Getting Started with Frontend Technology: A Beginner’s Guide

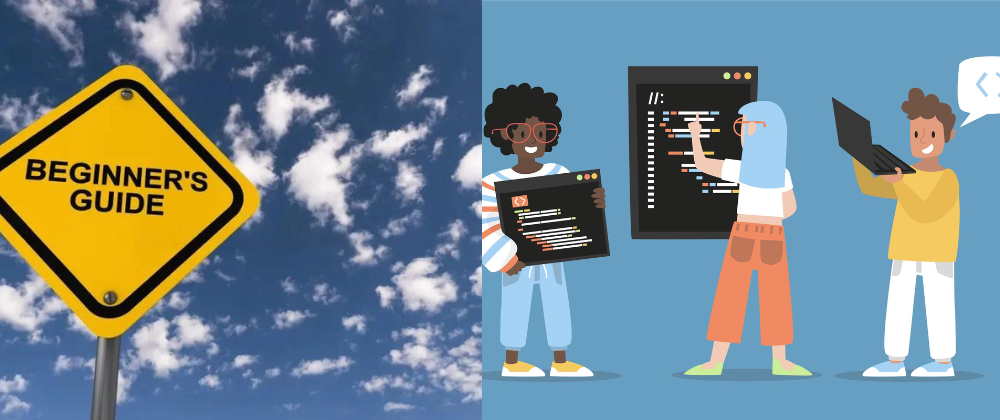
As the face of modern web development, frontend technology is a vital skill for anyone looking to dive into programming. Frontend development is all about creating the part of websites and web applications that users interact with directly. From the layout of content to the colors, fonts, and interactive elements, frontend development combines creativity and technical know-how. This blog will guide you through the essential tools and steps to get started in frontend technology.
1. Understanding Frontend Development
Frontend development refers to the implementation of the visual and interactive elements of a website. While the backend (server-side) manages data and logic, the frontend (client-side) focuses on user experience and design. Frontend developers work with three main building blocks:
HTML (HyperText Markup Language): The skeleton of a webpage. It structures the content and forms the foundation of all websites.
CSS (Cascading Style Sheets): CSS adds style to HTML elements, controlling the design aspects such as colors, fonts, and layouts.
JavaScript (JS): JavaScript makes the website interactive. It allows you to add dynamic behaviors, such as sliders, animations, form validations, and more.
2. Setting Up Your Development Environment
Before writing code, you need a proper environment to develop and test your websites. Here are the basic tools you’ll need:
Code Editor: A code editor is where you’ll write your HTML, CSS, and JavaScript. Popular editors include Visual Studio Code, Sublime Text, or Atom. They offer features like syntax highlighting, code suggestions, and debugging tools.
Web Browser: You’ll need a browser to see your work in action. Google Chrome, Firefox, and Safari are popular choices. You can use the browser’s developer tools (usually opened by pressing F12 or right-clicking and selecting “Inspect”) to view your code, debug, and test your designs across devices.
Version Control (Git): As your projects grow, version control becomes critical for managing code changes. Git, along with GitHub or GitLab, allows you to track changes, collaborate with others, and back up your projects.
3. Learning the Basics: HTML, CSS, and JavaScript
HTML: The Structure
HTML provides the structure and layout of your webpage. Here’s an example of basic HTML code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My First Webpage</title>
</head>
<body>
<h1>Hello World</h1>
<p>Welcome to my first webpage.</p>
</body>
</html>
In this code, the HTML elements like <h1>
(heading) and <p>
(paragraph) are used to define content.
CSS: The Design
CSS is what makes a website visually appealing. It allows you to apply styles like color, layout, and fonts. Here’s how CSS works with HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Styled Webpage</title>
<style>
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
color: #333;
}
h1 {
color: #0056b3;
}
</style>
</head>
<body>
<h1>Welcome to My Styled Page</h1>
<p>This page has a background color and custom font.</p>
</body>
</html>
CSS can be included in the same HTML file (as shown above), in the <style>
section, or in an external stylesheet to keep the code organized.
JavaScript: The Interactivity
JavaScript allows you to add functionality and interactivity to your site. It’s used for anything from simple button clicks to complex animations and dynamic content loading. Here’s a simple example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Interactive Webpage</title>
<style>
p { color: red; }
</style>
</head>
<body>
<h1>Click the button to change the color!</h1>
<p id="text">This is a red paragraph.</p>
<button onclick="changeColor()">Click Me</button>
<script>
function changeColor() {
document.getElementById('text').style.color = 'green';
}
</script>
</body>
</html>
When the button is clicked, the changeColor
function is triggered, changing the color of the text.
4. Explore Frontend Frameworks and Libraries
Once you are comfortable with the basics, you can explore popular frontend frameworks and libraries that simplify and speed up development:
Bootstrap: A CSS framework that offers pre-built responsive layouts and components, making it easier to design web pages quickly without writing custom CSS from scratch.
React: A JavaScript library developed by Facebook that allows you to build fast and dynamic user interfaces using components. It’s widely used for building single-page applications (SPAs).
Vue.js and Angular: Alternatives to React, these are also popular for creating dynamic, interactive web applications.
Frameworks help in organizing your code and make complex web apps easier to manage and maintain.
5. Building Your First Projects
The best way to solidify your understanding of frontend development is by building projects. Start small with static websites or landing pages and work your way up to interactive projects.
Here are some project ideas for beginners:
Personal Portfolio Website: A great project for showcasing your skills and a must-have for every developer.
To-Do List: This can help you practice JavaScript DOM manipulation.
Interactive Quiz: A fun way to dive deeper into form handling and JavaScript functionality.
6. Responsive Design: Mobile-Friendly Web Development
A crucial skill in frontend development is making sure your websites work well across different screen sizes. With mobile devices accounting for more than half of web traffic, responsive design is essential.
Learn about CSS Flexbox and CSS Grid to help create layouts that automatically adjust for different screen sizes. Additionally, CSS media queries allow you to apply specific styles depending on the device or screen size.
For example, to change the background color of a webpage for screens smaller than 600px, you could use:
@media (max-width: 600px) {
body {
background-color: lightblue;
}
}
7. Learn Version Control and Collaboration
As you grow as a developer, version control using Git becomes essential. Platforms like GitHub allow you to:
Track changes in your codebase.
Collaborate with other developers.
Share your projects with the world.
Learn basic Git commands such as git add
, git commit
, and git push
, and create a GitHub repository for each of your projects.
8. Staying Up-to-Date
Frontend technology evolves quickly. Stay up-to-date by:
Following web development blogs and tutorials.
Joining developer communities on platforms like Reddit, Stack Overflow, or GitHub.
Exploring new tools, techniques, and libraries.
Some good learning resources include free platforms like MDN Web Docs, freeCodeCamp, and W3Schools.
Conclusion
Starting with frontend technology may feel overwhelming at first, but by focusing on one step at a time—learning HTML, CSS, and JavaScript—you’ll gain the core skills necessary to create beautiful and functional websites. Remember to practice by building your own projects and keep up with the latest trends to grow as a frontend developer. With time and dedication, you'll master the tools and techniques that power the modern web. Happy coding!
Subscribe to my newsletter
Read articles from akash javali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

akash javali
akash javali
A passionate 'Web Developer' with a Master's degree in Electronics and Communication Engineering who chose passion as a career. I like to keep it simple. My goals are to focus on typography, and content and convey the message that you want to send. Well-organized person, problem solver, & currently a 'Senior Software Engineer' at an IT firm for the past few years. I enjoy traveling, watching TV series & movies, hitting the gym, or online gaming.