Day 56 - Creating and Updating Models on Prisma + MySQL in AdonisJs

Table of contents
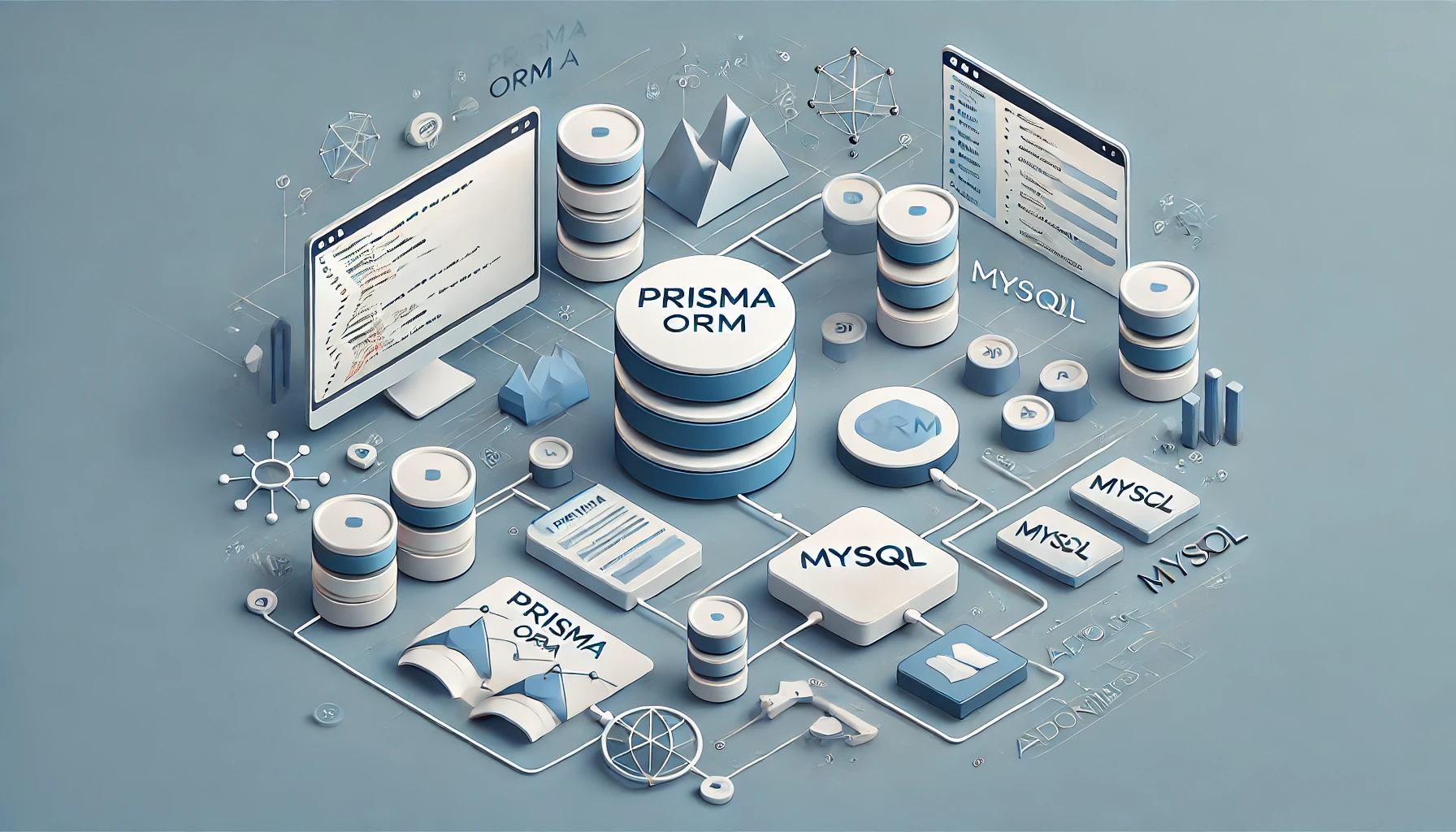
Today marks Day 56 of #100DaysOfCode.
In this article, I’ll walk through how you can create and update database models using Prisma ORM in an AdonisJS application with a MySQL database.
Before proceeding, ensure that your AdonisJS project is set up and connected to your MySQL database using Prisma ORM. If you haven’t done this yet, refer to my previous article for a detailed guide.
Creating a Model in Prisma
To begin, let's build a basic User
model that stores data about the users in your application.
Define the Model: In
prisma/schema.prisma
, define aUser
model as follows:model User { id Int @id @default(autoincrement()) email String @unique name String createdAt DateTime @default(now()) }
id
: A unique integer that automatically increments.email
: A unique string to store the user’s email.name
: A string to store the user’s name.createdAt
: A DateTime field that automatically records when the user was created.
Run Prisma Migrations: After defining your model, build the matching table in your MySQL database by executing the following command:
npx prisma migrate dev --name init
This will create a migration file, apply the changes to your database and also creates/updates the Prisma client with the latest schema, so the new or modified fields and models are available for use in your code.
Updating a Model
Now, let’s add more fields like firstname
, lastname
, password
, and phone
to the User
model:
Update the Prisma Model: In
prisma/schema.prisma
, change theUser
model:model User { id Int @id @default(autoincrement()) email String @unique name String firstname String lastname String password String phone String? // Optional field createdAt DateTime @default(now()) }
firstname: The user's first name.
lastname: The user's last name.
password: A string to store the password.
phone: An optional string for the user’s phone number (indicated by the
?
).
Generate a New Migration: After editing the model, create a new migration to update the database schema:
npx prisma migrate dev --name <migration name>
Replace
<migration-name>
with a descriptive name (e.gadd_user_fields
) for the migration.This will apply the changes to your MySQL database, add the new fields and also updates the Prisma client with the latest schema, so the new fields are available for use in your code.
By following these steps, you will be able to create and update database models using Prisma ORM in an AdonisJS application with a MySQL database.
By following these steps, you should now be able to create and update database models using Prisma ORM within an AdonisJS application backed by a MySQL database.
In the next article, I’ll guide you through creating an Entity Relationship Diagram (ERD) using draw.io, and demonstrate how to establish relationships between models in AdonisJS.
Watch out!. Thanks 🙏🏿
Subscribe to my newsletter
Read articles from Allahisrabb directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Allahisrabb
Allahisrabb
Software Engineer | Freelancer