Essential String Methods in Python – Part 2 🔑

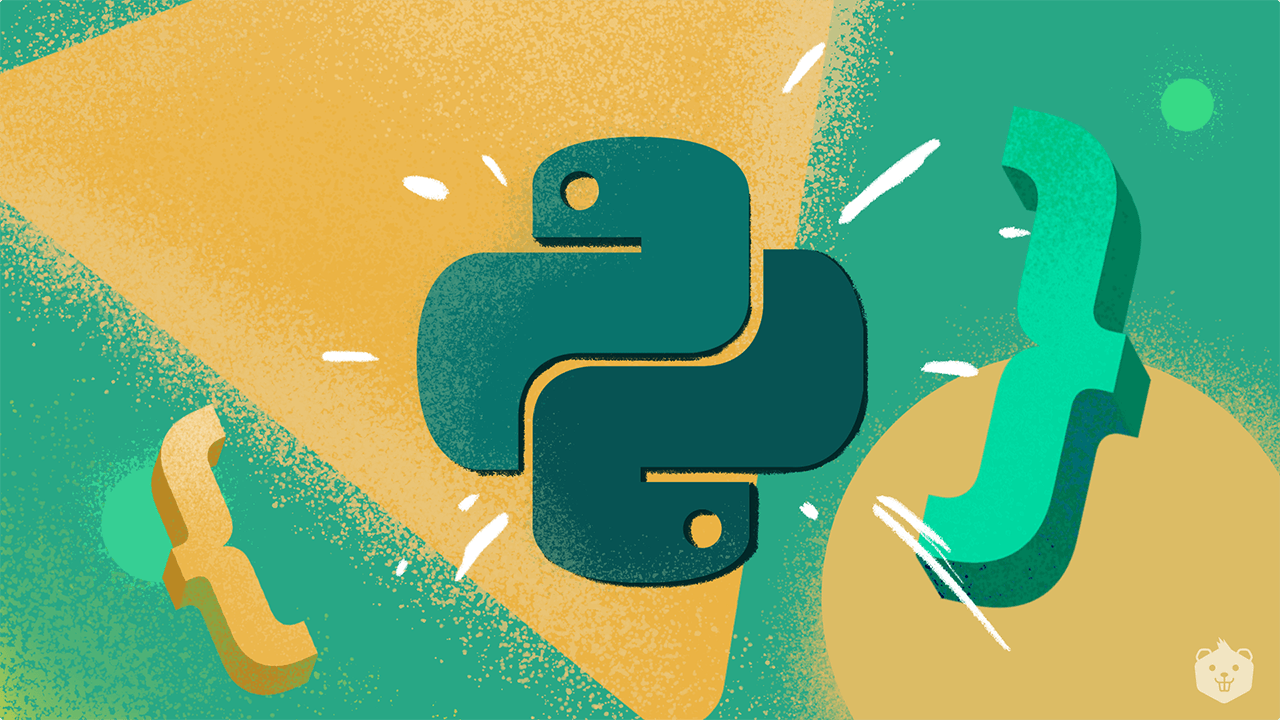
Introduction
In the previous blog, we explored a few commonly used string methods. In this blog, we’ll continue our journey through Python’s string methods, focusing on additional techniques that help you clean, check, and format strings effectively.
1. strip()
, lstrip()
, and rstrip()
: Removing Unwanted Whitespace 🚮
These methods are used to remove leading and/or trailing whitespace (or other specified characters) from a string.
strip()
: Removes whitespace from both ends.lstrip()
: Removes whitespace from the beginning (left side).rstrip()
: Removes whitespace from the end (right side).
pythonCopy codetext = " Hello World "
print(text.strip()) # Output: Hello World
print(text.lstrip()) # Output: Hello World (leading spaces removed)
print(text.rstrip()) # Output: Hello World (trailing spaces removed)
2. isdigit()
, isalpha()
, isalnum()
: Checking String Content ✔️
These methods help in validating the content of strings:
isdigit()
: ReturnsTrue
if all characters are digits.isalpha()
: ReturnsTrue
if all characters are alphabets.isalnum()
: ReturnsTrue
if all characters are alphanumeric (letters or digits).
pythonCopy codetext1 = "12345"
text2 = "Hello"
text3 = "Python3"
print(text1.isdigit()) # Output: True
print(text2.isalpha()) # Output: True
print(text3.isalnum()) # Output: True
3. zfill()
: Add Leading Zeros 🅾️
The zfill()
method pads the string with leading zeros until it reaches the specified width.
pythonCopy codenum_str = "42"
padded_str = num_str.zfill(5)
print(padded_str) # Output: 00042
4. center()
, ljust()
, rjust()
: Aligning Text 🧭
These methods align a string to the center, left, or right and pad the rest of the width with spaces or specified characters.
center()
: Aligns the string in the center.ljust()
: Aligns the string to the left.rjust()
: Aligns the string to the right.
pythonCopy codetext = "Python"
print(text.center(10, '-')) # Output: --Python--
print(text.ljust(10, '-')) # Output: Python----
print(text.rjust(10, '-')) # Output: ----Python
5. partition()
and rpartition()
: Dividing Strings Based on a Substring 🛠️
The partition()
method splits the string into three parts: the substring before the separator, the separator itself, and the substring after the separator.
pythonCopy codetext = "I love Python"
print(text.partition("love"))
# Output: ('I ', 'love', ' Python')
Similarly, rpartition()
works from the right.
6. casefold()
: Aggressive Lowercasing 🔡
The casefold()
method is similar to lower()
, but it is more aggressive in making the string case-insensitive. It’s especially useful for comparing strings.
pythonCopy codetext1 = "Python"
text2 = "python"
print(text1.casefold() == text2.casefold()) # Output: True
7. expandtabs()
: Expanding Tabs to Spaces ⌨️
If you are working with tab-delimited text, expandtabs()
allows you to replace the tab characters (\t
) with a specified number of spaces.
pythonCopy codetext = "Python\tis\tfun"
print(text.expandtabs(4))
# Output: Python is fun (replaces \t with 4 spaces)
8. format()
and format_map()
: String Formatting 📝
While Python's f-strings
are commonly used for string interpolation, the format()
and format_map()
methods are powerful alternatives that provide dynamic formatting options.
pythonCopy code# format example
text = "My name is {name} and I am {age} years old."
print(text.format(name="John", age=30))
# Output: My name is John and I am 30 years old.
With these additional string methods, you now have a comprehensive toolkit for handling a wide variety of string manipulation tasks. These methods enable you to format, check, and process text with ease, adding efficiency to your Python programming.
This concludes our exploration of Python's essential string methods. By combining these techniques with the slicing and basic string operations discussed earlier, you will be well-equipped to handle any text processing challenge that comes your way!
Subscribe to my newsletter
Read articles from Shrey Dikshant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shrey Dikshant
Shrey Dikshant
Aspiring data scientist with a strong foundation in adaptive quality techniques. Gained valuable experience through internships at YT Views, focusing on operation handling. Proficient in Python and passionate about data visualization, aiming to turn complex data into actionable insights.