Rust ownership and borrowing
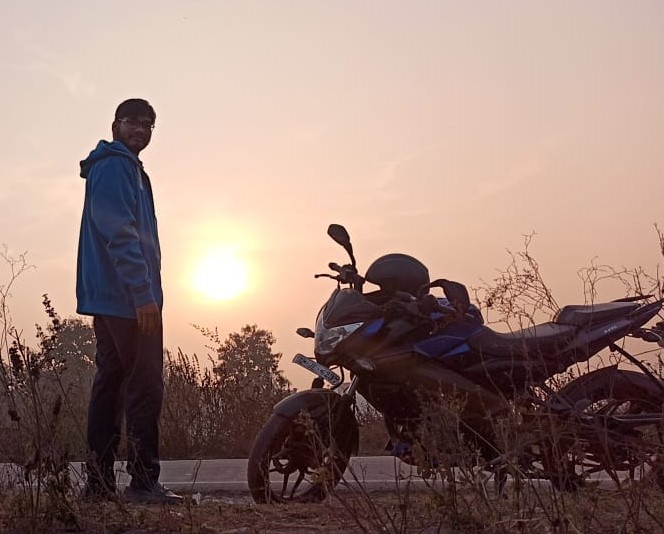
This is my attempt at explaining Rust ownership and borrowing rules. Lets look at the rules first.
Each value in Rust has an owner.
There can only be one owner at a time.
When the owner goes out of scope, the value will be dropped.
Before understanding the rules let’s first understand the meaning of words that are used in these rules, few such words are owner
and scope
.
Owner
In English “Owner” means a person who owns something, In other words when something belongs to a person, that person is called owner of that particular thing.
Now, replace person with variable and something with value and you have the definition of owner for rust.
Scope
In English “Scope” mean the extend of area that do or deals with something, In other words Scope is an area within an bigger area that does or deals with some specific thing.
In rust {}
anything within these brackets is a scope even main.rs
file is a global scope within that there is main()
function which is a scope.
Below is the program which will help us understand rust ownership and borrowing.
// Global scope.
use std::io;
fn main() { // main function scope.
let mut user_input = String:new();
io::stdin().read_line(&mut user_input);
{ // Inner scope that does the printing of user's input
println!("your input was: {user_input}")
}
}
here are few questions that came in my mind when I first this.
Q. why do we have let mut user_input
why not let user_input
?
A. Because read_line
function take a String type as an argument and appends the data on to that string. therefore, so that read_line
function can append data on that String variable we’ll need to define it as a mutable variable.
Q. Why can we pass user_input
as is?
A. Just like we have a scope for main function slandered library would also have a scope for read_line
function so if we have read_line(user_input)
the user_input
value will be freed (not available for us to use) as read_line
function finishes its computation
Q Why can’t we pass &user_input
to read_line
function?
A. As &
give the reference to user_input
variable meaning it does not move it. it only gives a pointer where user_input
’s value is stored in memory(heap), read line will have to append (update/change) the current value of user_input
. and thus we pass &mut user_input
saying that here is the mutable reference meaning pointer to user_input
value as well as permission for you to update/change its value.
That’s it. answer of these 3 questions are enough for me to understand what those rules means and helps me understand that above code that we wrote.
If you have any other questions let me know and I’ll try to answer them for you as well :)
Thanks.
Bye
Subscribe to my newsletter
Read articles from Sahaj Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
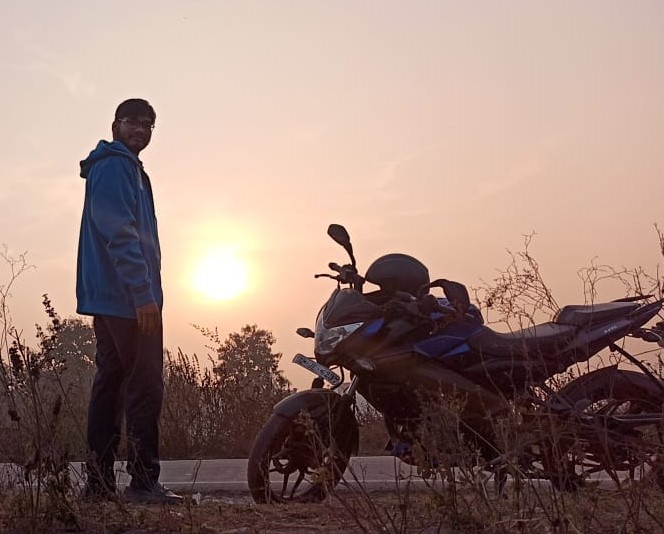