Advanced MySQL Topics

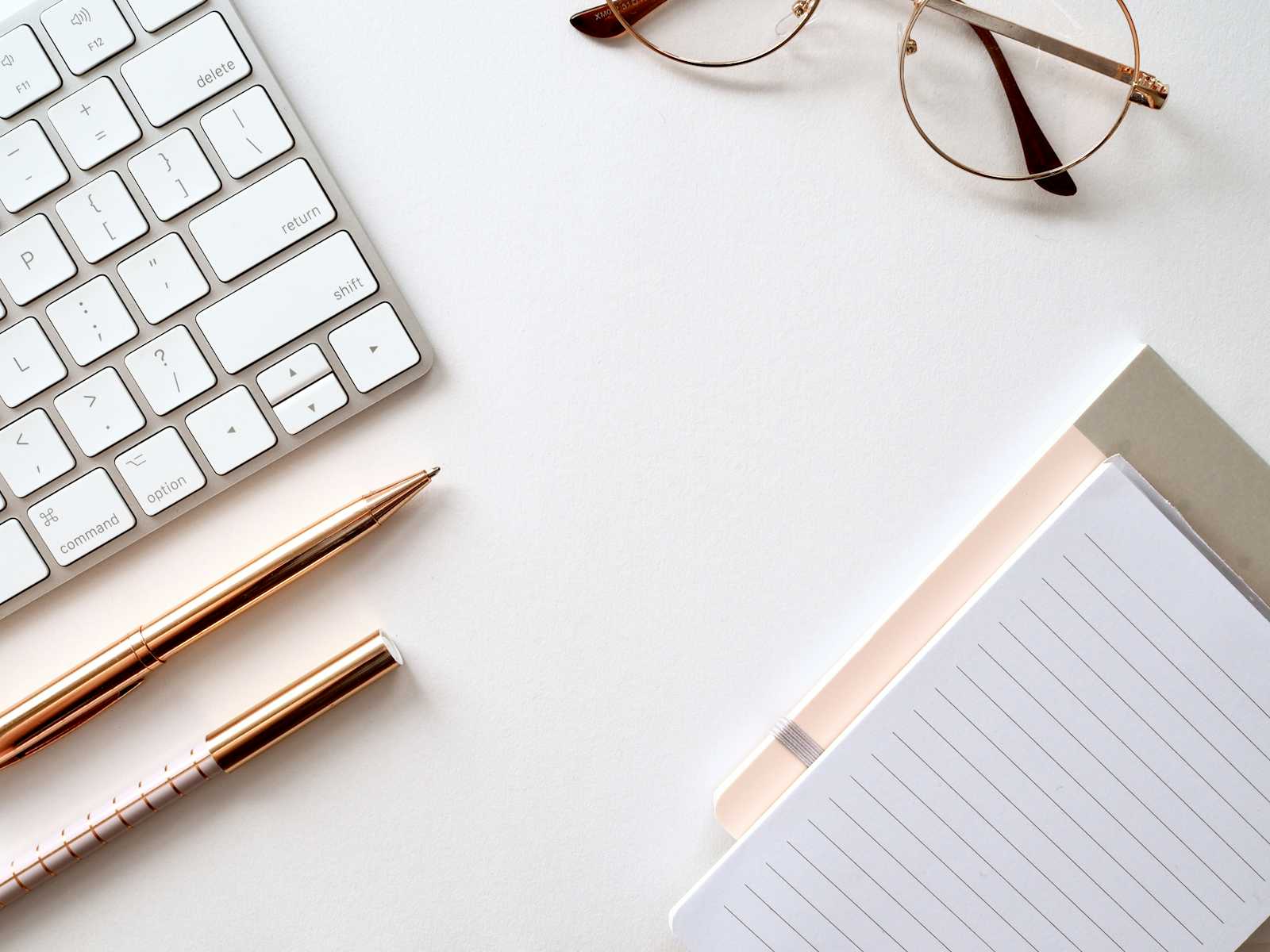
1. Introduction
MySQL is a powerful relational database management system widely used in web applications, data warehousing, and enterprise solutions. This document covers advanced topics that every experienced DBA should master to optimize performance, ensure data integrity, and maintain security.
2. Indexing Techniques
Types of Indexes
Indexes improve query performance by allowing the database engine to find rows faster. The main types of indexes in MySQL include:
B-Tree Indexes: The default index type. Suitable for a wide range of queries.
CREATE INDEX idx_name ON employees(name);
Hash Indexes: Used primarily with the MEMORY storage engine, providing fast equality comparisons but not range queries.
CREATE INDEX idx_hash ON orders USING HASH(order_id);
Full-Text Indexes
Full-text indexes are used for complex queries on text columns, allowing for natural language searches.
ALTER TABLE articles ADD FULLTEXT(title, content);
SELECT * FROM articles WHERE MATCH(title, content) AGAINST('MySQL');
Composite Indexes
Composite indexes are useful for queries filtering on multiple columns.
CREATE INDEX idx_composite ON sales(customer_id, sale_date);
3. Query Optimization
Analyzing Queries
Understanding how queries interact with the database is crucial for optimization.
EXPLAIN Statement
The EXPLAIN
statement provides insights into how MySQL executes a query.
EXPLAIN SELECT * FROM employees WHERE department_id = 3;
Query Rewriting
Sometimes rewriting queries can significantly improve performance.
-- Original query
SELECT * FROM orders WHERE customer_id = 1 AND status = 'shipped';
-- Rewritten using a JOIN
SELECT o.* FROM orders o JOIN customers c ON o.customer_id = c.id WHERE c.id = 1 AND o.status = 'shipped';
4. Partitioning
Partitioning allows you to split large tables into smaller, more manageable pieces.
Types of Partitioning
Range Partitioning: Data is distributed based on a range of values.
CREATE TABLE sales ( sale_id INT, sale_date DATE, amount DECIMAL(10, 2) ) PARTITION BY RANGE (YEAR(sale_date)) ( PARTITION p0 VALUES LESS THAN (2020), PARTITION p1 VALUES LESS THAN (2021), PARTITION p2 VALUES LESS THAN (2022) );
List Partitioning: Based on a predefined list of values.
Benefits and Use Cases
Partitioning improves query performance and simplifies data management, especially for large datasets.
5. Replication
Replication allows data from one MySQL server (master) to be copied to one or more MySQL servers (slaves).
Master-Slave Replication
A common setup for load balancing and data redundancy.
-- On the master server
SHOW MASTER STATUS;
-- On the slave server
CHANGE MASTER TO
MASTER_HOST='master_host',
MASTER_USER='replication_user',
MASTER_PASSWORD='password',
MASTER_LOG_FILE='recorded_log_file',
MASTER_LOG_POS=recorded_log_position;
START SLAVE;
Multi-source Replication
Allows a single slave to replicate from multiple masters.
GTID-Based Replication
Global Transaction Identifiers (GTIDs) help track transactions across servers, simplifying failover and recovery.
6. Clustering
MySQL Cluster Overview
MySQL Cluster is designed for high availability and high throughput.
NDB Storage Engine
NDB provides data storage across multiple nodes, enabling horizontal scalability.
CREATE TABLE cluster_table (
id INT NOT NULL,
value VARCHAR(100),
PRIMARY KEY (id)
) ENGINE=NDB;
7. Stored Procedures and Functions
Stored procedures and functions allow you to encapsulate complex logic on the database side.
Creating Procedures and Functions
DELIMITER //
CREATE PROCEDURE GetEmployeeCount(IN dept_id INT, OUT emp_count INT)
BEGIN
SELECT COUNT(*) INTO emp_count FROM employees WHERE department_id = dept_id;
END //
DELIMITER ;
Error Handling
You can manage errors using the DECLARE
statement.
DECLARE EXIT HANDLER FOR SQLEXCEPTION
BEGIN
-- error handling code
END;
8. Triggers
Triggers are automated actions executed in response to certain events on a table.
Creating and Managing Triggers
CREATE TRIGGER before_insert_employee
BEFORE INSERT ON employees
FOR EACH ROW
BEGIN
SET NEW.created_at = NOW();
END;
Use Cases
Triggers are useful for auditing changes or enforcing business rules.
9. Security Best Practices
User Management
Always follow the principle of least privilege when granting user permissions.
CREATE USER 'new_user'@'localhost' IDENTIFIED BY 'password';
GRANT SELECT, INSERT ON database_name.* TO 'new_user'@'localhost';
Secure Connections
Enable SSL to encrypt connections.
-- Enable SSL in my.cnf
[mysqld]
require_secure_transport=ON
10. Performance Tuning
Configuration Parameters
Tune MySQL performance by adjusting parameters in the my.cnf
configuration file.
[mysqld]
innodb_buffer_pool_size=1G
query_cache_size=128M
Monitoring Performance
Use tools like SHOW STATUS
and performance_schema for monitoring.
SHOW GLOBAL STATUS LIKE 'Threads_connected';
11. Conclusion
Mastering these advanced MySQL topics will equip you with the tools necessary for efficient database management and optimization. Continuous learning and adaptation to new features are key to maintaining a robust MySQL environment.
Subscribe to my newsletter
Read articles from Data & Dev directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Data & Dev
Data & Dev
Data & Dev: Unveiling the Wonders of AI- ML, Data, and Dev. With over 15+ years of experience in AI- ML, Cloud computing and data integration. We specialize in helping businesses optimize their Data with AI and ML for maximum efficiency and scalability. My expertise spans across various cloud platforms including AWS, Azure, and Google Cloud, as well as database technologies like Python, Docker, Kube, SQL, NoSQL, and data warehousing solutions.