` Backticks ` in Go Lang
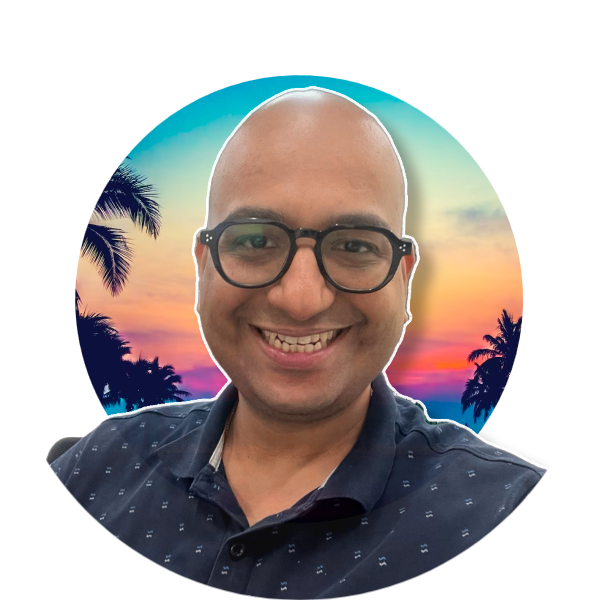
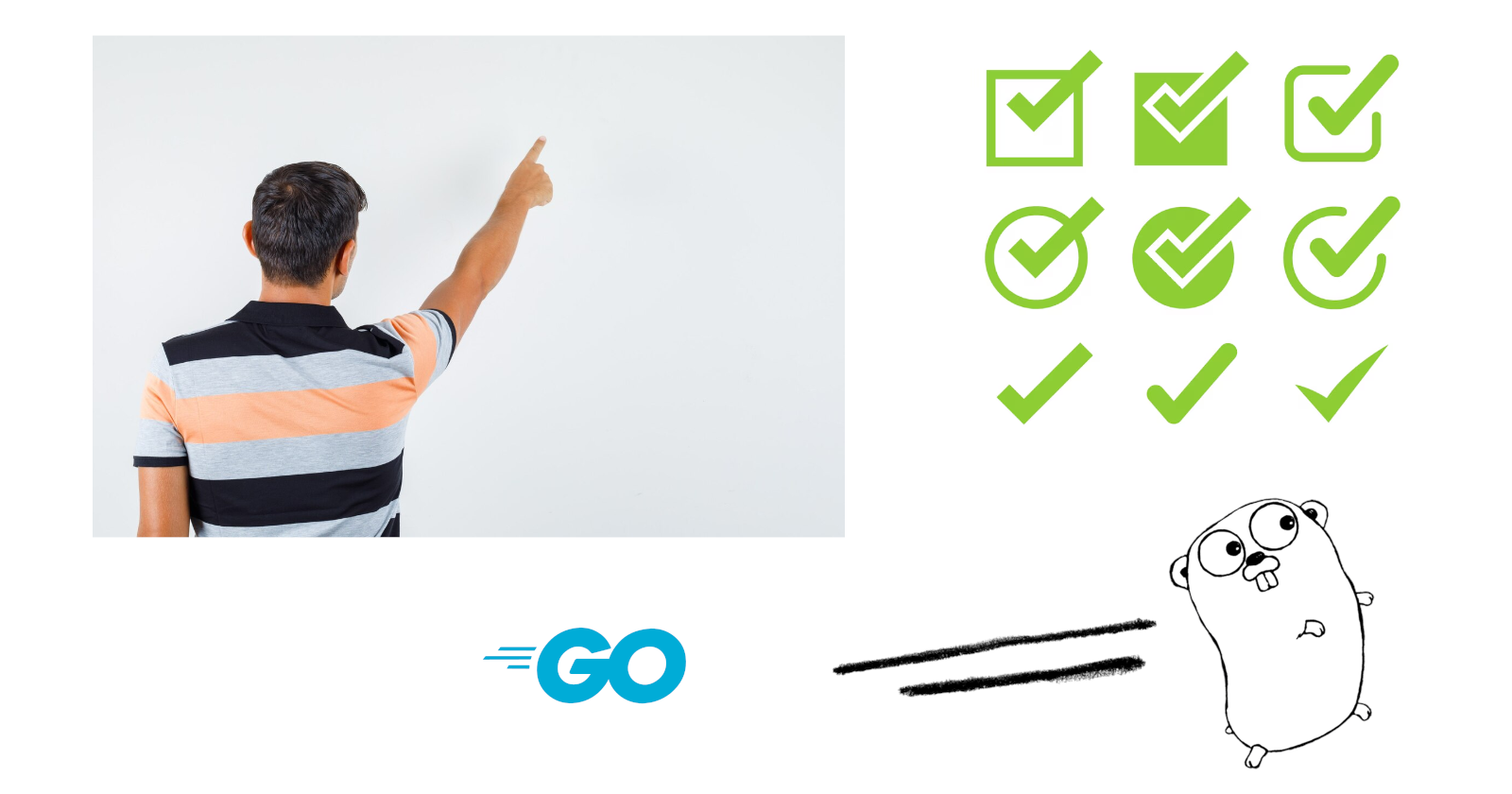
In Go, backtick tags are used in struct definitions to define metadata, typically for serialization or data validation. These tags are most commonly used with libraries like encoding/json
, encoding/xml
, or for other custom use cases. The backtick notation allows you to annotate struct fields with string-based metadata.
Here’s an example:
type Person struct {
Name string `json:"name"`
Age int `json:"age"`
Email string `json:"email,omitempty"`
}
Explanation:
The backticks enclose the struct field tags.
json:"name"
tells the JSON encoder/decoder to map theName
field to/from the"name"
key in a JSON object.json:"email,omitempty"
means theEmail
field is mapped to the"email"
key, but will be omitted from the output if it is empty (""
for strings,0
for numbers,nil
for pointers, etc.).
Other libraries may use similar mechanisms to interact with struct fields based on these tags.
Is this Meta-programming?
While Go struct tags resemble metadata, they are not considered full-fledged metaprogramming in the traditional sense. Go doesn't support dynamic code generation, reflection-based type alterations, or macros as seen in languages like Python or Lisp. However, Go does offer reflection through the reflect
package, which allows inspecting types and values at runtime, and struct tags play a role in this.
In Go, struct tags are more like static metadata annotations. They're mainly used in tandem with reflection for libraries like json
, xml
, or ORM libraries, but they don't alter the behavior of the program at compile time (like traditional metaprogramming might).
How it works:
- Struct tags are stored as part of the field’s definition and can be accessed at runtime via the
reflect
package. For instance, libraries read these tags to determine how to serialize or deserialize struct fields.
Example of accessing tags using reflection:
package main
import (
"fmt"
"reflect"
)
type Person struct {
Name string `json:"name"`
Email string `json:"email,omitempty"`
}
func main() {
personType := reflect.TypeOf(Person{})
field, _ := personType.FieldByName("Name")
fmt.Println(field.Tag.Get("json")) // Output: name
}
This allows libraries to interpret and utilize struct tags but without changing the structure or logic of the program dynamically at runtime.
Conclusion:
This tag system makes it possible to control how data is marshaled and un-marshaled between Go structs and external data formats. While Go struct tags are reflective metadata that can be introspected at runtime, they don't fall under true metaprogramming as Go lacks dynamic code modification capabilities. Instead, Go focuses on simplicity and explicitness, using tools like reflection sparingly to provide extensibility.
Image attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
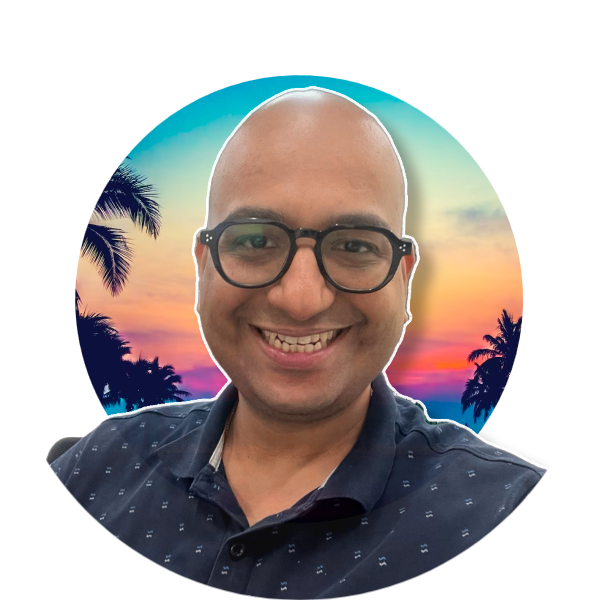
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.