useState

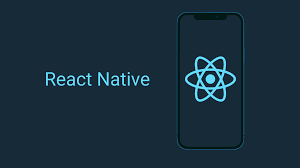
When you're building interactive applications with React, you often need a way to keep track of data that can change over time. For example, if you have a button that users can click to increase a counter, you'll need a way to store and update the counter's value. This is where useState
comes in!
useState
is a hook in React that allows you to add state to functional components. It’s the most basic and essential hook that helps you keep track of changes in your app.
What is State?
In React, state refers to a set of variables that determine how a component renders and behaves. When a state changes, React re-renders the component to reflect the new data. Think of state as the “memory” of your component.
For example, if you're building a to-do list app, the list of to-do items would be part of your component's state.
How useState
Works
Here’s the basic syntax for useState
:
const [stateVariable, setStateVariable] = useState(initialValue);
stateVariable
: This is the variable that holds the current state value.setStateVariable
: This is the function that allows you to update the state.initialValue
: This is the initial value that the state will have when the component is first rendered.
Example: A Simple Counter
Let’s look at an example where we use useState
to build a simple counter. Every time the button is clicked, the counter value will increase by 1.
import React, { useState } from 'react';
function Counter() {
// Declare a state variable called "count" with an initial value of 0
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
{/* When the button is clicked, we update the count */}
<button onClick={() => setCount(count + 1)}>
Click me
</button>
</div>
);
}
export default Counter;
Breakdown of the Example:
const [count, setCount] = useState(0)
: This line declares a state variablecount
initialized with the value0
. We also have a functionsetCount
to update the value ofcount
.<p>You clicked {count} times</p>
: This displays the current value of thecount
state variable.onClick={() => setCount(count + 1)}
: When the button is clicked, thesetCount
function is called, which increases the value ofcount
by 1.
Each time the button is clicked, React updates the state, causing the component to re-render and display the updated count
value.
Updating State
When using useState
, updating the state doesn’t immediately change the state. Instead, it triggers a re-render of the component, which then updates with the new state. Here's an example of how the state updates over time:
const [count, setCount] = useState(0);
// Updating the state
setCount(count + 1); // count is now 1
setCount(count + 1); // count is now 2
Important: The state is updated asynchronously, meaning multiple updates are batched together to optimize performance. Therefore, when updating the state based on the current state, it's better to use the function version of setState
, like this:
setCount(prevCount => prevCount + 1);
This ensures you always have the latest state when updating it multiple times.
Initial State
The initial state value passed to useState
can be a primitive value (like a number, string, or boolean), an object, or even a function. For example, if you wanted to store a list of items:
const [items, setItems] = useState([]);
In this case, items
starts as an empty array, and you can later use setItems
to add items to the list.
Example: Managing an Array with useState
Here’s how you can use useState
to manage a list of tasks in a to-do app:
import React, { useState } from 'react';
function TodoApp() {
const [tasks, setTasks] = useState([]);
const [task, setTask] = useState('');
const addTask = () => {
setTasks([...tasks, task]); // Add the new task to the existing list of tasks
setTask(''); // Clear the input field after adding the task
};
return (
<div>
<input
type="text"
value={task}
onChange={(e) => setTask(e.target.value)} // Update the "task" state when the input changes
/>
<button onClick={addTask}>Add Task</button>
<ul>
{tasks.map((t, index) => (
<li key={index}>{t}</li>
))}
</ul>
</div>
);
}
export default TodoApp;
How it Works:
The state variable
tasks
is used to keep track of the list of tasks.When the Add Task button is clicked, the current task is added to the list, and the input field is cleared.
The tasks are displayed in a list using the
map
function to iterate over thetasks
array.
Multiple States in One Component
You can use useState
multiple times in a single component to manage different pieces of state.
For example:
const [count, setCount] = useState(0);
const [name, setName] = useState('');
This component now has two independent pieces of state: count
and name
. Each can be updated separately without affecting the other.
Summary
useState
is a React hook that allows you to add state to functional components.It provides an array with two values: the current state value and a function to update that state.
The state is updated asynchronously, and updating the state triggers a re-render of the component.
You can use
useState
to store primitive values (numbers, strings) or more complex data like arrays and objects.You can have multiple
useState
hooks to handle different pieces of state in a component.
By understanding how useState
works, you can create dynamic, interactive React components that respond to user input and other changes in real-time.
Subscribe to my newsletter
Read articles from Ibrahim Abdullahi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
