Step-by-Step RSA Key Generation in JavaScript and Python for Beginners

Table of contents
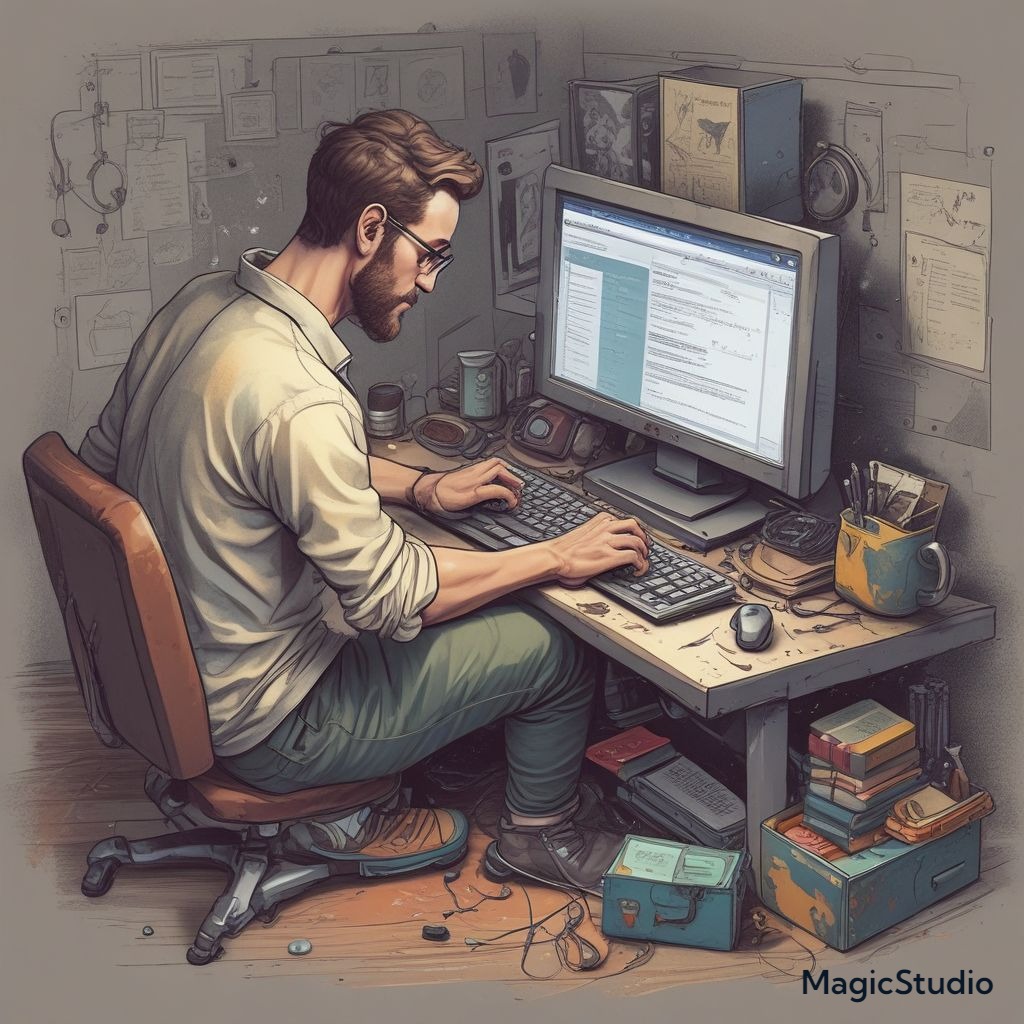
In today’s digital world, security is a top priority. One of the key technologies that help in securing data is RSA, an asymmetric cryptographic algorithm widely used for encryption, digital signatures, and secure data transmission. Generating RSA keys is the foundation of this system, as these keys are what keep your data safe. If you are a beginner looking to generate RSA key pairs in JavaScript or Python, this guide will walk you through the process in an easy-to-understand way.
What is RSA?
RSA (Rivest-Shamir-Adleman) is an asymmetric encryption algorithm. It uses two keys: a public key and a private key. The public key is used to encrypt data, while the private key is used to decrypt it. The keys are mathematically related, but the private key cannot be easily derived from the public key, making it a secure method for protecting sensitive information.
Why Use RSA?
Data encryption: RSA ensures secure communication between two parties.
Digital signatures: RSA helps verify the authenticity of a message or document.
Authentication: RSA enables secure user authentication in many applications.
Now that you understand the basics of RSA, let’s dive into how to generate RSA keys using JavaScript and Python.
Generating RSA Keys in OpenSSL (Command Line)
OpenSSL is a widely used tool to generate RSA keys.
To generate a 2048-bit RSA private key:
openssl genpkey -algorithm RSA -out private_key.pem -pkeyopt rsa_keygen_bits:2048
To extract the public key:
openssl rsa -pubout -in private_key.pem -out public_key.pem
This will create a private_key.pem
for the private key and public_key.pem
for the public key.
Generating RSA Keys in JavaScript
In JavaScript, you can use the crypto
module, which provides cryptographic functionalities, including generating RSA keys.
Step-by-Step Guide (JavaScript)
Set up your environment: First, ensure that you have Node.js installed on your machine. If not, download and install it from nodejs.org.
Use the
crypto
module: Thecrypto
module in Node.js allows you to generate key pairs using a simple function.
Here’s an example of how to generate a 2048-bit RSA key pair:
const { generateKeyPairSync } = require('crypto');
const { publicKey, privateKey } = generateKeyPairSync('rsa', {
modulusLength: 2048,
publicKeyEncoding: {
type: 'spki',
format: 'pem'
},
privateKeyEncoding: {
type: 'pkcs8',
format: 'pem'
}
});
console.log('Public Key:\n', publicKey);
console.log('Private Key:\n', privateKey);
Key Points:
The
modulusLength
option sets the key size, typically 2048 or 4096 bits for secure encryption.The public and private keys are returned in PEM format, which is widely used for storing keys.
With this simple script, you can generate your RSA key pair and use it for encryption, authentication, or digital signatures.
Generating RSA Keys in Python
Python is another popular language that allows you to generate RSA key pairs, and we’ll use the cryptography
library to do so.
Step-by-Step Guide (Python)
- Install the cryptography library: If you don’t have it installed yet, you can easily install it using
pip
:
pip install cryptography
- Generate the keys: Once the library is installed, use the following script to generate an RSA key pair.
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives.asymmetric import rsa
from cryptography.hazmat.primitives import serialization
private_key = rsa.generate_private_key(
public_exponent=65537,
key_size=2048,
backend=default_backend()
)
with open("private_key.pem", "wb") as private_file:
private_file.write(
private_key.private_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PrivateFormat.TraditionalOpenSSL,
encryption_algorithm=serialization.NoEncryption()
)
)
public_key = private_key.public_key()
with open("public_key.pem", "wb") as public_file:
public_file.write(
public_key.public_bytes(
encoding=serialization.Encoding.PEM,
format=serialization.PublicFormat.SubjectPublicKeyInfo
)
)
print("Keys generated and saved to files!")
Key Points:
This Python script generates a private key with a key size of 2048 bits and a public exponent of 65537, which is commonly used in RSA.
The private and public keys are stored in separate files in PEM format.
Understanding the Output
After running the scripts in both JavaScript and Python, you’ll have two files or variables:
Private Key: This key is kept secret and used for decryption or signing.
Public Key: This key can be shared and is used for encryption or verifying signatures.
These keys are usually stored in PEM format, which looks something like this:
-----BEGIN RSA PRIVATE KEY-----
MIIEpAIBAAKCAQEA...
-----END RSA PRIVATE KEY-----
How to Use the Keys?
Encryption/Decryption: You can use the public key to encrypt messages and the private key to decrypt them.
Signing/Verification: Sign a document with the private key and verify its authenticity using the public key.
Conclusion
Generating RSA key pairs is an essential task in many applications, whether you're building a secure web app, creating a digital signature, or encrypting sensitive data. In this guide, we’ve walked through simple examples in both JavaScript and Python. Using the crypto
module in Node.js and the cryptography
library in Python, you can easily generate secure RSA key pairs.
Key Takeaways:
RSA keys consist of a public key and a private key.
JavaScript’s
crypto
module and Python’scryptography
library make key generation easy.RSA key pairs can be used for encryption, digital signatures, and authentication.
By mastering the process of generating RSA keys, you can implement robust security features in your applications. Happy coding!
FAQs
Q: What is the recommended RSA key size for strong encryption?
A: A key size of at least 2048 bits is recommended for strong encryption. For even stronger security, 4096 bits can be used.
Q: Can I share my private key?
A: No, the private key should always be kept secure and never shared. Only the public key can be shared.
Q: What is the PEM format?
A: PEM (Privacy-Enhanced Mail) is a base64-encoded format commonly used for storing cryptographic keys.
This guide should now give you a solid understanding of generating RSA keys in both JavaScript and Python. Stay secure, and enjoy coding!
Subscribe to my newsletter
Read articles from Shubham Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shubham Khan
Shubham Khan
Full-Stack Software Engineer with over 3 years of experience in building scalable web applications using React, Spring Boot, and RDBMS. Proven ability to enhance system performance, increase efficiency, and build secure authentication systems. Skilled in developing and deploying applications with cloud services like AWS and Docker, while driving team collaboration through Agile methodologies.