Why Ruby on Rails 8 is the Best Framework for Building MVPs: Speed, Scalability, and Simplicity

Table of contents
- Why Ruby on Rails 8 is Best for Building MVPs
- 1. Speed of Development: Convention Over Configuration
- 2. Hotwire for Real-Time Features Without JavaScript
- 3. Scalability and Performance from the Start
- 4. Large Ecosystem and Vibrant Community
- 5. Maintainability and Flexibility
- 6. Cost-Effective Development
- Conclusion: Ruby on Rails 8 is the Perfect Framework for MVPs
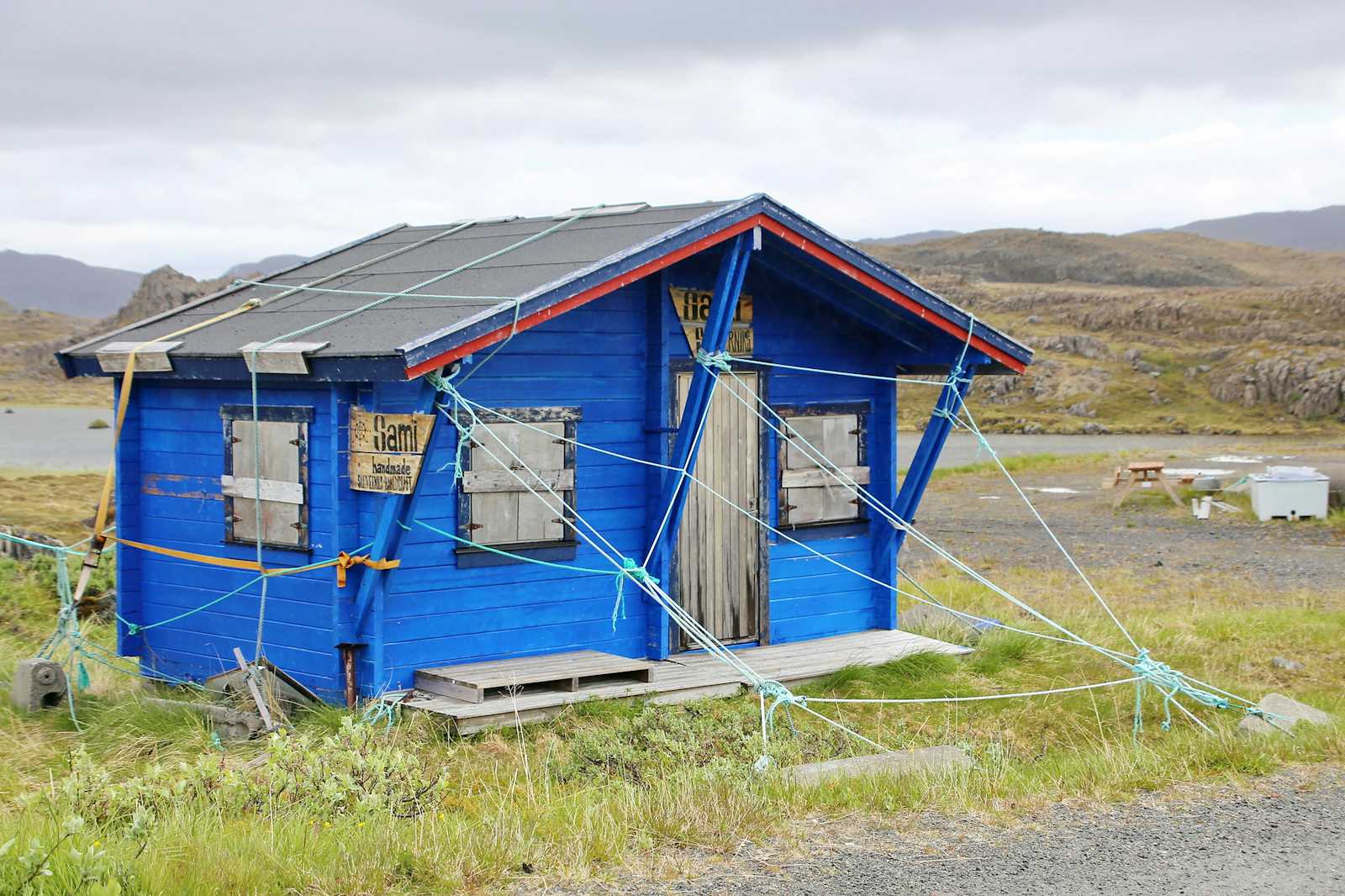
Why Ruby on Rails 8 is Best for Building MVPs
When it comes to building a Minimum Viable Product (MVP), selecting the right technology stack is crucial.
You need a framework that allows for rapid development, scalability, and simplicity—all while ensuring the product is maintainable and easy to extend as it grows.
Ruby on Rails 8 is one of the best choices for building MVPs, offering a range of features that make it the ideal framework for startups, entrepreneurs, and developers looking to launch quickly with minimal resources.
In this article, we’ll explore why Ruby on Rails 8 stands out as the top choice for MVP development, particularly focusing on its new features, ease of use, and ability to scale.
1. Speed of Development: Convention Over Configuration
Ruby on Rails has always been known for its speed of development, thanks to its "Convention over Configuration" philosophy. This concept reduces the amount of decision-making needed when setting up an application, as Rails provides sensible defaults for most aspects of the development process.
In Rails 8, this philosophy is more powerful than ever. With enhancements like SolidQueue, SolidCache, and SolidCable, Rails 8 eliminates the need for external dependencies like Redis, simplifying the infrastructure even further. This means developers can focus on building out core product features quickly, without worrying about setting up complex third-party services.
Key Speed Benefits:
Rapid prototyping: Get your MVP out in weeks rather than months.
Minimal configuration: Rails 8 comes with well-thought-out defaults, reducing the setup time.
Pre-built components: Features like user authentication, database handling, and background jobs are easy to implement with gems and built-in Rails features.
Code Example: Setting Up a New Rails 8 Project
Start a new Rails 8 project optimized for fast MVP development using SQLite:
rails new example --main
cd example
bundle install
rails db:create
This command creates a fully functional Rails app using SQLite, so you can focus on building features from the start without complex setup.
2. Hotwire for Real-Time Features Without JavaScript
One of the biggest challenges in MVP development is building interactive and real-time features, such as live updates or chats. Traditionally, this required complex JavaScript frameworks and external services like WebSockets.
However, with Hotwire, Rails 8 offers a JavaScript-free way to build real-time functionality right out of the box.
Hotwire, comprising Turbo and Stimulus, enables developers to create real-time features without writing any custom JavaScript code. This is a huge advantage for MVPs, as it cuts down on both development time and complexity.
You can now build dynamic, live-updating components like notifications, message feeds, or dashboards without needing to invest in a JavaScript-heavy frontend.
Key Hotwire Benefits:
Real-time updates: Build live features like notifications, comments, and chat without using JavaScript.
Less complexity: Rails developers can stay within the Ruby ecosystem, avoiding the need to manage a separate frontend stack.
Faster iteration: Because you don’t need to worry about frontend JS frameworks, you can make quick changes to the user interface and behavior directly from Rails.
Code Example: Adding Real-Time Notifications Using Hotwire
Add Hotwire to your Rails project by including the gem and installing it:
# Gemfile
gem 'hotwire-rails'
Then install Hotwire:
bundle install
rails hotwire:install
Now, implement real-time notifications using Turbo Streams:
# app/models/notification.rb
class Notification < ApplicationRecord
after_create_commit { broadcast_append_to "notifications" }
end
In the view, use Turbo Streams to dynamically display new notifications:
<!-- app/views/notifications/index.html.erb -->
<%= turbo_stream_from "notifications" %>
<%= render @notifications %>
<div id="notifications">
<%= turbo_frame_tag 'notifications' do %>
<!-- Real-time updates will appear here -->
<% end %>
</div>
With this setup, notifications will appear on the user's screen as soon as they're created, with no need for page refreshes.
3. Scalability and Performance from the Start
Rails 8 introduces features that make it easier than ever to scale an MVP into a full-fledged product.
Tools like SolidQueue (for background job processing) and SolidCache (for efficient caching) are designed to help your app handle increased traffic and complex workloads without requiring a complete re-architecture.
This ensures that your MVP is built to last, and can easily evolve into a production-ready app without the need to rewrite large portions of the codebase.
As your product grows and you need to handle more data, Rails 8's seamless database management and job processing allow you to scale smoothly.
Key Scalability Benefits:
Database-backed queuing: SolidQueue uses your existing database, reducing the need for external job-processing services like Sidekiq or Resque.
Built-in caching: SolidCache eliminates the need for Redis and offers scalable caching solutions from day one.
Real-time communication: SolidCable enables WebSocket communication without Redis, allowing your app to handle real-time user interactions as it scales.
Code Example: Using SolidQueue for Background Jobs
SolidQueue manages background jobs directly in your database, eliminating the need for external services like Redis or Sidekiq.
# app/jobs/send_welcome_email_job.rb
class SendWelcomeEmailJob < ApplicationJob
queue_as :default
def perform(user)
UserMailer.with(user: user).welcome_email.deliver_later
end
end
To configure SolidQueue, simply ensure your project uses the database for job management:
rails active_job:solid_queue
SolidQueue uses the same database as your app, meaning you don’t need additional services for job management.
Code Example: Implementing Caching with SolidCache
SolidCache replaces Redis as the default caching solution in Rails 8, making it easier and more efficient to cache data directly in your database:
# app/controllers/products_controller.rb
class ProductsController < ApplicationController
def index
@products = Rails.cache.fetch("products", expires_in: 12.hours) do
Product.all
end
end
end
This simple caching system ensures that your app scales efficiently without adding external infrastructure.
4. Large Ecosystem and Vibrant Community
One of the standout benefits of using Ruby on Rails is its mature ecosystem and vibrant, supportive community.
Rails has been around for nearly two decades, and over the years, it has accumulated a wealth of tools, gems, and extensions that can accelerate your MVP development process.
For example, you can easily add authentication with Devise, manage file uploads with ActiveStorage, or enhance API development with GraphQL Ruby. The extensive ecosystem means that for almost any feature your MVP requires, there’s likely a well-documented gem to help you build it quickly.
Community and Ecosystem Benefits:
Thousands of gems: The Rails community has built countless gems that allow you to easily add functionality like user authentication, API management, and payment gateways.
Supportive community: Rails’ large community offers resources, tutorials, and forums where you can seek help and collaborate with other developers.
Established best practices: Rails follows well-established coding standards and practices, making it easier to build maintainable code that others can easily contribute to.
5. Maintainability and Flexibility
An MVP isn’t just about launching quickly—it’s about creating a product that you can easily improve and extend.
Ruby on Rails is designed for maintainability, and its architecture encourages developers to write clean, modular code.
Rails 8 introduces further enhancements to make sure that as your MVP grows, your codebase remains organized and easy to maintain.
The framework’s Model-View-Controller (MVC) architecture ensures that your business logic, presentation, and data are well-separated, making future updates and changes easier to implement.
Whether you need to pivot, add new features, or refactor parts of your app, Rails’ structure supports flexible and sustainable growth.
Maintainability Benefits:
Clear MVC structure: Rails enforces good architectural patterns, which lead to more maintainable code.
Easy refactoring: Rails' clear separation of concerns makes it simple to change or add features without disrupting the existing codebase.
Test-friendly: Rails has a strong testing culture and integrates well with testing tools like RSpec, helping you maintain code quality as your MVP evolves.
6. Cost-Effective Development
For startups and entrepreneurs, the cost of development can be a major concern when building an MVP.
Ruby on Rails 8 is an extremely cost-effective framework because it reduces the number of external services required (e.g., Redis, Sidekiq, or complex JavaScript frontends).
This not only lowers the infrastructure costs but also reduces the time needed to configure and maintain third-party systems.
With Rails 8’s built-in tools, developers can build robust, full-featured MVPs using a minimal number of external services, saving on hosting, infrastructure, and ongoing maintenance costs.
Cost Efficiency Benefits:
Fewer external services: SolidQueue, SolidCache, and SolidCable reduce the need for Redis and other external services, cutting down on hosting costs.
Single technology stack: By focusing on Rails, you avoid the costs associated with maintaining separate frontend (JavaScript) and backend stacks.
Free open-source tools: Most Rails gems and tools are open-source, eliminating licensing fees and reducing the overall development cost.
Conclusion: Ruby on Rails 8 is the Perfect Framework for MVPs
Whether you're a startup founder looking to validate a business idea or a developer tasked with delivering a fully functional MVP, Ruby on Rails 8 is the ideal framework for the job. Its speed, real-time capabilities (thanks to Hotwire), built-in scalability, and mature ecosystem make it a cost-effective and reliable choice.
By leveraging the latest features of Rails 8, such as SolidQueue, SolidCache, and SolidCable, you can build a robust MVP quickly without sacrificing performance or future scalability. With its balance of simplicity and power, Ruby on Rails 8 offers everything you need to take your MVP from concept to launch—and beyond.
Subscribe to my newsletter
Read articles from BestWeb Ventures directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

BestWeb Ventures
BestWeb Ventures
From cutting-edge web and app development, using Ruby on Rails, since 2005 to data-driven digital marketing strategies, we build, operate, and scale your MVP (Minimum Viable Product) for sustainable growth in today's competitive landscape.