Mastering Data Binding in Angular: Parent-Child Communication, Interpolation, and More
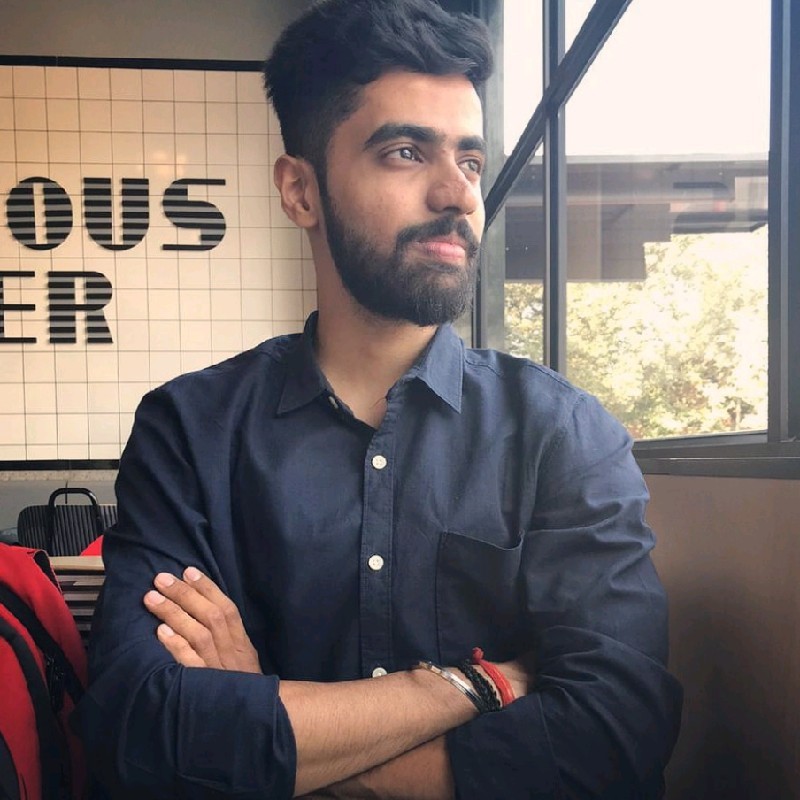
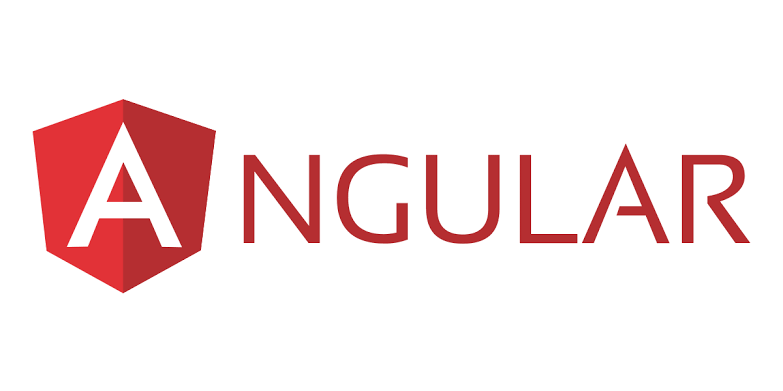
Data binding in Angular is a powerful way to manage the flow of data between your TypeScript logic and the UI in your templates. It allows for seamless interaction between components and their views.
In this post, we’ll cover the following essential concepts:
Parent-Child Communication
String Interpolation
{{}}
One-Way Binding (Custom Binding, Property Binding)
Two-Way Binding
[(ngModel)]
Let’s dive in and explore each of these concepts with code examples!
1. Parent-Child Communication in Angular
Angular uses Input and Output decorators to manage communication between parent and child components.
Example:
Parent Component (app.component.html):
<app-child [childMessage]="messageFromParent" (messageEvent)="receiveMessage($event)"></app-child>
<p>Message from child: {{ receivedMessage }}</p>
Parent Component (app.component.ts):
export class AppComponent {
messageFromParent = "Hello from Parent!";
receivedMessage: string;
receiveMessage($event: string) {
this.receivedMessage = $event;
}
}
Child Component (child.component.ts):
export class ChildComponent {
@Input() childMessage: string;
@Output() messageEvent = new EventEmitter<string>();
sendMessage() {
this.messageEvent.emit('Hello from Child!');
}
}
Child Component (child.component.html):
<p>Message from parent: {{ childMessage }}</p>
<button (click)="sendMessage()">Send Message to Parent</button>
In this example:
The parent passes a message to the child using the
@Input()
decorator.The child sends a message back to the parent using
@Output()
andEventEmitter
.
2. String Interpolation {{}}
String interpolation is used to bind data from the component’s TypeScript class to the template.
Example:
app.component.ts:
export class AppComponent {
title = 'Data Binding in Angular';
}
app.component.html:
<h1>{{ title }}</h1>
Here, the value of the title
property in the component is rendered inside the h1
element using {{}}
. It’s a straightforward and clean way to bind data from your component.
3. One-Way Binding
One-way data binding in Angular refers to when the data flows in a single direction: from the component class to the view or vice versa.
Property Binding
Property binding allows you to bind values to HTML element properties.
Example:
app.component.ts:
export class AppComponent {
isDisabled = true;
}
app.component.html:
<button [disabled]="isDisabled">Click Me</button>
Here, the disabled
property of the button element is bound to the isDisabled
property in the component. The button will be disabled if isDisabled
is true
.
Custom Binding (Event Binding)
With custom binding, you can listen to events such as clicks, key presses, or other DOM events, and trigger component methods accordingly.
Example:
app.component.html:
<button (click)="onClick()">Click Me</button>
<p>{{ message }}</p>
app.component.ts:
export class AppComponent {
message = '';
onClick() {
this.message = 'Button was clicked!';
}
}
Here, the button click triggers the onClick()
method, which updates the message in the template.
4. Two-Way Binding with [(ngModel)]
Two-way data binding allows changes in the view to reflect in the component class, and changes in the component class to update the view automatically. It is commonly used in forms.
Example:
First, import FormsModule
into your module:
import { FormsModule } from '@angular/forms';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule, FormsModule],
bootstrap: [AppComponent]
})
export class AppModule { }
Then, implement two-way binding using [(ngModel)]
.
app.component.ts:
export class AppComponent {
name = '';
}
app.component.html:
<input [(ngModel)]="name" placeholder="Enter your name">
<p>Hello, {{ name }}!</p>
In this example:
The
[(ngModel)]
directive binds the input field to thename
property in the component.When the user types into the input field, the
name
property is updated in real time, and the updated value is reflected in thep
tag.
Summary
To wrap it up:
Parent-Child Communication uses
@Input()
and@Output()
decorators to share data between components.String Interpolation lets you insert dynamic values in your templates using
{{}}
.One-Way Binding (Property and Custom Binding) directs the flow of data from the component to the view or handles user events.
Two-Way Binding with
[(ngModel)]
synchronizes data between the view and the component class.
Mastering these concepts will make your Angular applications more interactive and dynamic. Data binding is one of the core principles that makes Angular such a powerful and flexible framework.
Happy coding! 🚀
Subscribe to my newsletter
Read articles from Shikhar Shukla directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
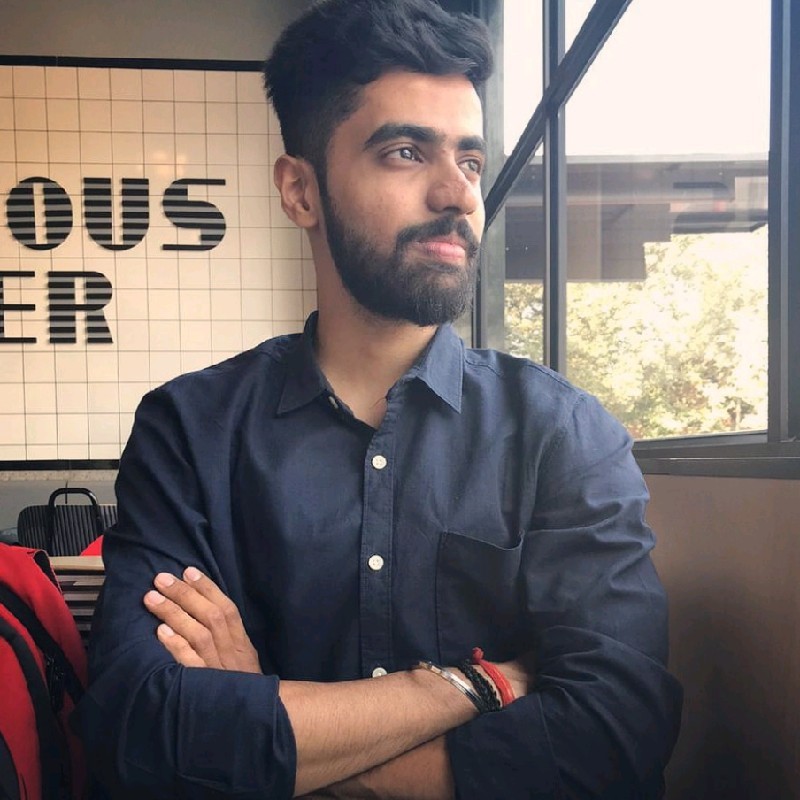