Queue, Java

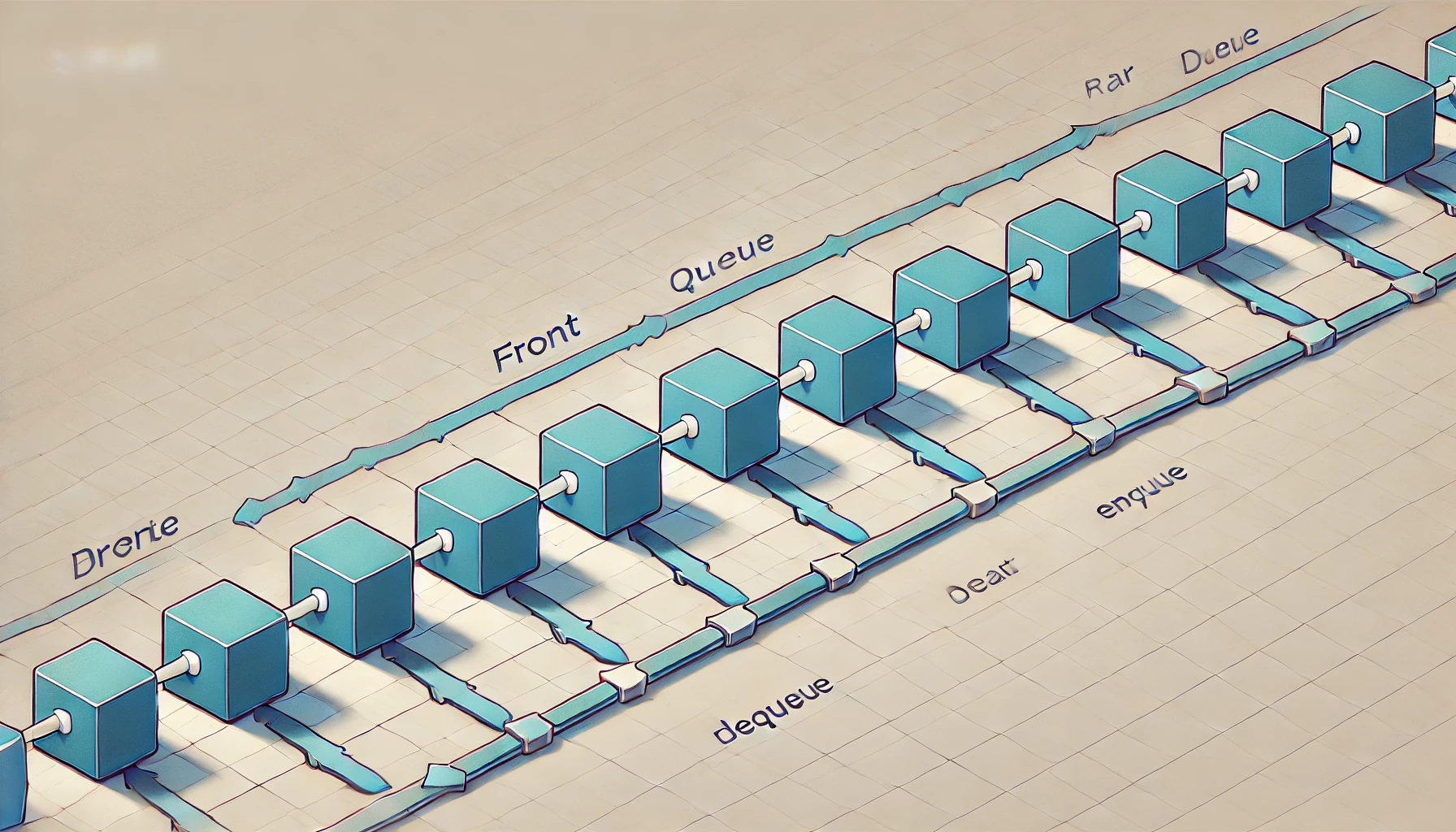
A Queue is a linear data structure that follows the First In First Out (FIFO) principle, meaning that the first element added to the queue will be the first one to be removed. This structure is widely used in various applications, including scheduling tasks, managing resources, and handling asynchronous data.
Types of Queues
Simple Queue:
Insertion occurs at the rear and removal occurs at the front, strictly following FIFO.
Example: line of customers at a service desk.
Circular Queue:
The last element points back to the first element, forming a circle.
This improves memory utilization by allowing new elements to be added in empty spaces created by dequeued elements.
Example: Buffer management in streaming data.
Priority Queue:
Each element is associated with a priority; elements are served based on their priority rather than their order in the queue.
If two elements have the same priority, they are served according to their order in the queue.
Example: Job scheduling in operating systems where high-priority tasks are executed first.
Double Ended Queue (Deque):
Insertion and removal can occur from both ends (front and rear).
This allows for more flexible data processing, supporting both stack and queue operations.
Example: Undo functionality in applications where actions can be reversed from either end.
Advantages of Using Queue
Order Preservation: Maintains the order of elements, which is crucial for many applications.
Efficient Operations: Enqueue and dequeue operations can be performed in constant time O(1).
Flexibility: Can be implemented using arrays or linked lists, allowing for dynamic resizing.
Disadvantages of Using Queue
Limited Access: Only allows access to the front element; other elements cannot be accessed directly.
Memory Consumption: If implemented using arrays, resizing can lead to memory wastage or overflow.
Complexity in Implementation: Implementing a queue from scratch requires careful management of indices (for array-based queues).
Implementation of Queue in Java
Java provides a built-inQueue
interface, which can be implemented using various classes such asLinkedList
,ArrayDeque
, etc. Below are examples demonstrating how to implement a queue both manually and using the Java Collections Framework.
Implementation of Queue
Java'sQueue
interface can be easily utilized with theLinkedList
class:
import java.util.LinkedList;
import java.util.Queue;
public class Main {
public static void main(String[] args) {
Queue<Integer> numbers = new LinkedList<>();
// Enqueue
numbers.offer(1);
numbers.offer(2);
numbers.offer(3);
System.out.println("Queue: " + numbers);
// Dequeue
int removedNumber = numbers.poll();
System.out.println("Removed Element: " + removedNumber);
System.out.println("Queue after deletion: " + numbers);
}
}
Usage of Queues
Queues are used in various scenarios, including:
Task Scheduling: Managing tasks in operating systems.
Buffering: Handling data streams in IO operations.
Breadth-First Search (BFS): In graph algorithms where nodes are explored level by level.
Conclusion
The queue data structure is essential for managing ordered collections of elements efficiently. With its FIFO nature, it serves numerous applications across computing and algorithm design. Java's built-in support for queues through interfaces and classes simplifies their implementation and usage, making them a powerful tool for developers. Understanding different types of queues enhances flexibility in solving various computational problems efficiently.
Subscribe to my newsletter
Read articles from Arpit Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arpit Singh
Arpit Singh
AI engineer at Proplens AI, a final year student pursuing bachelor's in computer science and engineering.