Creating a Maintenance Page in Next.js: A Comprehensive Guide
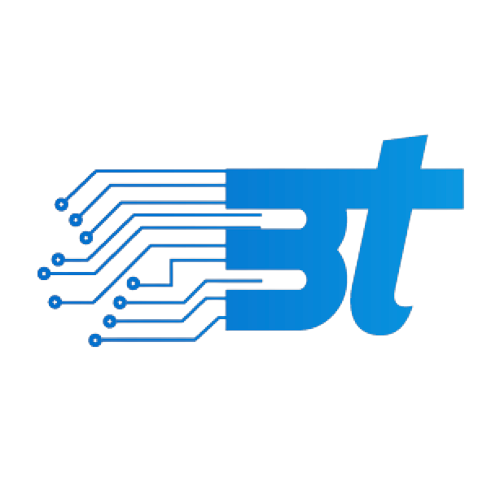
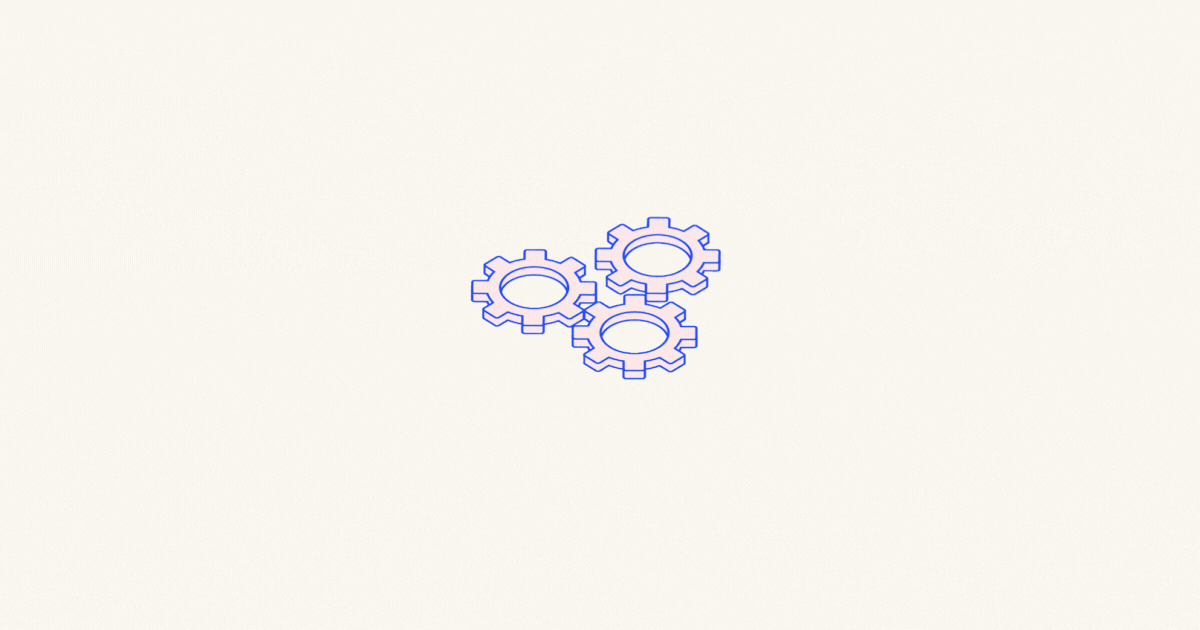
When building web applications, maintenance periods are inevitable. Whether you're deploying updates, performing server migrations, or addressing unforeseen issues, displaying a maintenance page ensures that your users are informed and your site remains professional during downtimes. In this comprehensive guide, we'll walk you through creating a robust maintenance page in Next.js, a powerful React framework renowned for its server-side rendering and static site generation capabilities.
Why Implement a Maintenance Page?
Before diving into the implementation, it's essential to understand why a maintenance page is crucial:
User Experience: Inform users about ongoing maintenance to prevent confusion and frustration.
SEO Considerations: Proper maintenance pages can signal search engines about temporary downtimes, preventing negative SEO impacts.
Professionalism: A well-designed maintenance page maintains your brand's professionalism even during downtimes.
Security: Temporarily restricting access can prevent exposure to vulnerabilities during updates.
Prerequisites
To follow along with this guide, ensure you have:
Node.js installed on your machine.
Basic understanding of Next.js and React.
Familiarity with JavaScript and TypeScript (optional, as Next.js supports both).
Approaches to Creating a Maintenance Page in Next.js
There are multiple ways to implement a maintenance page in Next.js. We'll explore three primary approaches:
1. Using Middleware
Next.js 12 introduced Middleware, allowing you to run code before a request is completed. This is a powerful feature to intercept requests and redirect users to a maintenance page.
2. Custom Server Setup
By configuring a custom server (using Node.js or another server framework), you can control how requests are handled and redirect them to a maintenance page as needed.
3. Environment Variables and Conditional Rendering
Utilizing environment variables, you can toggle maintenance mode on or off, conditionally rendering the maintenance page based on the current state.
Step-by-Step Implementation
Let's implement the maintenance page using Middleware, as it's the most efficient and aligns with Next.js best practices.
Step 1: Setting Up Your Next.js Project
If you don't already have a Next.js project, you can create one using the following commands:
npx create-next-app@latest nextjs-maintenance-page
cd nextjs-maintenance-page
Step 2: Creating the Maintenance Page Component
First, create a dedicated maintenance page. This page will inform users about the maintenance status.
Create a new file at pages/maintenance.js
:
// pages/maintenance.js
import React from 'react';
import Head from 'next/head';
const Maintenance = () => {
return (
<>
<Head>
<title>Site Under Maintenance</title>
</Head>
<div style={styles.container}>
<h1 style={styles.heading}>We'll be back soon!</h1>
<p style={styles.paragraph}>
Sorry for the inconvenience but we're performing some maintenance at the moment. We'll
be back online shortly!
</p>
<div style={styles.spinner}></div>
</div>
</>
);
};
const styles = {
container: {
height: '100vh',
display: 'flex',
flexDirection: 'column',
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#f3f4f6',
textAlign: 'center',
padding: '0 20px',
},
heading: {
fontSize: '2.5rem',
marginBottom: '1rem',
color: '#111827',
},
paragraph: {
fontSize: '1.25rem',
marginBottom: '2rem',
color: '#6b7280',
},
spinner: {
width: '50px',
height: '50px',
border: '6px solid #e5e7eb',
borderTopColor: '#3b82f6',
borderRadius: '50%',
animation: 'spin 1s linear infinite',
},
};
// Adding keyframes for spinner animation
const styleSheet = typeof document !== 'undefined' ? document.styleSheets[0] : null;
if (styleSheet) {
const keyframes =
`@keyframes spin {
to { transform: rotate(360deg); }
}`;
styleSheet.insertRule(keyframes, styleSheet.cssRules.length);
}
export default Maintenance;
Explanation:
Head Component: Sets the page title for better SEO and user experience.
Styling: Inline styles are used for simplicity. For larger projects, consider using CSS modules or styled-components.
Spinner: A simple CSS spinner provides visual feedback.
Step 3: Implementing Middleware for Redirection
Next.js Middleware allows you to execute code before a request is processed. We'll use it to check if the site is in maintenance mode and redirect accordingly.
Create a new file at middleware.js
in the root directory:
// middleware.js
import { NextResponse } from 'next/server';
// Define paths that should not trigger the maintenance mode (e.g., maintenance page itself, API routes)
const maintenancePaths = ['/maintenance', '/_next', '/api'];
export function middleware(req) {
const url = req.nextUrl.clone();
const { pathname } = url;
// Bypass middleware for maintenance page and API routes
if (maintenancePaths.some((path) => pathname.startsWith(path))) {
return NextResponse.next();
}
// Check if maintenance mode is enabled
const isMaintenance = process.env.MAINTENANCE_MODE === 'true';
if (isMaintenance) {
// Redirect to the maintenance page
url.pathname = '/maintenance';
return NextResponse.redirect(url);
}
return NextResponse.next();
}
Explanation:
Maintenance Paths: Define routes that should bypass the maintenance check, such as the maintenance page itself and API routes.
Environment Variable:
MAINTENANCE_MODE
controls whether the maintenance mode is active.Redirection: If maintenance mode is active and the request isn't for an excluded path, redirect the user to
/maintenance
.
Step 4: Controlling Maintenance Mode with Environment Variables
Using environment variables provides flexibility to toggle maintenance mode without changing the codebase.
# .env.local
MAINTENANCE_MODE=true
Explanation:
- MAINTENANCE_MODE: Set to
true
to enable maintenance mode orfalse
to disable it.
Important: Ensure that .env.local
is added to .gitignore
to prevent sensitive configurations from being pushed to version control.
Accessing Environment Variables in Middleware:
Next.js requires environment variables used in Middleware to be prefixed with NEXT_PUBLIC_
. However, since Middleware runs on the edge and doesn’t have access to server-side variables, we'll need to use a different approach.
Update the environment variable:
# .env.local
NEXT_PUBLIC_MAINTENANCE_MODE=true
Update the Middleware:
// middleware.js
import { NextResponse } from 'next/server';
const maintenancePaths = ['/maintenance', '/_next', '/api'];
export function middleware(req) {
const url = req.nextUrl.clone();
const { pathname } = url;
if (maintenancePaths.some((path) => pathname.startsWith(path))) {
return NextResponse.next();
}
const isMaintenance = process.env.NEXT_PUBLIC_MAINTENANCE_MODE === 'true';
if (isMaintenance) {
url.pathname = '/maintenance';
return NextResponse.redirect(url);
}
return NextResponse.next();
}
Caveat:
As of the latest Next.js versions, accessing process.env
directly in Middleware may not work as expected. An alternative is to use a file-based configuration or integrate with external services. For simplicity, we'll proceed with NEXT_PUBLIC_MAINTENANCE_MODE
.
Alternative: Using a JSON Configuration
If environment variables don't propagate correctly to Middleware, consider using a configuration file.
Create maintenance.json
in the root directory:
// maintenance.json
{
"enabled": true
}
Update Middleware to read the configuration:
// middleware.js
import { NextResponse } from 'next/server';
import maintenanceConfig from './maintenance.json';
const maintenancePaths = ['/maintenance', '/_next', '/api'];
export function middleware(req) {
const url = req.nextUrl.clone();
const { pathname } = url;
if (maintenancePaths.some((path) => pathname.startsWith(path))) {
return NextResponse.next();
}
if (maintenanceConfig.enabled) {
url.pathname = '/maintenance';
return NextResponse.redirect(url);
}
return NextResponse.next();
}
Benefits:
Dynamic Control: Easily toggle maintenance mode by editing
maintenance.json
.Simpler Access: Avoids complications with environment variables in Middleware.
Note: After making changes to Middleware or configuration files, restart the development server to apply updates.
Styling Your Maintenance Page
A maintenance page should be clear, concise, and visually appealing. While the example provided earlier uses inline styles for simplicity, consider enhancing it with better styling techniques.
Using CSS Modules
Create a CSS module for the maintenance page:
Create styles/Maintenance.module.css
:
/* styles/Maintenance.module.css */
.container {
height: 100vh;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
background-color: #f3f4f6;
text-align: center;
padding: 0 20px;
}
.heading {
font-size: 2.5rem;
margin-bottom: 1rem;
color: #111827;
}
.paragraph {
font-size: 1.25rem;
margin-bottom: 2rem;
color: #6b7280;
}
.spinner {
width: 50px;
height: 50px;
border: 6px solid #e5e7eb;
border-top-color: #3b82f6;
border-radius: 50%;
animation: spin 1s linear infinite;
}
@keyframes spin {
to {
transform: rotate(360deg);
}
}
Update pages/maintenance.js
to use the CSS module:
// pages/maintenance.js
import React from 'react';
import Head from 'next/head';
import styles from '../styles/Maintenance.module.css';
const Maintenance = () => {
return (
<>
<Head>
<title>Site Under Maintenance</title>
</Head>
<div className={styles.container}>
<h1 className={styles.heading}>We'll be back soon!</h1>
<p className={styles.paragraph}>
Sorry for the inconvenience but we're performing some maintenance at the moment. We'll
be back online shortly!
</p>
<div className={styles.spinner}></div>
</div>
</>
);
};
export default Maintenance;
Benefits:
Scoped Styles: CSS Modules scope styles locally, preventing conflicts.
Maintainability: Easier to manage and update styles as your project grows.
Enhancing with Tailwind CSS
For more advanced styling, integrating Tailwind CSS can expedite the design process.
Installation Steps:
Install Tailwind CSS:
npm install -D tailwindcss postcss autoprefixer npx tailwindcss init -p
Configure
tailwind.config.js
:// tailwind.config.js module.exports = { content: ['./pages/**/*.{js,ts,jsx,tsx}', './components/**/*.{js,ts,jsx,tsx}'], theme: { extend: {}, }, plugins: [], };
Add Tailwind directives to
styles/globals.css
:/* styles/globals.css */ @tailwind base; @tailwind components; @tailwind utilities;
Update
pages/_app.js
to include global styles:// pages/_app.js import '../styles/globals.css'; function MyApp({ Component, pageProps }) { return <Component {...pageProps} />; } export default MyApp;
Update pages/maintenance.js
with Tailwind classes:
// pages/maintenance.js
import React from 'react';
import Head from 'next/head';
const Maintenance = () => {
return (
<>
<Head>
<title>Site Under Maintenance</title>
</Head>
<div className="flex flex-col items-center justify-center min-h-screen bg-gray-100 text-center p-4">
<h1 className="text-4xl font-semibold text-gray-800 mb-4">We'll be back soon!</h1>
<p className="text-xl text-gray-600 mb-6">
Sorry for the inconvenience but we're performing some maintenance at the moment. We'll
be back online shortly!
</p>
<div className="w-12 h-12 border-4 border-gray-300 border-t-blue-500 rounded-full animate-spin"></div>
</div>
</>
);
};
export default Maintenance;
Benefits:
Rapid Styling: Tailwind's utility classes allow for quick and responsive designs.
Customization: Easily customize themes and components to match your brand.
Testing the Maintenance Page
Ensuring your maintenance page functions correctly across different scenarios is crucial.
Enable Maintenance Mode:
- Set
NEXT_PUBLIC_MAINTENANCE_MODE=true
in.env.local
or setenabled: true
inmaintenance.json
.
- Set
Run the Development Server:
npm run dev
Access Your Site:
Navigate to
http://localhost:3000/
and verify that you're redirected to the maintenance page.Try accessing
/maintenance
directly to ensure it loads without redirection loops.
Disable Maintenance Mode:
Set
NEXT_PUBLIC_MAINTENANCE_MODE=false
orenabled: false
.Restart the development server and confirm that the site is accessible normally.
Production Testing
Before deploying to production, it's advisable to test the maintenance mode in a staging environment to ensure seamless user experience.
Deploy to a Staging Environment:
- Use platforms like Vercel, Netlify, or your preferred hosting service.
Toggle Maintenance Mode:
- Update environment variables or configuration files as per your setup.
Verify Functionality:
- Access the staging site to confirm the maintenance page appears when enabled and the site functions normally when disabled.
Best Practices
Implementing a maintenance page is not just about redirection; it's about ensuring a smooth experience for your users and maintaining the integrity of your application. Here are some best practices to consider:
1. Clear Messaging
Informative Text: Clearly state that the site is undergoing maintenance.
Expected Downtime: If possible, provide an estimated time for when the site will be back online.
2. Consistent Branding
Logo and Colors: Use your brand's logo and color scheme to maintain consistency.
Typography: Stick to fonts that align with your brand guidelines.
3. Accessibility
Semantic HTML: Use proper HTML elements to ensure the page is accessible to screen readers.
Contrast Ratios: Ensure text has sufficient contrast against backgrounds for readability.
4. Performance Optimization
Minimal Assets: Keep the maintenance page lightweight to ensure quick loading times.
Caching: Leverage caching headers to serve the maintenance page efficiently.
5. SEO Considerations
Status Code: Serve a
503 Service Unavailable
status code to inform search engines that the downtime is temporary.Retry-After Header: Optionally, include a
Retry-After
header indicating when the site will be back.
Implementing SEO Headers:
Update the Middleware to include a 503
status code when redirecting to the maintenance page.
// middleware.js
import { NextResponse } from 'next/server';
import maintenanceConfig from './maintenance.json';
const maintenancePaths = ['/maintenance', '/_next', '/api'];
export function middleware(req) {
const url = req.nextUrl.clone();
const { pathname } = url;
if (maintenancePaths.some((path) => pathname.startsWith(path))) {
return NextResponse.next();
}
if (maintenanceConfig.enabled) {
url.pathname = '/maintenance';
const response = NextResponse.redirect(url);
response.status = 503;
response.headers.set('Retry-After', '3600'); // Retry after 1 hour
return response;
}
return NextResponse.next();
}
Note: Not all browsers and proxies respect the Retry-After
header, but it's beneficial for SEO and certain clients.
6. Allow Access to Admin Routes
Ensure that administrative or internal routes remain accessible even during maintenance to perform necessary updates.
Update Middleware Paths:
const maintenancePaths = ['/maintenance', '/_next', '/api', '/admin'];
Benefits:
Continuous Management: Allows site administrators to access backend functionalities.
Flexibility: Ensures maintenance tasks can be performed without additional downtimes.
Conclusion
Remember to adhere to best practices such as clear messaging, consistent branding, accessibility, and SEO considerations to maximize the effectiveness of your maintenance page. With this setup, you can confidently manage maintenance periods, ensuring minimal disruption and maintaining trust with your user base.
Happy Coding! 🚀
If you found this guide helpful, consider sharing it with fellow developers or leaving a comment below with your experiences and additional tips on managing maintenance modes in Next.js projects.
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
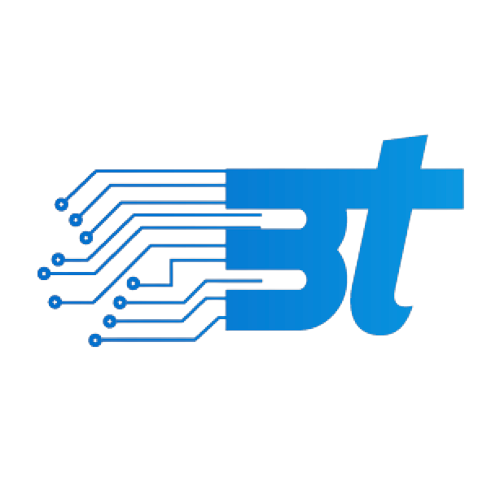
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.