The Best AI Models for JavaScript Developers - Use Cases, Examples, and Recommendations

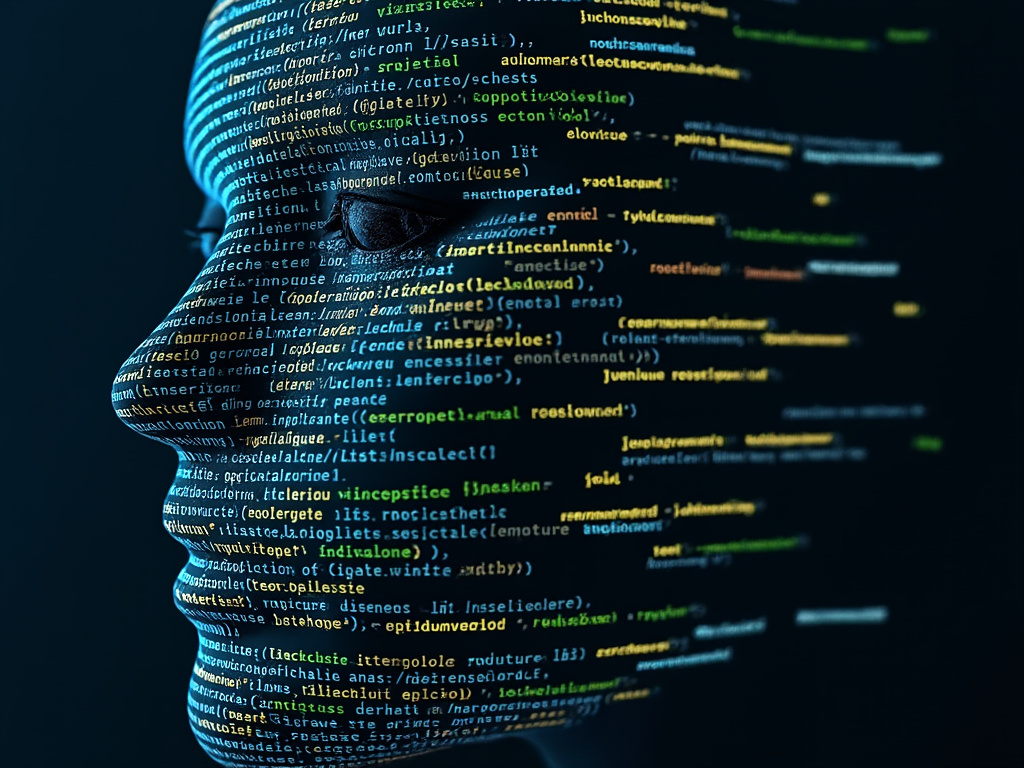
Artificial Intelligence (AI) is revolutionizing development, and JavaScript developers can leverage various AI models to enhance their projects. From generating text to creating images, AI models offer powerful capabilities that can be integrated into web and server-side applications. This guide explores some of the best AI models for JavaScript developers, including practical use cases and code examples.
1. GPT-4 (Generative Pre-trained Transformer 4)
Use Cases:
Chatbots: Build intelligent chatbots that can handle complex conversations.
Content Generation: Automatically generate text for blogs, articles, and more.
Code Assistance: Provide code suggestions or auto-completion.
Code Example:
Here’s how you can use GPT-4 with JavaScript using the openai
npm package:
const { Configuration, OpenAIApi } = require("openai");
// Set up the OpenAI API client
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
// Define the prompt
const prompt =
"Write a brief introduction to the best AI models for JavaScript developers.";
(async () => {
try {
const response = await openai.createCompletion({
model: "gpt-4",
prompt: prompt,
max_tokens: 100,
});
console.log(response.data.choices[0].text.trim());
} catch (error) {
console.error("Error fetching completion:", error);
}
})();
Pros:
High-quality text generation
Versatile and adaptable to various tasks
Cons:
Requires careful prompt engineering
Usage can be costly depending on the volume
Link: GPT-4
2. BERT (Bidirectional Encoder Representations from Transformers)
Use Cases:
Text Classification: Categorize user comments or feedback.
Named Entity Recognition (NER): Extract entities from user inputs or text data.
Sentiment Analysis: Determine the sentiment of user reviews or social media posts.
Code Example:
To use BERT in a JavaScript environment, you can interact with it via APIs such as Hugging Face’s hosted models. Here’s a sample code using fetch
to access a BERT-based model:
const fetch = require("node-fetch");
const apiUrl = "https://api-inference.huggingface.co/models/bert-base-uncased";
const headers = { Authorization: `Bearer YOUR_HUGGING_FACE_API_KEY` };
const inputText = "I love coding with AI!";
(async () => {
try {
const response = await fetch(apiUrl, {
method: "POST",
headers: headers,
body: JSON.stringify({ inputs: inputText }),
});
const result = await response.json();
console.log(result);
} catch (error) {
console.error("Error fetching BERT model:", error);
}
})();
Pros:
Effective for understanding the context in text
Versatile for various NLP tasks
Cons:
Requires API access for JavaScript environments
Can be resource-intensive
Link: BERT
3. DALL-E
Use Cases:
Image Generation: Create unique images from textual descriptions for web applications.
Marketing Materials: Generate visuals for promotional content.
Design Prototyping: Quickly visualize design ideas or concepts.
Code Example:
To generate images using DALL-E in JavaScript, you can use the openai
npm package:
const { Configuration, OpenAIApi } = require("openai");
// Set up the OpenAI API client
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
// Define the prompt for image generation
const prompt = "A futuristic cityscape with flying cars.";
(async () => {
try {
const response = await openai.createImage({
prompt: prompt,
n: 1,
size: "1024x1024",
});
console.log("Generated Image URL:", response.data.data[0].url);
} catch (error) {
console.error("Error generating image:", error);
}
})();
Pros:
High-quality and creative image generation
Useful for various visual content needs
Cons:
Access can be limited and expensive
Generated images may need refinement
Link: DALL-E
4. CLIP (Contrastive Language–Image Pre-training)
Use Cases:
Image Classification: Classify images based on text descriptions.
Visual Search: Improve search functionalities by matching images to textual queries.
Content Moderation: Automatically detect inappropriate content based on images and descriptions.
Code Example:
Using CLIP via an API with JavaScript:
const fetch = require("node-fetch");
const apiUrl =
"https://api-inference.huggingface.co/models/openai/clip-vit-base-patch32";
const headers = { Authorization: `Bearer YOUR_HUGGING_FACE_API_KEY` };
const imageUrl = "IMAGE_URL";
const textPrompt = "A beautiful sunset over the mountains";
(async () => {
try {
const response = await fetch(apiUrl, {
method: "POST",
headers: headers,
body: JSON.stringify({ image: imageUrl, text: textPrompt }),
});
const result = await response.json();
console.log(result);
} catch (error) {
console.error("Error fetching CLIP model:", error);
}
})();
Pros:
Strong performance on multi-modal tasks
Versatile integration for text and image data
Cons:
Setup can be complex
Large model size requires significant resources
Link: CLIP
Explore Basestack Platform
Ready to elevate your development experience even further? Check out Basestack ↗, our open-source stack designed specifically for developers and startups. BaseStack offers a comprehensive suite of tools, including Feature Flags, with more exciting tools like Feedback and Forms on the horizon.
Discover BaseStack:
Join our community and take advantage of the tools that can empower you to build exceptional products!
Subscribe to my newsletter
Read articles from Vitor Amaral directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vitor Amaral
Vitor Amaral
Hello! I am a skilled and motivated Full Stack Developer with a background in Management and Information Programming from Portugal 🇵🇹. Throughout my career, I have developed and managed a range of projects from start to finish, demonstrating my adaptability and strong communication skills. I have a diverse set of technical skills, including expertise in Serverless Computing, Cloud Management, and various forms of web, desktop, and mobile development such as React, Styled-Components, Svelte, Electron, WinForms, and React Native. In addition to these technical skills, I also have a strong understanding of UI/UX design principles and excellent problem-solving abilities.