Task Tree and Task Cancellation in Swift Concurrency: Key Concepts Explained
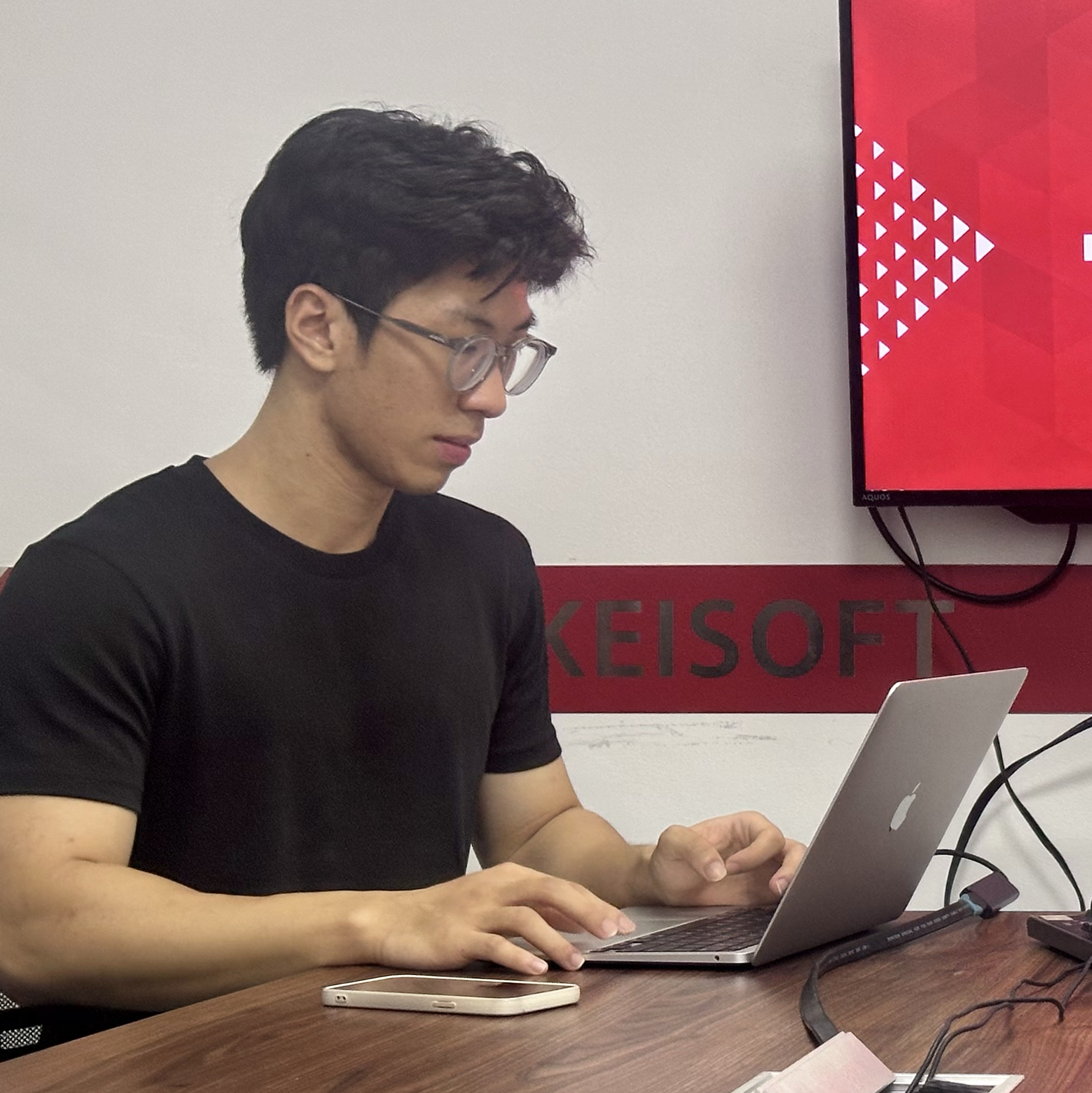
Table of contents
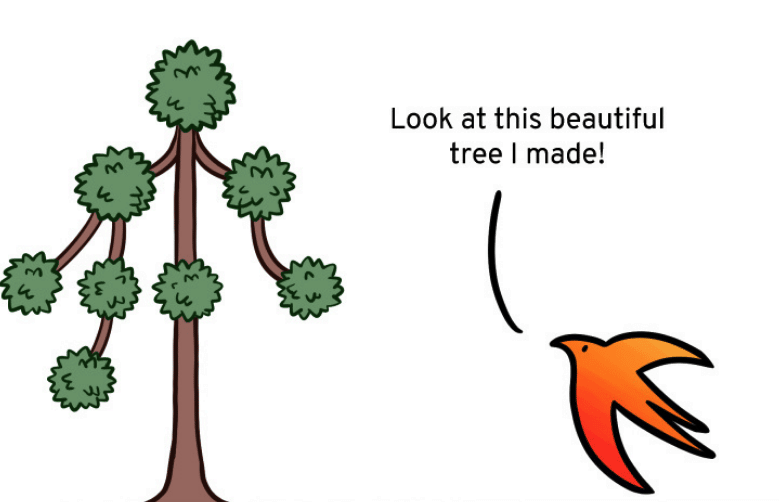
Task Tree
Let’s take a look at the code below
As you can see, the function printStudentInfo()
has 2 child Tasks defined using async let. Inside the child Task getClassesAndScores()
, it also has 2 child Tasks getClasses()
and getScores()
. So we have a Task tree with 3 levels here:
Although there are 5 Tasks in the parent-child relationship here, they all run simultaneously. Because the Tasks have parent-child relationships, they call this approach Structured Concurrency.
The explicit parent-child relationships between tasks have several advantages:
The parent task is complete when all of its child tasks are complete.
When setting a higher priority on a child task, the parent task’s priority is automatically escalated.
When a parent task is canceled, each of its child tasks is also automatically canceled.
When a child task throws an error, the error propagates to the parent task
Task Cancellation
What happens to other tasks when a Task is canceled?
If a Task is canceled, the cancellation state cascades down from the parent to all its children. This is called cooperative cancellation. Tasks continue their work when canceled and don’t return anything. So it’s important that we should stop running canceled Tasks, we can check this canceled state in our code and terminate processing.
There are two ways a task can do this:
- by calling the Task.checkCancellation() type method
Calling Task.checkCancellation()
throws an error if the task is canceled, a throwing task can propagate the error out of the task, stopping all of the task’s work. This has the advantage of being simple to implement and understand
- reading the Task.isCancelled type property
For more flexibility, use the Task.isCancelled
property, which lets you perform clean-up work as part of stopping the task, like closing network connections and deleting temporary files.
Thanks for Reading! ✌️
If you have any questions or corrections, please leave a comment below or contact me via my LinkedIn account Pham Trung Huy.
Happy coding 🍻
Subscribe to my newsletter
Read articles from Phạm Trung Huy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
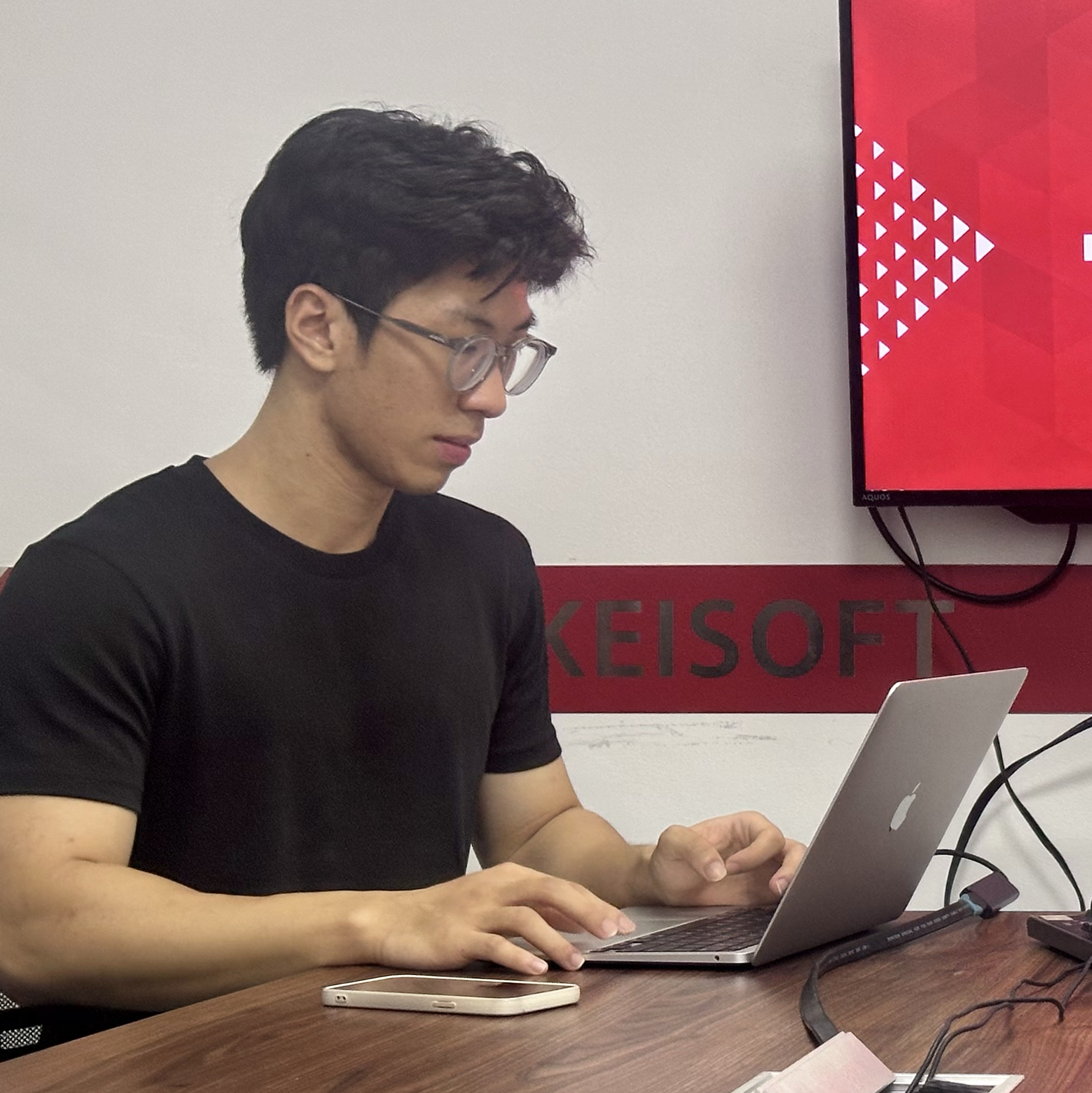
Phạm Trung Huy
Phạm Trung Huy
👋 I am a Mobile Developer based in Vietnam. My passion lies in continuously pushing the boundaries of my skills in this dynamic field.