API Gateway
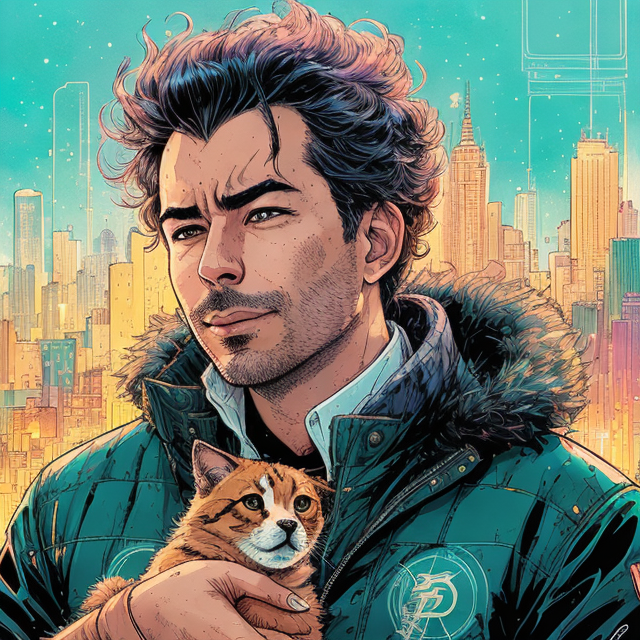
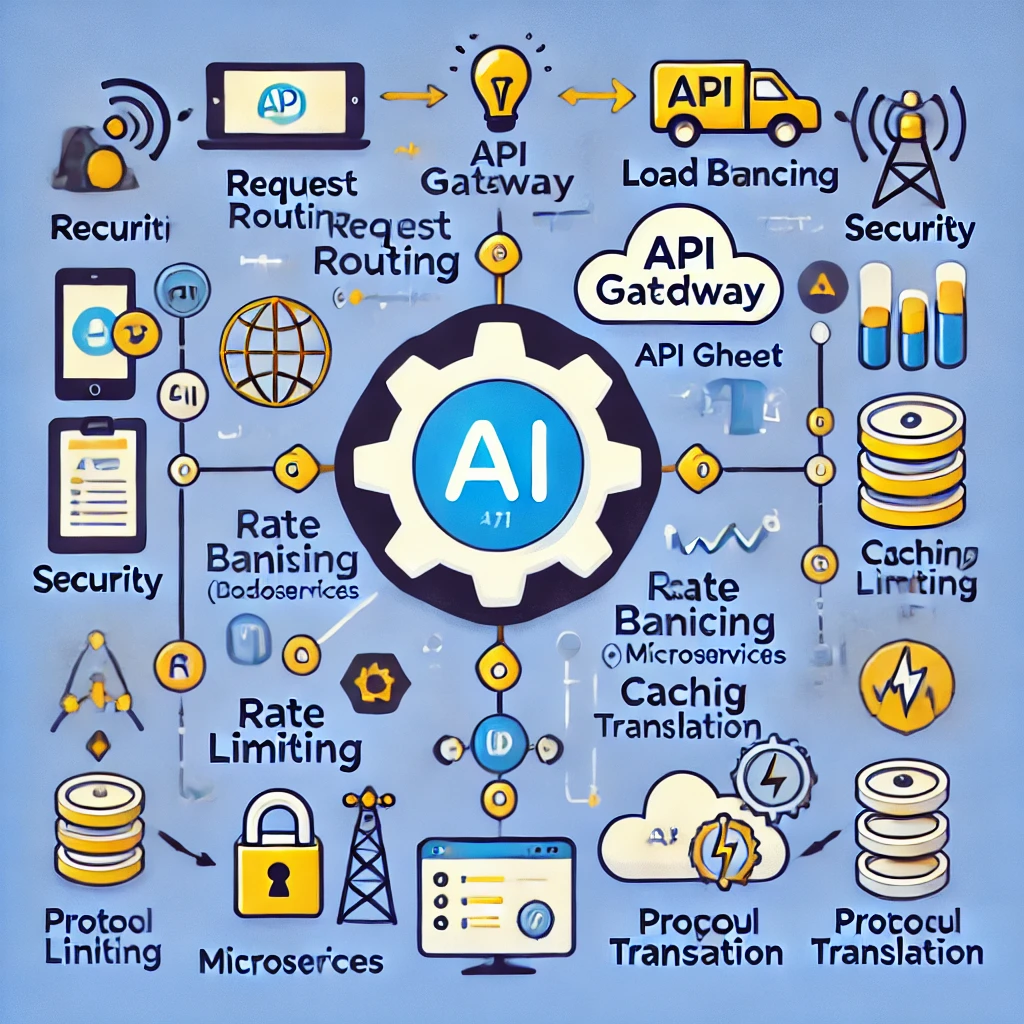
What is an API Gateway ?
An API Gateway acts as a single entry point or “gateway” through which external applications can access multiple backend services in an API-driven architecture. It sits between the client and a collection of backend services, handling all the requests from clients, routing them to the appropriate service, and then returning the responses.
An API Gateway simplifies client interactions by aggregating and routing requests to the appropriate backend services. It can also perform functions such as request transformation, load balancing, security enforcement, and analytics. It's often used in microservices architectures where many services work together to provide the desired functionality.
Benefits of an API Gateway
Simplified Client Communication: Clients only interact with the API Gateway, which hides the complexity of multiple microservices from the clients.
Centralized Security: API gateways centralize authentication, authorization, and other security measures like rate limiting and encryption, making it easier to secure your microservices.
Load Balancing: The gateway can distribute requests across multiple services or instances of a service, improving performance and fault tolerance.
Caching: By providing caching at the gateway level, response times can be reduced, and the load on backend services is minimized.
Rate Limiting and Throttling: API Gateways can prevent overuse of the API by implementing rate-limiting mechanisms, which prevent the backend from being overwhelmed by traffic.
Protocol Translation: It allows you to convert different protocols such as HTTP, WebSockets, and gRPC, enabling backward compatibility or compatibility with multiple devices.
Service Aggregation: The gateway can aggregate the responses from different services into one, which is useful when building composite APIs or handling cross-service transactions.
Monitoring and Analytics: API Gateways can collect valuable metrics on API usage, response times, and error rates, enabling improved monitoring and optimization.
Key Features of an API Gateway
Request Routing: Routes incoming client requests to the appropriate backend service.
Load Balancing: Distributes requests across multiple instances of a service to ensure even distribution of load.
Security: Provides SSL termination, API key validation, token validation (OAuth 2.0, JWT), and rate limiting to secure APIs.
Protocol Handling: Supports different communication protocols like HTTP, HTTPS, WebSockets, gRPC, etc.
Transformation and Mapping: Can modify requests or responses on the fly, changing formats (e.g., JSON to XML) or restructuring data.
Rate Limiting and Quotas: Helps prevent abuse by setting limits on the number of requests a user or application can make over time.
Caching: Stores frequently requested responses to improve response time and reduce the load on backend services.
Analytics and Monitoring: Provides insights into how APIs are used, including tracking metrics such as request counts, latency, error rates, and more.
Platforms Providing API Gateway Solutions
There are several popular API gateway platforms, including both open-source and commercial options:
AWS API Gateway:
Amazon's managed solution that works with other AWS services like Lambda, S3, DynamoDB.
Key features: Built-in scaling, monitoring, integration with AWS IAM for security, and low-code transformation.
Kong:
Open-source API gateway that is extensible and highly customizable.
Key features: Plugin architecture, support for service mesh, and integrations with cloud-native tools like Kubernetes.
NGINX:
A widely used open-source reverse proxy server that also functions as an API gateway.
Key features: Fast performance, scalability, load balancing, SSL/TLS offloading.
Google Cloud API Gateway:
Fully managed service for deploying APIs on Google Cloud.
Key features: Deep integration with Google Cloud services, authentication, and detailed API usage analytics.
Apigee (Google):
A commercial API management solution with full lifecycle API management capabilities.
Key features: API design, security enforcement, monitoring, analytics, and monetization.
Azure API Management:
A fully managed API gateway solution offered by Microsoft Azure.
Key features: API design, security, monitoring, and seamless integration with other Azure services.
Traefik:
A modern, cloud-native reverse proxy and load balancer that can also act as an API Gateway.
Key features: Integrated with Docker and Kubernetes, easy dynamic configuration, and support for HTTP/2, WebSockets, and GRPC.
Tyk:
A commercial, open-source API gateway.
Key features: Rate limiting, throttling, OAuth 2.0/JWT security, load balancing, and monitoring.
how to secure backend with api gateway
Securing a backend with an API Gateway involves implementing several key strategies that provide protection for your backend services, manage access control, and ensure data security. Here’s how you can secure your backend using an API Gateway:
1. Authentication and Authorization
OAuth 2.0 / OpenID Connect: Require clients to authenticate via OAuth 2.0 or OpenID Connect. API Gateway can validate the OAuth tokens or JWTs (JSON Web Tokens) to ensure only authenticated users access the API.
API Key Validation: Assign unique API keys to clients and validate these keys at the gateway level. API keys help in controlling access and identifying the consumers of your API.
Role-Based Access Control (RBAC): Assign roles and permissions based on users or client types, and enforce authorization based on these roles at the API Gateway level.
2. SSL/TLS Termination
Enable HTTPS: API Gateways should terminate SSL/TLS connections, ensuring all traffic between clients and the gateway is encrypted. This protects data in transit from eavesdropping and man-in-the-middle attacks.
Backend Encryption: Optionally, use SSL/TLS between the API Gateway and the backend services, especially if sensitive data is involved.
3. Rate Limiting and Throttling
Protect Against DDoS: Use rate limiting to control how many requests a client can make within a given timeframe. This helps prevent abuse or Distributed Denial of Service (DDoS) attacks.
Throttling: Throttle traffic for different clients based on their service tier (e.g., free users may have lower limits than premium users).
4. IP Whitelisting and Blacklisting
- Restrict Access: Configure the API Gateway to only allow requests from specific trusted IP addresses (whitelisting) and deny access from malicious or unknown sources (blacklisting).
5. Input Validation
Request Validation: Ensure that incoming requests conform to expected formats (e.g., JSON, XML). Validate all inputs against predefined rules (e.g., length, format, data type) to prevent malicious payloads, such as SQL injections or cross-site scripting (XSS) attacks.
Schema Validation: Use schema validation (e.g., OpenAPI, JSON Schema) to ensure requests adhere to the defined structure before reaching backend services.
6. Data Masking and Transformation
Mask Sensitive Data: Use the API Gateway to mask or obfuscate sensitive data (e.g., personally identifiable information or credit card numbers) before sending it to the client.
Data Encryption: Ensure that sensitive data transmitted to the client or between services is encrypted, and apply decryption logic at the gateway level when necessary.
7. Logging and Monitoring
Audit Trails: Enable logging to capture detailed information about API requests, including request origin, timestamps, and authentication status. This helps in auditing and detecting suspicious activity.
Real-time Monitoring: Use monitoring tools to track traffic patterns, detect anomalies, and identify potential security breaches in real time.
8. Cross-Origin Resource Sharing (CORS)
- CORS Policy Enforcement: Set up proper CORS rules to ensure that only trusted domains can access your APIs. This prevents unauthorized domains from accessing the backend.
9. API Versioning
- Avoid Breaking Changes: Use versioning to control access to different API versions, preventing clients from accessing deprecated or less secure versions of your API.
10. Web Application Firewall (WAF) Integration
- WAF Protection: Integrate a WAF with your API Gateway to protect against common attacks such as SQL injection, cross-site scripting (XSS), and other OWASP top 10 threats.
11. Service-to-Service Authentication (Mutual TLS)
- Mutual TLS (mTLS): Use mutual TLS to ensure secure, authenticated communication between the API Gateway and backend services. In this setup, both the client (gateway) and server (backend services) verify each other's certificates.
12. Token Revocation and Expiry
Set Token Expiry: Ensure that tokens, such as OAuth 2.0 tokens or API keys, have a limited lifespan to minimize the impact of compromised credentials.
Token Revocation: Allow clients to revoke tokens when needed (e.g., after logout), preventing them from being used after they're no longer valid.
13. Protect Against Payload Attacks
Payload Size Limits: Set maximum payload sizes at the API Gateway level to prevent resource exhaustion from large or malformed requests.
Sanitize Headers and Query Parameters: Strip or sanitize headers and query parameters to avoid header injections or other forms of attack.
14. Caching
- Response Caching: Cache responses at the gateway level to prevent repetitive load on backend services and minimize exposure to unnecessary API calls, enhancing security by reducing traffic to the backend.
By applying these measures, the API Gateway acts as a central point to enforce security and control, ensuring that backend services remain protected from malicious clients and unauthorized access. This comprehensive security approach helps mitigate risks and enhances the overall resilience of your microservices or API-driven architecture.
Subscribe to my newsletter
Read articles from BEN.R directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
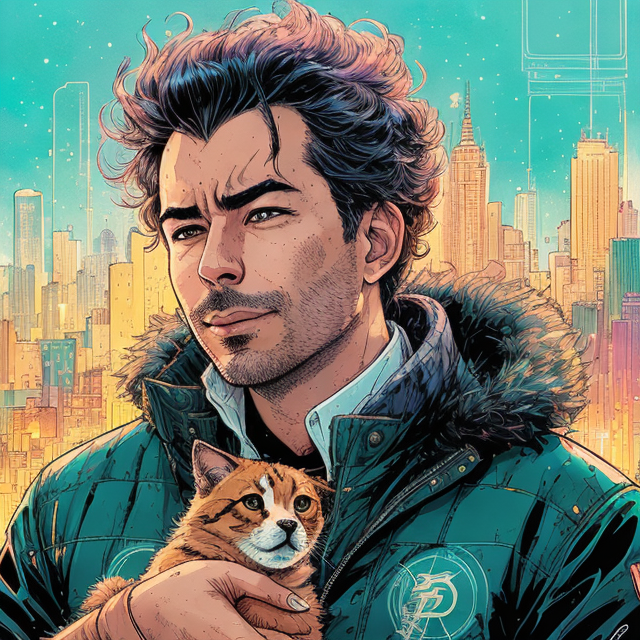
BEN.R
BEN.R
Je suis un solution architecte dans le cloud Azure, avec des multiples expériences dans le monde du consulting. Je parcours l'univers informatique depuis déjà plus de 15 ans, m'amuse à jouer les magiciens du code et les alchimistes du cloud. J'ai été apprenti sorcier en conception des applications, et je fais partie des super-héros de développement backend, web et mobile, toujours prêt à sauver le monde du chaos informatique ! Mon pouvoir spécial réside dans l'art de construire des architectures gigantesques et de rendre les sites invincibles. Imaginez-moi, tel un maître des nuages, déployant des serveurs en un claquement de doigts et écrivant des sorts magiques pour des logiciels d'application éblouissants. N'hésitez pas à me contacter si vous avez besoin d'aide ! Je suis toujours prêt à défendre l'Internet des méchants bugs et des pannes maléfiques ! :)