Dynamic Routes in Next.js: Understanding the Significance of params and searchParams

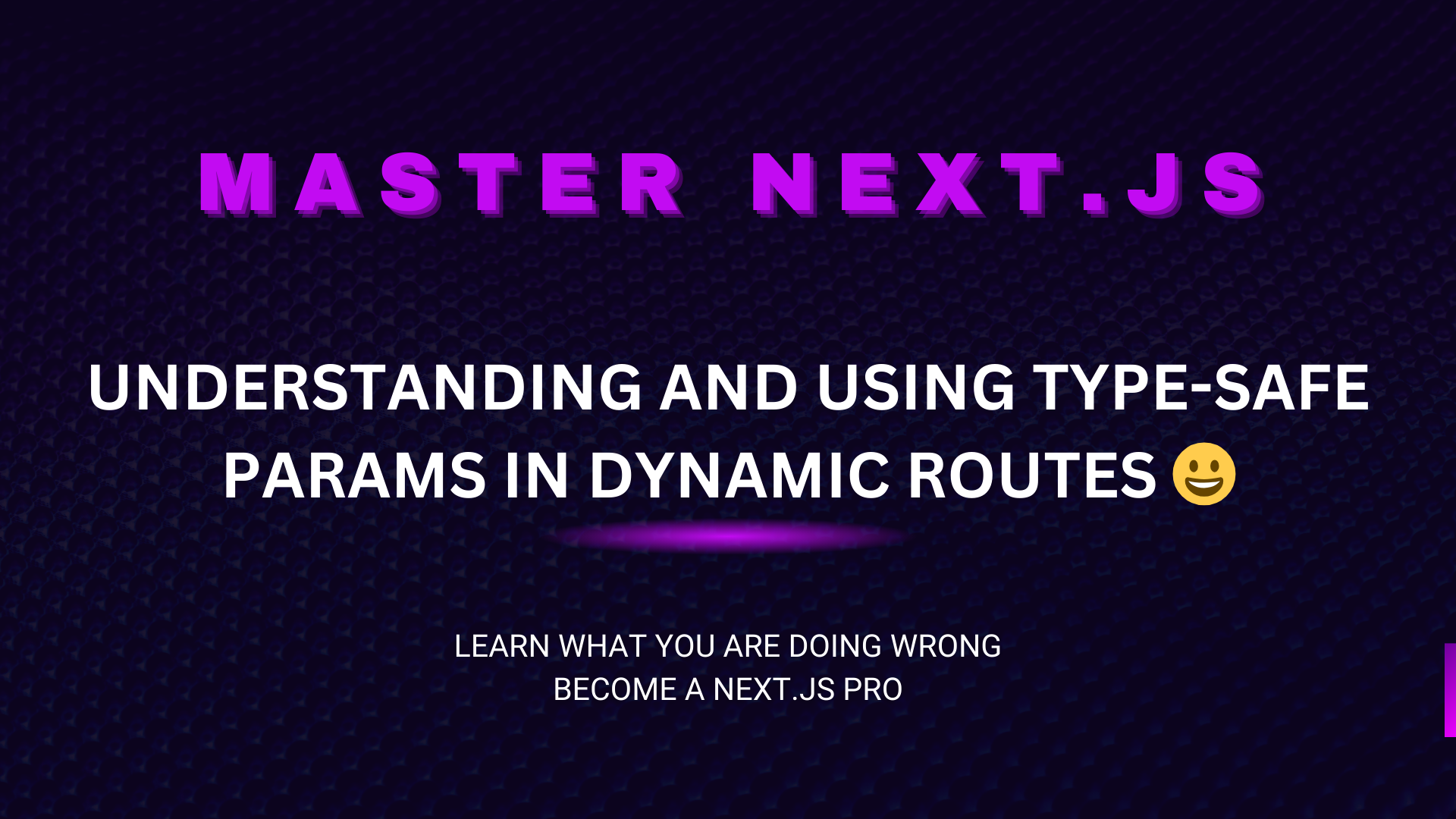
One common mistake developers make in Next.js is misunderstanding how dynamic routes work, particularly regarding the params
and searchParams
props. It’s crucial to understand that these props are only accessible in the page components and not in any other server components. Let’s explore this concept with intuitive examples.
What Are Dynamic Routes?
Dynamic routes in Next.js allow us to create pages that can change based on the URL parameters. For instance, if we want to create a product page that displays different products based on their IDs, we would set up a dynamic route.
Setting Up a Dynamic Route
To create a dynamic route in Next.js, we can structure our folder like this:
/app
└── /products
└── /[id]
└── page.tsx
In the example above, the [id]
folder indicates that it’s a dynamic route where id
is a placeholder for the actual product ID. This structure allows us to access the ID from the URL.
Accessing params
in a Page Component
Inside the page.tsx
file, we can access the dynamic parameter id
using the params
prop. Here’s how we can implement it:
// app/products/[id]/page.tsx
import { useEffect } from "react";
const ProductPage = ({ params }: { params: { id: string } }) => {
const productId = params.id;
useEffect(() => {
// Example fetch call using the productId
const fetchProduct = async () => {
const response = await fetch(`/api/products/${productId}`);
const productData = await response.json();
console.log(productData); // Handle product data
};
fetchProduct();
}, [productId]);
return (
<div>
<h1>Product ID: {productId}</h1>
{/* Display product details here */}
</div>
);
};
export default ProductPage;
Accessing searchParams
in a Page Component
Unlike params
, we don't need to create a special folder for searchParams
. These are available directly in the page component. For example, consider a URL like localhost:3000/products/3?color=green
. We can access the color
search parameter as follows:
// app/products/[id]/page.tsx
const ProductPage = ({ params, searchParams }: { params: { id: string }; searchParams: { color?: string } }) => {
const productId = params.id;
const color = searchParams.color;
return (
<div>
<h1>Product ID: {productId}</h1>
{color && <p>Selected Color: {color}</p>}
{/* Display product details based on color filter */}
</div>
);
};
export default ProductPage;
In this case, we access color
using searchParams.color
. This allows for shareable URLs with filters applied, making it easier to manage state in your application.
Key Takeaways
Accessing
params
andsearchParams
: These props can only be accessed in page components, not in other server components. Understanding this limitation is crucial for properly handling dynamic routes in Next.js.Dynamic Routing Structure: By structuring folders and files correctly, you can utilize dynamic routing to create responsive applications.
Using Search Parameters: Search parameters enhance the user experience by allowing filtering and customization of data displayed based on user selections.
Type Safety: Type your
params
andsearchParams
for better TypeScript support and to catch potential errors at compile time.
Conclusion
Understanding how to work with params
and searchParams
in Next.js is essential for effective dynamic routing. By recognizing that these props are only available in page components, you can better structure your applications and avoid common pitfalls. This knowledge will enhance your ability to build dynamic, data-driven applications with Next.js.
Subscribe to my newsletter
Read articles from Rishi Bakshi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Rishi Bakshi
Rishi Bakshi
Full Stack Developer with experience in building end-to-end encrypted chat services. Currently dedicated in improving my DSA skills to become a better problem solver and deliver more efficient, scalable solutions.