Basic CRUD Python Flask API
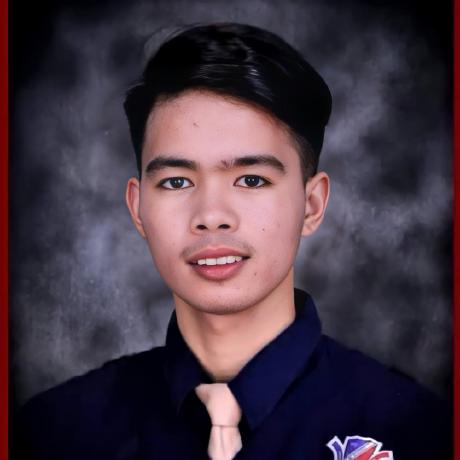
Prerequisites
https://software-engineer.thirdygayares.com/virtual-environment-and-python-package-manager
https://software-engineer.thirdygayares.com/how-to-run-python-flask
https://software-engineer.thirdygayares.com/data-structure-again
To start,
Create a Python Virtual Environment
Activate Virtual Environment
make sure Flask is installed:
pip install Flask
BLUEPRINT
OUR BLUEPRINT
# TODO: import flask LIBRARY
from flask import Flask, jsonify, request
# call the flask object
app = Flask(__name__)
# create list of dictionaries
students = [{}]
# get method
@app.route('/students', methods=['GET'])
def get_students():
# get method : Get a specific student by ID
@app.route('/students/<int:student_id>', methods=['GET'])
def get_student(student_id):
# put method
@app.route('/students/<int:student_id>', methods=['PUT'])
def update_student(student_id):
# delete method
@app.route('/students/<int:student_id>', methods=['DELETE'])
def delete_student(student_id):
# Dont forget this
if __name__ == '__main__':
app.run(debug=True)
When Testing the API
Run the server by executing the Python script:
python app.py
Flask API Code
create app.py
import flask
from flask import Flask, jsonify, request
app = Flask(__name__)
Create a List of Dictionaries
# Sample data: list of students
students = [
{
"name": "Juan Carlos",
"section": "BSIT-4B",
"id": 1,
"email": "juan@gmail.com",
"age": 22
},
{
"name": "Jose Rizal",
"section": "BSIT-2A",
"id": 2,
"email": "jose@gmail.com",
"age": 21
},
{
"name": "Juan Luna",
"section": "BSIT-3A",
"id": 3,
"email": "juan@gmail.com",
"age": 20
},
{
"name": "Andres Bonifacio",
"section": "BSIT-3A",
"id": 4,
"email": "andres@gmail.com",
"age": 20
},
{
"name": "Justin Bieber",
"section": "BSIT-2A",
"id": 5,
"email": "justin@gmail.com",
"age": 21
},
{
"name": "Michael Jordan",
"section": "BSIT-4A",
"id": 6,
"email": "michael@gmail.com",
"age": 19
},
{
"name": "Andrew Jordan",
"section": "BSIT-3A",
"id": 7,
"email": "andrew@gmail.com",
"age": 20
},
{
"name": "Jessa Boe",
"section": "BSIT-2B",
"id": 8,
"email": "jessa@gmail.com",
"age": 18
},
{
"name": "Ted Talk",
"section": "BSIT-3B",
"id": 9,
"email": "ted@gmail.com",
"age": 19
}
]
Get All Student
@app.route('/students', methods=['GET'])
def get_students():
return jsonify(students)
Get All Students (GET /students
): Returns a list of all students in JSON format.
GET STUDENT BY ID
# READ: Get a specific student by ID
@app.route('/students/<int:student_id>', methods=['GET'])
def get_student(student_id):
student = next((student for student in students if student["id"] == student_id), None)
if student:
return jsonify(student)
else:
return jsonify({"message": "Student not found"}), 404
Get a Specific Student (GET /students/<id>
): Returns details of a student by their id
.
ADD STUDENT
@app.route('/students', methods=['POST'])
def add_student():
new_student = request.get_json()
new_student['id'] = max(student['id'] for student in students) + 1 # Generate new ID
students.append(new_student)
return jsonify(new_student), 201
Add a New Student (POST /students
): Accepts student details in JSON format and adds a new student with a unique id
.
UPDATE STUDENT
@app.route('/students/<int:student_id>', methods=['PUT'])
def update_student(student_id):
student = next((student for student in students if student["id"] == student_id), None)
if student:
data = request.get_json()
student.update(data)
return jsonify(student)
else:
return jsonify({"message": "Student not found"}), 404
Update an Existing Student (PUT /students/<id>
): Updates the details of a student by their id
using JSON data from the request.
# DELETE: Remove a student by ID
@app.route('/students/<int:student_id>', methods=['DELETE'])
def delete_student(student_id):
student = next((student for student in students if student["id"] == student_id), None)
if student:
students.remove(student)
return jsonify({"message": "Student deleted"})
else:
return jsonify({"message": "Student not found"}), 404
if __name__ == '__main__':
app.run(debug=True)
REMEMBER ADD THIS CODE
if __name__ == '__main__':
app.run(debug=True)
Delete a Student (DELETE /students/<id>
): Deletes a student by their id
.
- Using Postman, you can test each endpoint by sending the appropriate requests.
This API provides a simple and clean structure to manage students' data with CRUD operations.
Subscribe to my newsletter
Read articles from Thirdy Gayares directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
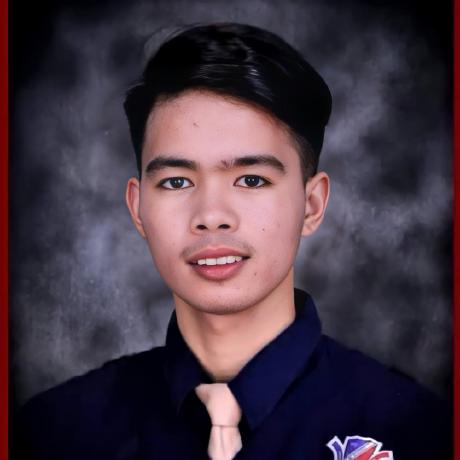
Thirdy Gayares
Thirdy Gayares
I am a dedicated and skilled Software Engineer specializing in mobile app development, backend systems, and creating secure APIs. With extensive experience in both SQL and NoSQL databases, I have a proven track record of delivering robust and scalable solutions. Key Expertise: Mobile App Development: I make high-quality apps for Android and iOS, ensuring they are easy to use and work well. Backend Development: Skilled in designing and implementing backend systems using various frameworks and languages to support web and mobile applications. Secure API Creation: Expertise in creating secure APIs, ensuring data integrity and protection across platforms. Database Management: Experienced with SQL databases such as MySQL, and NoSQL databases like Firebase, managing data effectively and efficiently. Technical Skills: Programming Languages: Java, Dart, Python, JavaScript, Kotlin, PHP Frameworks: Angular, CodeIgniter, Flutter, Flask, Django Database Systems: MySQL, Firebase Cloud Platforms: AWS, Google Cloud Console I love learning new things and taking on new challenges. I am always eager to work on projects that make a difference.