A Comprehensive Guide to Web Scraping with Python

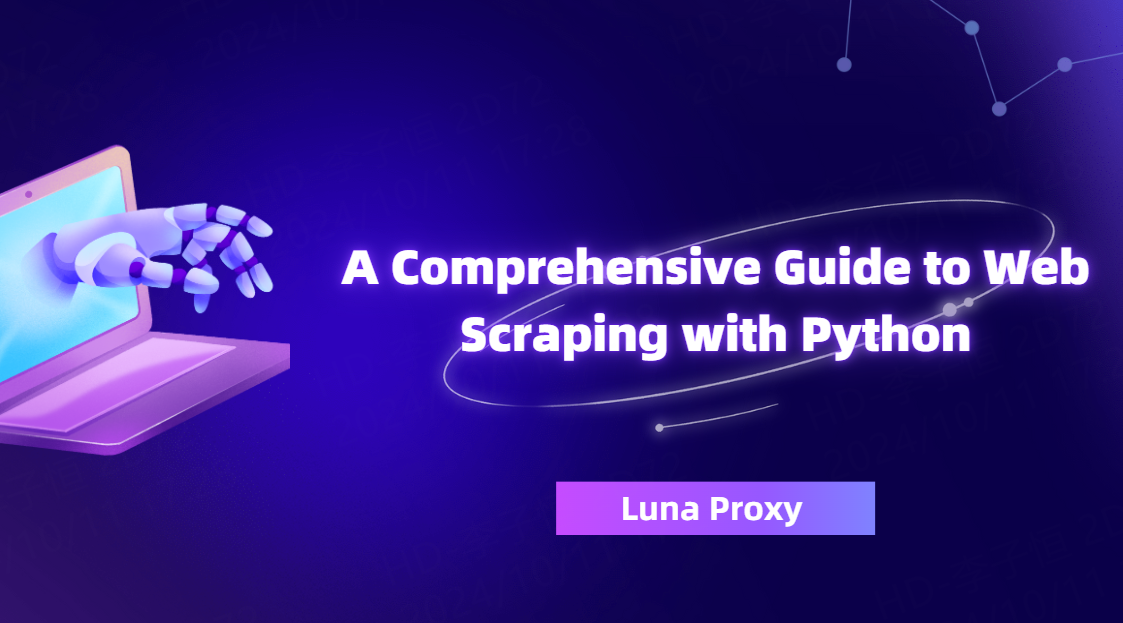
Web scraping has become an essential skill for many developers, data analysts, and businesses. Whether you're collecting data from competitors, tracking pricing trends, or extracting useful information from various websites, web scraping can be a powerful tool. Python, in particular, is one of the most popular programming languages for web scraping due to its simplicity and vast ecosystem of libraries.
What is Web Scraping?
Web scraping is the automated process of extracting data from websites. Instead of manually copying and pasting data from web pages, you can write a script that pulls the information for you. This can save significant amounts of time, particularly when working with large volumes of data.
Why Use Python for Web Scraping?
Python is highly regarded for web scraping for several reasons:
Ease of Use: Python’s simple syntax makes it accessible for beginners and fast for experienced developers.
Libraries: Python has a rich ecosystem of libraries designed for web scraping, such as BeautifulSoup, Scrapy, and Selenium.
Community Support: With an active community, there’s no shortage of tutorials, documentation, and help forums to guide you through any challenges.
Key Libraries for Web Scraping in Python
There are several popular Python libraries that simplify the web scraping process. Below are some of the most commonly used ones:
1. BeautifulSoup
BeautifulSoup is a Python library used for parsing HTML and XML documents. It creates a parse tree for web pages that can be used to extract data easily. It’s simple to use and great for beginners.
from bs4 import BeautifulSoup
import requests
# Fetch the page content
url = "https://example.com"
response = requests.get(url)
# Parse the HTML content with BeautifulSoup
soup = BeautifulSoup(response.text, 'html.parser')
# Extract the data you need
print(soup.title.text)
2. Scrapy
Scrapy is a more advanced web scraping framework that allows you to build robust and scalable web scrapers. It is ideal when scraping larger websites or when handling more complex scraping tasks.
pip install scrapy
import scrapy
class ExampleSpider(scrapy.Spider):
name = "example"
start_urls = ['https://example.com']
def parse(self, response):
title = response.css('title::text').get()
yield {'title': title}
3. Selenium
Selenium is a powerful tool when dealing with JavaScript-heavy websites. Unlike BeautifulSoup and Scrapy, Selenium can interact with a web page just like a real user would, which makes it perfect for scraping dynamic content.
pip install selenium
from selenium import webdriver
# Initialize WebDriver
driver = webdriver.Chrome()
driver.get("https://example.com")
# Extract the page title
print(driver.title)
# Close the browser
driver.quit()
Ethical and Legal Considerations of Web Scraping
Before you start scraping websites, it’s essential to consider the ethical and legal implications of your actions. Not all websites permit scraping, and some have terms of service that explicitly prohibit it. Ignoring these rules can lead to legal repercussions, such as being blocked from the site or even facing lawsuits.
Key Guidelines to Follow:
Respect the website’s
robots.txt
file: This file specifies which parts of a website can be crawled by bots. Always check it before scraping.Do not overload servers: Be mindful of the website’s server load by not sending too many requests in a short period. Use techniques like rate-limiting or adding random delays between requests.
Use public APIs when available: Many websites provide APIs that are designed for data access. Always prefer using an API over scraping where possible.
Example of Checking robots.txt
import requests
robots_url = "https://example.com/robots.txt"
response = requests.get(robots_url)
print(response.text)
Common Challenges in Web Scraping
While web scraping can be a powerful tool, it does come with its challenges. Some of the most common difficulties include:
JavaScript-rendered content: Many modern websites load content dynamically with JavaScript, making it invisible to libraries like BeautifulSoup. In such cases, Selenium or tools like Playwright can help.
Anti-scraping mechanisms: Some websites implement protections to block scraping, such as CAPTCHAs, IP blocking, or rate-limiting. Using proxies, rotating user agents, and handling cookies carefully can sometimes overcome these barriers.
Changing website structures: Websites frequently change their HTML structure, which can break your scraper. Regular maintenance of your scraping scripts is necessary to keep them functioning.
Best Practices for Web Scraping
Here are a few tips to ensure your web scraping project runs smoothly:
Start small: Begin by scraping a small portion of the data to test your script before scaling up.
Stay organized: Make sure to store scraped data in an organized format, such as CSV or JSON, and track your progress.
Handle errors: Always include error handling in your code to manage unexpected events, such as a page not loading or changes to the website’s structure.
Respect rate limits: If a website has a limit on the number of requests you can make in a given time period, be sure to respect that to avoid being blocked.
Conclusion
Web scraping with Python can unlock a world of possibilities for data collection and analysis. Whether you’re a beginner or an experienced developer, Python’s range of libraries and ease of use make it a fantastic choice for scraping projects. However, it’s essential to approach scraping with a clear understanding of the ethical and legal landscape, ensuring that your methods are respectful and responsible.
By following best practices and keeping up with the latest tools, you’ll be able to efficiently and effectively scrape data from the web, opening new doors for your projects and insights.
Subscribe to my newsletter
Read articles from Marx Lee directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
