JavaScript ES6 Features: Promises, Async/Await, and Fetch API Explained
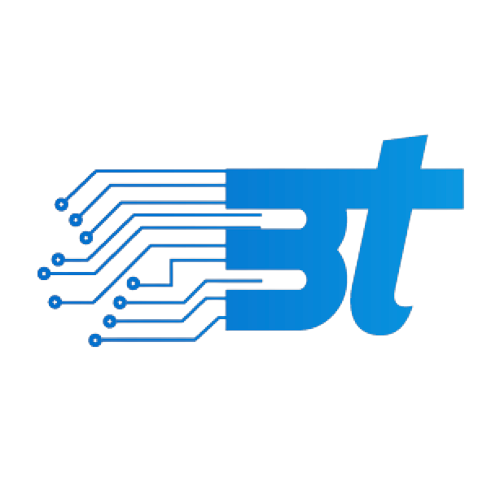
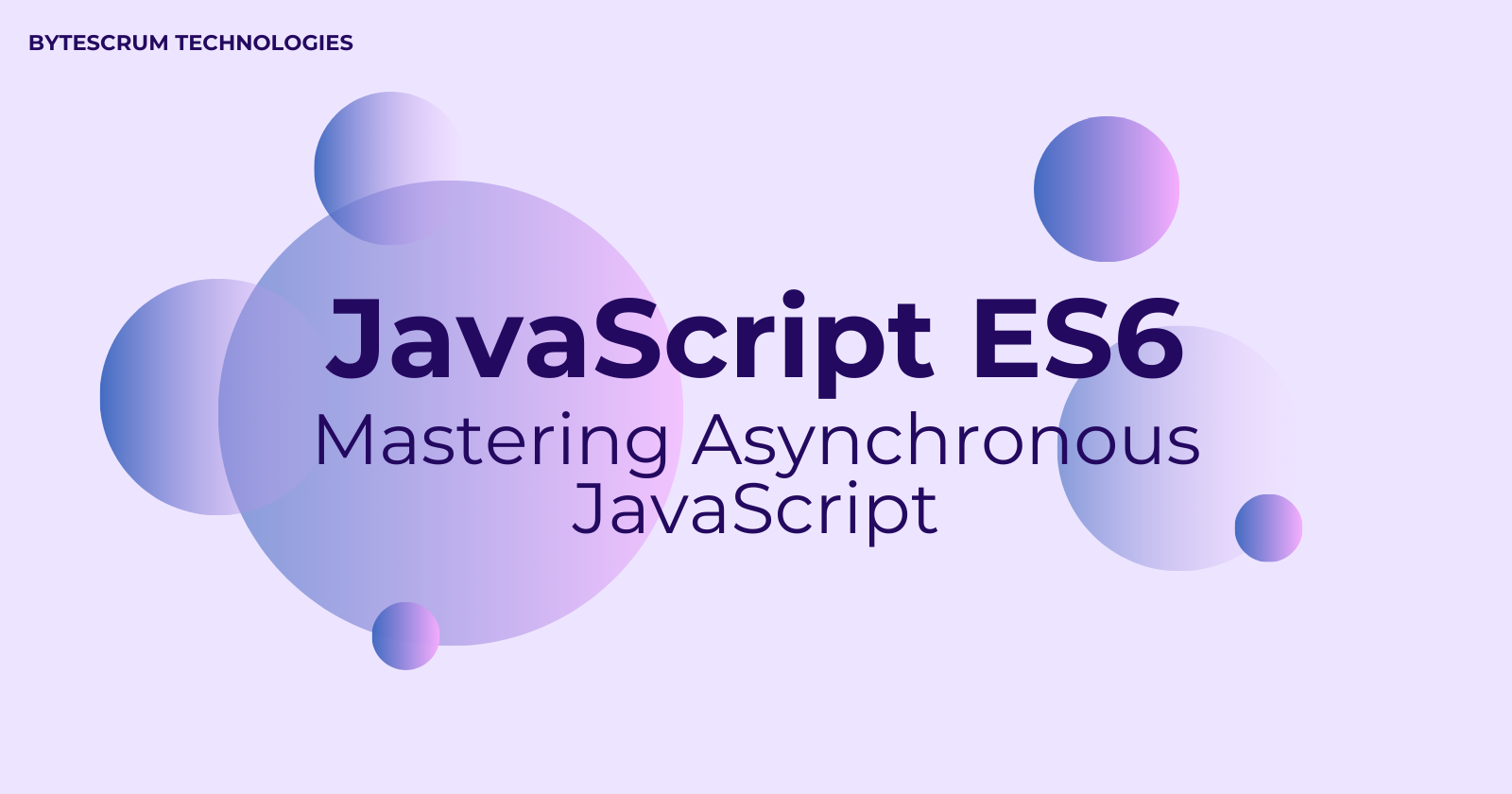
JavaScript is an essential programming language for creating dynamic and responsive websites. With the advent of ES6 (ECMAScript 2015), JavaScript evolved with several new features that make development more manageable and efficient, especially for handling asynchronous operations. This article dives into three of the most significant advancements: Promises, Async/Await, and the Fetch API. These features allow developers to handle asynchronous tasks (like fetching data from a server) more gracefully and improve code readability.
In this comprehensive guide, we will explore these key features and show how to use them effectively with practical, easy-to-understand examples. By the end of this guide, you’ll have a solid understanding of how to work with asynchronous code in modern JavaScript.
1. Understanding Promises in JavaScript
What is a Promise?
In JavaScript, a Promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. As JavaScript is non-blocking, handling operations like network requests, file I/O, and timers can be tricky. Promises provide a better alternative to the traditional callback approach, which often led to messy and difficult-to-read code.
Promises can be in one of three states:
Pending: The initial state of the promise—neither fulfilled nor rejected.
Fulfilled: The operation was completed successfully, and a value is available.
Rejected: The operation failed, and an error occurred.
Why Use Promises?
Promises allow you to write cleaner, more readable code when dealing with asynchronous operations. Instead of nesting callbacks (which leads to “callback hell”), promises offer a structured approach with .then()
and .catch()
for handling success and errors, respectively.
Creating a Promise
To create a Promise, use the Promise
constructor that takes two arguments: resolve
and reject
.
const myPromise = new Promise((resolve, reject) => {
const success = true; // Simulate a condition
if (success) {
resolve("Operation succeeded!");
} else {
reject("Operation failed!");
}
});
Benefits of Async/Await
Readability: Async/await makes asynchronous code easier to read and maintain.
Error Handling: You can use traditional
try...catch
blocks for handling errors, which is more intuitive than using.catch()
with Promises.Sequential Execution: With
await
, you can ensure that the code waits for a promise to resolve before continuing to the next line.
Practical Example: Simulating API Calls with Async/Await
async function fetchPost() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts/1');
const data = await response.json();
console.log(data); // Logs the data from the API
} catch (error) {
console.error('Error fetching post:', error);
}
}
fetchPost();
In this example, the fetchPost
function waits for the API request to complete before processing the data. The await
keyword pauses the execution until the fetch()
Promise resolves.
3. The Fetch API: A Modern Approach to HTTP Requests
The Fetch API is a built-in JavaScript API that allows you to make network requests to retrieve resources, such as data from a server. It’s a more modern and cleaner alternative to XMLHttpRequest
.
Fetching Data with Fetch API
The Fetch API is promise-based, so it fits naturally with Async/Await or .then() chains. Here’s a basic example of fetching data from a REST API:
fetch('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.json(); // Parse the JSON response
})
.then(data => console.log(data)) // Handle the data
.catch(error => console.error('Error fetching data:', error));
Using Fetch with Async/Await
Fetch can also be used with async/await
, which makes the code cleaner and more readable.
async function getPost() {
try {
const response = await fetch('https://jsonplaceholder.typicode.com/posts/1');
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
console.log(data); // Logs the fetched data
} catch (error) {
console.error('Error fetching post:', error);
}
}
getPost();
Advantages of Fetch API
Simplicity: Fetch is simpler and more modern than the older
XMLHttpRequest
.Promise-Based: Fetch returns a Promise, making it easy to integrate with modern JavaScript features like
async/await
.Readable Responses: You can easily parse JSON, text, or other response formats.
Handling Different Response Types
The Fetch API allows you to handle various response types, such as text, JSON, and blobs. Here’s an example of fetching text data:
async function fetchText() {
try {
const response = await fetch('https://example.com/data.txt');
const textData = await response.text(); // Parse response as text
console.log(textData);
} catch (error) {
console.error('Error fetching text data:', error);
}
}
fetchText();
4. Real-World Application: Combining Promises, Async/Await, and Fetch
Let’s combine everything we’ve learned so far into a practical example. Imagine we want to fetch a list of posts from an API and then fetch the details of a specific post. This task involves multiple asynchronous operations that need to be handled sequentially.
Example: Fetching Multiple Posts and Details
async function fetchPostsAndDetails() {
try {
// Fetch a list of posts
const postsResponse = await fetch('https://jsonplaceholder.typicode.com/posts');
const posts = await postsResponse.json();
console.log('Posts:', posts.slice(0, 5)); // Display the first 5 posts
// Fetch the details of the first post
const postDetailsResponse = await fetch(`https://jsonplaceholder.typicode.com/posts/${posts[0].id}`);
const postDetails = await postDetailsResponse.json();
console.log('First Post Details:', postDetails);
} catch (error) {
console.error('Error fetching posts or details:', error);
}
}
fetchPostsAndDetails();
This example fetches a list of posts and, once it’s done, fetches the details of the first post. Both operations are asynchronous, but thanks to async/await
, the code looks clean and easy to follow.
Conclusion
Promises: Provide a cleaner and more structured approach to asynchronous operations compared to callbacks.
Async/Await: Make asynchronous code easier to read, more maintainable, and allow the use of traditional error-handling techniques like
try...catch
.Fetch API: Offers a modern, promise-based approach to making network requests, simplifying the way we interact with HTTP resources.
By mastering these ES6 features, you’ll be able to write more readable, maintainable, and efficient JavaScript code, making you a more effective web developer.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
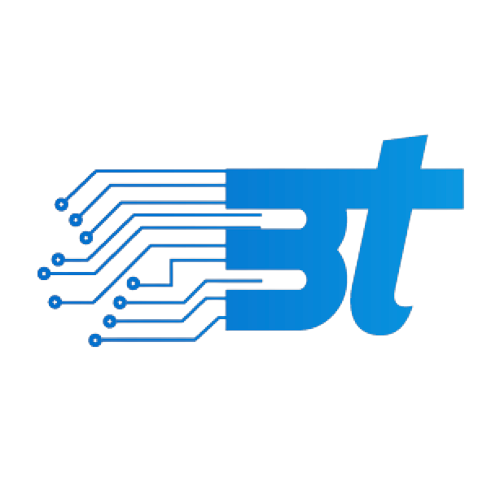
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.