Automating Scheduled Payments with Paystack API in PHP
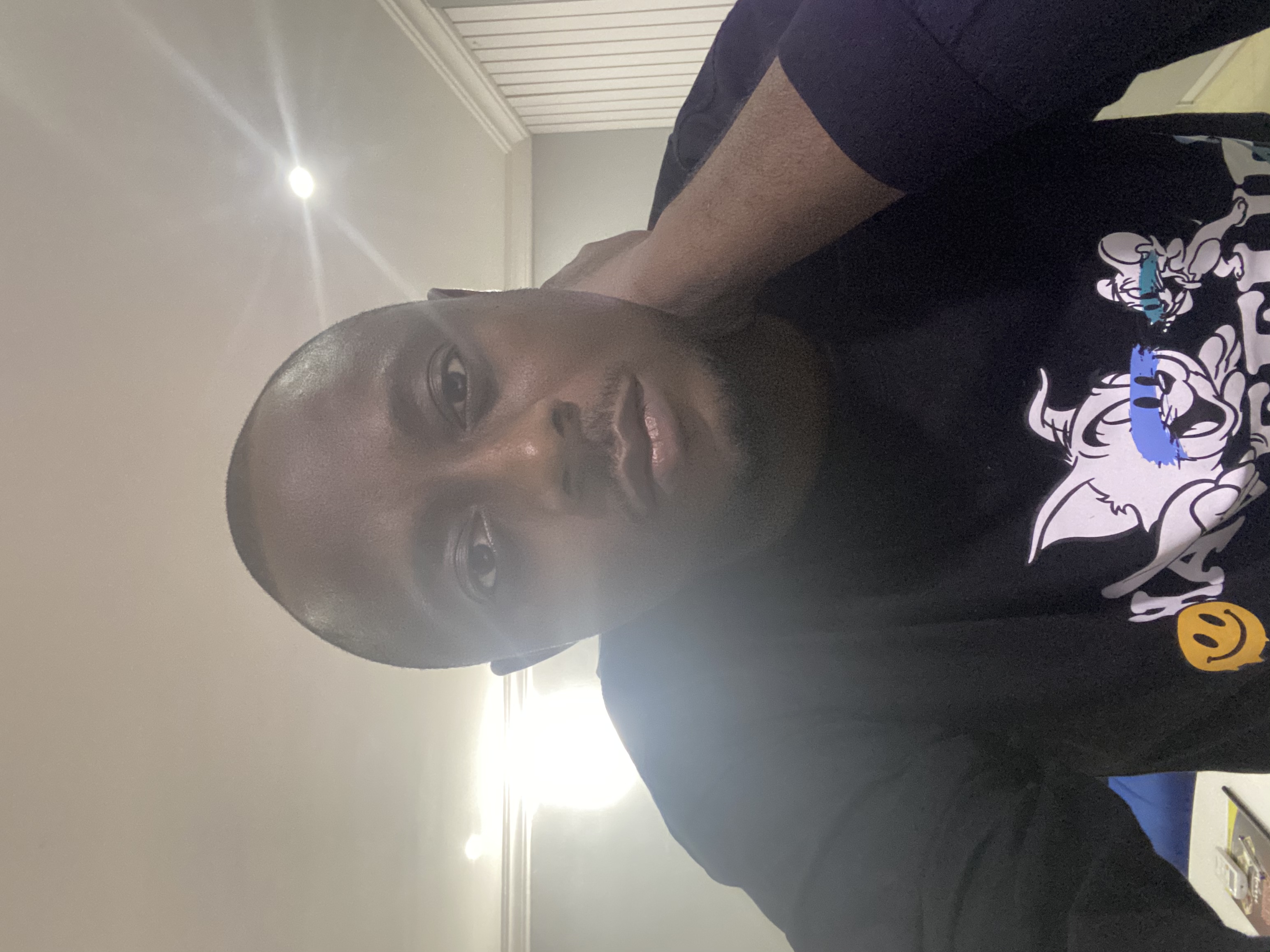
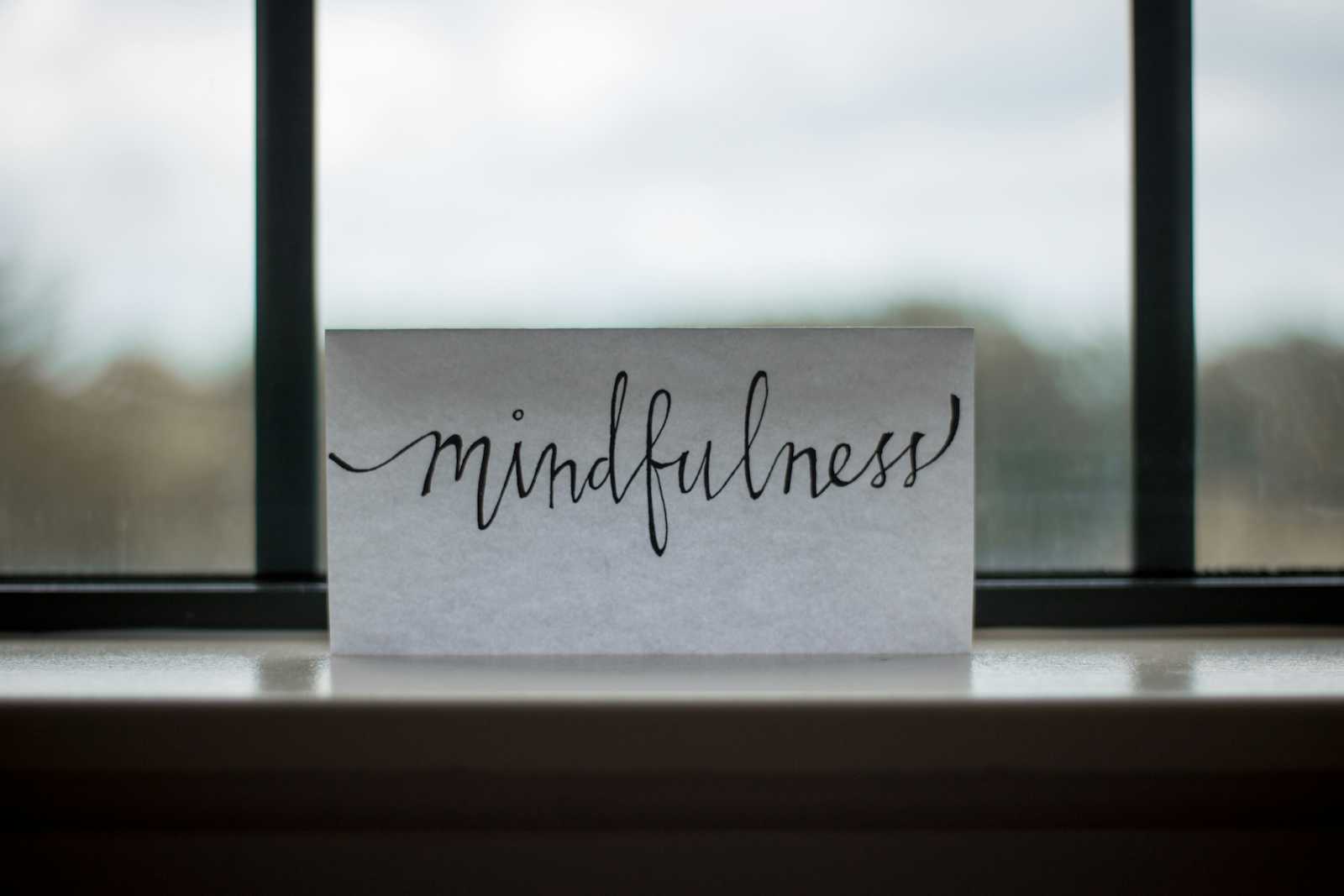
Managing scheduled payments manually can be tedious and prone to errors, especially when dealing with multiple recipients. Automating this process with the Paystack API simplifies the workflow, ensuring that payments are processed quickly and securely. In this guide, we will walk through how to create a PHP script that interacts with the Paystack API to automate scheduled payments.
We'll cover everything from retrieving payment details from a database to creating Paystack transfer recipients and finally processing payments. Additionally, we’ll update our database to reflect the status of each transaction.
Prerequisites
Paystack Account: You will need a Paystack account with access to their API keys. Sign up at
Paystack.
PHP: Make sure PHP is installed on your server.
MySQL: A MySQL database will be used to store the payout details.
cURL: Your server must support cURL, as it is required to send HTTP requests to the Paystack API.
Paystack API Endpoints
Transfer Recipient Endpoint: This is used to create a recipient profile in Paystack.
Transfer Endpoint: This is used to initiate a transfer to a previously created recipient.
Step 1: Database Structure
We will use a MySQL table paystackpayout
to store the payment details, including the status of the transaction. The basic structure of the table is as follows:
CREATE TABLE `paystackpayout` (
`id` INT(11) NOT NULL AUTO_INCREMENT PRIMARY KEY,
`email` VARCHAR(100) NOT NULL,
`fullname` VARCHAR(255) NOT NULL,
`reference` VARCHAR(50) NOT NULL UNIQUE,
`amount` DECIMAL(10,2) NOT NULL,
`bankCode` VARCHAR(10) NOT NULL,
`accountNumber` VARCHAR(10) NOT NULL,
`status8` TINYINT(1) DEFAULT 0,
`status` VARCHAR(50) DEFAULT 'Pending'
);
status8
: This column will be set to0
for unpaid transactions and updated to1
once a payment is successful.reference
: Each transaction will have a unique reference to ensure no duplicate payments.
Step 2: PHP Script to Automate Payments
Including Configuration File
In your script, you need to include the configuration file where the database connection ($link
) is established:
include("config.php");
Fetching Pending Payments
We'll select records where status8 = 0
, indicating that the payment is yet to be made.
$sql = "SELECT email, fullname, reference, amount, bankCode, accountNumber FROM paystackpayout WHERE status8 = 0";
$result = mysqli_query($link, $sql);
Creating Paystack Transfer Recipients
For each recipient, we need to create a transfer recipient using Paystack’s API. This requires the recipient’s name, account number, and bank code.
$url = "https://api.paystack.co/transferrecipient";
$authorization = "Authorization: Bearer your_secret_key"; // Replace with your secret key
$data = json_encode([
"type" => "nuban",
"name" => $fullname,
"account_number" => $accountNumber,
"bank_code" => $bankCode,
"currency" => "NGN"
]);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
$authorization
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
$response = curl_exec($ch);
$responseData = json_decode($response, true);
curl_close($ch);
if ($responseData['status']) {
$recipient_code = $responseData['data']['recipient_code'];
} else {
echo "Error creating transfer recipient: " . $responseData['message'];
continue;
}
If successful, the recipient’s code will be used in the next step to initiate a transfer.
Initiating the Transfer
After successfully creating a recipient, we can now make the transfer using Paystack’s transfer
API.
$url = "https://api.paystack.co/transfer";
$data = json_encode([
"source" => "balance",
"reason" => "Withdrawal",
"amount" => intval($amount * 100), // Convert the amount to kobo
"recipient" => $recipient_code
]);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
$authorization
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
$response = curl_exec($ch);
$responseData = json_decode($response, true);
curl_close($ch);
if ($responseData['status']) {
$transfer_code = $responseData['data']['transfer_code'];
echo "Transfer successful for reference $reference. Transfer Code: $transfer_code<br>";
} else {
echo "Error initiating transfer: " . $responseData['message'];
continue;
}
Updating the Payment Status
If the transfer is successful, we update the status8
field to 1
and the status
to 'Success'
in the database.
$updateSql = "UPDATE paystackpayout SET status='Success', status8=1 WHERE reference='$reference'";
mysqli_query($link, $updateSql);
Complete Script
Here’s the complete script that ties everything together:
<?php
include("config.php");
// Fetch records from paystackpayout where status8=0
$sql = "SELECT email, fullname, reference, amount, bankCode, accountNumber FROM paystackpayout WHERE status8 = 0";
$result = mysqli_query($link, $sql);
if (mysqli_num_rows($result) > 0) {
while ($row = mysqli_fetch_assoc($result)) {
$email = $row['email'];
$fullname = $row['fullname'];
$reference = $row['reference'];
$amount = $row['amount'];
$bankCode = $row['bankCode'];
$accountNumber = $row['accountNumber'];
// Create Transfer Recipient
$url = "https://api.paystack.co/transferrecipient";
$authorization = "Authorization: Bearer your_secret_key";
$data = json_encode([
"type" => "nuban",
"name" => $fullname,
"account_number" => $accountNumber,
"bank_code" => $bankCode,
"currency" => "NGN"
]);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
$authorization
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
$response = curl_exec($ch);
$responseData = json_decode($response, true);
curl_close($ch);
if ($responseData['status']) {
$recipient_code = $responseData['data']['recipient_code'];
// Initiate Transfer
$url = "https://api.paystack.co/transfer";
$data = json_encode([
"source" => "balance",
"reason" => "Withdrawal",
"amount" => intval($amount * 100),
"recipient" => $recipient_code
]);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
"Content-Type: application/json",
$authorization
]);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
$response = curl_exec($ch);
$responseData = json_decode($response, true);
curl_close($ch);
if ($responseData['status']) {
$transfer_code = $responseData['data']['transfer_code'];
echo "Transfer successful for reference $reference. Transfer Code: $transfer_code<br>";
// Update status8 to 1 in paystackpayout
$updateSql = "UPDATE paystackpayout SET status='Success', status8=1 WHERE reference='$reference'";
mysqli_query($link, $updateSql);
} else {
echo "Error initiating transfer for reference $reference: " . $responseData['message'];
}
} else {
echo "Error creating transfer recipient for reference $reference: " . $responseData['message'];
}
}
} else {
echo "No pending payments found.";
}
?>
Step 3: Testing
Ensure that you have filled in your Paystack secret key where appropriate.
Populate the
paystackpayout
table with test data.Run the script and monitor the output for successful transfers.
Check the Paystack dashboard to verify the transfers.
Conclusion
By automating your payment process with Paystack's API, you can eliminate the need for manual intervention, reduce errors, and streamline your payment workflow. This script fetches payment details, creates transfer recipients, initiates transfers, and updates your database accordingly, ensuring a smooth
and automated payment process.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
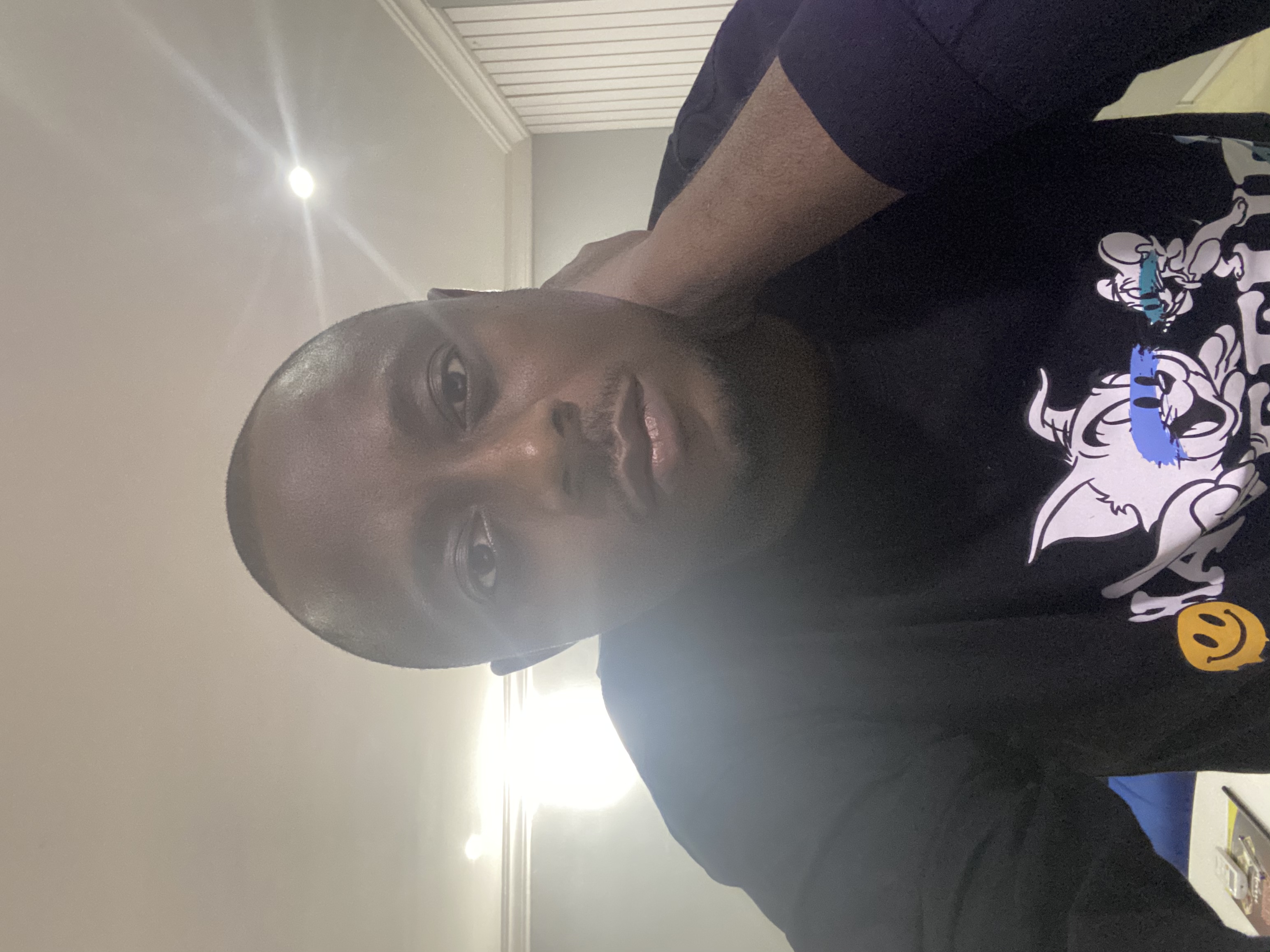
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.