Create a Voucher in X++
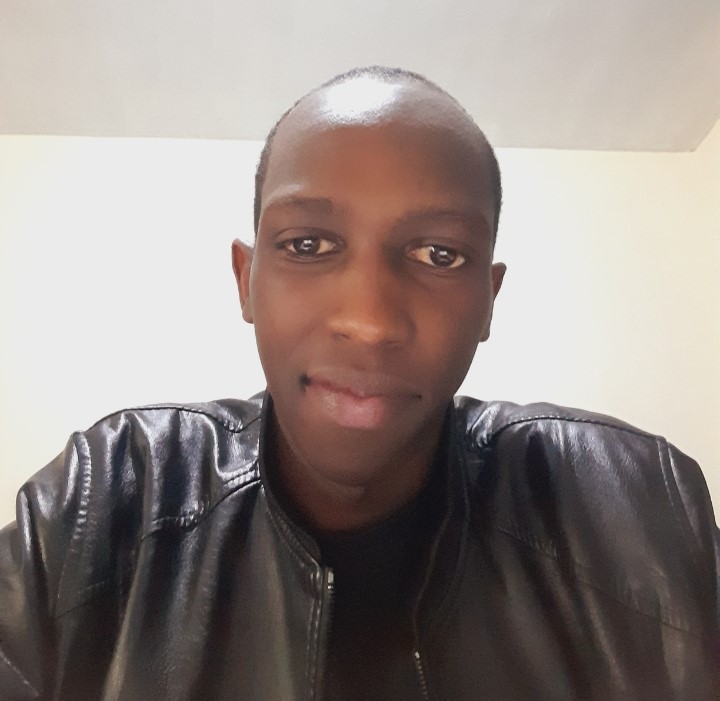
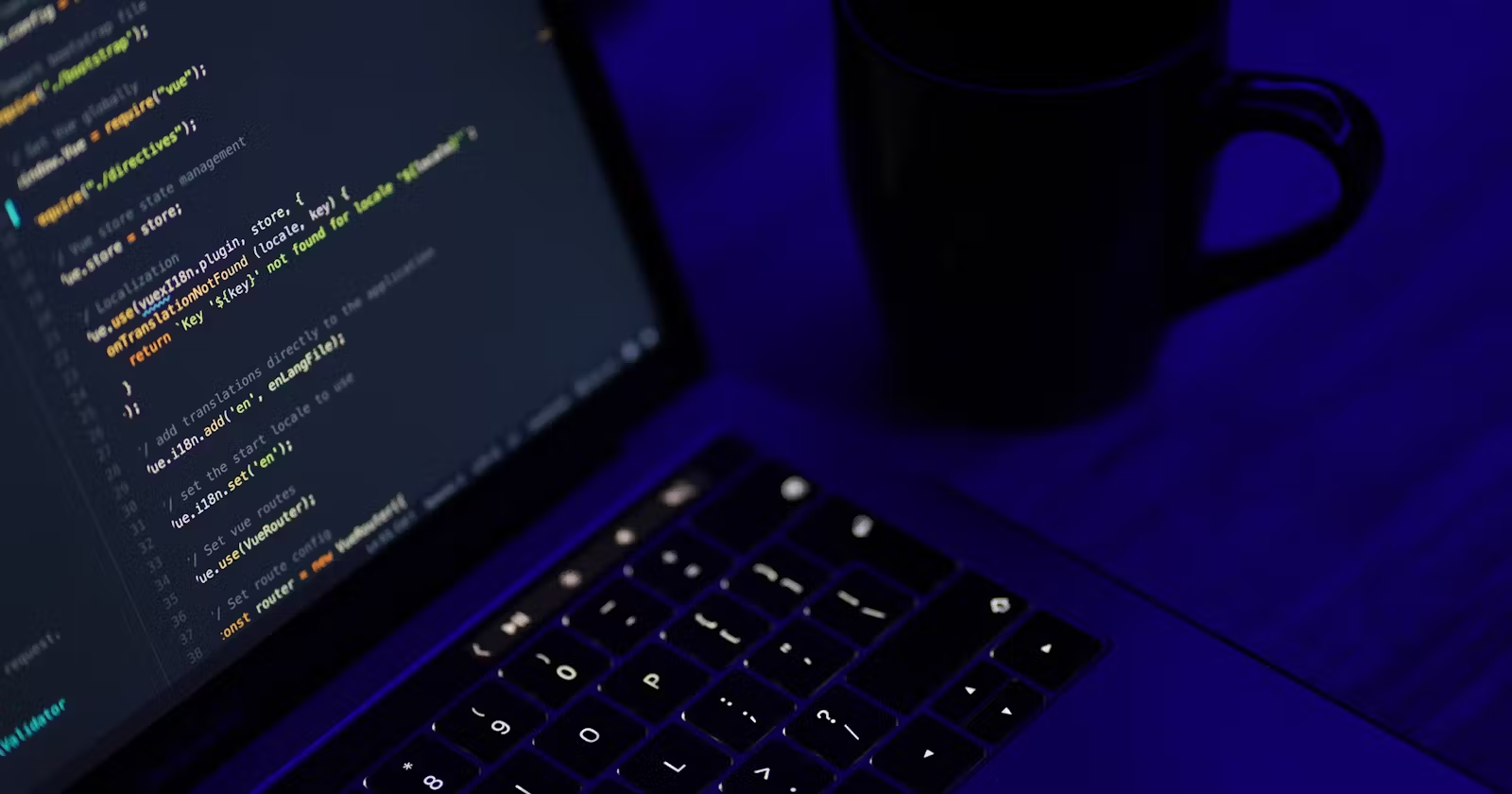
Creating a voucher in X++ without having to create a journal.
Initialize Voucher Details
Initializes key details like the amount, ledger account, vendor account, posting types, and original voucher. The VoucherTrans
method is called with these parameters to create a voucher.
Amount amount = journalAccountEntry.ReportingCurrencyAmount;
LedgerPostingType postingMain = journalAccountEntry.PostingType;
LedgerPostingType postingOffset = LedgerPostingType::VendOffsetAccount;
CurrencyCode currency = journalAccountEntry.Currency;
DimensionDynamicAccount ledgerAccount = journalAccountEntry.ledgerAccount;
DimensionDynamicAccount vendorAccount = journalAccountEntry.vendorAccount ;
Calling the VoucherTrans
method
Next, you call the VoucherTrans
helper method, passing the initialized values as arguments.
Voucher voucher = IBSProductRecieptDeemedVAT::VoucherTrans(
amount,
currency,
ledgerAccount,
vendorAccount,
postingMain,
postingOffset
);
Method to create the voucher
The VoucherTrans
method handles the actual creation of the voucher. It defines the main and offset transactions and then posts them to the ledger. The method returns the newly created voucher.
public static Voucher VoucherTrans(
Amount amountInReportingCurrency,
Amount amountUSD,
CurrencyCode currency,
DimensionDynamicAccount ledgerAccount,
DimensionDynamicAccount vendorAccount,
LedgerPostingType postingMain,
LedgerPostingType postingOffset
)
{
LedgerVoucher voucherLedger;
LedgerVoucherObject voucherObj;
LedgerVoucherTransObject voucherTrObj1;
LedgerVoucherTransObject voucherTrObj2;
NumberSeq numberSeq;
Voucher voucher;
numberSeq = NumberSeq::NewGetVoucherFromCode('Acco_139');
voucher = numberSeq.voucher();
voucherLedger = LedgerVoucher::newLedgerPost(DetailSummary::Detail, SysModule::Ledger, voucher);
voucherObj = LedgerVoucherObject::newVoucher(voucher);
voucherLedger.addVoucher(voucherObj);
// Main Account
voucherTrObj1 = LedgerVoucherTransObject::newBasicDefault(
voucherObj,
postingMain,
ledgerAccount,
currency,
-amountInReportingCurrency,
0, 0
);
// Offset Account
voucherTrObj2 = LedgerVoucherTransObject::newBasicDefault(
voucherObj,
postingOffset,
vendorAccount,
currency,
amountInReportingCurrency,
0, 0
);
voucherLedger.addTrans(voucherTrObj1);
voucherLedger.addTrans(voucherTrObj2);
info("Voucher transactions added successfully.");
voucherLedger.end();
return voucherObj.parmVoucher();
}
Conclusion
In conclusion, the above code creates a voucher. This is a requirement when you want a voucher transaction to appear without having to create a journal.
Subscribe to my newsletter
Read articles from Donald Kibet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
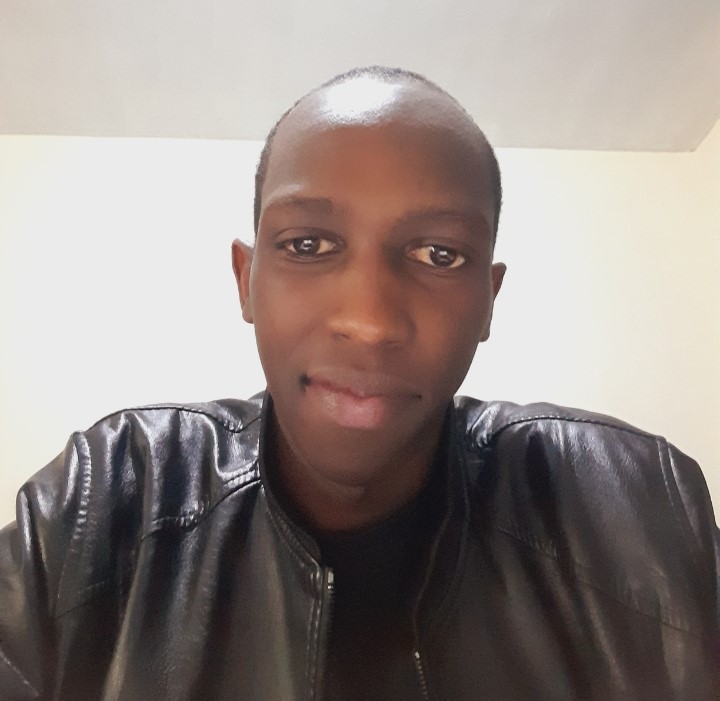
Donald Kibet
Donald Kibet
I'm a seasoned software developer specializing in customizing D365 F&O applications and creating impressive user interfaces using React and React Native for web and Android applications. Additionally, I develop secure and scalable APIs with Django (Python) or Spring Boot (Java).