Learning Go Data Types and Declarations Day 2
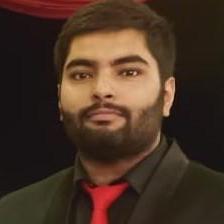
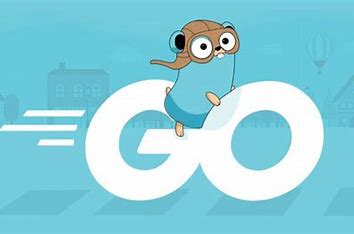
Today, I explored core concepts in Go, focusing on data types, constants, type conversions, and variable declarations. This session has deepened my understanding of how Go handles different types and how to efficiently work with them. Here’s a summary of my learnings.
Variable Declaration in Go
Go allows for various ways to declare variables, which makes the language flexible. There are three main ways to declare variables:
Using var
This is the most explicit method. You declare the type and optionally assign a value.
var i int = 10
Without Specifying the Type
If you don’t specify the type, Go will infer it based on the value.
var name = "Sahal" // Go infers that 'name' is a string
Short Declaration Syntax :=
Go allows for shorthand declaration, typically used inside functions. It both declares and initializes the variable in one step.
age := 25 // Equivalent to 'var age int = 25'
Each of these methods has its own use case. Explicitly declaring the type provides more clarity, while using inference can make the code cleaner. I used these variable declaration techniques throughout my practice today.
Complex Numbers in Go
Go has native support for complex numbers. I wrote a program to perform basic arithmetic operations (addition, subtraction, multiplication, division) on complex numbers. This was a great way to understand how Go handles more complex data types beyond just integers and strings.
x := complex(2.5, 3.1)
y := complex(10.2, 2)
fmt.Println(x + y) // Addition
fmt.Println(x - y) // Subtraction
fmt.Println(x * y) // Multiplication
fmt.Println(x / y) // Division
fmt.Println(real(x)) // Real part of x
fmt.Println(imag(x)) // Imaginary part of x
fmt.Println(cmplx.Abs(x)) // Magnitude of x
Rune Type in Go
The rune
type represents a Unicode code point, which can store any character from the UTF-8 set. I wrote a program to declare a rune
and print its integer (ASCII) value.
var myFirstInitial rune = 'J'
fmt.Println(myFirstInitial) // Output: 74, as 'J' is ASCII 74
Explicit Type Conversion
In Go, implicit type conversion is not allowed, so I practiced explicit type conversion by converting an integer to a floating-point number and vice versa.
var x int = 10
var y float64 = 30.2
var sum1 float64 = float64(x) + y // Converting int to float64
var sum2 int = int(y) // Converting float64 to int
fmt.Println(sum1, sum2) // Output: 40.2, 30
Working with Constants
Go supports constants that, once declared, cannot be modified. Constants are useful for values that shouldn’t change during the execution of the program. I experimented with single and grouped constant declarations.
const x int64 = 10
const y = "hello"
fmt.Println(x, y) // Output: 10, hello
Look-alike Code Points
Go differentiates between variables with similar-looking Unicode characters. This led me to experiment with look-alike characters (a
vs. a
).
a := "hello" // Unicode U+FF41
a := "goodbye" // Standard lowercase a (Unicode U+0061)
fmt.Println(a) // Output: hello
fmt.Println(a) // Output: goodbye
Practical Programs to Understand Data Types and Conversions
I implemented several programs to reinforce my understanding:
Program 1: Integer to Float Conversion
This program declares an integer i
and converts it to a floating-point variable f
.
var i int = 20
var f float64 = float64(i) // Explicit type conversion
fmt.Println(i, f) // Output: 20 20.0
const value = 10
i = value
f = value
fmt.Println(i, f) // Output: 10 10.0
var b byte = math.MaxUint8
var smallI int32 = math.MaxInt32
var bigI uint64 = math.MaxInt64
fmt.Printf("%d\n%d\n%d\n", b, smallI, bigI)
// Output: 255, 2147483647, 9223372036854775807
Conclusion and Next Steps
Today’s focus was on understanding how Go handles different data types, type conversion, and constants. By practicing these concepts, I’m becoming more comfortable writing efficient Go programs. The next step is learning about composite types like arrays, slices, maps, and structs in Go. These types allows to handle collections of values and organize data more efficiently. Once comfortable with them, I be able to write more complex and powerful programs
Subscribe to my newsletter
Read articles from Sahal Imran directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
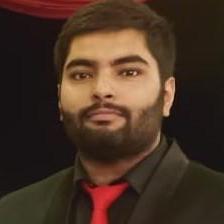