Top Node.js Frameworks for 2025 - Powering the Future of Backend Development

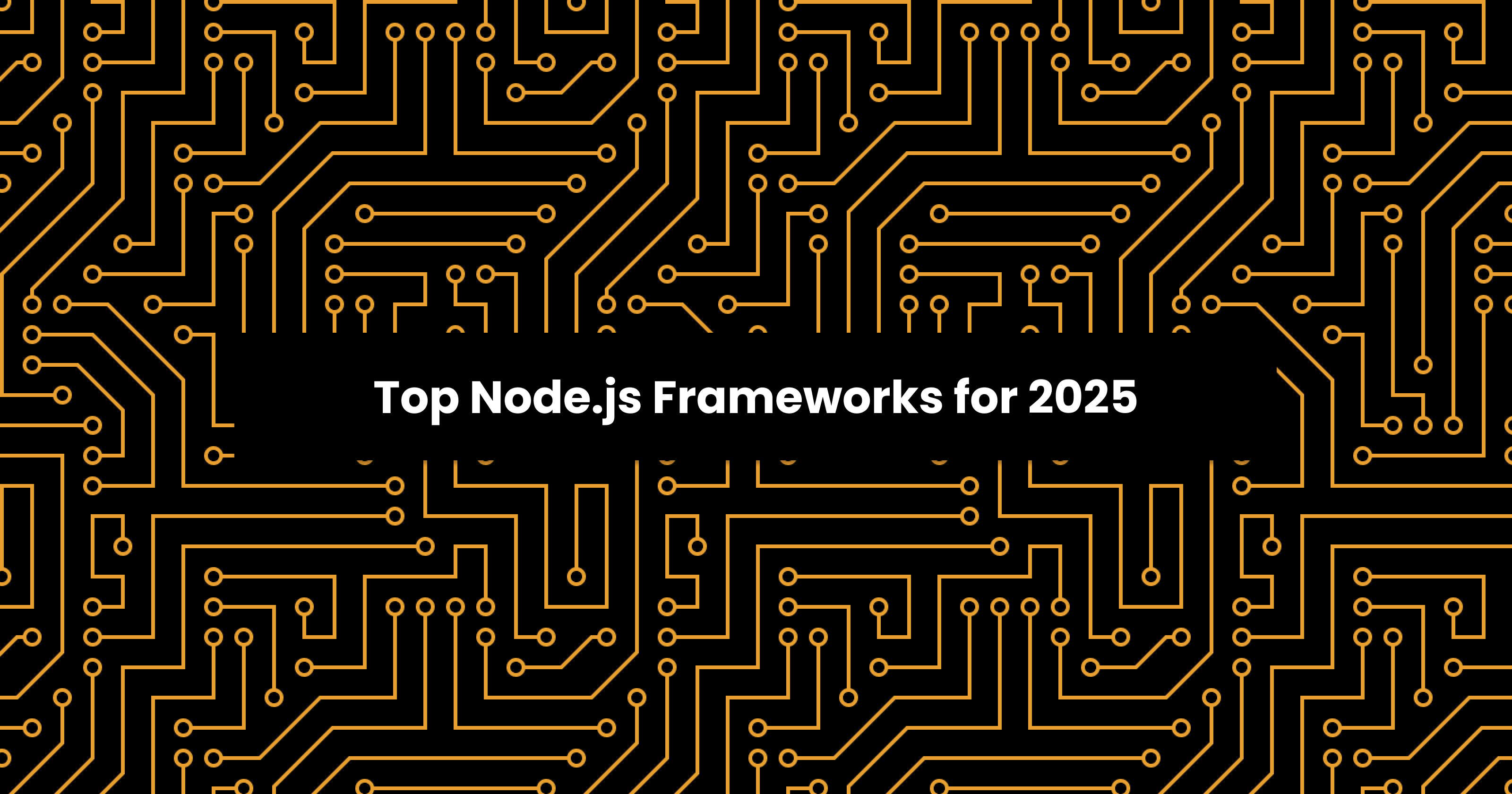
Node.js is the go-to for JavaScript developers building modern backend solutions, offering speed, scalability, and a vibrant ecosystem. But what really elevates Node.js is its wide variety of frameworks—tools that simplify development, improve performance, and offer specialized features. As we head into 2025, let's explore the top frameworks you should consider, and why they matter for your next big project.
What Exactly Are Node.js Frameworks?
Node.js frameworks are pre-built, modular code structures that provide developers with tools to build server-side applications more easily and efficiently. Think of them as the "building blocks" for creating web apps, APIs, and real-time services. By automating common tasks—like routing, middleware integration, and database interaction—these frameworks save you from reinventing the wheel with every new project.
Why Should You Use a Node.js Framework?
With so many libraries and packages already available in Node.js, why bother with a framework? Here are a few key reasons:
Time Efficiency: Frameworks offer out-of-the-box functionality, enabling you to jump straight into coding without worrying about the boilerplate setup.
Scalability: Popular frameworks are optimized for handling large-scale applications with high traffic, so you don’t have to build scalability from scratch.
Security: Many frameworks come with built-in security features like request validation, user authentication, and more, reducing the risk of common vulnerabilities.
Maintainability: Frameworks are designed with best practices in mind, making your code cleaner and more maintainable in the long run.
Best Node.js Frameworks to Use in 2025
1. Express.js: The Classic, No-Nonsense Framework
Why it's popular:
Express.js is the backbone of the Node.js ecosystem. It’s fast, unopinionated, and gives developers full control over their projects. With a minimalist approach, Express is perfect for developers who want a flexible structure without being locked into specific patterns.
Key Features:
Simple routing system
Minimalistic and highly extensible
Huge ecosystem of middleware
When to use it:
Express is perfect for APIs, microservices, and small to medium-sized applications. Its flexibility lets you integrate other libraries as needed, making it a great choice for developers who want full control.
const express = require("express");
const app = express();
app.get("/", (req, res) => {
res.send("Hello World!");
});
app.listen(3000, () => {
console.log("Server is running on port 3000");
});
2. NestJS: The Modern, Enterprise-Ready Framework
Why it stands out:
NestJS takes a unique approach to backend development by bringing a structured, opinionated framework to Node.js—much like Angular for frontend. Built with TypeScript, it’s a powerful tool for large-scale enterprise applications, offering features like dependency injection, module-based architecture, and built-in testing utilities.
Key Features:
Modular architecture for scalability
TypeScript out of the box
Built-in support for WebSockets, GraphQL, and Microservices
When to use it:
NestJS is ideal for large enterprise applications or when you need a structured, opinionated framework that’s ready for complex features like GraphQL or WebSockets.
import { Controller, Get } from "@nestjs/common";
@Controller()
export class AppController {
@Get()
getHello(): string {
return "Hello Nest!";
}
}
3. Fastify: The Speed Demon
Why it’s a rising star:
Fastify’s main claim to fame is its speed. Built with performance in mind, it promises low overhead and high throughput, making it perfect for building blazing-fast APIs. With an emphasis on schema-based validation, Fastify is also great for ensuring that your data is always in the right format.
Key Features:
Extremely fast (up to 20-30% faster than Express)
Schema-based validation
Optimized for HTTP/2
When to use it:
Fastify is the go-to framework when performance is a priority, especially in microservice architectures or for applications where speed is critical.
const fastify = require("fastify")({ logger: true });
fastify.get("/", async (request, reply) => {
return { message: "Hello Fastify!" };
});
fastify.listen(3000, (err, address) => {
if (err) throw err;
console.log(`Server running at ${address}`);
});
4. Koa.js: The Lightweight Alternative to Express
Why it’s a solid choice:
Koa.js, developed by the creators of Express, is a lightweight and more modern take on server-side development. It avoids the callbacks found in Express by leveraging async/await, leading to cleaner and more manageable code.
Key Features:
Async/await support for cleaner code
No middleware by default, allowing complete control
Lightweight and minimalistic
When to use it:
Koa.js is great for developers who love the simplicity of Express but want a more modern and lightweight framework. It's ideal for building small to medium-sized applications that don’t need the overhead of a larger framework.
const Koa = require("koa");
const app = new Koa();
app.use(async ctx => {
ctx.body = "Hello Koa!";
});
app.listen(3000);
5. AdonisJS: The Laravel of Node.js
Why it’s a strong contender:
AdonisJS is often called the "Laravel of Node.js" for its elegant syntax, powerful ORM, and all-in-one development experience. It’s a fully-fledged MVC framework that comes with everything you need out of the box—from routing to middleware, to ORM and authentication. If you're looking for a framework that helps you focus on building features rather than worrying about infrastructure, AdonisJS might be for you.
Key Features:
Fully-fledged MVC framework
Built-in ORM (Lucid)
Simple yet powerful routing and middleware
Out-of-the-box authentication and validation
When to use it:
AdonisJS is perfect for developers who want an all-in-one, batteries-included framework for building robust applications quickly. It’s ideal for projects where you want the simplicity of Laravel but with the performance and flexibility of Node.js.
import Route from "@ioc:Adonis/Core/Route";
Route.get("/", async ({ response }) => {
response.send("Hello from AdonisJS!");
});
How to Pick the Best Node.js Framework for 2025
With so many great options, choosing the right framework can feel overwhelming. Here's how you can make the best decision:
Project Requirements: Are you building a fast API, a real-time app, or a large-scale enterprise application? Each framework excels in different areas.
Community Support: Look at the size of the community and available resources. A large, active community can make a big difference when you run into challenges.
Performance Needs: If speed is critical, frameworks like Fastify should be high on your list.
Learning Curve: Consider how much time you’re willing to invest. Express is easy to learn, while NestJS has a steeper learning curve but offers advanced features.
Wrapping Up
Node.js frameworks continue to evolve, and 2025 is shaping up to be an exciting year for backend development. Whether you need the flexibility of Express, the speed of Fastify, or the structured approach of NestJS and AdonisJS, there’s a framework tailored to your needs. Experiment with a few, assess their performance, and see which one fits your next project best!
Explore BaseStack Platform
Ready to elevate your development experience even further? Check out Basestack ↗, our open-source stack designed specifically for developers and startups. BaseStack offers a comprehensive suite of tools, including Feature Flags, with more exciting tools like Feedback and Forms on the horizon.
Discover BaseStack:
Join our community and take advantage of the tools that can empower you to build exceptional products!
Subscribe to my newsletter
Read articles from Vitor Amaral directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vitor Amaral
Vitor Amaral
Hello! I am a skilled and motivated Full Stack Developer with a background in Management and Information Programming from Portugal 🇵🇹. Throughout my career, I have developed and managed a range of projects from start to finish, demonstrating my adaptability and strong communication skills. I have a diverse set of technical skills, including expertise in Serverless Computing, Cloud Management, and various forms of web, desktop, and mobile development such as React, Styled-Components, Svelte, Electron, WinForms, and React Native. In addition to these technical skills, I also have a strong understanding of UI/UX design principles and excellent problem-solving abilities.