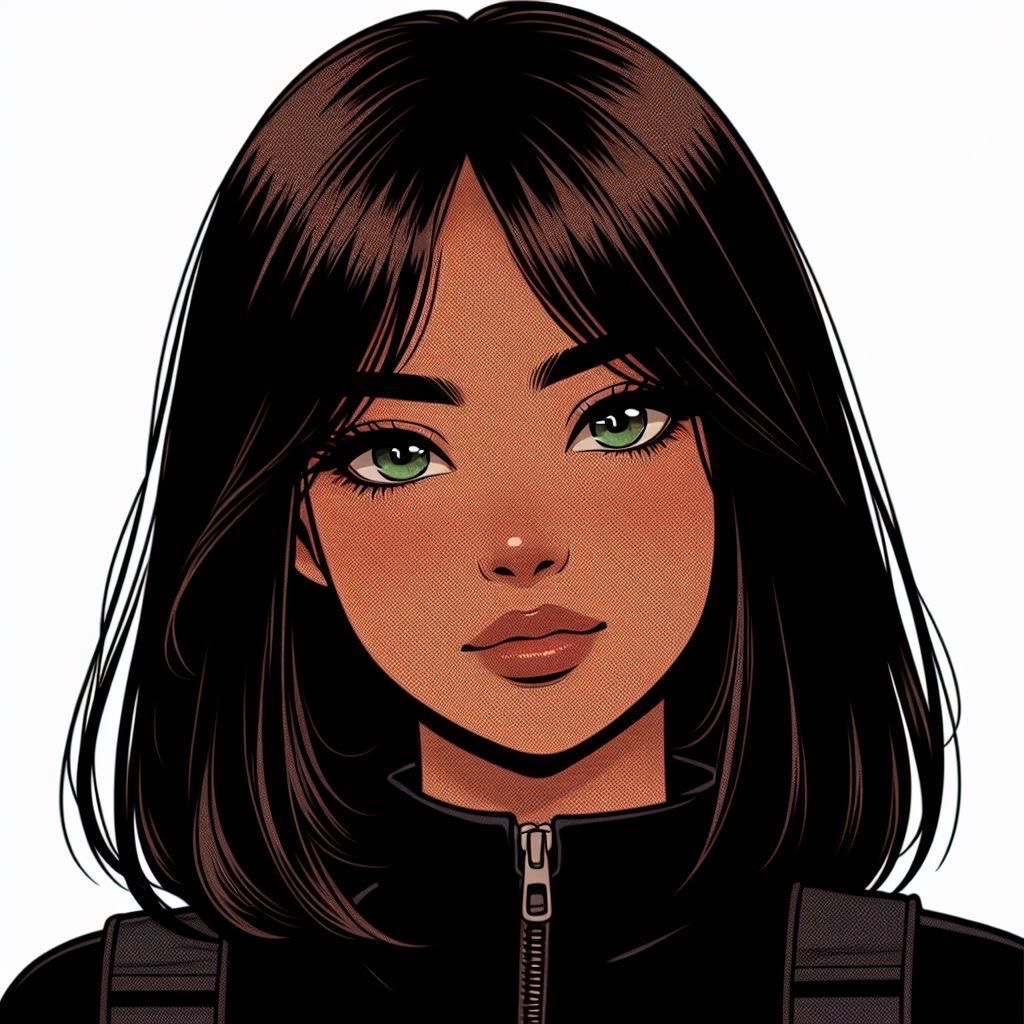