Understanding the Event Loop in JavaScript
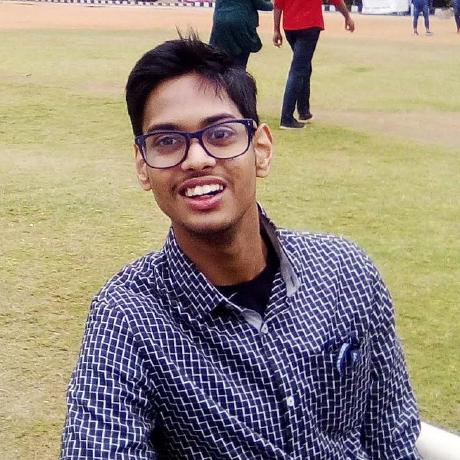
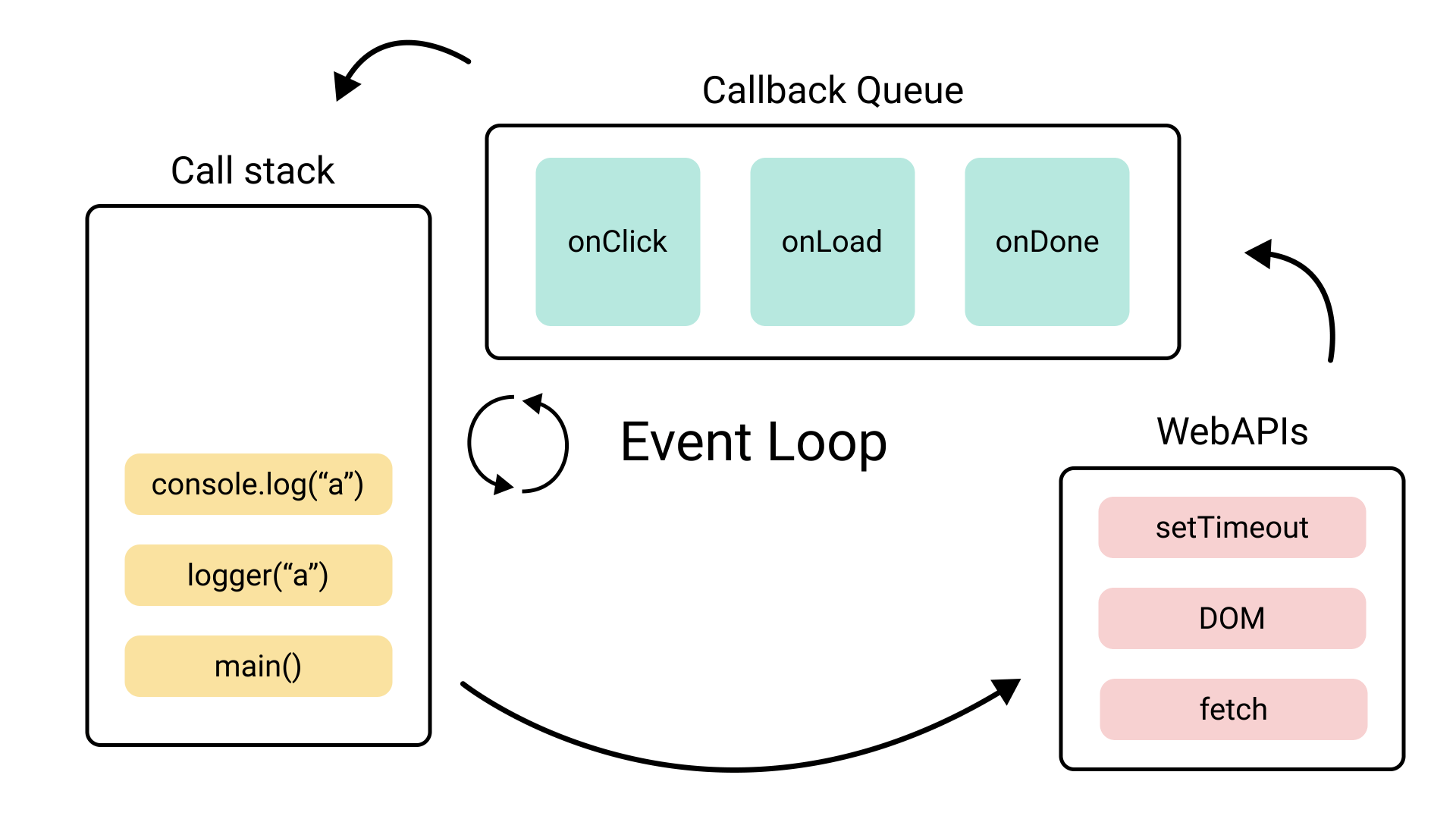
JavaScript is known for being a single-threaded, non-blocking, asynchronous programming language. But how does JavaScript manage to handle multiple tasks simultaneously, despite having only one call stack? The secret lies in the event loop.
The event loop is an essential concept for anyone diving into JavaScript, especially when dealing with asynchronous operations like callbacks, promises, and async/await
.
How JavaScript Works
JavaScript operates on a single thread, meaning it can only do one thing at a time. It has a single call stack, where it processes functions sequentially. This may raise questions like, "How does JavaScript handle things like HTTP requests, timers, and event handlers without blocking the entire application?"
That’s where the event loop steps in.
Components of the Event Loop System
Call Stack
The call stack is where the function execution context is managed. Each function call creates a new frame on the stack, which is removed when the function completes. JavaScript runs synchronously, meaning it processes code line by line, placing function calls on the stack and popping them off once they finish.Web APIs
When you call functions that are asynchronous, such assetTimeout
,fetch
, or event listeners, JavaScript hands them off to Web APIs. These APIs can handle timers, network requests, and other asynchronous tasks independently, freeing up the call stack.Callback Queue (Task Queue)
Once an asynchronous operation is completed, the associated callback function is placed into the callback queue. The queue follows the First In, First Out (FIFO) principle, so callbacks are executed in the order they are added to the queue.Microtask Queue
In addition to the callback queue, JavaScript has a microtask queue, primarily used for promises andMutationObserver
tasks. The microtask queue has a higher priority than the callback queue. Before the event loop checks the callback queue, it first processes the microtask queue.Event Loop
The event loop continuously monitors both the call stack and the callback queue. Its main job is to check whether the call stack is empty. If it is, it pushes the first function from the callback queue (or microtask queue) onto the call stack for execution. This cycle repeats, ensuring JavaScript can handle asynchronous tasks while maintaining a non-blocking flow.
How the Event Loop Works in Practice
Let’s go through a simple example:
console.log('Start');
setTimeout(() => {
console.log('Inside setTimeout');
}, 0);
console.log('End');
Step-by-step breakdown:
'Start'
is logged immediately because theconsole.log('Start')
statement is synchronous.setTimeout()
is called, but since it’s asynchronous, the function inside it is passed to the Web API. JavaScript doesn’t wait for the timer and continues.'End'
is logged as the next synchronous operation.Once the stack is clear, the event loop checks the callback queue and places the
setTimeout
callback onto the stack, logging'Inside setTimeout'
.
Event Loop and Promises
Promises operate with the microtask queue, meaning they are prioritized over standard asynchronous callbacks like setTimeout
. Let’s look at an example:
console.log('Start');
setTimeout(() => {
console.log('Inside setTimeout');
}, 0);
Promise.resolve().then(() => {
console.log('Inside Promise');
});
console.log('End');
In this case:
'Start'
is logged.setTimeout()
is passed to the Web API.The
Promise.resolve()
creates a promise and places the.then()
callback in the microtask queue.'End'
is logged.The event loop checks the microtask queue first, executing the promise callback and logging
'Inside Promise'
.Then, it processes the callback queue, logging
'Inside setTimeout'
.
Why Is the Event Loop Important?
Understanding the event loop helps prevent common pitfalls in JavaScript, especially when dealing with async programming. For example:
Avoid blocking the main thread: Long-running, synchronous operations can block the event loop and degrade performance.
Proper use of async functions: Knowing how the event loop works ensures you don’t mistakenly expect asynchronous functions to behave like synchronous ones.
Efficient promise handling: Realizing that promises are handled via the microtask queue helps optimize your code, as promises are processed faster than callbacks.
Conclusion
The event loop is the beating heart of JavaScript's asynchronous capabilities. By offloading async tasks to the Web APIs and using the callback queue, JavaScript maintains a non-blocking flow. For developers, mastering the event loop is crucial for writing efficient, performant, and responsive JavaScript applications.
Subscribe to my newsletter
Read articles from Anish Agrawal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
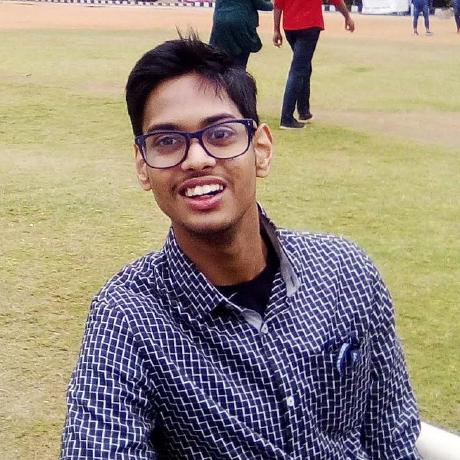