Mastering Swift JSON Decoding
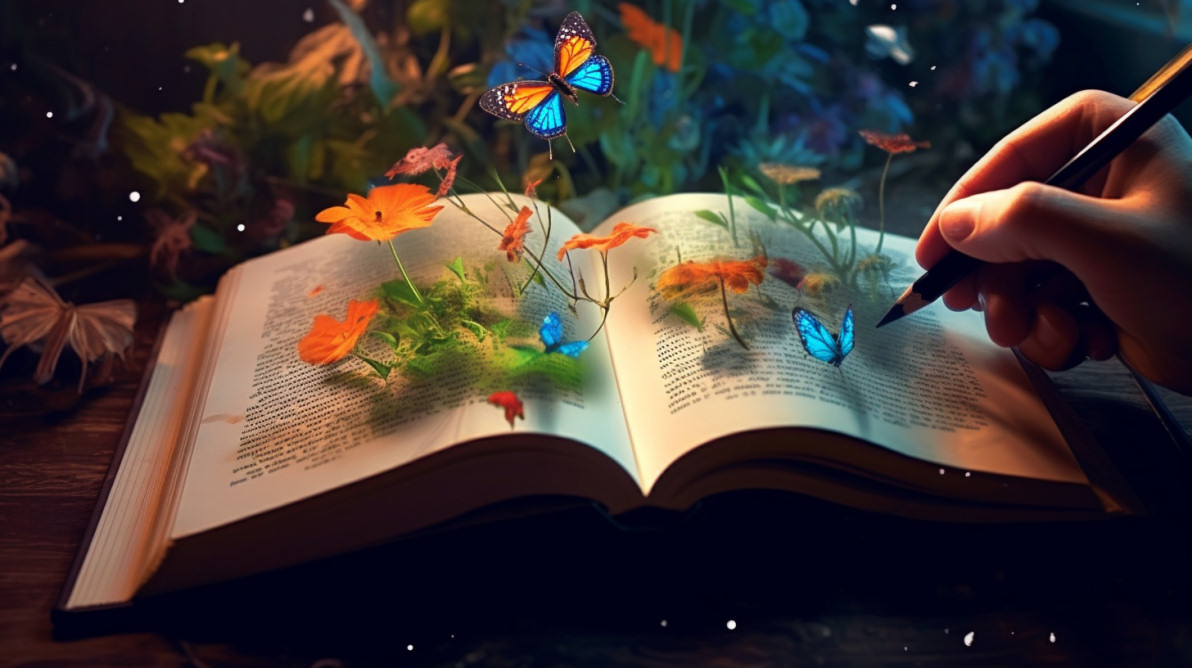
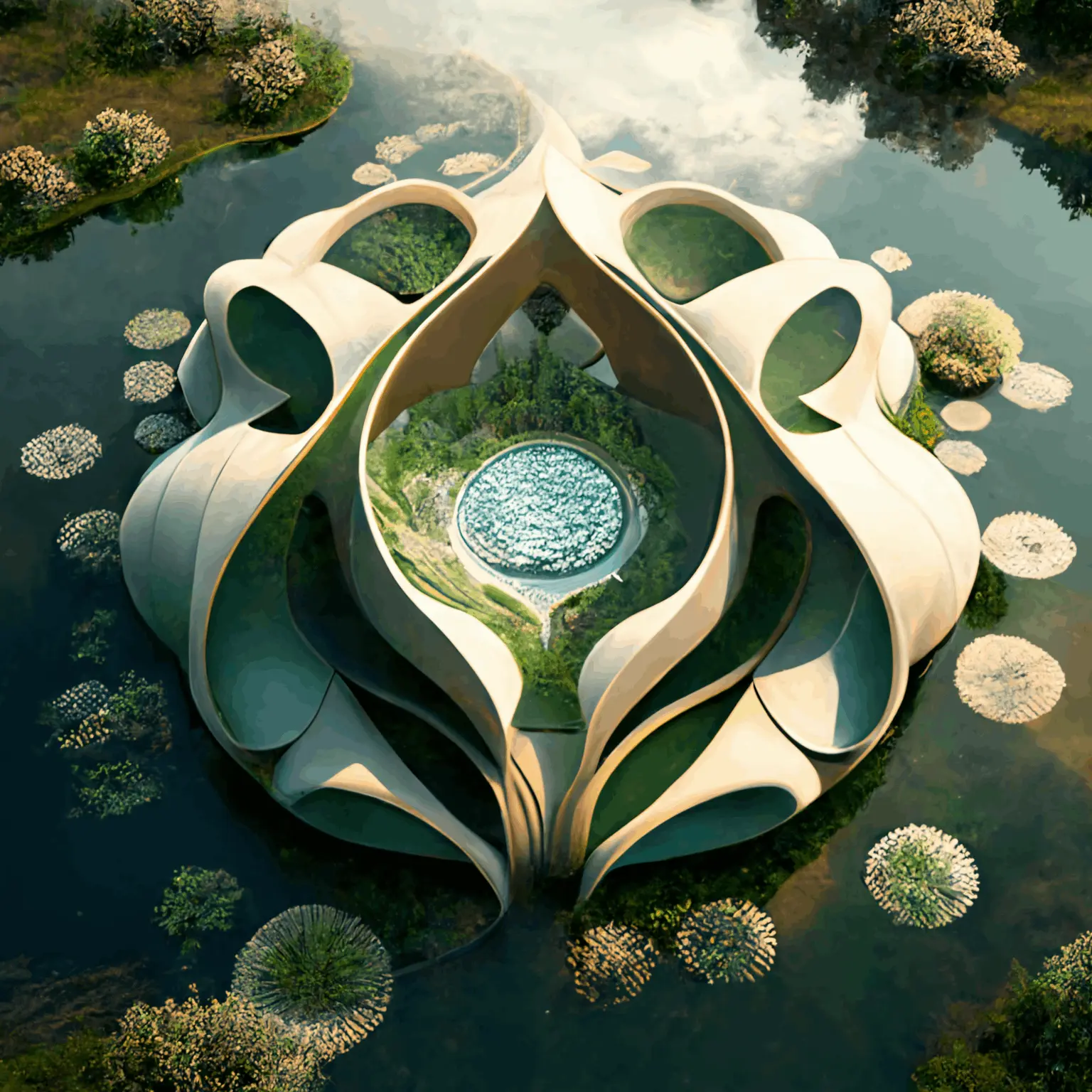
In the world of iOS development, working with JSON data is a common task. While Swift's Codable
protocol has simplified this process, handling potential decoding errors gracefully remains crucial for creating robust applications. This article will guide you through implementing a comprehensive error handling system for JSON decoding in Swift, ensuring your app can gracefully manage various decoding scenarios.
The Challenge
When decoding JSON, several issues can arise:
Missing keys
Type mismatches
Missing values
Corrupted data
General decoding errors
Our goal is to create a system that can:
Identify and categorize these errors
Provide localized error messages
Log errors for debugging
Handle successful decodes seamlessly
The Solution
We'll build a solution using several components:
A custom
DecodeError
enumA simple logging system
A generic decoding function
A sample model to test our implementation
Let's break down each component:
1. Custom DecodeError Enum
enum DecodeError: Error {
case fileNotFound(String)
case keyNotFound(String)
case typeMismatch
case valueNotFound
case dataCorrupted
case dataNotFound
case decodingError(Error)
var status: String {
switch self {
case .fileNotFound(let message):
return message
case .keyNotFound(let key):
return String(format: NSLocalizedString("keyNotFound", comment: ""), key)
case .typeMismatch:
return String(localized: "typeMismatch")
case .valueNotFound:
return String(localized: "valueNotFound")
case .dataCorrupted:
return String(localized: "dataCorrupted")
case .dataNotFound:
return String(localized: "dataNotFound")
case .decodingError(let error):
return String(format: NSLocalizedString("decodingError", comment: ""), error.localizedDescription)
}
}
}
This enum covers various error scenarios and provides localized error messages. The status
computed property returns user-friendly, localized error descriptions.
2. Simple Logger
struct Logger {
enum LogType {
case error, info, debug
}
static func log(type: LogType, _ message: String) {
print("[\(type)] - \(message)")
}
}
This basic logger allows us to log errors, which is crucial for debugging and monitoring.
3. Sample Decodable Model
struct User: Decodable {
let id: Int
let name: String
let email: String
let isAdmin: Bool?
enum CodingKeys: String, CodingKey {
case id
case name
case email
case isAdmin = "is_admin"
}
}
This User
struct demonstrates a typical model you might decode from JSON, including an optional property and a custom coding key.
4. Generic Decoding Function
func decode<T: Decodable>(_ data: Data) -> Result<T, DecodeError> {
do {
let decoder = JSONDecoder()
let result = try decoder.decode(T.self, from: data)
return .success(result)
} catch let DecodingError.keyNotFound(key, context) {
Logger.log(type: .error, "Key '\(key.stringValue)' not found: \(context.debugDescription)")
return .failure(DecodeError.keyNotFound(key.stringValue))
} catch let DecodingError.typeMismatch(type, context) {
Logger.log(type: .error, "Type '\(type)' mismatch: \(context.debugDescription) \(context.codingPath.debugDescription)")
return .failure(DecodeError.typeMismatch)
} catch let DecodingError.valueNotFound(value, context) {
Logger.log(type: .error, "Value '\(value)' not found: \(context.codingPath.description)")
return .failure(DecodeError.valueNotFound)
} catch let DecodingError.dataCorrupted(context) {
Logger.log(type: .error, "Data corrupted: \(context.debugDescription) \(context.codingPath.debugDescription)")
return .failure(DecodeError.dataCorrupted)
} catch {
Logger.log(type: .error, "Decoding error: \(error.localizedDescription)")
return .failure(DecodeError.decodingError(error))
}
}
This function is the heart of our solution. It attempts to decode the provided data and returns a Result
type, which will either contain the decoded object or a DecodeError
.
Putting It All Together
Here's how you can use this system:
let jsonString = """
{
"id": 1,
"name": "John Doe",
"email": "john.doe@example.com",
"is_admin": true
}
"""
guard let jsonData = jsonString.data(using: .utf8) else {
fatalError("Invalid JSON format.")
}
let result: Result<User, DecodeError> = decode(jsonData)
switch result {
case .success(let user):
Logger.log(type: .info, "User decoded successfully: \(user)")
case .failure(let error):
Logger.log(type: .error, "Failed to decode User: \(error.status)")
}
This example demonstrates how to use the decode
function with our User
model. It handles both successful and failed decoding scenarios.
Benefits of This Approach
Type Safety: The use of generics ensures type safety at compile-time.
Comprehensive Error Handling: All potential JSON decoding errors are caught and categorized.
Localization Ready: Error messages are set up for easy localization.
Debugging Support: The logging system aids in identifying and fixing issues.
Flexibility: The
Result
type allows for easy handling of both success and failure cases.
Conclusion
Implementing robust error handling for JSON decoding is crucial for creating reliable iOS applications. This approach provides a solid foundation that you can build upon and customize for your specific needs. By categorizing errors, providing meaningful messages, and utilizing Swift's powerful type system, you can ensure that your app gracefully handles any JSON decoding scenario it encounters.
Remember, the key to mastering JSON decoding in Swift is not just about successfully parsing data, but also about handling failures intelligently. With this system in place, you're well-equipped to tackle even the most complex JSON structures with confidence.
Happy coding!
Subscribe to my newsletter
Read articles from Nurul Islam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
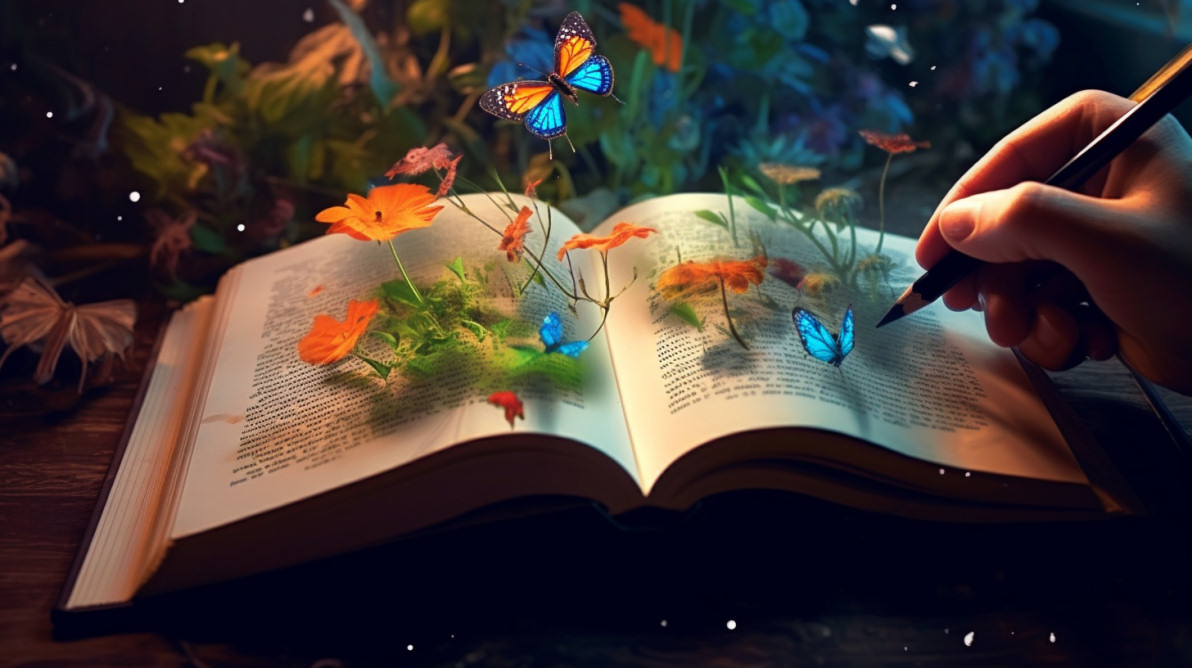
Nurul Islam
Nurul Islam
I'm an experienced mobile application developer with over 10 years of expertise in iOS development using Swift, Objective-C, and Flutter. Throughout my career, I've had the opportunity to work for multiple renowned companies where I developed and architected robust mobile applications used by millions of users worldwide. In addition to my extensive iOS experience, I also have experience working with ASP.Net Core, Entity Framework, and LINQ for backend development as well as Machine Learning and Deep Learning using Python and TensorFlow which has allowed me to collaborate closely with different teams to develop different types of products, apps or services.