Idempotency In Laravel

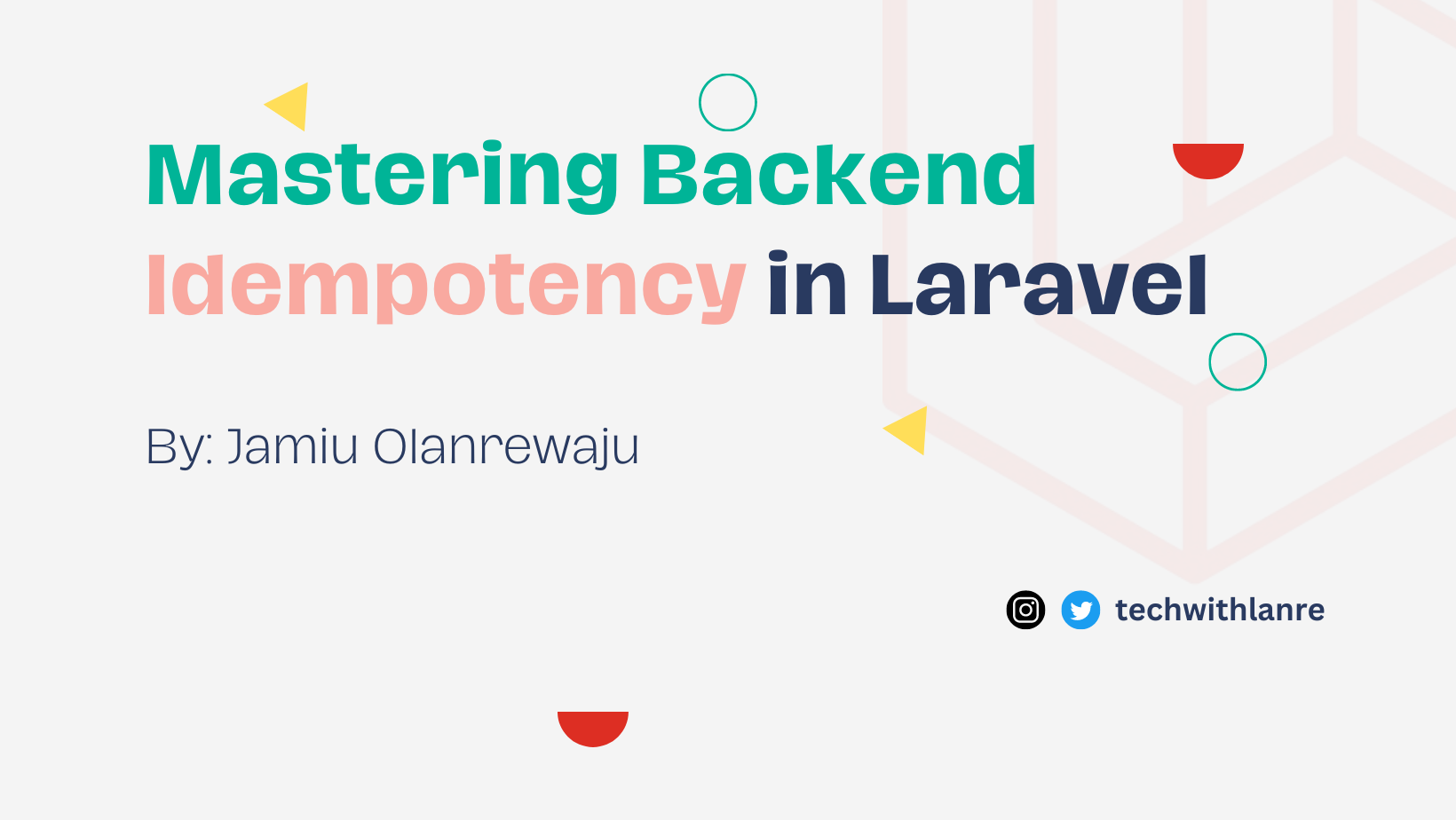
In modern web applications, ensuring that certain operations, such as payment processing or resource creation, are only executed once—even if the client retries the request—is critical. This behavior is achieved through idempotency. In this article, we will walk through how to implement idempotency in a Laravel API, ensuring that repeated requests are processed only once.
What is Idempotency?
Idempotency refers to the property of certain operations where the result remains the same no matter how many times the operation is performed. In APIs, this is particularly useful for preventing duplicate operations when clients retry requests due to network issues or timeouts.
For example, submitting a payment twice should not result in two charges. By implementing idempotency, we ensure that only one payment is processed, regardless of how many times the client retries the request.
When Should You Implement Idempotency?
Idempotency is crucial in scenarios where:
Transactions are involved, such as payments or transfers.
Resource creation can lead to duplication, like creating orders, bookings, or accounts.
Client retries due to timeouts or network failures are common, and the client cannot know if the operation was successful.
Steps to Implement Idempotency in Laravel
1. Setting up an idempotency_key
The first step is to provide a mechanism for tracking requests. This is usually done by introducing an idempotency_key
. The client generates this key and sends it along with the request. If the same key is used again, the server should return the result of the first request without re-processing the operation.
Creating the Database Migration
You need to add an idempotency_key
column to the table that tracks the operation, such as a transactions
or orders
table.
php artisan make:migration add_idempotency_key_to_transactions_table
In the generated migration file, define the idempotency_key
column:
public function up()
{
Schema::table('transactions', function (Blueprint $table) {
$table->string('idempotency_key')->nullable()->unique();
});
}
public function down()
{
Schema::table('transactions', function (Blueprint $table) {
$table->dropColumn('idempotency_key');
});
}
Run the migration:
php artisan migrate
This will add a idempotency_key
column to your transactions
table, which will store the unique key for each idempotent request.
2. Creating Middleware to Handle Idempotency
The next step is to create middleware that checks whether an idempotency_key
has already been processed.
Generate the middleware:
php artisan make:middleware IdempotencyMiddleware
In app/Http/Middleware/IdempotencyMiddleware.php
, add the following code:
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
use App\Models\Transaction;
class IdempotencyMiddleware
{
public function handle(Request $request, Closure $next)
{
$idempotencyKey = $request->header('Idempotency-Key');
// If no idempotency key is provided, proceed as usual.
if (!$idempotencyKey) {
return $next($request);
}
// Check if the idempotency key already exists in the database
$existingTransaction = Transaction::where('idempotency_key', $idempotencyKey)->first();
if ($existingTransaction) {
// If the transaction already exists, return the cached result
return response()->json([
'message' => 'Idempotent request already processed.',
'transaction' => $existingTransaction
], 200);
}
return $next($request);
}
}
This middleware checks for the presence of an Idempotency-Key
in the request header. If the key is found and it has already been used, the middleware returns the result of the previous request.
3. Applying Middleware to Routes
Now that we have the middleware, you can apply it to the routes that require idempotency, such as payment or order creation routes.
In routes/api.php
, apply the middleware to the specific route:
Route::post('transactions', [TransactionController::class, 'store'])->middleware('idempotent');
4. Storing the Idempotency Key and Processing the Request
In the controller handling the request (e.g., TransactionController
), store the idempotency key when the request is processed for the first time. This ensures that future requests with the same key are not re-processed.
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Transaction;
class TransactionController extends Controller
{
public function store(Request $request)
{
$idempotencyKey = $request->header('Idempotency-Key');
// Create a new transaction and store the idempotency key
$transaction = Transaction::create([
'amount' => $request->input('amount'),
'user_id' => $request->input('user_id'),
'idempotency_key' => $idempotencyKey
]);
return response()->json([
'message' => 'Transaction created successfully.',
'transaction' => $transaction
], 201);
}
}
5. Handling Concurrency and Race Conditions
To avoid race conditions where the same idempotency_key
might be used simultaneously, you can use a database lock or wrap the operation inside a database transaction.
For example, using a transaction in Laravel:
use Illuminate\Support\Facades\DB;
DB::transaction(function () use ($request, $idempotencyKey) {
// Check if the idempotency key has already been used
$existingTransaction = Transaction::where('idempotency_key', $idempotencyKey)->first();
if (!$existingTransaction) {
// If not, create a new transaction
$transaction = Transaction::create([
'amount' => $request->input('amount'),
'user_id' => $request->input('user_id'),
'idempotency_key' => $idempotencyKey
]);
return response()->json([
'message' => 'Transaction processed successfully.',
'transaction' => $transaction
], 201);
}
// Return the existing transaction
return response()->json([
'message' => 'Idempotent request already processed.',
'transaction' => $existingTransaction
], 200);
});
Conclusion
Implementing idempotency in Laravel is a powerful way to ensure your API is robust and resilient to repeated requests due to client retries, network failures, or timeouts. By adding an idempotency_key
, creating middleware to handle duplicate requests, and storing this key alongside the original request, you prevent duplicate operations and make your API safer.
Key steps include:
Adding an
idempotency_key
column to your database.Creating middleware to handle idempotency checks.
Storing the
idempotency_key
when processing the request.Handling concurrency to avoid race conditions.
By following this guide, you can ensure that your critical operations, like payments or order creation, remain idempotent, improving both user experience and system reliability.
Subscribe to my newsletter
Read articles from Jamiu Olanrewaju directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Jamiu Olanrewaju
Jamiu Olanrewaju
I am a Fullstack Developer from Nigeria, I craft solutions with Laravel, VueJS and Flutter.