Scopes in JavaScript
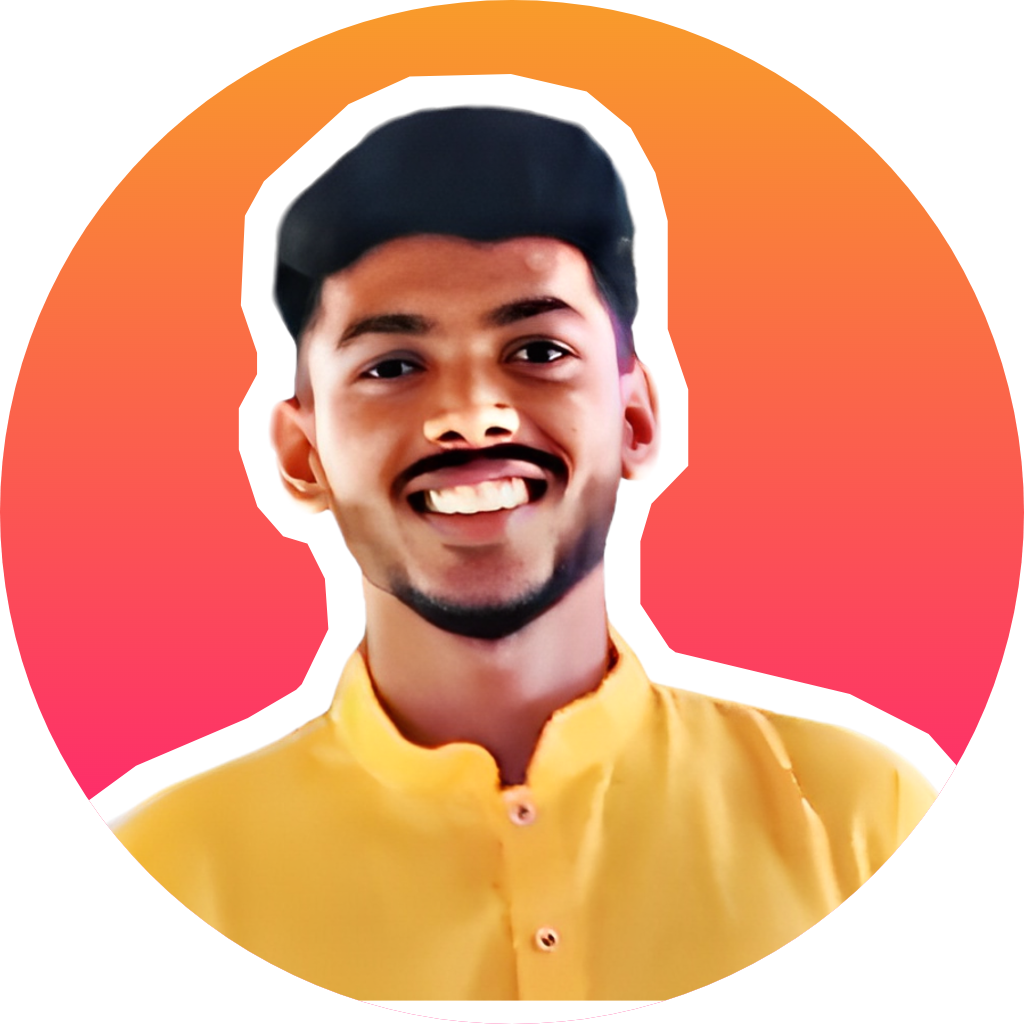
What are scopes?
In JavaScript, scope defines the accessibility or visibility of variables and functions at different parts of your code. Understanding scope is essential to ensure that variables are only available where needed and to avoid conflicts or errors.
There are three primary types of scopes in JavaScript:
1. Global Scope
A variable declared in the global context (i.e., outside of any function or block) has a global scope and is accessible from anywhere in the code.
Global variables: Variables declared without
let
,const
, orvar
automatically become global (which is generally not recommended for clean code).Declared global variables: Variables declared with
var
,let
, orconst
outside of any function are global.
Example:
javascriptCopy codelet globalVar = "I am global";
function displayGlobalVar() {
console.log(globalVar); // Accessible
}
displayGlobalVar(); // Output: I am global
2. Function Scope
A variable declared inside a function is only accessible within that function. This is called function scope.
var
declarations are function-scoped.Functions themselves create a new scope.
Example:
javascriptCopy codefunction myFunction() {
let localVar = "I am local";
console.log(localVar); // Accessible within the function
}
myFunction(); // Output: I am local
console.log(localVar); // Error: localVar is not defined
3. Block Scope (ES6)
With the introduction of ES6, let
and const
introduced block scope. Block scope refers to variables that are limited in scope to the block, statement, or expression in which they are used (e.g., inside {}
braces).
let
andconst
are block-scoped, meaning they are only accessible within the block they are declared in, such as loops,if
statements, or other code blocks.
Example:
javascriptCopy codeif (true) {
let blockVar = "I am block-scoped";
console.log(blockVar); // Accessible inside the block
}
console.log(blockVar); // Error: blockVar is not defined
4. Lexical (or Static) Scope
JavaScript uses lexical scoping (also known as static scoping), meaning that the scope of a variable is determined by its location in the source code. Inner functions have access to variables declared in their outer functions.
Example:
javascriptCopy codefunction outerFunction() {
let outerVar = "Outer";
function innerFunction() {
console.log(outerVar); // Accessible because of lexical scope
}
innerFunction();
}
outerFunction(); // Output: Outer
5. var
and Hoisting
var
has function scope, but it also has a behavior called hoisting, which means thatvar
declarations are "hoisted" to the top of their scope.This means you can use a
var
variable even before it is declared, although its value will beundefined
until the actual declaration line.
Example:
javascriptCopy codeconsole.log(x); // Output: undefined (due to hoisting)
var x = 5;
console.log(x); // Output: 5
However, let
and const
are not hoisted in the same way; they are block-scoped and will throw an error if accessed before declaration.
Example:
javascriptCopy codeconsole.log(y); // Error: Cannot access 'y' before initialization
let y = 10;
6. Nested Scopes
When a function is nested inside another function, it forms a nested scope. The inner function can access variables in its own scope and also in the scope of the outer function.
Example:
javascriptCopy codefunction outer() {
let outerVar = "I'm outer!";
function inner() {
let innerVar = "I'm inner!";
console.log(outerVar); // Can access outerVar
console.log(innerVar); // Can access innerVar
}
inner();
}
outer();
// Output:
// I'm outer!
// I'm inner!
7. The this
Keyword and Scope
The this
keyword refers to the context from which a function is called. In global scope, this
refers to the global object (window
in browsers). Inside a function, the value of this
depends on how the function is called.
Example:
javascriptCopy codeconsole.log(this); // In browser, `this` refers to the `window` object
function showThis() {
console.log(this); // Value of `this` depends on how the function is called
}
showThis(); // In a regular function call, `this` refers to the global object
Interview questions for scopes
What is scopes?
What is variable scope?
What is variable shadowing?
What is Illegal shadowing?
Declaration for var let and const?
Which variable can be declare without Initialization?
Which variables can reinitialization?
What is hoisting?
What is temporal dead zone?
Subscribe to my newsletter
Read articles from Vitthal Korvan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
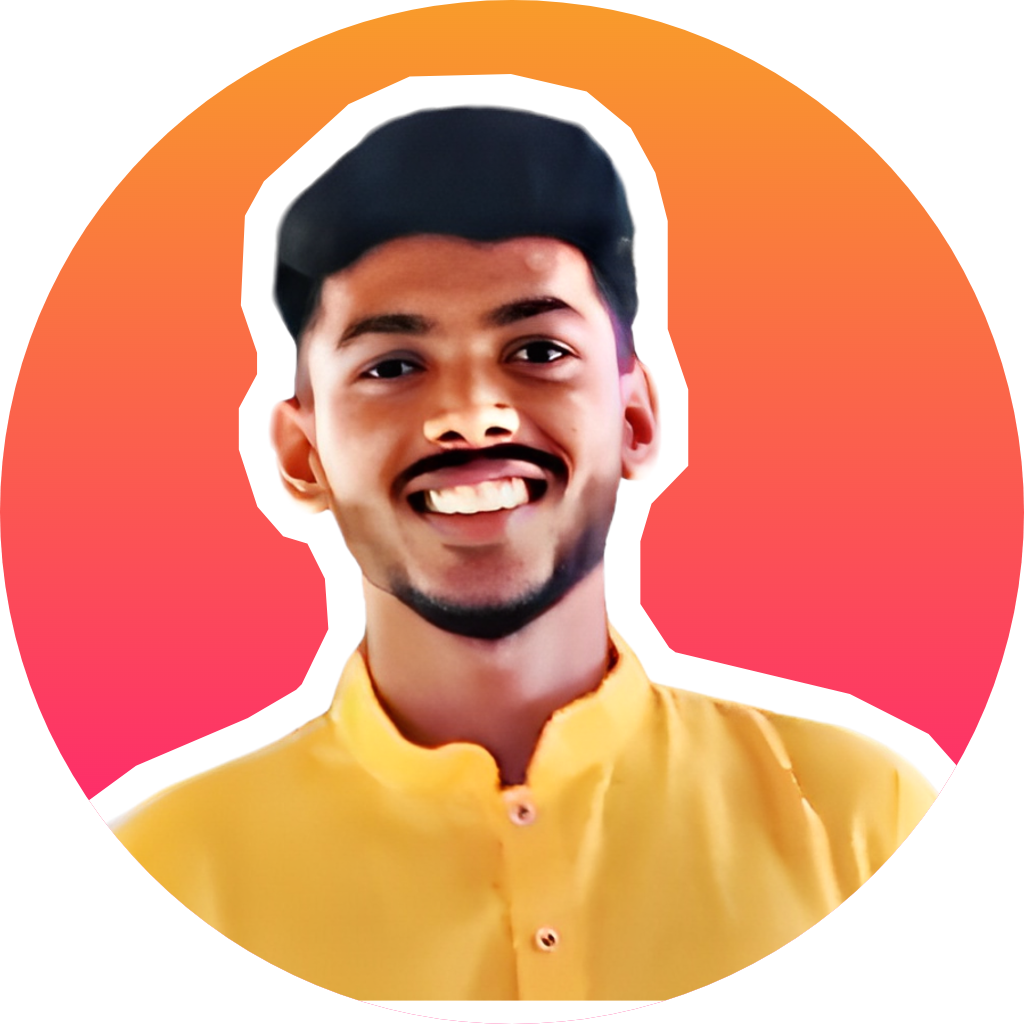
Vitthal Korvan
Vitthal Korvan
๐ Hello, World! I'm Vitthal Korvan ๐ As a passionate front-end web developer, I transform digital landscapes into captivating experiences. you'll find me exploring the intersection of technology and art, sipping on a cup of coffee, or contributing to the open-source community. Life is an adventure, and I bring that spirit to everything I do.