Mastering JavaScript's Rest and Spread Operators for Cleaner Code
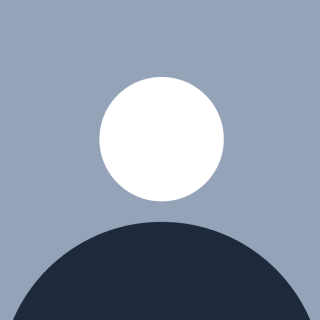
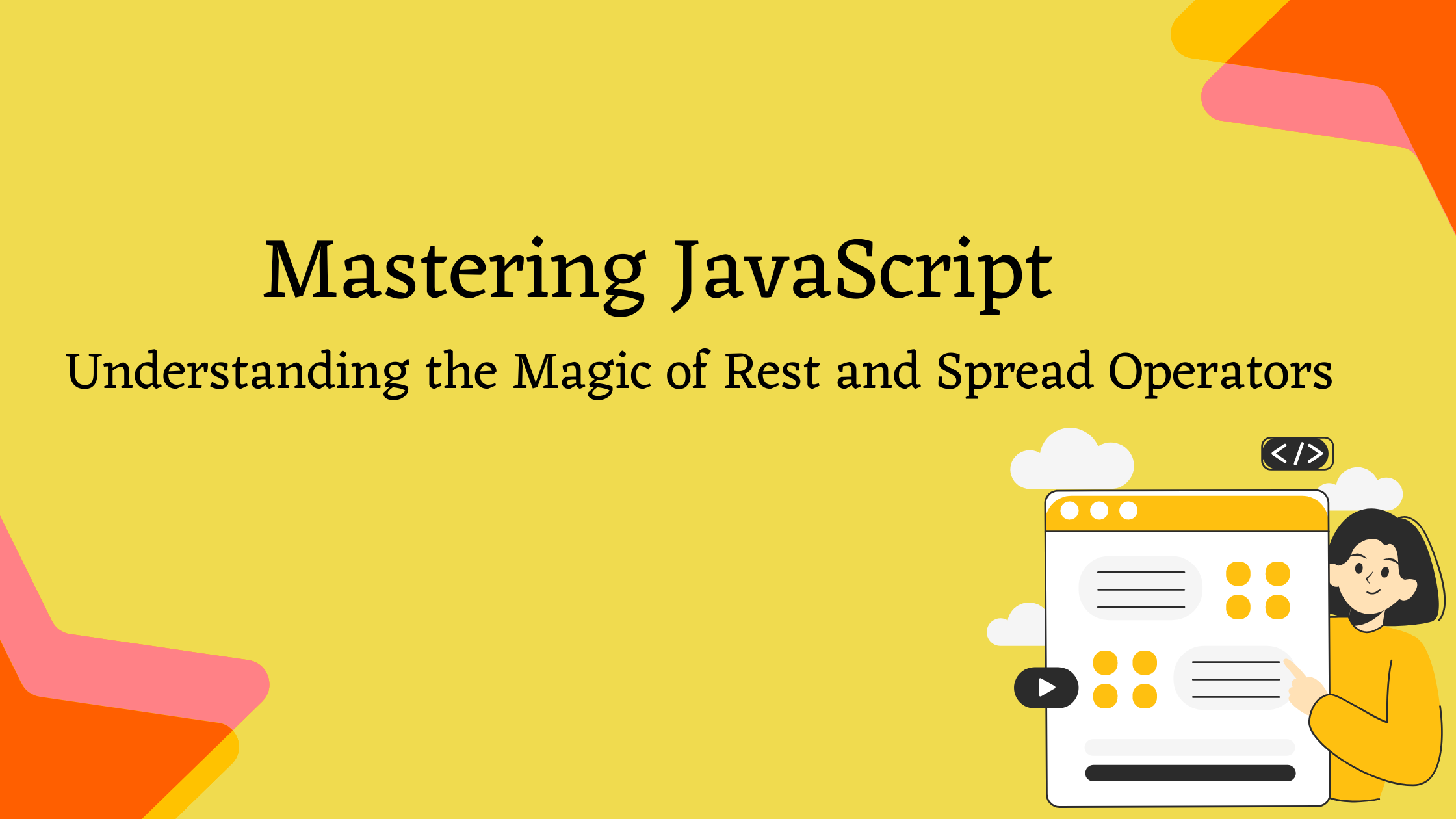
Ever found yourself repeatedly writing tedious code to merge arrays or handle function arguments dynamically? I have, and trust me, there’s a much better way to do it. Enter the magic of the rest and spread operators (...args)! Let me share how I discovered them and how they made my JavaScript code cleaner and more efficient.
Scene 1: The Tedious Task of Merging Arrays
I was working on a production-level project, where I needed to merge multiple arrays into one. Before discovering the spread operator, I used the good old concat()
method like this:
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const combined = array1.concat(array2);
console.log(combined); // [1, 2, 3, 4, 5, 6]
It worked, but every time I had to merge arrays, my code started to look cluttered. That’s when I stumbled upon the spread operator (...
), and it changed everything.
Scene 2: The Spread Operator Saves the Day
The spread operator allows you to expand or "spread" an array or object, making operations like merging or copying way simpler.
Here’s how I rewrote the array merge example using the spread operator:
const array1 = [1, 2, 3];
const array2 = [4, 5, 6];
const combined = [...array1, ...array2];
console.log(combined); // [1, 2, 3, 4, 5, 6]
Boom! Just like that, merging arrays became cleaner and faster. It’s not just for arrays though—you can use the spread operator on objects too.
Scene 3: Using Spread in Objects
In another project, I had two different user objects, one holding basic info and the other containing preferences. I needed to combine them into one.
Here’s how I did it using the spread operator:
const basicInfo = { name: "John", age: 30 };
const preferences = { theme: "dark", language: "English" };
const userProfile = { ...basicInfo, ...preferences };
console.log(userProfile); // { name: 'John', age: 30, theme: 'dark', language: 'English' }
In a single line of code, I was able to merge the objects. Imagine how much time it saved in a larger application with lots of merging happening in various functions.
Scene 4: Enter the Rest Operator
Let’s talk about the rest operator now. If the spread operator expands things, the rest operator collects them. It’s particularly useful when you’re dealing with functions that need to accept a variable number of arguments.
For example, I was working on a function that needed to sum any number of arguments. With the traditional approach, I used arguments
, but it was messy. The rest operator made my code way more elegant:
function sum(...numbers) {
return numbers.reduce((total, number) => total + number, 0);
}
console.log(sum(1, 2, 3)); // 6
console.log(sum(5, 10, 15, 20)); // 50
Here, the ...numbers
collects all arguments passed to the sum
function into an array. Now the function can handle as many numbers as you throw at it without breaking a sweat.
Scene 5: Using Rest in Objects
The rest operator is not just limited to function arguments; you can also use it with objects. Let’s say I had a user object, but I only wanted to extract certain properties and ignore the rest.
Here’s how I did it:
const user = { name: "Alice", age: 25, city: "Wonderland", occupation: "Engineer" };
const { name, age, ...rest } = user;
console.log(name); // Alice
console.log(age); // 25
console.log(rest); // { city: 'Wonderland', occupation: 'Engineer' }
By using the rest operator in destructuring, I could pull out name
and age
, while collecting the remaining properties (city
and occupation
) into the rest
variable. This is super useful when you want to separate core properties from extras in a production app.
Scene 6: Practical Use Cases in Production
Q: How do these operators benefit real-world production code?
Handling Dynamic Function Arguments: In a project that involved calculating shopping cart totals, I used the rest operator to collect all the item prices passed into the function. This way, the function could handle any number of items dynamically without modifying the function signature.
function calculateTotal(...prices) { return prices.reduce((total, price) => total + price, 0); }
Merging and Copying Objects: In a Redux state management project, I often needed to update parts of the state immutably. The spread operator was invaluable in ensuring that objects were copied properly:
const newState = { ...currentState, newProperty: 'value' };
Simplifying API Responses: While working with an API response, I needed to extract specific data and ignore the rest. Rest and spread operators helped streamline the process:
const { importantData, ...extraData } = apiResponse;
Final Thoughts: Why Rest and Spread are Game-Changers
Rest and spread operators have become essential tools in modern JavaScript development. They allow you to write cleaner, more concise code while reducing the need for manual argument handling, array manipulation, and object merging.
By incorporating them into my production-level projects, I’ve saved time and effort, and my code has become more readable and maintainable.
So next time you're dealing with multiple arguments, merging arrays, or combining objects, remember the power of the ...
operator—it’s the little magic that makes a big difference!
Thank You!
Thank you for reading!
I hope you enjoyed this post. If you did, please share it with your network and stay tuned for more insights on software development. I'd love to connect with you on LinkedIn or have you follow my journey on HashNode for regular updates.
Happy Coding!
Darshit Anjaria
Subscribe to my newsletter
Read articles from Darshit Anjaria directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Darshit Anjaria
Darshit Anjaria
Experienced professional with 4.5+ years in the industry, collaborating effectively with developers across domains to ensure timely project delivery. Proficient in Android/Flutter and currently excelling as a backend developer in Node.js. Demonstrated enthusiasm for learning new frameworks, with a quick-learning mindset and a commitment to writing bug-free, optimized code. Ready to learn and adopt to cloud technologies.