Understanding WebRTC Behind the Scenes: A Developer’s Journey from Confusion to Clarity

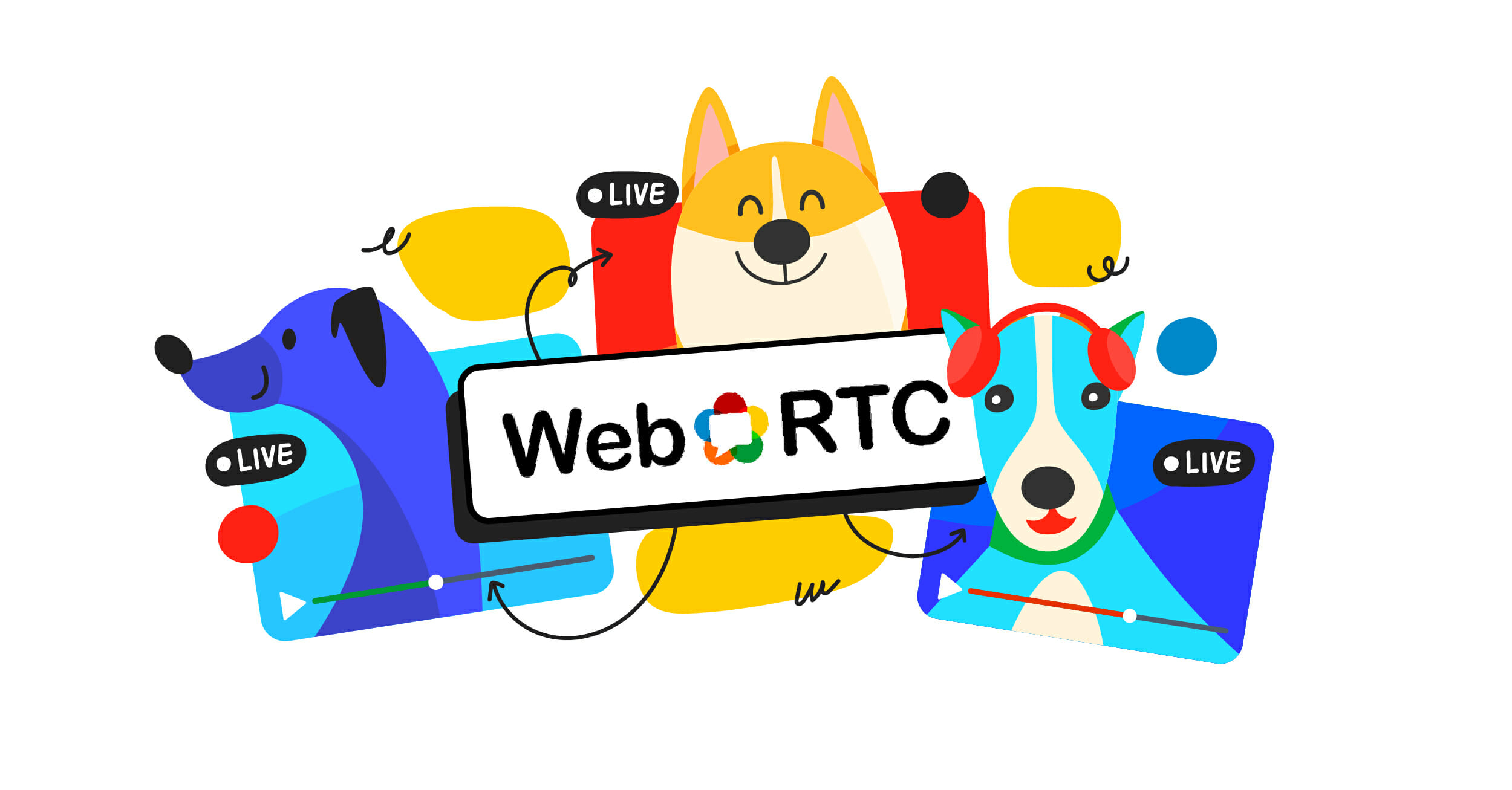
You can easily find various YouTube tutorials explaining the basics of how WebRTC works with diagrams and high-level overviews. However, if you want to dive deeper and truly understand how WebRTC works behind the scenes, clear up some common confusions, and explore real coding flow, this article is for you.
WebRTC (Web Real-Time Communication) is an incredible technology that enables browsers to communicate directly with each other in real-time, without relying on a central server. It powers video calls, file transfers, and media streaming, all within the browser. However, getting a true understanding of how this works behind the scenes is both intriguing and challenging.
When I first worked with WebRTC, several questions arose. The biggest confusion? How do browsers communicate with each other without a server, and do both browsers need to run specific code to make this work? To truly grasp this, let’s walk through the technical workings of WebRTC step by step and answer these questions in a developer-friendly way.
Top Technologies Using WebRTC
Before diving into the technical details, it’s important to note that WebRTC is used by some of the biggest tech companies and services for real-time communication:
Google Meet and Google Duo for video conferencing
Discord for voice and video communication
Slack for integrated voice and video chats
Facebook Messenger and WhatsApp for video calling
Zoom (as part of their web client)
These platforms rely on WebRTC to deliver low-latency, peer-to-peer video, and voice communications without relying on traditional centralized servers to handle every part of the interaction.
1. Core Confusion: Do Both Browsers Need to Run Code?
In my journey, one of the first things that puzzled me was how WebRTC communication happens between browsers. I thought maybe one browser would act like a server and handle requests, much like in a client-server model. However, I quickly realized that both browsers must actively run WebRTC code to establish a connection. They need to create offers, accept incoming connections, and exchange media or data in real time.
Understanding the Code Flow
For WebRTC to work, both peers (browsers) need to execute JavaScript and HTML code. Unlike the usual client-server architecture where the server is always running, in WebRTC, both browsers are equal participants in the connection. If one side isn't running its code, the connection simply won't happen. Let's break this down.
WebRTC Code Breakdown
Here's a simple, detailed WebRTC code example that explains how each part works:
Step 1: Accessing Local Media
Before browsers can communicate, they need to access media devices like cameras or microphones. This is done using the getUserMedia()
API. Both browsers capture audio and video streams that will later be sent to each other.
navigator.mediaDevices.getUserMedia({ video: true, audio: true })
.then((stream) => {
// Attach the stream to the local video element
localVideo.srcObject = stream;
// Add the stream to the peer connection
peerConnection.addStream(stream);
})
.catch((error) => {
console.error("Error accessing media devices.", error);
});
Step 2: Creating and Exchanging an Offer
One browser (let’s call it Peer A) initiates the connection by creating an "offer" using RTCPeerConnection.createOffer()
. This offer is then sent to the other browser (Peer B) using a signaling server.
peerConnection.createOffer()
.then((offer) => peerConnection.setLocalDescription(offer))
.then(() => {
// Send the offer to Peer B through the signaling server
});
Step 3: Receiving and Answering the Offer
When Peer B receives the offer, it creates an "answer" using RTCPeerConnection.createAnswer()
and sends it back to Peer A.
peerConnection.createAnswer()
.then((answer) => peerConnection.setLocalDescription(answer))
.then(() => {
// Send the answer back to Peer A through the signaling server
});
Step 4: Exchanging ICE Candidates
To establish a peer-to-peer connection, both browsers exchange ICE candidates. These candidates help browsers figure out the best network routes to communicate directly.
peerConnection.onicecandidate = (event) => {
if (event.candidate) {
// Send the ICE candidate to the other peer via the signaling server
}
};
Step 5: Receiving Remote Media
Once the connection is established, the media streams from the remote peer are attached to the respective video element to display the incoming video.
peerConnection.ontrack = (event) => {
remoteVideo.srcObject = event.streams[0];
};
This is the general flow of a WebRTC connection from start to finish. Now, let’s address how WebRTC makes browsers behave like temporary servers.
2. How WebRTC Makes Browsers Act Like Temporary Servers
While working with WebRTC, I began thinking: Are browsers becoming temporary servers? In some ways, yes. WebRTC allows browsers to send and receive data without the need for a middleman server after the initial signaling phase. This temporary server-like behavior is what makes WebRTC unique.
But there’s a difference. Browsers don’t become full-fledged servers like a traditional web server. Instead, they act as peers that both send and receive data directly from each other. WebRTC uses the concept of peer-to-peer communication, but both browsers must be active for it to work.
Why Browsers Can Act as Temporary Servers
Handling Requests and Responses: Once the connection is established, browsers can handle media streams, data requests, and responses without external servers. This means each browser is both sending and receiving data just like a server would.
Decentralized Communication: In traditional web communication, everything flows through a server. In WebRTC, the communication is direct between browsers, reducing the reliance on external servers and increasing the efficiency of real-time communication.
This concept of making browsers behave like temporary servers is revolutionary because it allows real-time communication while offloading the processing load from central servers to users' browsers.
3. The Inception of WebRTC: How the Idea Originated
While we know WebRTC empowers browsers to communicate in real-time, it’s fascinating to think about how this concept came into being. Before WebRTC, the standard model was client-server communication. Developers began thinking, "If browsers can send requests and receive responses, why can’t they communicate directly with each other?"
That’s where WebRTC comes in. The idea of turning browsers into participants that can exchange data directly was revolutionary. This decentralized approach mimics server-like behavior without the overhead of running a full server.
4. Conclusion: Turning Browsers Into Temporary Servers
WebRTC gives browsers temporary, limited server-like capabilities by enabling them to communicate directly with each other. While they don’t act as full servers, browsers can send and receive real-time data (audio, video, or files) without needing a server for the data exchange itself. The key takeaway is that browsers become peers rather than traditional clients, reducing server load and enabling more decentralized communication.
For developers, this means building applications that let users communicate or share data in real-time—from video chats to file-sharing apps—using only their browsers. Understanding the mechanics of WebRTC opens up endless possibilities for decentralized communication applications, while minimizing server dependency.
Final Thoughts
By understanding the basics of WebRTC and how signaling works, you can create powerful peer-to-peer applications that reduce the reliance on traditional servers. Though there are some limitations, this technology opens the door to new, decentralized possibilities for web applications.
This article now seamlessly incorporates your thoughts, solving the confusions you had and emphasizing how WebRTC works behind the scenes, transforming browsers into temporary servers. It balances both the technical explanation and the conceptual idea of decentralized communication, along with code samples for clarity.
Subscribe to my newsletter
Read articles from ritiksharmaaa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

ritiksharmaaa
ritiksharmaaa
Hy this is me Ritik sharma . i am software developer