Two pointer with Golang
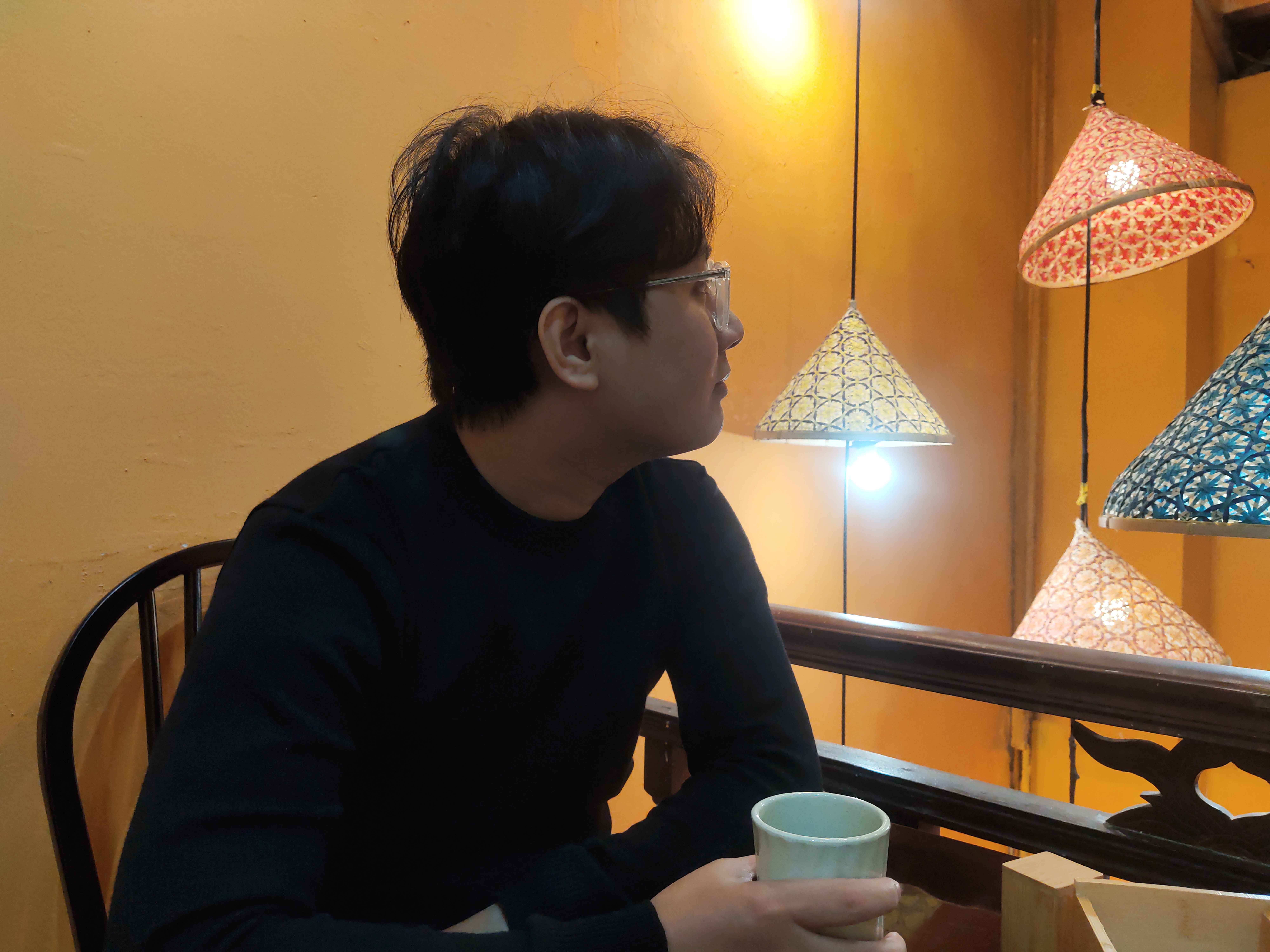
1 min read
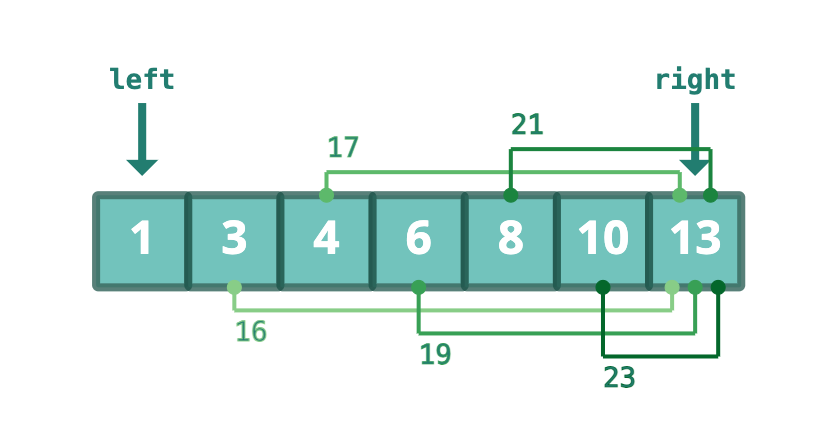
Write a function that reverses a string. The input string is given as an array of characters s
.
You must do this by modifying the input array in-place with O(1)
extra memory.
Example 1:
Input: s = ["h","e","l","l","o"]
Output: ["o","l","l","e","h"]
Example 2:
Input: s = ["H","a","n","n","a","h"]
Output: ["h","a","n","n","a","H"]
Constraints:
1 <= s.length <= 10<sup>5</sup>
s[i]
is a printable ascii character.
Reference: https://leetcode.com/problems/reverse-string/description/
Idea:
Implement with Golang:
package main
import "fmt"
func reverse[T comparable](arr []T) []T {
left, right := 0, len(arr)-1
for left < right {
arr[left], arr[right] = arr[right], arr[left]
left++
right--
}
return arr
}
func main() {
arr := []int{1, 2, 3, 4, 5, 6, 7, 8, 9}
fmt.Println(reverse(arr))
}
0
Subscribe to my newsletter
Read articles from Nguyen Van Tuan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
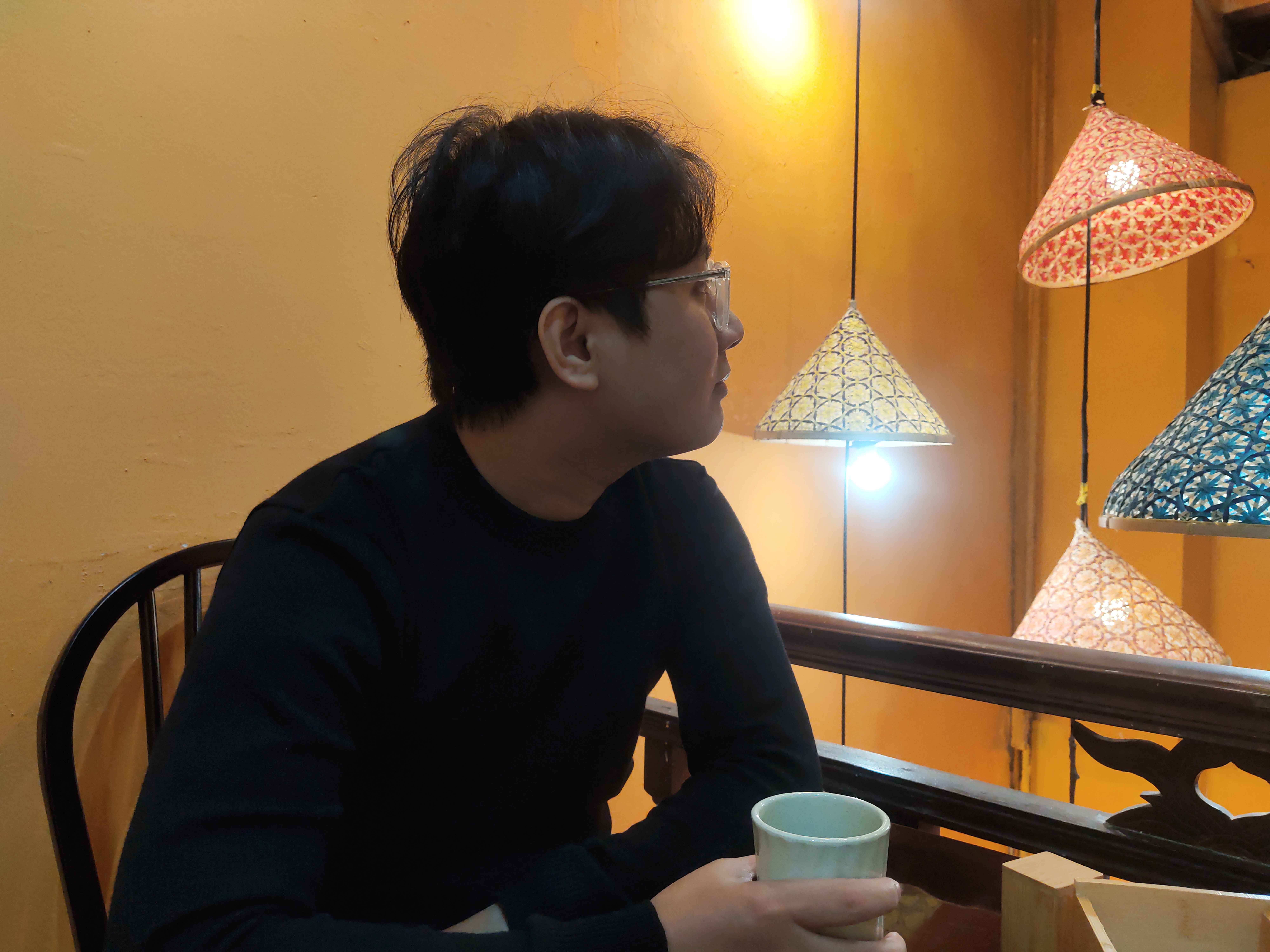
Nguyen Van Tuan
Nguyen Van Tuan
I'm Tuan. I graduated Hanoi University of Science and Technology in 2019 Major: Information Technology Leetcode : nguyenvantuan2391996 My blog: https://tuannguyenhust.hashnode.dev/ Linkedin : Tuan Nguyen Van