Mastering Hash Tables: The Key to Fast Data Retrieval
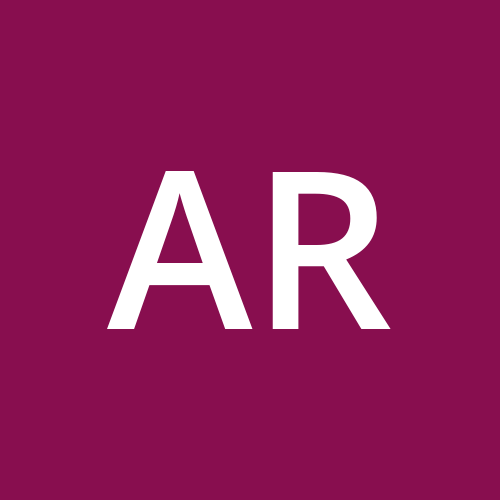
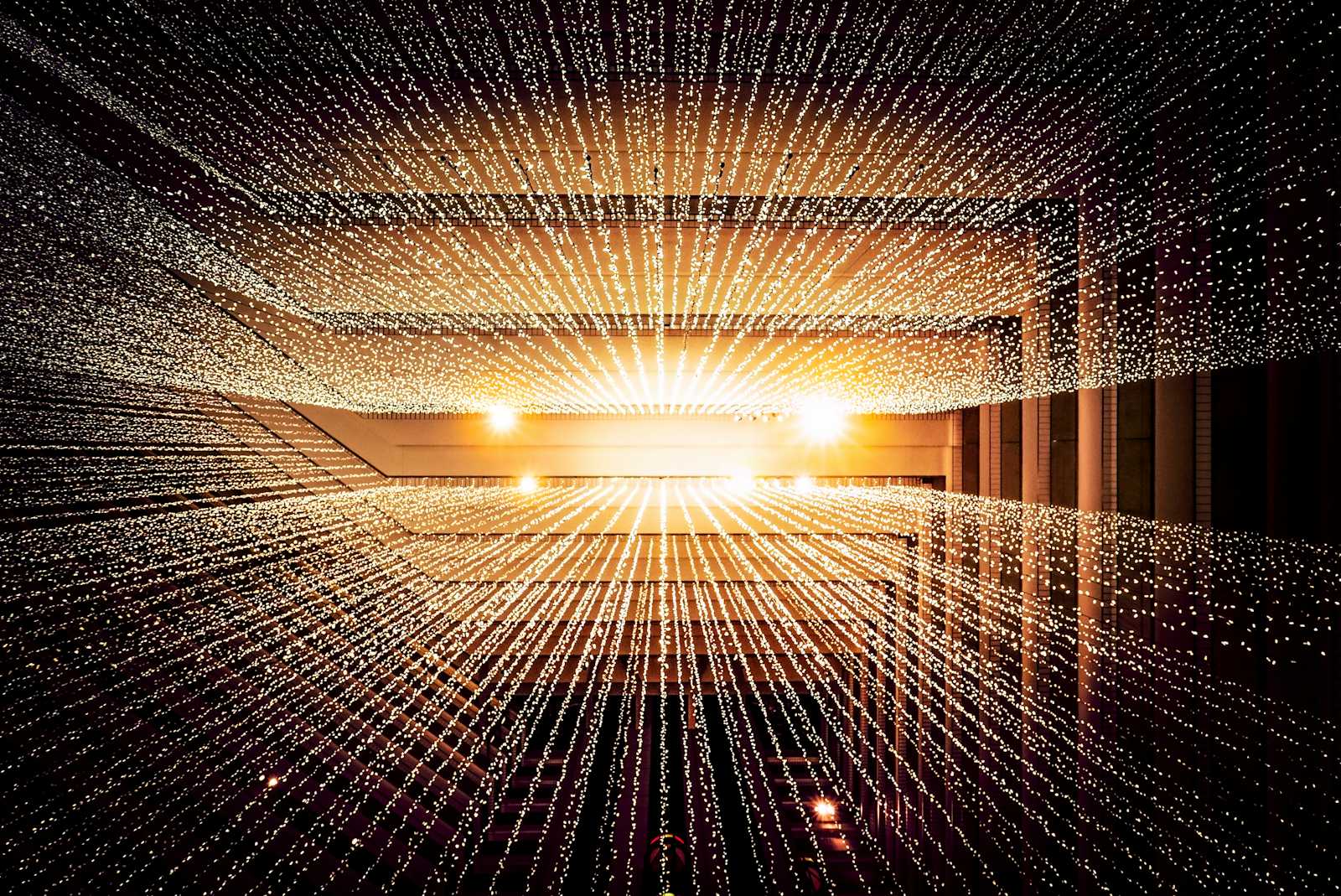
Introduction
In programming, efficiency is key, and one of the most powerful tools to achieve fast data access is a hash table. Hash tables are a fundamental data structure that allows us to store and retrieve data in constant time (O(1)) on average. Whether you’re building a caching system, handling user sessions, or counting the frequency of elements, hash tables are a go-to solution for many developers. In this post, we'll dive deep into what hash tables are, how they work, and why they're such a popular choice in computer science.
What is a Hash Table?
A hash table is a data structure that stores data in key-value pairs, allowing for quick and efficient retrieval. Think of it like a dictionary, where each word (the key) is associated with a definition (the value). Similarly, in a hash table, you can access a specific value by using its corresponding key, without the need to search through all the data, making lookups incredibly fast.
Imagine it as a phone book: instead of flipping through every name, you can jump directly to a person’s phone number by knowing their name (the key). This direct access is made possible by a hash function that converts the key into a specific index in the table.
In a hash table, keys must be unique. If you insert a new value with an existing key, the old value will be overwritten, ensuring that each key is always linked to only one value.
How Do Hash Tables Work?
The magic behind hash tables lies in their hash function. A hash function takes a key and converts it into an integer, which is then used as an index in an array where the associated value is stored. This allows for instant lookups, as the hash function directly maps the key to a specific location in the array, avoiding the need to search through the entire list of items.
Here’s a simplified step-by-step breakdown of how it works:
Provide a key: You input a key (like a string or number) that will be used to store or retrieve a value.
Hash the key: The hash function takes the key and converts it into a unique integer (called a "hash").
Store the value: The hash table uses this integer as an index in its internal array and stores the associated value at that index.
Retrieve the value: When you want to access the value, you provide the same key. The hash function generates the same integer, and the hash table instantly retrieves the value stored at that index.
Hash Functions
A hash function is the core component that powers a hash table. It takes a key and converts it into an integer, known as a "hash." This integer determines where the key-value pair will be stored in the array. A well-designed hash function ensures that keys are distributed evenly across the array, minimizing the chances of collisions (which we'll cover shortly).
Key Characteristics of a Good Hash Function:
Deterministic: The hash function should always produce the same hash for the same input. This ensures consistency when storing and retrieving data.
Efficient: The hash function must compute the hash quickly, even for large datasets, so it doesn't slow down the overall performance of the hash table.
Minimizes Collisions: A good hash function distributes keys in a way that minimizes the chance of different keys generating the same hash, which reduces collisions and improves lookup efficiency.
Example of Hash Function
function hash(key, arraySize) {
let hash = 0;
for (let i = 0; i < key.length; i++) {
hash = (hash + (key.charCodeAt(i) * (i + 1))) % arraySize; // Multiply by position to reduce collisions
}
return hash;
}
This hash function generates a hash by calculating the ASCII value of each character in the key and multiplying it by its position (index + 1). This weighting gives more significance to characters later in the string, helping differentiate similar keys, such as "apple"
and "apples"
. The resulting sum is then passed through the modulus operator (% arraySize
) to ensure the hash value stays within the bounds of the hash table’s size, preventing out-of-bounds indexing.
Tips for Crafting Good Hash Functions
Avoid simplicity that leads to clustering: Simple hash functions can result in key clustering, where multiple keys map to the same area in the array. This increases the likelihood of collisions. To avoid this, use more sophisticated logic, like multiplying by character positions or using prime numbers, to ensure a better distribution of values across the entire array.
Ensure uniqueness for similar keys: A well-designed hash function ensures that similar keys (like
"apple"
and"apples"
) produce different hash values. This minimizes the risk of collisions by spreading keys across different indices, even when their strings are similar.
Handling Collisions
In a perfect world, every key would map to a unique index in the hash table. However, collisions occur when two keys produce the same hash and, therefore, the same index. Fortunately, there are strategies to handle collisions:
Chaining
With chaining, each spot in the hash table doesn't just hold a single item but points to a list (or similar structure) where multiple items can be stored. So, if two different keys end up at the same spot (a collision), the new item is just added to the list at that spot.
Open Addressing
Open addressing doesn’t use lists. Instead, when a collision happens, it looks for the next available spot in the hash table itself. There are a few ways to do this:
Linear Probing: It checks the next spot in the table.
Quadratic Probing: It jumps by increasing intervals, like checking the next spot, then skipping ahead by 4 spots, then 9, and so on.
Double Hashing: It uses another hash function to find a new spot.
Note: In this post, I’m not covering these techniques in detail, but it’s helpful to know that different methods exist to handle collisions in a hash table.
Time Complexity of Hash Table Operations
One of the main reasons hash tables are so efficient is due to their average-case time complexity for common operations:
Insertion: O(1) on average. You hash the key and insert the value at the calculated index.
Search: O(1) on average. The key is hashed, and the corresponding value is accessed directly from the array.
Deletion: O(1) on average. The key is hashed, and the value is removed from the calculated index in constant time.
However, in the worst case, such as when many collisions occur or when all keys hash to the same index, these operations can degrade to O(n). But with a well-designed hash function and effective collision-handling strategies like chaining or open addressing, these worst-case scenarios are rare in practice.
Why is it instant?
In JavaScript, objects store data as key-value pairs, making them a fundamental data structure. Internally, JavaScript engines often implement objects using hash tables, which use arrays to store values at specific indices. This allows for instant lookups, as the hash function computes an index and the hash table can directly access that location in the array, rather than looping through all items. This is why hash tables—and by extension, objects—are so efficient for fast data retrieval.
It’s important to note that while arrays and objects are both core data structures in JavaScript, they serve different purposes. Arrays are optimized for ordered, sequential data with numeric indices, while objects are meant for key-value pairs, where keys can be strings, symbols, or numbers. The internal use of arrays in objects is an implementation detail that optimizes performance, but objects and arrays remain distinct in how they are used.
Practical Examples of Hash Tables
Hash tables are widely used in various real-world applications due to their efficiency in storing and retrieving data. Let's look at a few common scenarios where hash tables are invaluable:
Caching in Web Development: Hash tables are frequently used in caching systems to store frequently accessed data. For example, when a web server retrieves data from a database, it can store the result in a hash table. The next time the same data is requested, the server can return the cached result instantly, avoiding slow database queries and speeding up response times.
Storing User Sessions: In websites or applications with user authentication, a hash table can be used to store session data. Each user's session ID serves as the key, and the session details are stored as the value. This allows the server to quickly look up and manage session information for multiple users without having to search through all session records.
Counting the Frequency of Elements: Hash tables are ideal for counting how often certain elements appear in a collection. For example, when analyzing a text document, each word can be stored as a key, and its count (frequency) as the value. As you process the document, you simply increment the value in the hash table each time the word is encountered, making it an efficient solution for word frequency analysis.
Example in JavaScript
class HashTable {
constructor(size) {
this.table = new Array(size);
}
hash(key) {
let hash = 0;
for (let i = 0; i < key.length; i++) {
hash = (hash + (key.charCodeAt(i) * (i + 1))) % this.table.length;
}
return hash;
}
set(key, value) {
const index = this.hash(key); // 1. Hash the key to get the index.
if (!this.table[index]) { // 2. If no bucket (list) exists at that index,
this.table[index] = []; // create an empty array (bucket) for that index.
}
this.table[index].push([key, value]); // 3. Add the key-value pair to the bucket (array).
}
get(key) {
const index = this.hash(key);
const currentBucket = this.table[index];
if (currentBucket) {
for (let i = 0; i < currentBucket.length; i++) {
if (currentBucket[i][0] === key) {
return currentBucket[i][1];
}
}
}
return undefined;
}
}
// Example usage
const hashTable = new HashTable(50);
hashTable.set('apple', 10);
hashTable.set('banana', 20);
console.log(hashTable.get('apple')); // Output: 10
console.log(hashTable.get('banana')); // Output: 20
Array Size for the Hash Table
In a hash table, the initial size of the underlying array is typically set based on an estimate of how much data you plan to store. Since it’s impossible to predict the exact size needed upfront, most hash table implementations start with a default array size—commonly a power of two, such as 8 or 16—and adjust dynamically as the table grows. This strategy helps balance memory efficiency and performance, starting with a small array and expanding only when necessary.
A crucial concept in this resizing process is the load factor. The load factor represents the ratio of the number of elements in the hash table to the size of the array. For example, a load factor of 0.7 means that when the table is 70% full, the hash table will trigger a resize. When the number of elements exceeds the threshold (e.g., more than 7 elements in an array of size 10), the hash table doubles its size—for instance, from 10 to 20.
After resizing, the hash table must rehash all existing keys. This step is necessary because the hash function depends on the size of the array, and a larger array means new index positions for each key. Rehashing redistributes the elements across the newly resized array, ensuring an even spread and minimizing collisions.
Limitations of Hash Tables
While hash tables are a powerful and efficient data structure, they come with a few limitations:
Memory Usage: Hash tables can consume more memory than other data structures, as they often require large arrays to minimize collisions. This extra space, especially when the load factor is kept low, can lead to higher memory overhead.
Lack of Order: Hash tables do not preserve any particular order for the stored elements. If you need to iterate through data in a specific order (like sorted keys), a hash table may not be the ideal choice, as elements are stored based on their hash value, not their original order.
Collisions: Poorly designed hash functions can lead to excessive collisions, where multiple keys map to the same index. If collisions occur frequently, the hash table's performance can degrade from O(1) (ideal case) to O(n) (worst case) for search and insert operations, as it may need to traverse lists or chains of values at the same index.
Conclusion
Hash tables are a powerful and essential data structure in computer science, known for their ability to deliver fast and efficient data access. By mastering how they work, understanding collision handling techniques, and considering their time complexity, you can confidently leverage hash tables in various applications—whether it’s for caching, session management, or frequency counting. Their flexibility and speed make them an indispensable tool in your programming toolkit.
If you have any further questions or would like to dive deeper into how hash tables work, feel free to connect with me on LinkedIn: Artur Navolovskyi. I'm always happy to help and chat about all things tech!
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
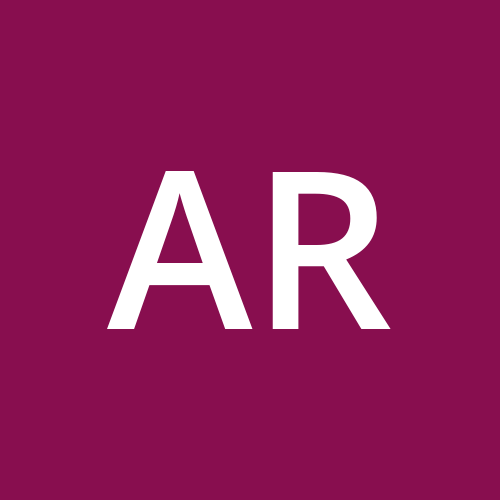