From Creation to Collection: Journey of A Java Variable
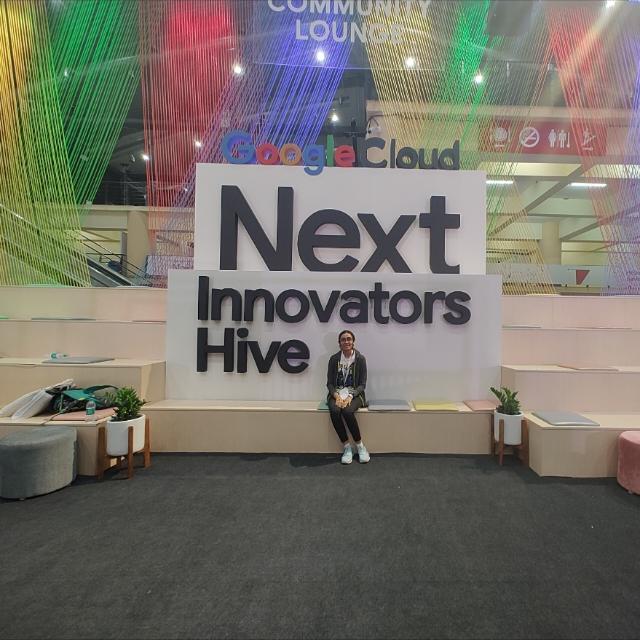
Before diving into the details, it's important to note that a variable in Java embarks on a fascinating journey from its initial creation in an IDE to the moment it’s cleaned up by the garbage collector. Along the way, it interacts with various parts of memory and the CPU, each step playing a crucial role in the execution of your program.
1. Declaration in the IDE
When we start writing a Java program, we use an Integrated Development Environment (IDE) to declare your variables. This is where we specify the type of data we want to store.
Let us consider a variable ‘a’ of Integer type.
int a = 10;
This variable ‘a’ will hold the integer type data throughout its lifecycle.
2. Compilation
The next step is to compile the piece of code we have written. Compiling is the process where the Java compiler (javac) translates our high-level Java code into an intermediate form called bytecode,and it is stored in .class files. This bytecode is platform-independent, meaning it can run on any system that has a Java Virtual Machine (JVM).
3. Class Loading
The JVM takes control by loading the compiled bytecode from the .clas files into memory. This process includes verifying the bytecode for correctness to ensure it meets Java’s security and safety standards, followed by preparing the necessary data structures to store the class's metadata, methods, and variables.
4. Overview of Memory Architecture
Java manages memory through different areas, each serving a specific purpose:
CPU Registers: These are the fastest storage locations within the CPU that hold data currently being processed.
Cache Memory: This is a small, high-speed storage area close to the CPU that stores frequently accessed data to speed up processing.
Main Memory (RAM): This is where active data and program instructions are stored while the program is running.
Storage: Hard drives and SSDs provide persistent storage for data that needs to be retained even when the computer is turned off.
5. Variable Initialization
When the JVM executes our program, it allocates memory to the variables. This allocation occurs in the following areas:
Heap Memory: This is where all Java objects and their instance variables are allocated. Whenever you create an object using the new keyword, memory is allocated in the heap.
Stack Memory: Each thread in Java has its stack, which stores method calls and local variables. When a method is invoked, a new stack frame is created, containing local variables. Once the method execution completes, this memory is deallocated, and the stack frame is removed.
6. Execution
As the program executes, it reaches the point where our variable is utilized:
At this stage, the CPU fetches the instruction from RAM, retrieves the ‘a’ from the heap, and processes the output. The CPU interacts with both RAM and cache to ensure efficient data access, executing instructions sequentially.
System.out.println(a);
7. Scope and Lifetime
The variable exists within the context of its scope. For local variables, this means they are accessible only within the method or block in which they were declared. Once the method completes execution, the variable goes out of scope and becomes eligible for garbage collection if there are no remaining references to it.
8. Eligibility for Garbage Collection
If a variable goes out of scope or is explicitly set to null, it becomes eligible for garbage collection. The JVM tracks objects that are no longer reachable through active references, ensuring memory is effectively managed and freed when no longer in use.
9. Garbage Collection Process
The garbage collector is a crucial part of Java’s memory management system. It operates in the background and can be triggered under certain conditions, such as low memory.
Finally, the journey of the variable comes to an end, marking the conclusion of its delightful ride through memory. As it gracefully exits the stage, it leaves behind a more efficient and well-managed environment for the next adventure in Java programming.
Subscribe to my newsletter
Read articles from Laasya Kandukuri directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
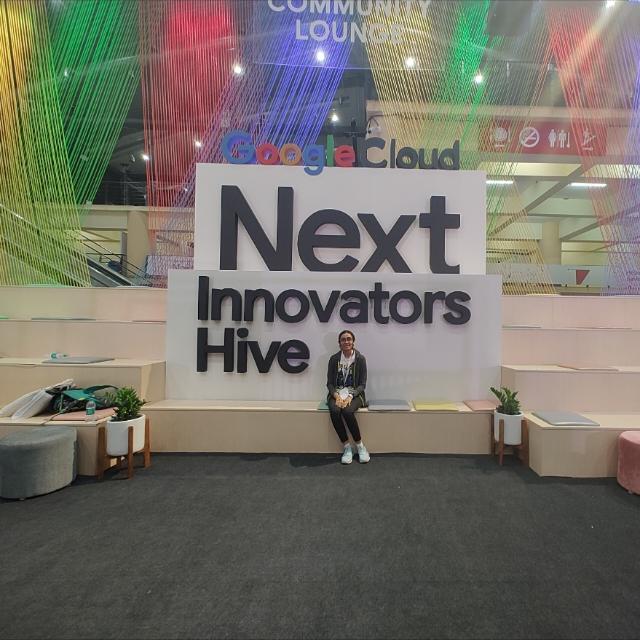
Laasya Kandukuri
Laasya Kandukuri
Hello, I'm Laasya, a Computer Science Engineering graduate passionate about Java development. Skilled in building efficient backend systems and solving complex problems through object-oriented programming. Eager to explore new challenges in software development and backend technologies.