Get Started with GraphQl
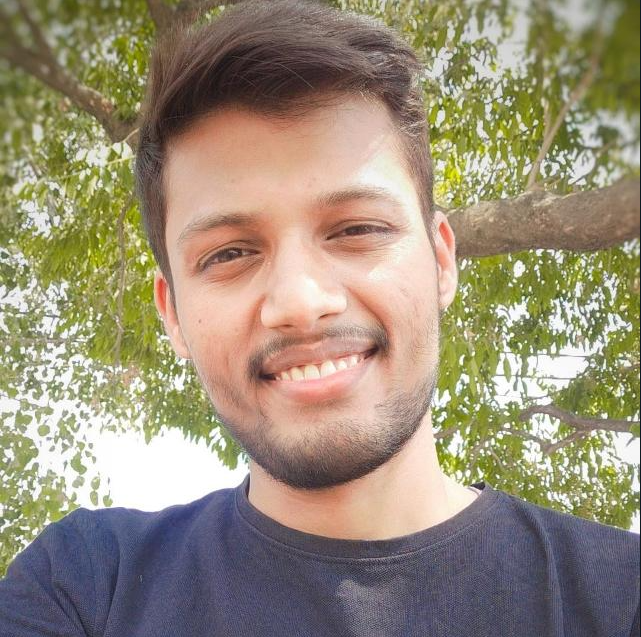
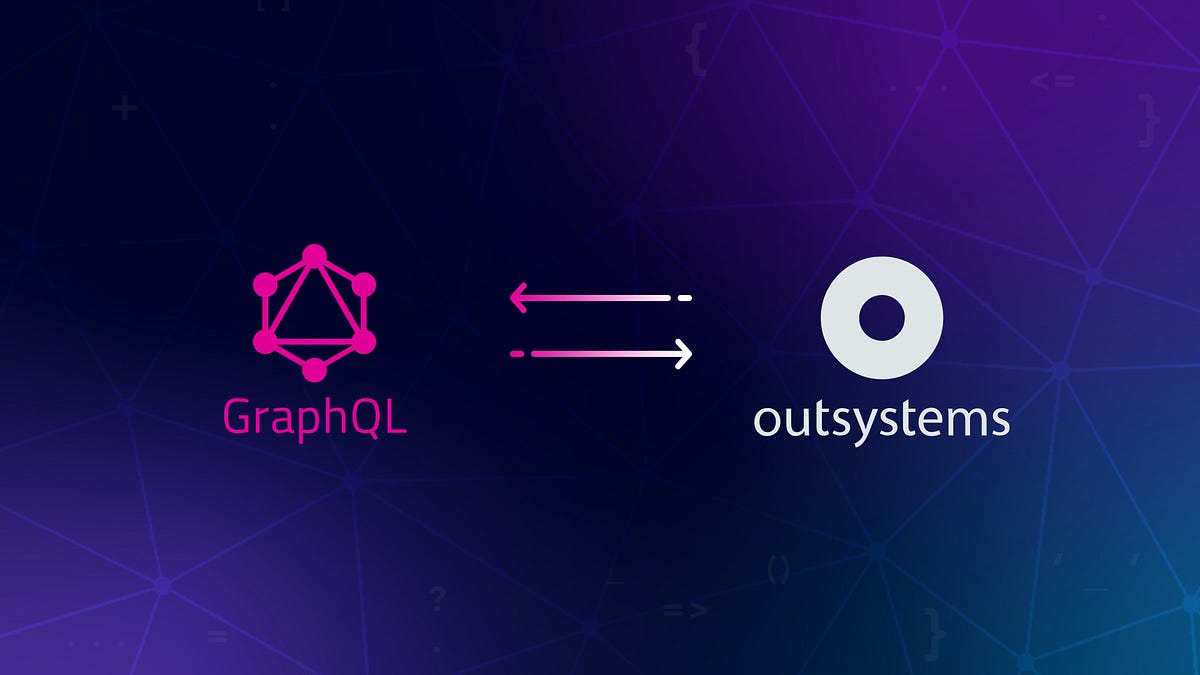
Unleash the Power of GraphQL in Next.js 14 with TypeScript!
Hey everyone, it's Aman!
I recently ran into some issues getting started with GraphQL in Next.js 14. Most of the blogs I found were outdated, focusing on Next.js 13. So, I decided to give it a shot myself and share my experience—hope you find it helpful!
First things first, make sure you're running Node.js 16 or higher. Then, create a new Next.js app with the command below. Don't forget to select "Yes" for TypeScript—we’ll be using it throughout this guide.
npx create-next-app@latest [project-name] [options]
If you hit any snags, don't worry—you can always check out the official Next.js docs for solutions. Nextdocs
Now, let's get into the fun part! To kick things off with GraphQL, you’ll need to install a few dependencies. Here’s the list to get you started:
npm i @apollo/client
npm i @apollo/server
So your whole app needed to wrap with apolloWrapper , but first you have to make it ,below is the apolloprovider.tsx file.
"use client";
import { ApolloClient, ApolloProvider, InMemoryCache } from "@apollo/client";
import { useState } from "react";
export function ApolloWrapper({ children }: { children: React.ReactNode }) {
const [client] = useState(
new ApolloClient({
uri: "/api/playarea/graphql",
cache: new InMemoryCache(),
})
);
return <ApolloProvider client={client}>{children}</ApolloProvider>;
}
Now, you'll need to wrap your layout.tsx
file with the following code. This is how your layout.tsx
file should look:
import { ApolloWrapper } from "@/components/playcomponent/ApolloWrapper";
export default function PlayAreaLayout({
children,
}: {
children: React.ReactNode;
}) {
return <ApolloWrapper>{children}</ApolloWrapper>;
}
In the ApolloProvider.tsx
file, you might have noticed the uri
parameter. This is the endpoint where your GraphQL API calls will be sent. Keep in mind, the URI can vary depending on your setup, but it essentially defines the route for your API requests. Make sure to customize it to match your backend.
Now, we’re ready to write the API! In GraphQL, there are two core concepts to keep in mind: schema and query. The schema defines your data structure, while the query is how you fetch that data. When someone calls the API, they interact with the query to retrieve what they need. Let’s take a look at the code to see how it all comes together.
import { ApolloServer } from "@apollo/server";
import { startServerAndCreateNextHandler } from "@as-integrations/next";
import { gql } from "graphql-tag";
import Users from "@/models/user";
import { connectDb } from "@/util/db";
const typeDefs = gql`
type User {
id: ID!
name: String!
email: String!
phone:String!
}
type Query {
hello: String
users: [User!]!
user(id: ID!): User
}
`;
const resolvers = {
Query: {
hello: () => "Hello from GraphQL!",
users: async () => {
await connectDb();
const users = await Users.find();
return users.map((user) => {
if (!user._id) {
console.error("User without an ID found:", user);
return {
id: "N/A",
name: user.name || "Unknown",
email: user.email || "Unknown",
phone: user.phone || "N/A",
gender: user.gender || "N/A",
};
}
return {
id: user._id.toString(),
name: user.name,
email: user.email,
phone: user.phone || "N/A",
gender: user.gender,
};
});
},
},
};
const server = new ApolloServer({
typeDefs,
resolvers,
});
export const POST = startServerAndCreateNextHandler(server);
This code sets up a basic GraphQL API using Apollo Server in Next.js. First, we define a schema (using typeDefs
) that outlines a User
type and two GraphQL queries: hello
, which simply returns a greeting, and users
, which fetches all users from a MongoDB database.
Resolvers connect the queries to their logic, like fetching users from the database, handling missing fields, and formatting user IDs. The ApolloServer
is initialized with the schema and resolvers, and finally, a handler is created to process POST requests using startServerAndCreateNextHandler
In this code I have startServerAndCreateNextHandler
that manage the post call you don’t need that I am copy pasting this via my existing project that’s why it is here , you can go with the get call to get the user data.
So, we’ve successfully created our first GraphQL API! Now, it’s time to build the frontend to consume this API. Below is the frontend code where you'll fetch data from the API and display it.
We’ll use Apollo Client to interact with the GraphQL server efficiently, leveraging caching for fast, smooth interactions. Let’s dive into the frontend implementation to get the most out of our API!
"use client";
import { gql, useQuery } from "@apollo/client";
import Loader from "../loader";
const GET_USERS_QUERY = gql`
query {
users {
id
name
email
phone
gender
}
}
`;
interface User {
id: string;
name: string;
email: string;
phone: string;
gender: string;
}
export default function TestQuery() {
const { loading, error, data } = useQuery(GET_USERS_QUERY, {
fetchPolicy: "cache-first",
});
if (loading)
return (
<div>
<Loader />
</div>
);
if (error) return <p>Error: {error.message}</p>;
console.log(data, "Data will print here");
return (
<div>
<h2>User List</h2>
<ul>
{data?.users?.map((user: User) => (
<li key={user.id}>
<p>Name: {user.name}</p>
<p>Email: {user.email}</p>
<p>Phone: {user.phone}</p>
<p>Gender{user.gender}</p>
</li>
))}
</ul>
</div>
);
}
If you run into any issues during the process or have questions, feel free to reach out! I’m happy to help make the journey smoother for you.
Be liberal in what you accept, and conservative in what you send" was a bad idea for protocols , keep ion mind…
Subscribe to my newsletter
Read articles from Aman Trivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
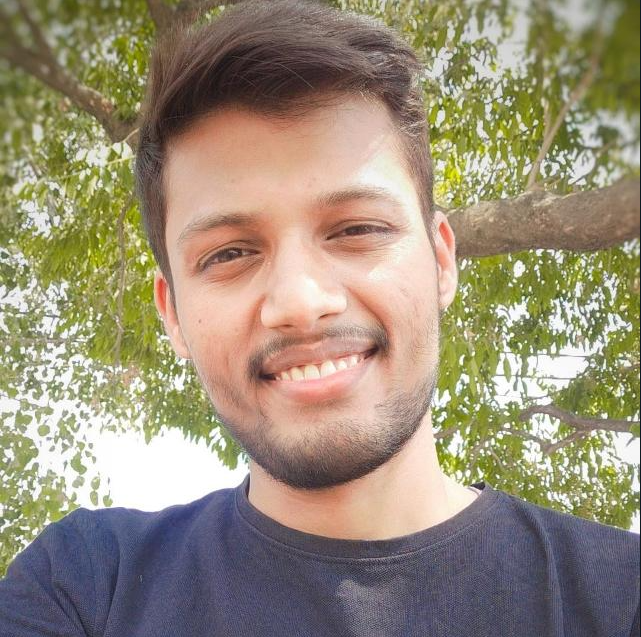