Exploring Deno: A Modern Runtime for JavaScript and TypeScript with Effortless Deployment
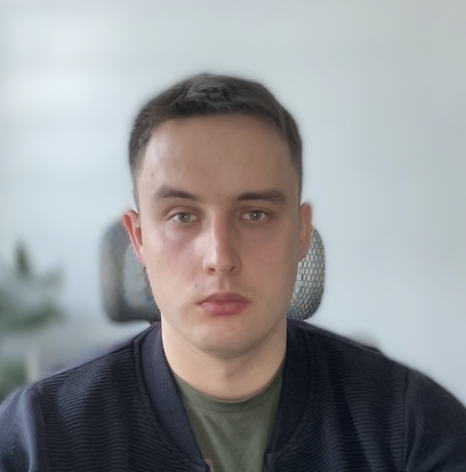
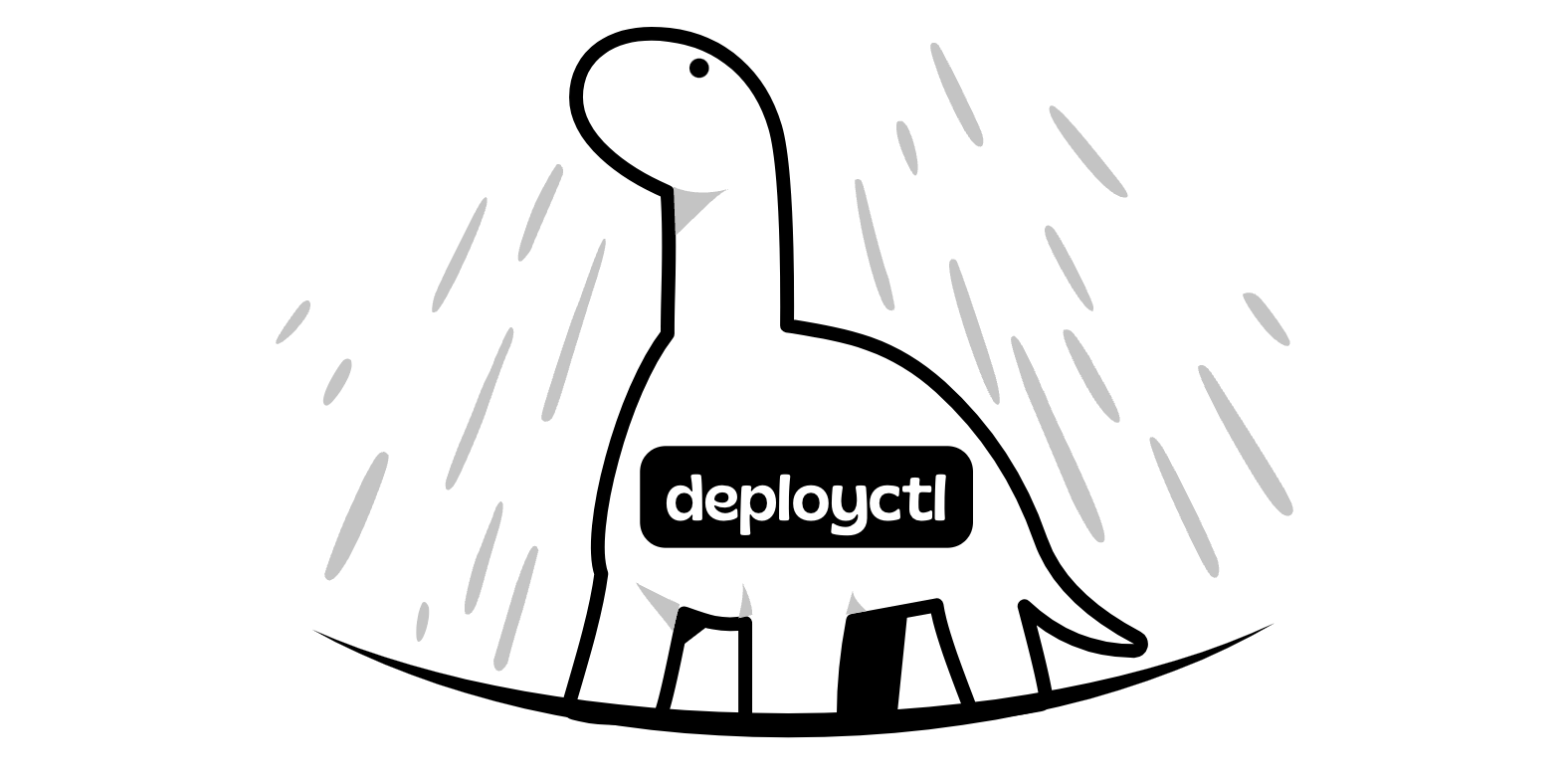
One of the things that truly impressed me was its deployment tool. 🛠️ With just one command, the server was up and running! 🎉 No extra configurations, complex setups, or long checklists required. 📋 ❌ In this article, I'll share my experience with Deno, provide an in-depth look at what it is, how to set it up, and why you might want to consider using it.
What is Deno?
Deno is a modern, secure runtime for JavaScript and TypeScript that was built by the creator of Node.js, Ryan Dahl. It's designed to fix some of the shortcomings and design mistakes of Node.js by introducing a fresh approach to server-side JavaScript development. Key features of Deno include:
Built-in TypeScript support: Unlike Node.js, Deno has first-class TypeScript support without needing additional tooling.
Secure by default: Access to the file system, network, and environment must be explicitly enabled.
Single executable: There's no need to install a package manager like
npm
– Deno can import modules directly from URLs.Simplified standard library: Deno provides a built-in standard library for common tasks.
Out the box: testing, linting, formatting, deploy.
Installing Deno
To start using Deno, you need to install it on your machine. The installation process is straightforward, and Deno can be installed using various package managers:
1. Using Shell (Unix-like systems):
curl -fsSL https://deno.land/install.sh | sh
2. Windows:
irm https://deno.land/install.ps1 | iex
After installation, you can check if Deno is installed correctly by running:
deno --version
You should see output indicating the current version of Deno, TypeScript, and V8.
Basic Usage of Deno
Here’s a simple example to demonstrate how easy it is to create and deploy a server using Deno.
Creating a Basic HTTP Server
Let’s start by creating a basic HTTP server. This code listens on port 8000 and responds with "Hello, Deno!" to any incoming requests:
Deno.serve(() => new Response("Hello, world!"));
To run this script, use:
deno run --allow-net server.ts
The --allow-net
flag grants permission to access the network, reflecting Deno’s secure-by-default philosophy.
Deploying with Deno Deploy
Deploying a Deno app is extremely easy thanks to Deno Deploy. Here’s how to deploy the above server:
Install Deployctl:
If you haven't already, install
deployctl
, a CLI tool for Deno Deploy:deno install -A jsr:@deno/deployctl
Deploy the server:
With the code ready, you can deploy it using just one command:
deployctl deploy
And that's it! Your server is now live on Deno Deploy, accessible via a unique URL.
More Examples with Deno
Using TypeScript with Deno
Deno has built-in support for TypeScript, so you don’t need any extra configuration. Here’s how you can create a simple script using TypeScript:
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet("Deno"));
Run the script with:
deno run greet.ts3
Deno’s Standard Library
Deno has a standard library that provides utilities for common tasks, such as:
File System Operations:
import { readFileStr } from "https://deno.land/std/fs/mod.ts"; const text = await readFileStr("./example.txt"); console.log(text);
Testing:
import { assertEquals } from "https://deno.land/std/testing/asserts.ts"; Deno.test("simple test", () => { assertEquals(1 + 1, 2); });
Conclusion
Deno is a promising new runtime that combines the best aspects of JavaScript, TypeScript, and modern development practices. It simplifies many aspects of the development workflow while offering enhanced security features and better integration with TypeScript. If you’re looking for a modern approach to server-side JavaScript, Deno is definitely worth trying.
Give it a shot and see how it can improve your development experience! 🔍✨
Subscribe to my newsletter
Read articles from Dmytro Sapozhnyk directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
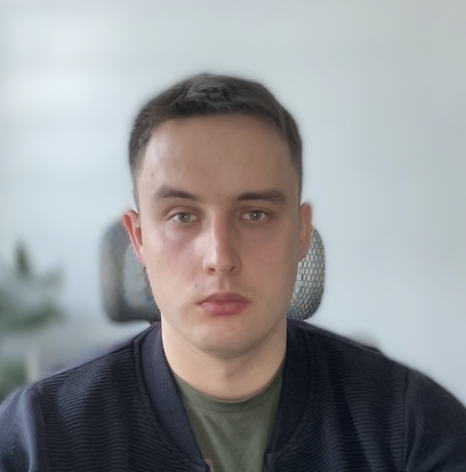
Dmytro Sapozhnyk
Dmytro Sapozhnyk
I have known in different languages (Python, C/C++, basic knows in Java, Solidity), but work with JavaScript. My stack is React.js, Node.js etc...